How to Retrieve Category Count in Magento 2.4.7
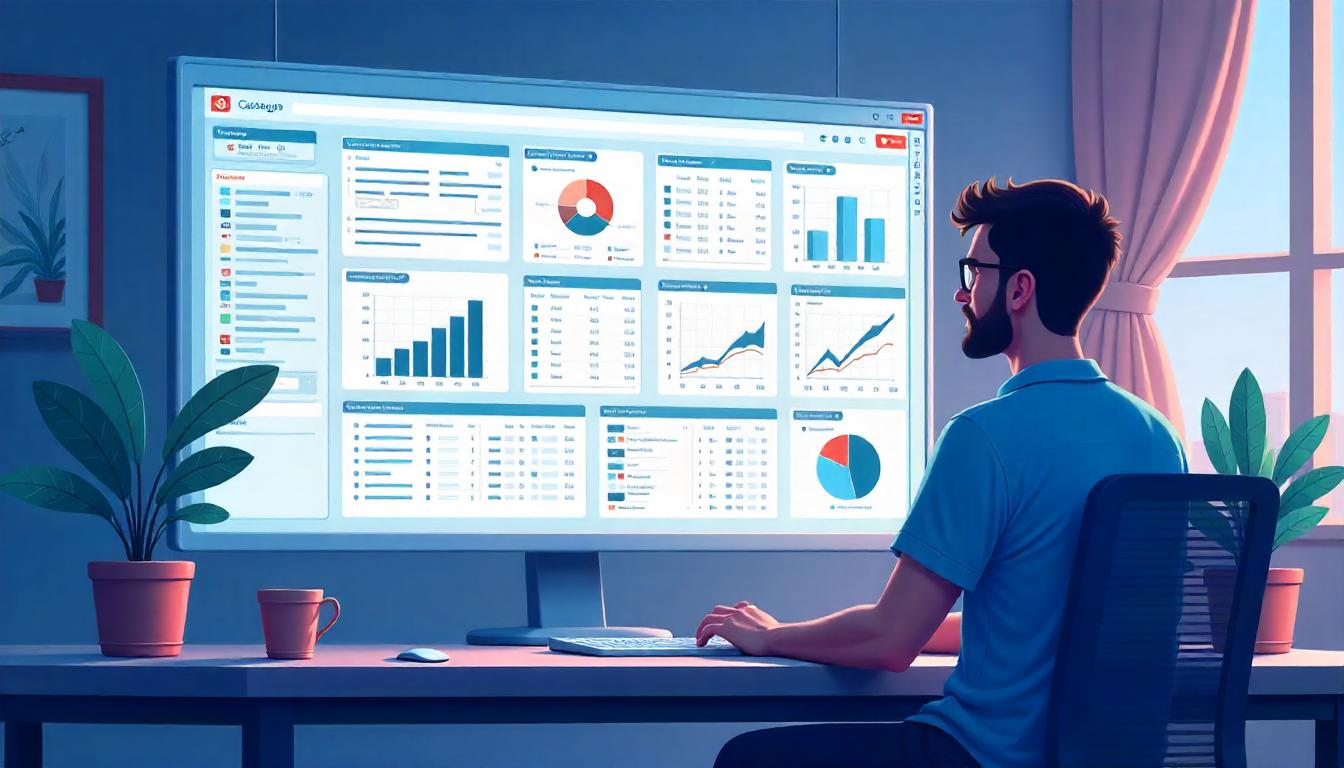
How to Retrieve Category Count in Magento 2.4.7
Categories in Magento 2 help structure the product catalog, making navigation smoother for customers and improving SEO. As an administrator or developer, you may need to retrieve the total number of categories programmatically. Magento 2.4.7 provides a clean API for fetching this data efficiently.
This guide will explain how to retrieve the category count using CategoryManagementInterface, along with best practices, troubleshooting tips, and real-world use cases.
