How to Retrieve Payment Gateway Token from Vault Payment Orders in Magento 2.4.7
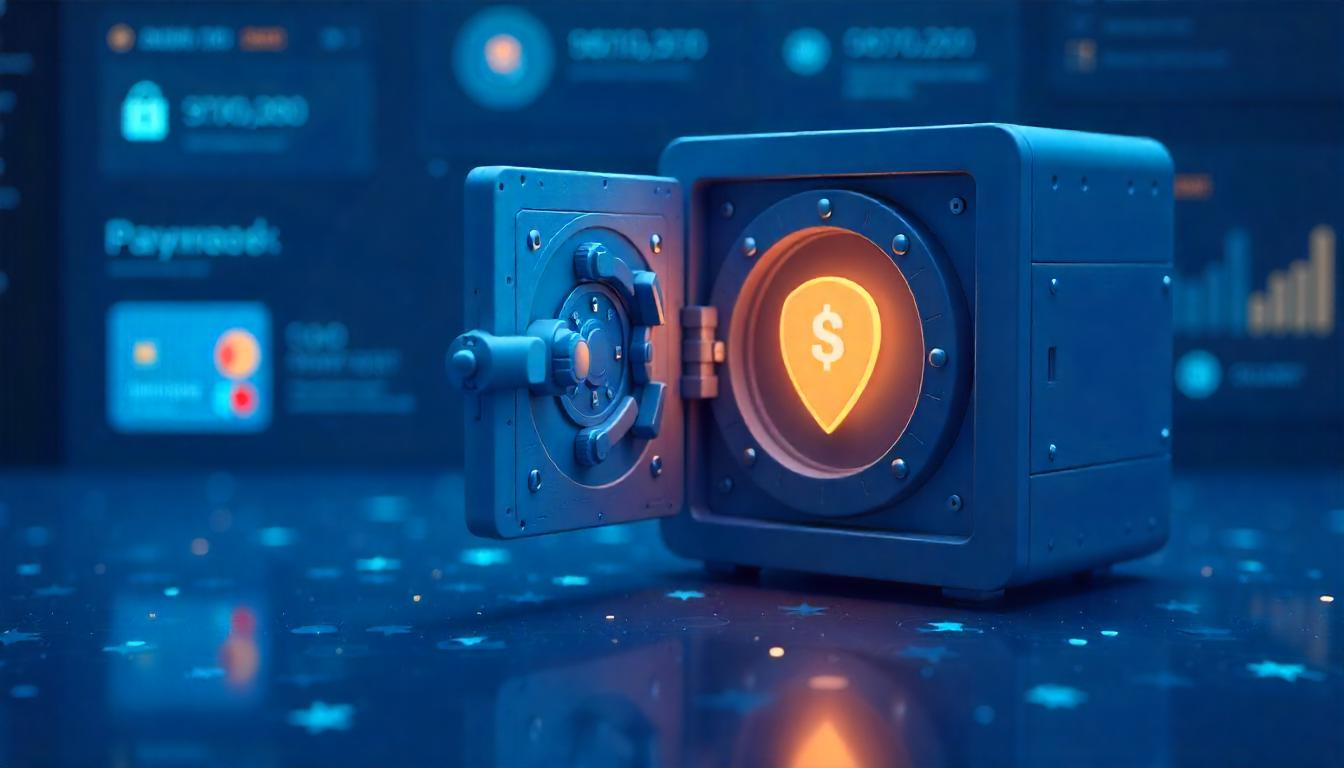
How to Retrieve Payment Gateway Token from Vault Payment Orders in Magento 2.4.7
In Magento 2.4.7, the Vault Payment feature allows customers to securely save their payment details for future purchases. This is particularly useful for improving checkout speed and enabling recurring transactions. When Vault is enabled and a customer chooses to save their credit card information, Magento stores a reference to the Payment Gateway Token associated with the transaction. This token can be retrieved from the order and used for backend operations like refunds, reauthorization, or billing.
Table Of Content
Magento 2 Vault Overview
Introduction to Vault in Magento
Magento 2 Vault is a secure system designed to handle tokenized payment data. It allows merchants to store payment method tokens instead of sensitive card information, which helps maintain security and compliance. This system is especially useful when working with payment gateways that support tokenization, such as Braintree, Authorize.Net, and others.
Vault is integrated into Magento’s core payment framework and provides seamless handling of saved payment methods for both frontend customers and backend operations.
Why Use Magento Vault?
Using Vault significantly improves the payment experience while maintaining the integrity of security standards. It is particularly beneficial for stores with repeat customers or subscription-based services.
