How to Retrieve list of Product attribute groups Magento 2.4.7
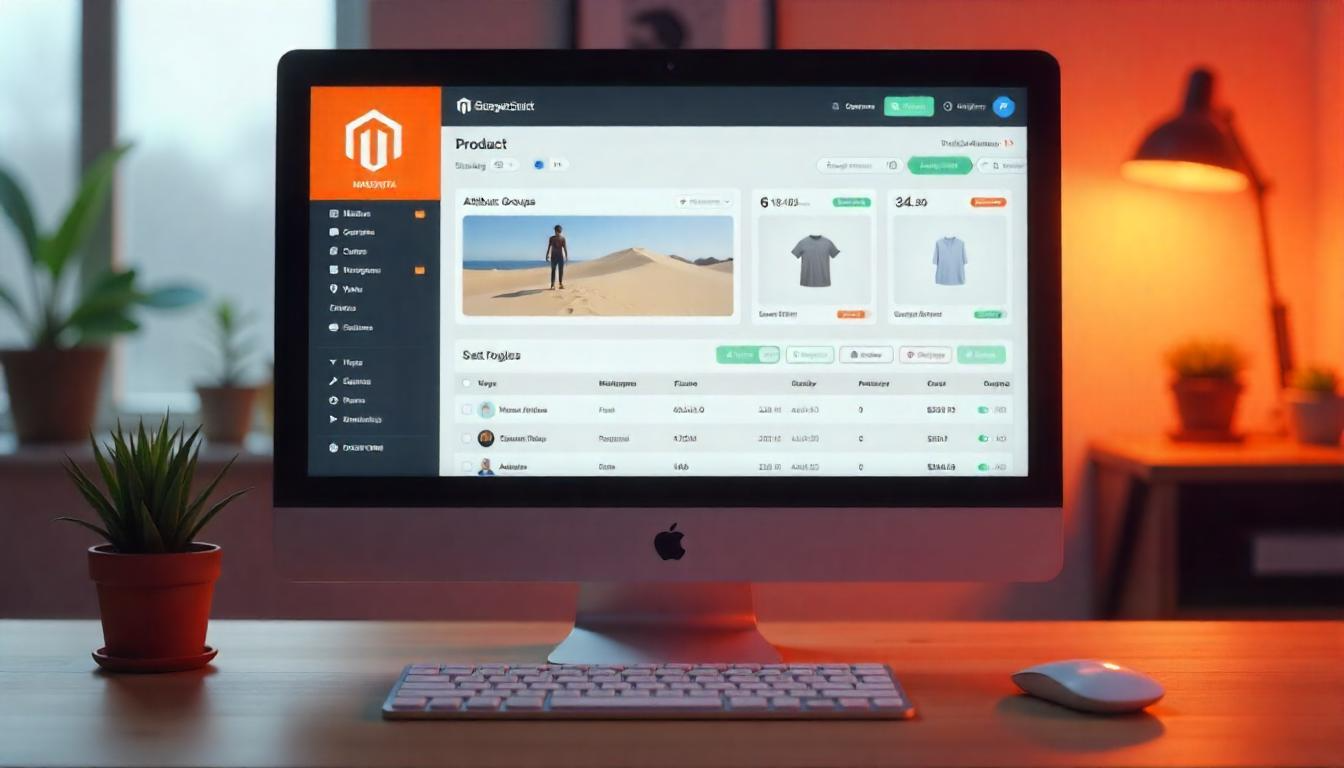
How to Retrieve list of Product attribute groups Magento 2.4.7
In Magento 2.4.7 (2025), managing product attributes efficiently is crucial for organizing product information and enhancing the user experience. Product attribute groups allow administrators to categorize related attributes, streamlining the product creation and editing processes. Retrieving a list of these attribute groups programmatically can be achieved using Magento's robust API interfaces.
Table Of Content
Understanding Product Attribute Groups in Magento 2
Product attribute groups are subsets within an attribute set that help organize product attributes efficiently. This organization streamlines attribute management and makes it easier to assign attributes to products. For example, attributes like color and size can be grouped under a General attribute group for better clarity and usability.
1. Importance of Attribute Groups
Why Are Attribute Groups Important?
