How to delete cookie in Magento 2?
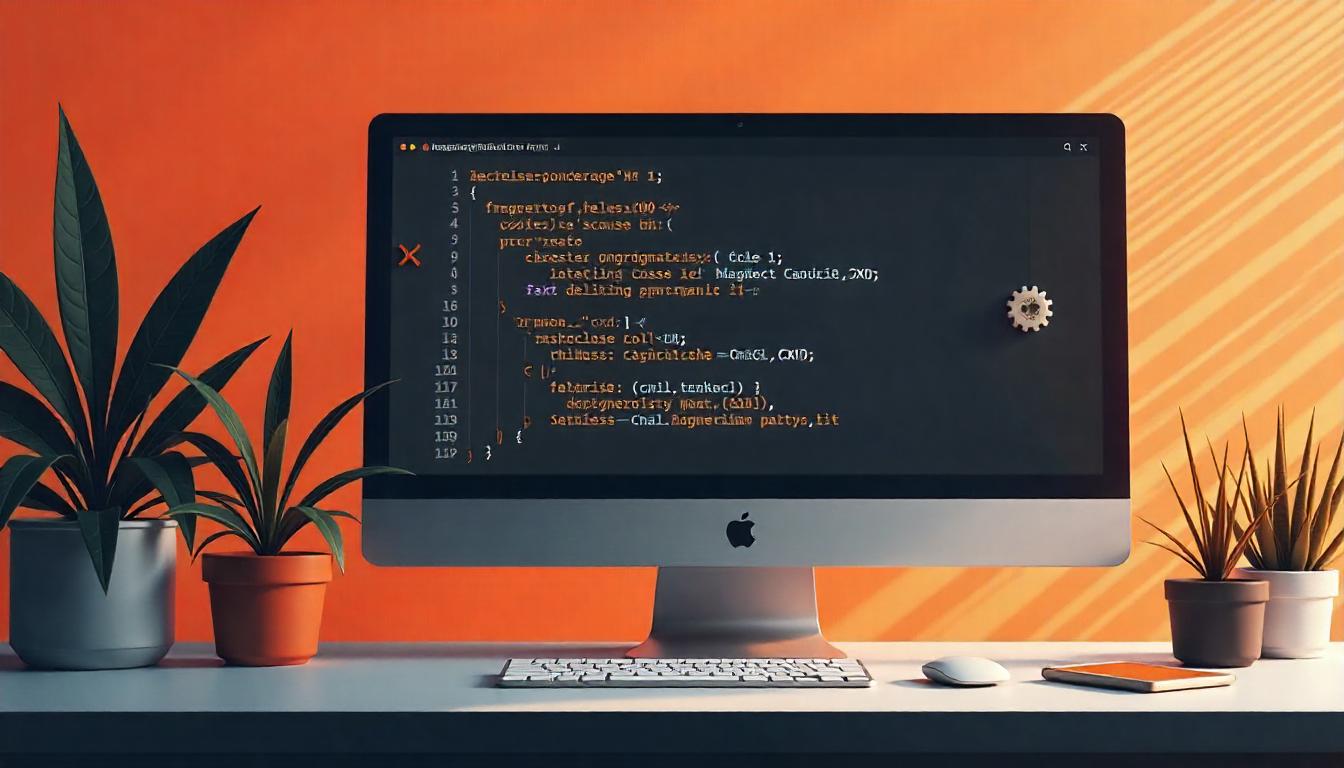
How to delete cookie in Magento 2?
To delete a cookie in Magento 2, use the CookieManagerInterface and optionally the CookieMetadataFactory if metadata was set when the cookie was created. This ensures precise removal and avoids stale cookie issues. Start by checking if the cookie exists using getCookie(). If it does, use deleteCookie() along with appropriate metadata like the path.
Table Of Content
How to delete cookie in Magento 2?
To delete cookies in Magento 2, use the Magento\Framework\Stdlib\CookieManagerInterface along with CookieMetadataFactory. This approach ensures precise control over cookie management, which is essential for maintaining user privacy and adhering to data protection standards.
Deleting a Specific Cookie
Here's how to delete a specific cookie named magento2cookie:
namespace Vendor\Module\Model;
use Magento\Framework\Stdlib\CookieManagerInterface;
use Magento\Framework\Stdlib\Cookie\CookieMetadataFactory;
class CookieHandler
{
private $cookieManager;
private $cookieMetadataFactory;
public function __construct(
CookieManagerInterface $cookieManager,
CookieMetadataFactory $cookieMetadataFactory
) {
$this->cookieManager = $cookieManager;
$this->cookieMetadataFactory = $cookieMetadataFactory;
}
public function deleteCookie()
{
if ($this->cookieManager->getCookie('magento2cookie')) {
$metadata = $this->cookieMetadataFactory->createPublicCookieMetadata();
$metadata->setPath('/');
$this->cookieManager->deleteCookie('magento2cookie', $metadata);
}
}
}
This code checks if the magento2cookie
exists. If it does, it creates metadata with the path set to '/'
and deletes the cookie using the deleteCookie
method.
Deleting All Cookies
To delete all cookies, you can retrieve all cookie names and delete them individually:
$cookieManager = $objectManager->get(\Magento\Framework\Stdlib\CookieManagerInterface::class);
$cookieNames = ['cookie1', 'cookie2', 'cookie3']; // Replace with actual cookie names
foreach ($cookieNames as $cookieName) {
$cookieManager->deleteCookie($cookieName);
}
Note: Magento doesn't provide a built-in method to retrieve all cookie names. You'll need to maintain a list of cookies you set and delete them as shown.
Cookie Deletion Methods Overview
Method | Description |
---|---|
deleteCookie($name) |
Deletes the specified cookie. |
deleteCookie($name, $meta) |
Deletes the specified cookie with metadata. |
Using metadata allows you to specify additional parameters like path and domain, ensuring the correct cookie is targeted.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What Is the Correct Way to Delete a Cookie in Magento 2?
You should use Magento\Framework\Stdlib\CookieManagerInterface
along with CookieMetadataFactory
to delete cookies safely and correctly.
Which Method Should I Use to Delete a Cookie?
Use the deleteCookie()
method from CookieManagerInterface
. This ensures proper cleanup and avoids unexpected behavior.
Do I Need Metadata to Delete Cookies?
Yes, if you originally set the cookie using metadata, you should delete it using metadata too. Use CookieMetadataFactory
to create metadata for deletion.
How Do I Delete a Specific Cookie Like 'magento2cookie'?
First, check if the cookie exists, then delete it using metadata as shown:
if ($this->cookieManager->getCookie('magento2cookie')) {
$metadata = $this->cookieMetadataFactory->createPublicCookieMetadata();
$metadata->setPath('/');
$this->cookieManager->deleteCookie('magento2cookie', $metadata);
}
Why Should I Avoid Manual Cookie Handling in Magento 2?
Using service classes ensures Magento handles cookie path, scope, and compatibility properly. Manual handling can lead to bugs or ignored settings.
Can I Delete All Cookies at Once in Magento 2?
Magento does not support deleting all cookies at once. You must know each cookie name and delete them individually with deleteCookie()
.
Where Can I Learn More About Cookie Handling in Magento 2?
Visit Magento’s official developer documentation or trusted community blogs for implementation patterns, best practices, and examples.