Core Features and Benefits of Magento Vault
Feature | Description |
---|---|
Secure Tokenization | Replaces raw credit card data with secure tokens provided by payment gateways, ensuring sensitive data never touches the Magento server. |
Fast Checkout | Enables returning customers to complete purchases faster using their saved payment methods. |
PCI Compliance | Supports adherence to PCI-DSS standards by minimizing the exposure of sensitive payment data. |
Token Management | Customers can access, view, and delete their saved cards directly from their account dashboard. |
Developer Integration | Offers developers APIs and interfaces to work with tokens programmatically. |
Gateway Compatibility | Compatible with major payment providers that support token-based processing. |
Additional Considerations
- User Experience: Vault greatly improves customer convenience and checkout speed, increasing conversion rates.
- Security: As tokenization is handled by the payment gateway, the Magento instance remains secure and out of scope for most PCI audits.
- Flexibility: Developers can programmatically retrieve or invalidate tokens as needed, offering advanced customization options.
Common Use Cases
- Recurring billing or subscription-based models
- High-volume eCommerce sites with loyal returning customers
- Mobile-first checkouts where speed is crucial
- Stores using multiple payment gateways with tokenization support
Retrieving Payment Gateway Tokens in Magento 2
In Magento 2, Vault is a powerful system designed to securely store and manage payment tokens. These tokens, often issued by third-party payment gateways, enable secure transactions without exposing raw credit card information. Accessing these tokens from placed orders is essential for extending Magento functionality in areas like custom payment flows, subscription modules, external integrations, or tokenized re-payments.
Use Cases for Gateway Token Retrieval
Retrieving gateway tokens tied to Magento orders supports a range of advanced use cases:
- One-Click and Recurring Payments
Enable customers to use previously stored cards without re-entering sensitive data. - Server-to-Server Integrations
Many payment providers (like Braintree, Authorize.Net, Stripe) allow refunds, subscriptions, or recharges using a token, not a card number. - Subscription and Membership Modules
Gateway tokens are crucial when managing auto-renewals or subscription billing logic via backend cron jobs or third-party APIs. - Custom Reporting and Reconciliation
Useful for audits and financial reconciliation tasks where gateway references are needed without exposing sensitive data. - Token Revocation or Updates
Build interfaces to let users revoke or update stored payment methods using Magento's Vault data.
Step-by-Step: How Token Retrieval Works
To retrieve a gateway token from a specific order:
- Load the Order
UseMagento\Sales\Api\OrderRepositoryInterface
to fetch the order object using its increment ID or entity ID. - Access the Payment Object
The payment information is retrieved from the order using$order->getPayment()
. - Access Extension Attributes
The extension attributes are available via the payment object. These attributes are Magento’s way of extending core entities without modifying their base structure. - Fetch Vault Payment Token
The Vault token contains the secure token reference returned by the gateway. - Get the Gateway Token
Finally, callgetGatewayToken()
on the Vault token object to retrieve the tokenized payment ID.
Implementation
<?php
use Magento\Sales\Api\OrderRepositoryInterface;
class RetrieveGatewayToken
{
protected $orderRepository;
public function __construct(OrderRepositoryInterface $orderRepository)
{
$this->orderRepository = $orderRepository;
}
/**
* Retrieve the stored payment gateway token from an order.
*
* @param int $orderId
* @return string|null
*/
public function getGatewayTokenByOrderId($orderId)
{
try {
$order = $this->orderRepository->get($orderId);
$payment = $order->getPayment();
$extensionAttributes = $payment->getExtensionAttributes();
$vaultPaymentToken = $extensionAttributes->getVaultPaymentToken();
return $vaultPaymentToken ? $vaultPaymentToken->getGatewayToken() : null;
} catch (\Exception $e) {
// Optional: log the error for debugging
return null;
}
}
}
Things to Consider
Aspect | Description |
---|---|
Vault Module Dependency | Ensure the Vault module is enabled (Magento_Vault ) and the payment provider supports tokenization. |
Secure Usage | Gateway tokens should only be accessed on the server side. Never expose them in frontend templates or logs. |
Data Consistency | Tokens are not always stored for every payment. Only token-based payment methods (e.g., credit card via Braintree) will generate them. |
Error Handling | If the Vault token is missing or the payment method doesn't support it, the return value should gracefully fall back to null . |
Token Format | Tokens are gateway-specific references (e.g., "8jf5kFjsk20@" ) and are used to authenticate server-to-server API calls with your payment processor. |
Advanced Development Tips
- Automated Renewals
Use the gateway token in a cron-based subscription module to renew services/products periodically. - Token Lifecycle Management
Build admin interfaces or observer classes that can handle token deletions or renewals in sync with customer actions. - Multi-Gateway Support
If your store uses multiple token-enabled gateways, ensure your logic correctly identifies the payment method before token use. - Auditing and Logging
Log usage of sensitive functions likegetGatewayToken()
to an audit table or secure log file to comply with internal security policies.