Benefit | Description |
---|---|
Better Organization | Groups similar attributes together, improving manageability. |
Simplified UI | Enhances the admin panel experience by reducing clutter. |
Faster Attribute Assignment | Easily apply attributes to products without searching manually. |
Improved Performance | Categorized attributes allow faster retrieval and database optimization. |
Real-World Use Cases
- Fashion Store: Attributes like color, size, and material are grouped under a Product Details group.
- Electronics Store: Attributes like RAM, processor, and storage are grouped under a Specifications group.
- Custom Modules:> Developers use attribute groups to fetch product attributes dynamically for filtering or API responses.
2. Database Structure of Attribute Groups
Magento stores product attribute group information in the eav_attribute_group
table. Below is the structure of the key columns:
Attribute Group Database Schema
Column Name | Description |
---|---|
attribute_group_id | Unique identifier for the attribute group. |
attribute_set_id | Identifier linking the group to an attribute set. |
attribute_group_name | Name of the attribute group, displayed in the admin panel. |
sort_order | Order in which the group appears in the admin panel. |
default_id | Indicates whether this is the default group. |
attribute_group_code | Machine-readable code identifier for the attribute group. |
tab_group_code | Code for grouping tabs in the admin panel (nullable). |
Retrieving Product Attribute Groups Programmatically
To fetch a list of product attribute groups programmatically, use ProductAttributeGroupRepositoryInterface
with SearchCriteriaBuilder
. Below is a step-by-step guide:
Step 1: Create a Custom Module
If not already done, set up a custom module in Magento 2 to extend functionality.
Step 2: Develop the Model File
Within your module, create a model file, e.g., ProductAttributeGroup.php
, and implement the following code:
<?php
namespace Vendor\Module\Model;
use Magento\Framework\Api\SearchCriteriaBuilder;
use Magento\Eav\Api\Data\AttributeGroupSearchResultsInterface;
use Magento\Catalog\Api\ProductAttributeGroupRepositoryInterface;
use Magento\Framework\Exception\LocalizedException;
class ProductAttributeGroup
{
private $productAttributeGroupRepository;
private $searchCriteriaBuilder;
public function __construct(
ProductAttributeGroupRepositoryInterface $productAttributeGroupRepository,
SearchCriteriaBuilder $searchCriteriaBuilder
) {
$this->productAttributeGroupRepository = $productAttributeGroupRepository;
$this->searchCriteriaBuilder = $searchCriteriaBuilder;
}
public function getAttributeGroupList()
{
$searchCriteria = $this->searchCriteriaBuilder->create();
try {
$attributeGroupList = $this->productAttributeGroupRepository->getList($searchCriteria);
} catch (LocalizedException $e) {
throw new LocalizedException(__('Error retrieving attribute groups: %1', $e->getMessage()));
}
return $attributeGroupList;
}
}
Step 3: Invoke the Method
Call the getAttributeGroupList()
method from your template or any PHP class to retrieve attribute groups.
/** @var \Vendor\Module\Model\ProductAttributeGroup $attributeGroupModel */
$attributeGroupList = $attributeGroupModel->getAttributeGroupList();
foreach ($attributeGroupList->getItems() as $attributeGroup) {
// Process each attribute group
}
Sample Output
The getList()
method returns an instance of AttributeGroupSearchResultsInterface
, containing an array of attribute groups. Each attribute group provides details such as:
Attribute | Sample Value |
---|---|
attribute_group_id | 1 |
attribute_set_id | 1 |
attribute_group_name | General |
sort_order | 1 |
default_id | 1 |
attribute_group_code | general |
tab_group_code | null |
Understanding the eav_attribute_group Table
Magento stores product attribute group information in the eav_attribute_group
table. Below are key columns in this table:
Column Name | Description |
---|---|
attribute_group_id | Unique identifier for the attribute group. |
attribute_set_id | Identifier linking the group to an attribute set. |
attribute_group_name | Name of the attribute group, displayed in the admin panel. |
sort_order | Order in which the group appears in the admin panel. |
default_id | Indicates whether this is the default group. |
attribute_group_code | Machine-readable code identifier for the attribute group. |
tab_group_code | Code for grouping tabs in the admin panel (nullable). |
Common Issues & Solutions
Issue | Cause | Solution |
---|---|---|
Attribute Group Not Found | The attribute_group_id does not exist in the database. | Verify the group ID using: SELECT * FROM eav_attribute_group WHERE attribute_group_id = X; |
Cannot Delete Group | Contains system-required attributes. | Move the attributes to another group before attempting deletion. |
Changes Not Visible | Cache is preventing updates from appearing. | Run: php bin/magento cache:flush and php bin/magento indexer:reindex |
Performance Issues | Fetching attribute groups frequently can slow down performance. | Use a collection model for bulk retrieval instead of multiple single queries. |
Permission Errors | The user lacks necessary permissions. | Check and adjust role permissions in Magento Admin: System → Permissions → User Roles |
Benefits of Using Product Attribute Groups
Benefit | Description |
---|---|
Better Organization | Groups similar attributes together, improving manageability. |
Simplified UI | Enhances the admin panel experience by reducing clutter. |
Faster Attribute Assignment | Easily apply attributes to products without searching manually. |
Improved Performance | Categorized attributes allow faster retrieval and database optimization. |
This structured approach ensures better attribute management in Magento 2, enhancing both usability and performance.
