How to Avoid the "Use of $_FILES is Discouraged in Magento 2" Warning
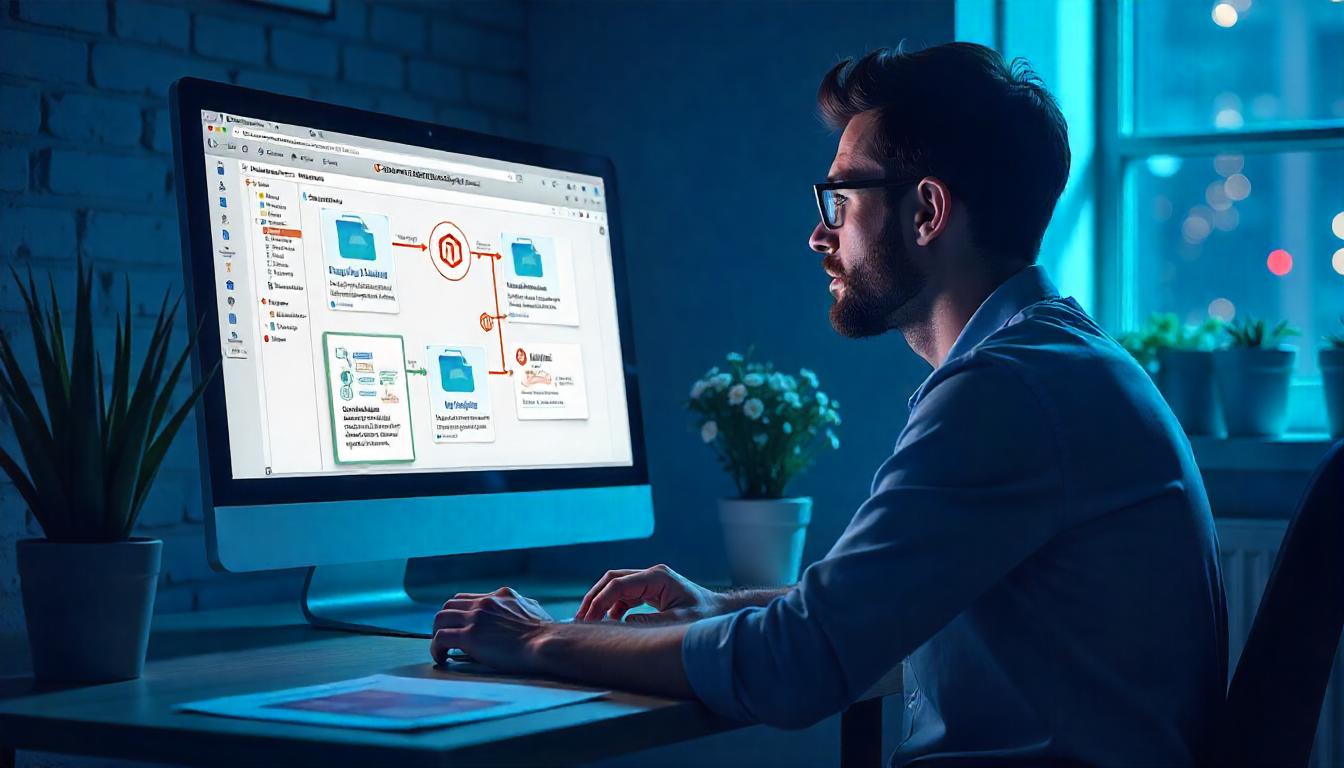
How to Avoid the "Use of $_FILES is Discouraged in Magento 2" Warning
Magento 2 discourages the direct use of the $_FILES superglobal due to security concerns and coding standards. If you're encountering the "Use of $_FILES is discouraged in Magento 2" warning, it's likely because tools like GrumPHP are flagging this practice during code commits.
How to Avoid the "Use of $_FILES is Discouraged in Magento 2" Warning
Why This Warning Occurs
Magento's coding standards prohibit the direct use of PHP superglobals such as $_FILES
, $_POST
, $_GET
, and others. This restriction aims to prevent potential security vulnerabilities and maintain code consistency. When these superglobals are used directly, tools like GrumPHP will flag them as errors.
Recommended Approach: Use Magento's Request Class
Instead of accessing $_FILES directly
, Magento provides a safer method through the Magento\Framework\App\Request\Http
class. This approach aligns with Magento's standards and ensures better security and maintainability.
Implementation Example
To handle file uploads correctly, inject the Http request class into your constructor:
use Magento\Framework\App\Request\Http;
class FileUploader
{
protected $request;
public function __construct(Http $request)
{
$this->request = $request;
}
public function uploadFile()
{
$files = $this->request->getFiles();
if (isset($files['my_file_field']['name'])) {
// Process the uploaded file
}
}
}
Key Points
- Avoid using
$_FILES
directly in your code. - Use
$this->request->getFiles()
to retrieve uploaded files. - Ensure your code passes GrumPHP checks by adhering to Magento's coding standards.
Additional Tips
- If you're using GrumPHP, ensure it's properly configured to catch such issues before commits.
- Regularly review Magento's coding standards to keep your codebase secure and compliant.
By following these practices, you can prevent the "Use of $_FILES is discouraged in Magento 2" warning and maintain a secure and standard-compliant codebase.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What Does the "Use of $_FILES is Discouraged in Magento 2" Warning Mean?
This warning appears when you directly use the $_FILES
superglobal in your code, which is discouraged by Magento 2’s coding standards due to potential security risks and poor maintainability.
Why Is Using $_FILES Directly Not Recommended in Magento 2?
Using $_FILES
directly exposes your application to security vulnerabilities. Magento 2 encourages using the Request
class instead, which improves data handling and adheres to coding standards.
How Can I Resolve the "Use of $_FILES is Discouraged" Warning?
To resolve this warning, replace direct usage of $_FILES
with $this->request->getFiles()
from the Magento\Framework\App\Request\Http
class.
How Do I Use the Magento 2 Request Class to Handle File Uploads?
Inject the Request
class into your constructor and use $this->request->getFiles()
to get uploaded files. This method returns files in a format similar to $_FILES
but in a safer, more standardized way.
Can You Show an Example of Using the Request Class for File Uploads?
Sure! Here’s an example:
use Magento\Framework\App\Request\Http;
class FileUploader
{
protected $request;
public function __construct(Http $request)
{
$this->request = $request;
}
public function uploadFile()
{
$files = $this->request->getFiles();
if (isset($files['my_file_field']['name'])) {
// Process the uploaded file
}
}
}
What Are the Benefits of Using $this->request->getFiles() Over $_FILES?
Using $this->request->getFiles()
follows Magento's best practices, ensures better security, and avoids triggering warnings from tools like GrumPHP. It also keeps your code cleaner and more compliant with Magento's standards.
How Can I Test If My Code Is Using $_FILES Correctly?
Use a static code analysis tool like GrumPHP to check for direct usage of $_FILES
in your code. If flagged, update the code to use $this->request->getFiles()
.
Can I Use $_FILES in Magento 2 If I Need to?
While it's possible, it’s not recommended. Magento's framework offers better alternatives, like the Request
class, which should be used to ensure code security and compliance with Magento's standards.
Where Can I Learn More About Magento 2 Best Practices for Handling File Uploads?
Check out Magento’s official documentation and community forums for best practices on file handling, security, and coding standards. Blogs and tutorials on trusted Magento resources also provide detailed guidance.