Magento 2’s Vault system provides a highly secure way to store and retrieve payment tokens. Accessing these tokens from order data allows for powerful extensions in payment automation, integrations, and custom module development. By using Magento’s service contracts and extension attributes properly, you maintain compliance, performance, and upgrade safety.
How to Retrieve a Payment Gateway Token from an Order in Magento 2
Retrieving a payment gateway token in Magento 2 involves several method calls and careful handling of the VaultPaymentToken
. This process is commonly required for operations like processing recurring payments, securely reusing payment methods, or integrating with external gateways using tokenization.
The steps below explain how to securely extract the gateway token from an order using Magento's API interfaces and extension attributes.
Step-by-Step Breakdown of Token Retrieval
Step | Method / Property | Description |
---|---|---|
1 | OrderRepositoryInterface->get($orderId) | Loads the full order object from the database using the provided order ID. This interface ensures the order is loaded using Magento’s standard repository pattern. |
2 | $order->getPayment() | Retrieves the payment information associated with the loaded order. This includes all details about how the customer paid. |
3 | $payment->getExtensionAttributes() | Accesses Magento’s extension attributes from the payment object. Extension attributes are used to provide additional metadata beyond core payment fields. |
4 | $extensionAttributes->getVaultPaymentToken() | Returns the VaultPaymentToken object if the payment method supports tokenization and the token exists. |
5 | $vaultPaymentToken->getGatewayToken() | Fetches the actual payment gateway token (such as a tokenized credit card reference), which can be used for further actions like refunds or recharges. |
Key Concepts and Considerations
Vault Token Eligibility
- Not all payment methods support vault token generation.
- Only methods that rely on tokenization (e.g., Braintree, Authorize.Net) will return valid gateway tokens.
Secure Access
- Gateway tokens should never be rendered in frontend templates or exposed in logs.
- Use server-side logic to handle and store these tokens securely.
Error Handling
- Always wrap the retrieval logic in a try-catch block to handle scenarios where the token is not available.
- Gracefully fallback to null or skip token-based logic if the token does not exist.
Use Case Examples
- Subscriptions and recurring billing.
- Third-party integrations needing secure recharges.
- Administrative interfaces that allow customer reorders using saved payment methods.
Developer Tip
To maintain forward compatibility, avoid directly accessing payment model internals. Always prefer interfaces like OrderRepositoryInterface and extension attribute methods provided by Magento.
Example Use Cases for Payment Gateway Tokenization
Tokenization in Magento can be used for a variety of practical scenarios that benefit both store owners and customers. The following are some common and valuable use cases:
Process Recurring Billing
For subscription-based services or products, payment gateways allow merchants to charge customers on a recurring basis without requiring customers to re-enter their payment details each time. By utilizing stored payment tokens, merchants can ensure smoother, hassle-free payments for recurring transactions.
Advantages:
- Seamless customer experience without repeated entry of sensitive data.
- Reduces cart abandonment during checkout for subscription renewals.
- Automates billing cycles and reduces administrative overhead.
Implementation:
Using tokens, merchants can initiate billing via APIs without needing to store or handle sensitive credit card information, ensuring compliance with PCI-DSS standards.
Re-authorize Transactions
Tokens can be reused for reauthorization of transactions that may have been delayed or failed due to network issues, insufficient funds, or temporary problems. This eliminates the need for the customer to manually re-enter their payment details for a re-attempt.
Advantages:
- Ensures faster payment processing for transactions that didn’t go through the first time.
- Improves customer satisfaction as they don't need to re-enter their information.
- Helps merchants retain sales without unnecessary friction.
Implementation:
When a transaction fails, the stored token can be used to re-authorize the transaction directly with the payment gateway.
Data Analysis & Reporting
Payment tokens can be securely logged to allow merchants to correlate transactions with specific gateway transaction IDs. This is useful for creating comprehensive reports on sales, refunds, or customer transaction history.
Advantages:
- Enhances transaction tracking by correlating tokens with customer orders.
- Improves financial reporting and reconciliation by linking gateway transactions to internal records.
- Makes auditing and dispute resolution easier, as tokenized transactions can be easily referenced.
Implementation:
Merchants can build reports that show token usage over time, helping them analyze payment success rates, transaction volumes, and customer behavior without exposing sensitive data.
Token Revocation
When a customer chooses to revoke their saved payment method (such as deleting or updating their credit card in the vault), the corresponding token should also be invalidated in the payment gateway to prevent unauthorized future transactions.
Advantages:
- Ensures security by revoking access to payment methods that are no longer valid.
- Protects against potential fraud by ensuring old tokens cannot be reused.
