Magento 2: Filtering Product Collections with Service Contracts
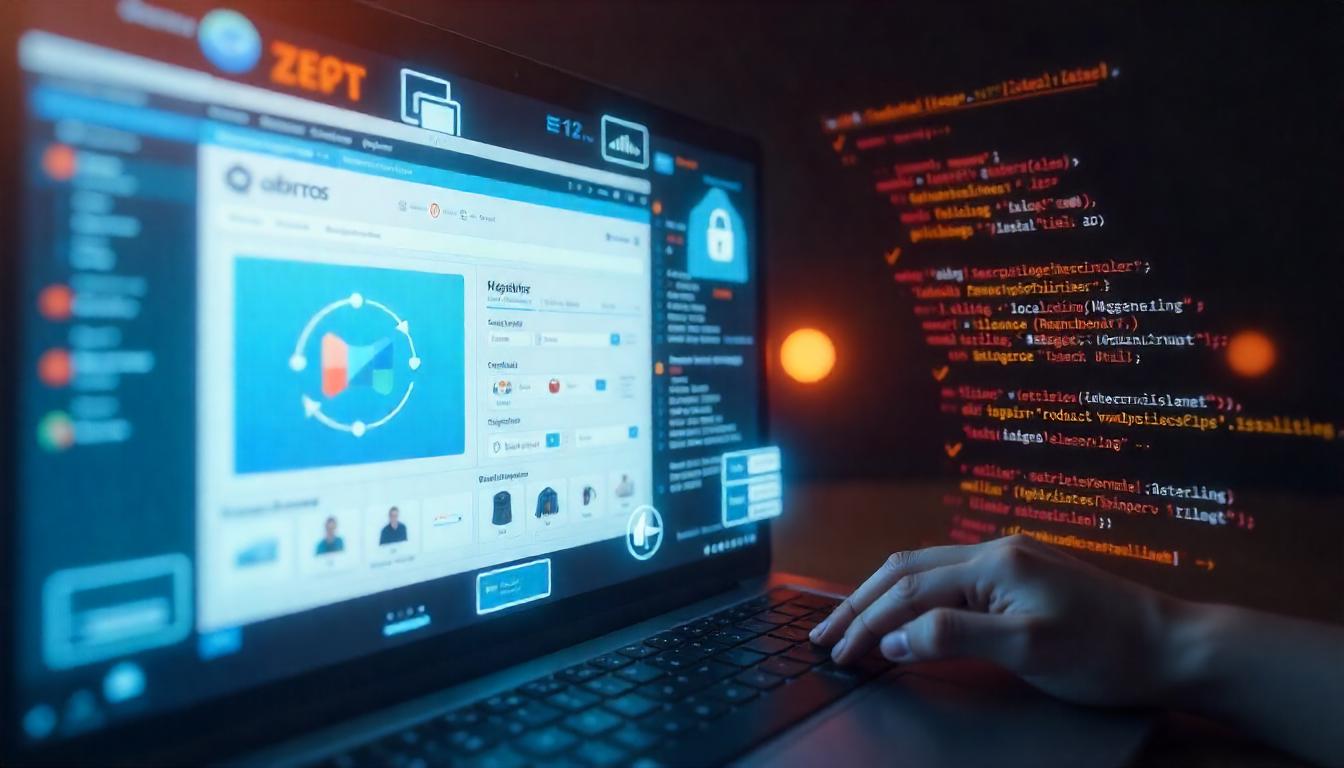
Magento 2: Filtering Product Collections with Service Contracts
Magento 2 uses service contracts to filter product collections efficiently. Instead of relying on deprecated methods like getCollection(), the recommended approach is to use ProductRepositoryInterface with SearchCriteriaBuilder. This method ensures better performance, cleaner code, and future compatibility.
Table Of Content
Magento 2: Filtering Product Collections with Service Contracts
In Magento 2, filtering product collections using service contracts is the recommended approach. Service contracts define how different modules interact, ensuring consistent data exchange and promoting clean code architecture.
To filter products by specific criteria, such as SKUs starting with "24-MB," you can utilize the ProductRepositoryInterface in conjunction with SearchCriteriaBuilder. Here's how you can implement this:
<?php
namespace Vendor\Module\Model;
use Magento\Catalog\Api\ProductRepositoryInterface;
use Magento\Framework\Api\SearchCriteriaBuilder;
class ProductService
{
private $productRepository;
private $searchCriteriaBuilder;
public function __construct(
ProductRepositoryInterface $productRepository,
SearchCriteriaBuilder $searchCriteriaBuilder
) {
$this->productRepository = $productRepository;
$this->searchCriteriaBuilder = $searchCriteriaBuilder;
}
public function getFilteredProducts()
{
$skuPrefix = '24-MB';
$this->searchCriteriaBuilder->addFilter('sku', $skuPrefix . '%', 'like');
$searchCriteria = $this->searchCriteriaBuilder->create();
$productList = $this->productRepository->getList($searchCriteria);
$products = $productList->getItems();
$productNames = [];
foreach ($products as $product) {
$productNames[] = $product->getName();
}
return $productNames;
}
}
In this implementation:
SearchCriteriaBuilder
adds a filter to select products whose SKUs start with "24-MB".ProductRepositoryInterface
retrieves the list of products matching the criteria.- The method returns an array of product names that meet the filter condition.
This method aligns with Magento 2's best practices by leveraging service contracts for data operations, ensuring a modular and maintainable codebase.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
Why Should I Use Service Contracts for Filtering Product Collections in Magento 2?
Service contracts provide a structured way to interact with data, ensuring maintainability and reducing dependency on direct model calls.
What Is the Recommended Method for Filtering Products Using Service Contracts?
Magento 2 recommends using SearchCriteriaBuilder
with ProductRepositoryInterface
to apply filters efficiently.
How Do I Filter Products by SKU Using Service Contracts?
Use the addFilter()
method in SearchCriteriaBuilder
to filter products based on SKU.
$this->searchCriteriaBuilder->addFilter('sku', '24-MB%', 'like');
$products = $this->productRepository->getList($this->searchCriteriaBuilder->create())->getItems();
What Are the Benefits of Using Service Contracts for Filtering?
- Scalability: Ensures compatibility with future Magento updates.
- Modularity: Separates business logic from data access.
- Efficiency: Optimizes queries and improves performance.
What Happens If I Use Deprecated Methods Like getCollection()?
Deprecated methods like getCollection()
are not recommended as they bypass service contracts, making the code harder to maintain.
How Can I Debug Filtering Issues in Magento 2?
Use Magento’s built-in logging and debugging tools to inspect query results and ensure correct filter application.
Where Can I Learn More About Magento 2 Service Contracts?
Refer to Magento’s official developer documentation and community forums for best practices and implementation details.