Testing InputException Messages in Magento 2
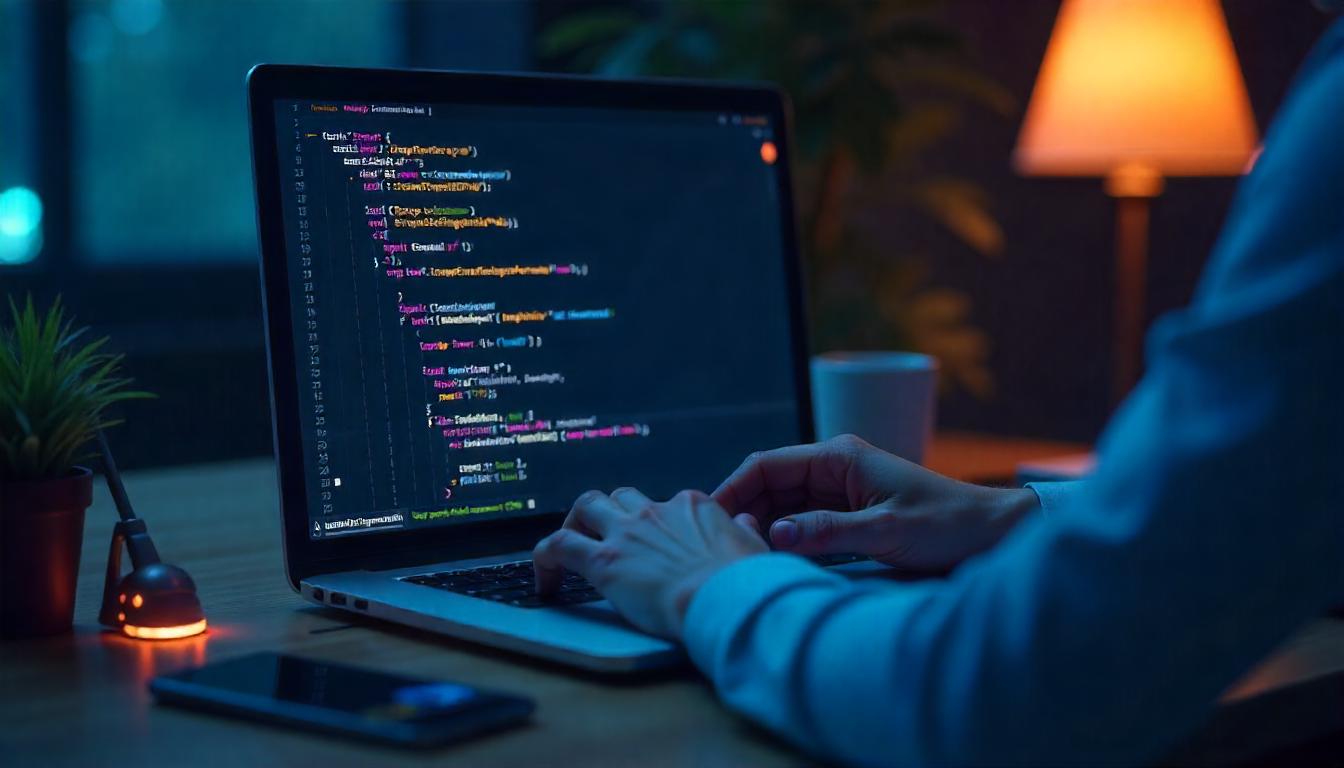
Testing InputException Messages in Magento 2
To test InputException error messages in Magento 2, you can write unit tests using PHPUnit's assertEquals() and assertStringMatchesFormat() methods. These tests ensure that your exception messages are accurate and consistent.
Table Of Content
Testing InputException Messages in Magento 2
When a method throws an InputException
, it's crucial to verify that the error message is correctly formatted. Here's how you can write a unit test for this scenario:
<?php
declare(strict_types=1);
namespace Vendor\Module\Test\Unit;
use Magento\Framework\Exception\InputException;
use Magento\Framework\Phrase;
use PHPUnit\Framework\TestCase;
class InputExceptionTest extends TestCase
{
public function testInputExceptionMessage(): void
{
$params = ['ExtraBlock'];
$inputException = new InputException(
new Phrase('Invalid block name: %1', $params)
);
$this->assertEquals(
'Invalid block name: %1',
$inputException->getRawMessage()
);
$this->assertEquals(
'Invalid block name: ExtraBlock',
$inputException->getMessage()
);
$this->assertEquals(
'Invalid block name: %1',
$inputException->getLogMessage()
);
}
}
In this test:
getRawMessage()
returns the unformatted message with placeholders.getMessage()
returns the formatted message with actual values.getLogMessage()
is typically used for logging purposes and often matches the raw message.
Additional Tips
- Use Descriptive Messages: Ensure your exception messages clearly describe the error to aid in debugging.
- Test All Exception Methods: Besides
getMessage()
, consider testing other relevant methods likegetRawMessage()
andgetLogMessage()
. - Maintain Consistency: Keep your exception messages consistent across your application for better maintainability.
By thoroughly testing your exception messages, you can ensure that your Magento 2 application provides clear and consistent error information, which is vital for both developers and users.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What Is an InputException in Magento 2?
InputException
is used to handle invalid input values in Magento 2. It's part of the framework's standardized way to raise clear, consistent error messages.
When Should I Use InputException?
Use InputException
when your method receives invalid or missing input that prevents it from continuing. It clearly signals an issue with the user's data.
How Do I Throw an InputException in My Code?
You can throw it like this: throw new InputException(__('Invalid block name: %1', $blockName));
. The %1
is replaced with the actual value passed to the message.
How Can I Write a Unit Test for InputException?
Create a PHPUnit test and use assertEquals()
or assertStringMatchesFormat()
to verify the exception message. This ensures that your exception provides accurate information.
What’s the Difference Between getMessage() and getRawMessage()?
getMessage()
returns the fully formatted message with values inserted. getRawMessage()
returns the original string with placeholders like %1
.
What Should I Assert in the Unit Test?
Assert that getRawMessage()
, getMessage()
, and getLogMessage()
return expected outputs. This verifies the exception is built and logged correctly.
Can I Use assertStringMatchesFormat for Dynamic Values?
Yes. It's helpful when the message contains dynamic content like a block name. Use it to validate the format while allowing variable data.
Where Should the Test File Be Located?
Place your test class inside your module’s Test/Unit
directory. Magento 2 recognizes and runs unit tests from this location.
Do I Need to Mock Anything in This Test?
Not usually for InputException
. You’re testing the message, so mocking is not required unless the message comes from another class or service.
Where Can I Learn More About Magento 2 Exception Handling?
Visit Magento’s official devdocs or search trusted blog tutorials. They provide details on exception types, message translation, and best practices.