Table Of Content
Efficient Category Management in Magento 2
Magento 2 offers multiple methods to interact with categories. However, the most efficient and recommended approach for retrieving category count and managing category structures is through the CategoryManagementInterface. This method ensures better performance, adheres to Magento's best practices, and integrates seamlessly with the system's dependency injection (DI) mechanism.
Why Use CategoryManagementInterface?
Using CategoryManagementInterface
provides several benefits:
- Performance Optimization: Fetching categories efficiently without excessive database queries.
- Scalability: Works well with large category trees without affecting performance.
- Best Practices: Complies with Magento 2’s architectural standards.
- Improved Maintainability: Easier debugging and future updates.
Retrieving Category Count in Magento 2
To retrieve the total number of categories within Magento, the CategoryManagementInterface
provides an efficient way to count categories within the store hierarchy.
Example Code
<?php
namespace Vendor\Module\Model;
use Magento\Catalog\Api\CategoryManagementInterface;
use Magento\Framework\Exception\NoSuchEntityException;
class CategoryHelper
{
/**
* @var CategoryManagementInterface
*/
private $categoryManagement;
/**
* Constructor
*
* @param CategoryManagementInterface $categoryManagement
*/
public function __construct(CategoryManagementInterface $categoryManagement)
{
$this->categoryManagement = $categoryManagement;
}
/**
* Get the total category count
*
* @param int|null $rootCategoryId
* @return int
* @throws NoSuchEntityException
*/
public function getCategoryCount($rootCategoryId = null)
{
try {
$categoryTree = $this->categoryManagement->getTree($rootCategoryId);
return count($categoryTree->getChildrenData());
} catch (NoSuchEntityException $e) {
throw new NoSuchEntityException(__('Category with ID %1 does not exist.', $rootCategoryId));
}
}
}
Explanation
- The
getCategoryCount()
method retrieves all categories under a given root category and returns the count. - The
getTree()
method fetches the category structure, and getChildrenData() retrieves the immediate child categories. - Exception handling ensures that invalid category IDs do not break the functionality.
Best Practices for Category Management
Below are essential best practices for optimizing category management in Magento 2:
Best Practice | Description |
---|---|
Limit Depth for Performance | Avoid fetching deep category levels unnecessarily to enhance speed. |
Enable Full-Page Caching | Store full category pages in cache to speed up category browsing. |
Use Flat Category Indexing | Reduce database queries by storing category data in a flat structure. |
Optimize Category URLs | Use SEO-friendly URLs (e.g., example.com/laptops ) for better ranking. |
Reindex After Updates | Run php bin/magento indexer:reindex after bulk category changes. |
Implement Caching for API Calls | Reduce API calls to avoid unnecessary database queries and slow performance. |
Optimize SQL Queries | Use indexing and efficient queries when retrieving category data. |
Efficient category management in Magento 2 requires leveraging best practices, optimizing API calls, and caching category data to enhance performance. Using CategoryManagementInterface is the cleanest and most reliable way to fetch category data while ensuring scalability.
Why Retrieve Category Count?
Knowing the total number of categories in your Magento store offers several benefits:
1. Analytics & Reporting
- Helps store owners monitor category growth over time.
- Supports business intelligence tools for better insights.
- Identifies trends in product categorization.
2. Custom Dashboard Widgets
- Useful for displaying category statistics on the admin dashboard.
- Helps admins track store structure at a glance.
- Can be integrated into store performance reports.
3. Performance Optimization
- Assists in managing category depth for faster page loads.
- Helps in reducing redundant categories for better organization.
- Optimizes indexing and caching strategies.
4. API Integrations
- Useful for third-party systems that require category data.
- Essential for marketplaces, ERP, and PIM integrations.
- Enables automated category synchronization across platforms.