- Enhances trust with customers who know their payment details are managed securely.
Implementation:
When a user deletes a stored card or requests revocation, the corresponding token is communicated to the payment gateway for invalidation. This can be automated using APIs provided by the payment gateway.
Simplified Refunds
Tokens make it easier to handle refunds, as they allow merchants to quickly process refund transactions using the same payment method originally used, without requiring the customer to provide payment details again.
Advantages:
- Faster processing of refunds, leading to improved customer satisfaction.
- Reduces the administrative burden on merchants to collect payment details for refunds.
- Ensures accuracy in processing refunds to the correct payment method.
Implementation:
Merchants can initiate refunds through the payment gateway using the token stored during the initial purchase, ensuring a seamless experience for the customer.
Multi-Device Payment Management
For customers who use multiple devices for shopping, tokenization enables seamless transactions across platforms. Once a token is generated on one device, it can be reused on another, allowing customers to complete transactions without having to re-enter their payment details.
Advantages:
- Provides a consistent and smooth experience for customers across devices.
- Enhances mobile shopping experiences by allowing users to save their payment methods across devices.
- Reduces friction for customers making purchases on different devices or browsers.
Implementation:
Merchants can link payment tokens to customer accounts, enabling the customer to use the same payment method on any device, improving the overall user experience.
Fraud Prevention
By using tokenized payment methods, merchants can reduce the risk of fraud. Tokens are unique and can’t be intercepted like raw credit card details, offering an additional layer of security in the payment process.
Advantages:
- Minimizes exposure of sensitive card details, reducing the potential for fraud.
- Tokens are gateway-specific, making them harder to misuse if intercepted.
- Reduces the complexity of complying with security standards like PCI-DSS.
Implementation
Tokenization offers an advanced method of securing transactions, ensuring that even if a token is intercepted, it’s useless without access to the original payment gateway.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Conclusion
Retrieving the payment gateway token from the Vault in Magento 2.4.7 is an essential step in managing saved credit card data for returning customers and subscription-based payments. With Magento's secure Vault system, sensitive payment information like the gateway token is stored safely using encryption and PCI-compliant practices, allowing merchants to offer a seamless and secure checkout experience.
By using $order->getPayment()->getExtensionAttributes()->getVaultPaymentToken()->getGatewayToken()
, developers can retrieve the exact token associated with a transaction, which can then be used for future reference, fraud investigations, automated reorders, or integration with external CRMs and payment services.
This process ensures minimal friction during repeat purchases while maintaining strong data security. For developers and store owners alike, understanding how to programmatically access this data gives more control over the payment lifecycle and improves the overall customer experience. Always ensure that proper logging, error handling, and permission checks are in place when working with payment data in production environments.
Mastering Vault token retrieval is not just a developer trick—it’s a critical eCommerce skill that bridges convenience, performance, and trust.
FAQs
What is a Vault Payment Token in Magento 2?
A Vault Payment Token in Magento 2 securely stores payment gateway information like credit card tokens for future transactions.
How can I retrieve the payment gateway token from an order?
You can access it using $order->getPayment()->getExtensionAttributes()->getVaultPaymentToken()->getGatewayToken()
from the order object.
Which Magento interface is used to manage Vault payment data?
Magento uses the Vault module and the extension attributes from PaymentInterface
to handle stored tokenized payment data.
Do all orders in Magento 2 store Vault tokens?
No, only orders where the customer opts to save their payment details during checkout will store a Vault token.
Is storing credit card tokens secure in Magento 2?
Yes, tokens are stored securely using PCI-compliant tokenization through the Vault module, without saving raw credit card data.
Can guest orders store Vault payment tokens?
No, Vault tokens are generally stored for logged-in users who choose to save their payment method for future use.
Where can I find the Vault token in the database?
The Vault token is stored in the vault_payment_token
table in Magento's database, linked with the customer ID.
Can I disable Vault token storage in Magento 2?
Yes, you can disable it by configuring your payment method settings to not support saving payment methods for future use.
What module provides Vault functionality in Magento 2?
The functionality is provided by the Magento_Vault
module, which manages secure storage of payment methods.
Can I reuse Vault tokens for recurring payments?
Yes, Vault tokens are often used in recurring billing or subscription-based models to process repeat transactions securely.
Do all payment gateways support Vault in Magento?
No, only specific gateways like Braintree and Authorize.Net that integrate with Magento’s Vault API can use tokenization.
How do I debug Vault payment token issues?
You can enable developer mode, use logs, and inspect the vault_payment_token
table or extension attributes during order placement.
Is Vault available in both Magento Open Source and Adobe Commerce?
Yes, Vault is available in both versions, though Adobe Commerce may offer extended capabilities for advanced payment workflows.