Advanced Features and Optimizations in Magento 2 Attribute Groups
Magento 2 provides flexible ways to manage attribute groups, but optimizing their retrieval, caching, and extension can significantly improve performance and maintainability.
1. Efficiently Retrieving Attribute Groups
Retrieving multiple attribute groups using a collection model is more efficient than querying one by one.
Implementation:
use Magento\Eav\Model\ResourceModel\Entity\Attribute\Group\CollectionFactory;
public function __construct(CollectionFactory $attributeGroupCollectionFactory)
{
$this->attributeGroupCollectionFactory = $attributeGroupCollectionFactory;
}
/**
* Retrieve all attribute groups in bulk
*
* @return \Magento\Eav\Model\Entity\Attribute\Group[]
*/
public function getAllAttributeGroups()
{
$collection = $this->attributeGroupCollectionFactory->create();
return $collection->getItems();
}
Advantages:
- Better Performance: Reduces multiple queries into a single batch request.
- Scalability: Works efficiently for large stores with many attribute groups.
- Flexible Filtering: Supports adding filters and conditions dynamically.
2. Optimizing Attribute Group Fetching with Caching
Since attribute groups rarely change, caching them can reduce database queries and speed up performance.
Implementation:
$cacheKey = 'custom_attribute_groups';
$cachedData = $this->cache->load($cacheKey);
if (!$cachedData) {
$data = $this->getAllAttributeGroups();
$this->cache->save(serialize($data), $cacheKey, ['custom_cache_tag'], 86400); // Cache for 24 hours
} else {
$data = unserialize($cachedData);
}
Why Use Caching?
- Faster Load Times: Reduces repeated database queries.
- Efficient Resource Usage: Reduces strain on the database server.
- Scalability: Ideal for stores with thousands of attributes.
3. Extending Attribute Groups with Custom Fields
You may need to add custom fields to the eav_attribute_group table to store additional data.
How to Add a Custom Field in Attribute Groups
Magento allows schema upgrades to add new fields to existing tables.
Setup Script for Adding a Custom Field
use Magento\Framework\Setup\UpgradeSchemaInterface;
use Magento\Framework\Setup\SchemaSetupInterface;
use Magento\Framework\Setup\ModuleContextInterface;
class UpgradeSchema implements UpgradeSchemaInterface
{
public function upgrade(SchemaSetupInterface $setup, ModuleContextInterface $context)
{
$setup->startSetup();
$table = $setup->getTable('eav_attribute_group');
$setup->getConnection()->addColumn(
$table,
'custom_field',
[
'type' => \Magento\Framework\DB\Ddl\Table::TYPE_TEXT,
'nullable' => true,
'comment' => 'Custom Field for Attribute Group'
]
);
$setup->endSetup();
}
}
Use Cases for Custom Fields:
- Adding Metadata: Store extra data like descriptions, internal notes, or tracking data.
- Enhanced Group Filtering: Assign special tags or statuses to attribute groups.
4. Best Practices for Managing Attribute Groups
Here are some best practices to ensure efficient handling of attribute groups in Magento 2.