Best Practices for Managing Categories in Magento 2
To ensure smooth category management and retrieval, follow these best practices:
Best Practice | Description |
---|---|
Limit Depth for Performance | Avoid fetching deep category levels unnecessarily to enhance speed. |
Enable Full-Page Caching | Store full category pages in cache to speed up category browsing. |
Use Flat Category Indexing | Reduce database queries by storing category data in a flat structure. |
Optimize Category URLs | Use SEO-friendly URLs (e.g., example.com/laptops) for better search ranking. |
Reindex After Updates | Run php bin/magento indexer:reindex after bulk category changes for optimal performance. |
Implement Caching for API Calls | Reduce API calls to avoid unnecessary database queries and slow performance. |
Optimize SQL Queries | Use indexing and efficient queries when retrieving category data. |
Use Dependency Injection (DI) | Inject services efficiently to follow Magento’s architectural standards. |
Handle Exceptions Gracefully | Implement proper error handling to manage cases where category IDs do not exist. |
Update Magento Version | Ensure your Magento installation is updated to benefit from the latest features and security improvements. |
Optimizing Category Management for Performance
1. Limit Category Depth
- Avoid excessive subcategories to reduce query complexity.
- Maintain a well-structured category tree to improve navigation speed.
2. Use Caching Mechanisms
- Implement
Magento\Framework\App\CacheInterface
to store category data. - Use Redis or Varnish caching for large-scale stores.
3. Optimize Indexing
- Enable Magento’s flat category indexing to improve query performance.
- Run
php bin/magento indexer:reindex
periodically to refresh category data.
4. API Best Practices
- Restrict API calls to retrieve only necessary category data.
- Use GraphQL or REST filters to limit retrieved data.
Retrieving and managing category counts in Magento 2 is essential for analytics, performance tuning, and API integrations. By following best practices such as caching, limiting depth, and optimizing indexing, store owners can ensure fast and efficient category management.
Retrieving Category Count in Magento 2 Using CategoryManagementInterface
Magento provides the CategoryManagementInterface
, which includes the getCount()
method to retrieve the total number of categories in the store.
Method Definition
/**
* Retrieve the total number of categories.
*
* @return int
*/
public function getCount();
Implementing Category Count Retrieval
1. Create a Model to Fetch Category Count
File:app/code/YourVendor/YourModule/Model/CategoryCount.php
<?php
namespace YourVendor\YourModule\Model;
use Magento\Catalog\Api\CategoryManagementInterface;
use Magento\Framework\Exception\LocalizedException;
class CategoryCount
{
/**
* @var CategoryManagementInterface
*/
private $categoryManagement;
/**
* Constructor
*
* @param CategoryManagementInterface $categoryManagement
*/
public function __construct(CategoryManagementInterface $categoryManagement)
{
$this->categoryManagement = $categoryManagement;
}
/**
* Retrieve total category count
*
* @return int
* @throws LocalizedException
*/
public function getCategoryCount()
{
try {
return $this->categoryManagement->getCount();
} catch (\Exception $e) {
throw new LocalizedException(__('Unable to retrieve category count: %1', $e->getMessage()));
}
}
}
2. Calling the Function in a Template or Class
Use the following code snippet to retrieve the category count in a block, controller, or helper class:
$categoryCount = $this->categoryCount->getCategoryCount();
echo "Total Categories: " . $categoryCount;
Detailed Explanation
Namespace & Imports
- The file is located in
YourVendor/YourModule/Model/
, following Magento's module structure.
Dependency Injection
CategoryManagementInterface
is injected into the class, avoiding direct ObjectManager usage.
Exception Handling
- The
try-catch
block ensures that errors are gracefully handled without breaking the site.
Reusability
- This model can be reused in multiple parts of the Magento system, including APIs and dashboards.
Best Practices for Category Management
Best Practice | Description |
---|---|
Limit Depth for Performance | Avoid fetching deep category levels unnecessarily to enhance speed. |
Enable Full-Page Caching | Store full category pages in cache to speed up category browsing. |
Use Flat Category Indexing | Reduce database queries by storing category data in a flat structure. |
Optimize Category URLs | Use SEO-friendly URLs (e.g., example.com/laptops) for better search ranking. |
Reindex After Updates | Run php bin/magento indexer:reindex after bulk category changes for optimal performance. |
Implement Caching for API Calls | Reduce API calls to avoid unnecessary database queries and slow performance. |
Optimize SQL Queries | Use indexing and efficient queries when retrieving category data. |
Use Dependency Injection (DI) | Inject services efficiently to follow Magento’s architectural standards. |
Handle Exceptions Gracefully | Implement proper error handling to manage cases where category IDs do not exist. |
Update Magento Version | Ensure your Magento installation is updated to benefit from the latest features and security improvements. |
Alternative Approach: Using ResourceModel
If you need an alternative way to get the category count, you can directly query the database using Magento’s ResourceModel
.
Implementation
File: app/code/YourVendor/YourModule/Model/CategoryCount.php
<?php
namespace YourVendor\YourModule\Model;
use Magento\Framework\App\ResourceConnection;
class CategoryCount
{
protected $resourceConnection;
/**
* Constructor
*
* @param ResourceConnection $resourceConnection
*/
public function __construct(ResourceConnection $resourceConnection)
{
$this->resourceConnection = $resourceConnection;
}
/**
* Retrieve total category count using direct SQL query
*
* @return int
*/
public function getCategoryCount()
{
$connection = $this->resourceConnection->getConnection();
$tableName = $connection->getTableName('catalog_category_entity');
$query = "SELECT COUNT(*) FROM " . $tableName;
return $connection->fetchOne($query);
}
}
Warning
Direct SQL queries should be used cautiously, as they bypass Magento’s ORM (Object-Relational Mapping). This can cause issues with future updates, caching, and indexing.
Which Method Should You Choose?
Approach | Advantages | Disadvantages |
---|---|---|
CategoryManagementInterface | Magento-recommended, safe, future-proof, supports DI | Slightly slower for very large category trees |
ResourceModel (SQL Query) | Faster for simple queries, minimal overhead | Not Magento-recommended, bypasses caching/indexing, potential update issues |
Retrieving category count in Magento 2 is an essential operation for analytics, reporting, and performance optimization.
- For long-term maintainability, use CategoryManagementInterface.
- For quick database queries, use ResourceModel, but be cautious about direct SQL usage.
- Follow best practices like caching, reindexing, and dependency injection to ensure smooth performance.
Optimizing Category Count Retrieval in Magento 2
Retrieving the category count efficiently in Magento 2 is crucial for performance optimization. Poorly optimized queries can lead to slow page loads and high server resource usage. Below are key techniques and best practices to ensure optimal performance.
1. Key Performance Considerations
1 Use Cached Data
Fetching the category count repeatedly increases database queries, slowing down page loads. Magento provides caching mechanisms to store results and reduce redundant database hits.
2 Limit API Calls
Repeatedly invoking getCount()
can negatively impact performance, especially in high-traffic stores. Store the result in memory or cache instead of making multiple calls.
3 Optimize Queries
If using direct SQL queries via ResourceModel, ensure proper indexing on category tables to enhance query execution speed.
4 Enable Indexing for Faster Retrieval
Magento provides indexing mechanisms to store category data efficiently. Keeping indexing updated ensures fast data retrieval.
5 Use Flat Category Tables
Magento allows storing category data in flat tables instead of the default EAV model. Enabling flat catalog indexing can improve performance significantly for category-related queries.