Use Dependency Injection (DI)
- Always inject interfaces in the constructor instead of using
ObjectManager
. - Example
public function __construct(
ProductAttributeGroupRepositoryInterface $productAttributeGroupRepository
) {
$this->productAttributeGroupRepository = $productAttributeGroupRepository;
}
Dynamic Group ID Handling
- Instead of hardcoding group IDs, fetch them dynamically.
- Example:
$groupId = $this->getGroupIdByName('General');
Implement Logging for Errors
- Use Magento’s
LoggerInterface
for better debugging. - Example
$this->logger->error('Attribute Group retrieval failed: ' . $e->getMessage());
Validate Inputs
- Ensure that
attribute_group_id
is valid before making queries.
Cache Attribute Data
- Utilize Magento’s built-in
cache storage
to reduce database load.
5. Benefits of Using Attribute Groups
Attribute groups improve store efficiency by simplifying attribute management.
Benefit | Description |
---|---|
Better Organization | Groups similar attributes together for easy manageability. |
Simplified UI | Reduces clutter in the admin panel for faster editing. |
Faster Attribute Assignment | Makes assigning attributes to products more efficient. |
Improved Performance | Categorized attributes allow faster retrieval and database optimization. |
Magento 2 provides powerful tools to manage product attribute groups efficiently. By implementing best practices, caching, logging, and dynamic retrieval methods, developers can optimize performance while maintaining flexibility and scalability.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Conclusion
Efficiently managing product attributes is crucial for organizing product data and enhancing the user experience in Magento 2.4.7. By leveraging Product Attribute Groups, store administrators can structure related attributes systematically, making product creation and editing more streamlined.
Retrieving product attribute groups programmatically using ProductAttributeGroupRepositoryInterface and SearchCriteriaBuilder allows developers to dynamically fetch attribute group details, reducing manual efforts and improving efficiency. Understanding the EAV structure and utilizing best coding practices—such as dependency injection, error handling, and optimized queries—ensures smooth integration and performance.
By implementing these techniques, Magento 2 store owners and developers can create a well-organized, scalable attribute management system, ultimately improving the overall eCommerce workflow.
FAQs
What is a product attribute group in Magento 2?
A product attribute group in Magento 2 is a collection of attributes within an attribute set that helps organize attributes logically in the admin panel.
How do I retrieve a list of product attribute groups in Magento 2?
You can retrieve the list of product attribute groups programmatically using the ProductAttributeGroupRepositoryInterface
and SearchCriteriaBuilder
.
Where is product attribute group data stored in Magento 2?
Product attribute group data is stored in the eav_attribute_group
database table.
How do I get product attribute groups using a repository in Magento 2?
You can use the getList()
method of ProductAttributeGroupRepositoryInterface
with a SearchCriteria object to fetch attribute groups.
Can I fetch attribute group details using attribute group ID?
Yes, you can fetch attribute group details by using the get()
method from ProductAttributeGroupRepositoryInterface
with the specific group ID.
What kind of details can I get from a product attribute group?
You can retrieve details such as attribute group ID, attribute set ID, group name, sort order, default ID, attribute group code, and tab group code.
How does Magento 2 organize product attributes?
Magento 2 organizes product attributes into attribute sets, which further contain attribute groups, helping to streamline product data management.
Why are attribute groups important in Magento 2?
Attribute groups help organize product attributes efficiently, making it easier for store admins to manage and update product information.
How do I programmatically fetch attribute groups in Magento 2?
You can programmatically fetch attribute groups using ProductAttributeGroupRepositoryInterface
and iterating over the retrieved data.
What happens if an attribute group ID does not exist?
If an attribute group ID does not exist, Magento will throw a "No such entity with ID" exception.
Can I create custom product attribute groups in Magento 2?
Yes, you can create custom product attribute groups manually in the admin panel or programmatically by modifying the eav_attribute_group
table.
How do I sort product attribute groups in Magento 2?
Product attribute groups are sorted using the sort_order
field in the eav_attribute_group
table.
Can I remove a product attribute group in Magento 2?
Yes, you can remove an attribute group using Magento’s admin panel or programmatically by modifying the eav_attribute_group
table.
Does updating an attribute group affect existing products?
No, updating an attribute group does not affect existing product attributes unless you remove or reassign attributes within the group.