2. Comparison of Different Methods
Approach | Advantages | Disadvantages |
---|---|---|
CategoryManagementInterface | Magento-recommended, safe, future-proof, supports DI, caching | Slightly slower for very large category trees |
ResourceModel (SQL Query) | Faster for simple queries, minimal overhead | Bypasses Magento’s ORM, not recommended, indexing issues |
Flat Category Tables | Reduces complex queries, improves performance | Requires additional setup and indexing maintenance |
Custom Cache Storage | Reduces repeated queries, speeds up page loads | Cache invalidation needs to be handled properly |
3. Best Practices for Performance Optimization
Use Full-Page Caching | Store entire category pages in cache to speed up browsing. |
Enable Magento Indexing | Run php bin/magento indexer:reindex to ensure data is optimized. |
Limit Depth for Category Trees | Retrieve only necessary category levels instead of full hierarchy. |
Use Dependency Injection (DI) | Inject services properly to follow Magento’s architectural standards. |
Optimize SQL Queries | Ensure efficient queries and indexing when using direct SQL methods. |
Handle Exceptions Gracefully | Implement proper error handling to avoid application crashes. |
Update Magento Version | Use the latest Magento version for security, performance, and feature updates. |
Efficient category count retrieval in Magento 2 requires a balance between performance, maintainability, and Magento best practices. Using CategoryManagementInterface
is the recommended approach, but caching, indexing, and optimized queries can significantly improve speed and scalability.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Conclusion
Retrieving the total category count in Magento 2.4.7 is essential for efficient store management, reporting, and analytics. By leveraging CategoryManagementInterface, developers can fetch category data programmatically without relying on direct database queries, ensuring a more secure and optimized approach. This method is highly scalable and beneficial for stores with extensive category hierarchies, allowing for better organization and performance monitoring. Implementing caching mechanisms can further enhance speed and efficiency, especially for large-scale eCommerce platforms. Additionally, handling exceptions properly ensures that the store runs smoothly without unexpected errors. By integrating this method into your Magento 2 store, you can streamline category management, improve data accuracy, and enhance overall store performance, making it easier to analyze and optimize your product catalog structure.
FAQs
What is a category tree in Magento 2?
A category tree in Magento 2 represents the hierarchical structure of categories and subcategories used to organize products in the store.
How can I retrieve the total category count in Magento 2?
You can retrieve the total category count using the CategoryManagementInterface
with the getCount()
method.
Which interface is used to get the category count in Magento 2?
The Magento\Catalog\Api\CategoryManagementInterface
is used to retrieve the category count in Magento 2.
What is the purpose of category management in Magento 2?
Category management in Magento 2 allows store owners to organize products into logical categories and subcategories for better navigation and user experience.
Can I get the category count without writing custom code?
Yes, you can get the category count using the Magento 2 admin panel under Catalog > Categories, but for programmatic retrieval, you need custom code.
What is the role of the getCount() method in category retrieval?
The getCount()
method in Magento 2 fetches the total number of categories in the store dynamically.
Can I fetch category count for a specific store view?
Yes, you can fetch the category count for a specific store view by passing store-specific parameters in your code.
What is the benefit of retrieving category count programmatically?
Retrieving the category count programmatically helps in automation, analytics, reporting, and optimizing category structure in large Magento 2 stores.
Does Magento 2 store category count in the database?
No, Magento 2 calculates category count dynamically instead of storing it in a dedicated database field.
How does caching affect category count retrieval?
Magento 2 caching can store category data, so if categories are updated, clearing cache may be required to get accurate category counts.
Can I retrieve the category count using GraphQL in Magento 2?
Yes, Magento 2 allows retrieving category details using GraphQL queries, but category count may require custom implementation.
Is it possible to filter category counts by parent category?
Yes, by modifying the query, you can count only the categories under a specific parent category in Magento 2.
What is the default root category in Magento 2?
The default root category is the top-level category in Magento 2, under which all other categories and subcategories are organized.
How do I debug category count issues in Magento 2?
You can debug category count issues by enabling developer mode, checking logs, and ensuring correct use of the getCount()
method.
Can I use category count data in reports and dashboards?
Yes, category count data can be used in Magento 2 reports, custom dashboards, and analytics for better inventory and store management.