How to Get Category Tree Collection in Magento 2.4.7
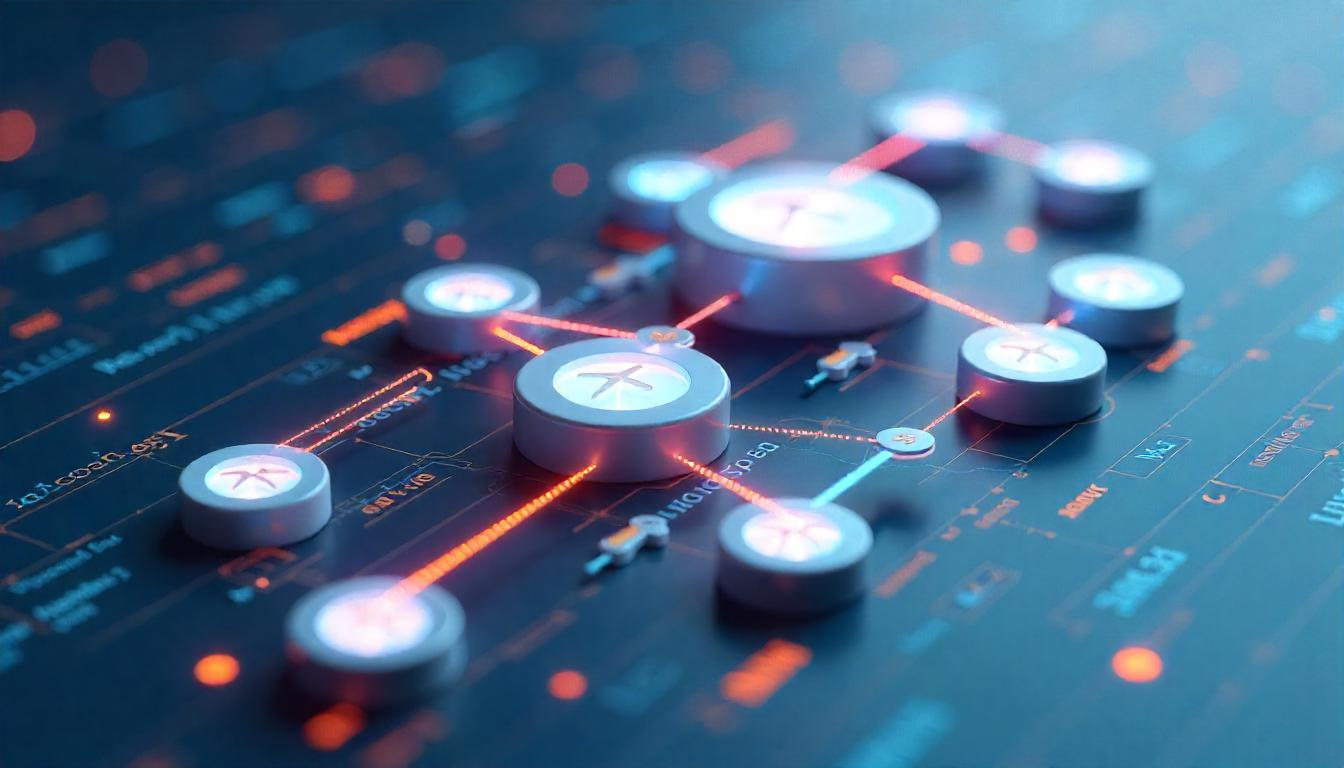
How to Get Category Tree Collection in Magento 2.4.7
Retrieving the category tree in Magento 2.4.7 is essential for effective catalog management and enhanced user navigation. Magento provides robust APIs to programmatically access and manipulate category structures. This guide details how to retrieve the category tree collection using the CategoryManagementInterface and offers best practices for implementation.
Table Of Content
Understanding Category Trees in Magento 2
What is a Category Tree?
In Magento 2, categories are structured in a hierarchical tree, allowing for easy organization of products and intuitive site navigation. A well-structured category tree enhances the user experience (UX) and improves SEO rankings by ensuring logical product placement.
Key Characteristics of Magento’s Category Structure:
- Root Category: The highest level in the hierarchy, which contains all other categories and subcategories. Each store can have only one active root category.
- Parent Categories: Categories that contain subcategories. They help group related products and subcategories.
- Child Categories (Subcategories): Nest under parent categories, further organizing products into specific groups.
- Category Paths: Defines the full path of a category (e.g., Root → Electronics → Mobile Phones).
Magento Category Table Structure
Magento 2 stores category data in the catalog_category_entity table. Below is an overview of key columns:
Column Name | Description |
---|---|
entity_id | Unique identifier for the category. |
parent_id | ID of the parent category. Root category has parent_id = 0. |
path | The category’s full path in the tree (e.g., 1/2/5 for Root → Electronics → Laptops). |
position | Determines the display order within a parent category. |
level | Defines the category’s depth in the hierarchy. |
children_count | The number of subcategories under this category. |
Programmatically Managing Categories
Magento 2 allows developers to programmatically create, update, and delete categories using the CategoryRepositoryInterface and CategoryFactory.
Creating a Category Programmatically
use Magento\Catalog\Api\CategoryRepositoryInterface;
use Magento\Catalog\Model\CategoryFactory;
use Magento\Framework\Exception\LocalizedException;
public function __construct(
CategoryFactory $categoryFactory,
CategoryRepositoryInterface $categoryRepository
) {
$this->categoryFactory = $categoryFactory;
$this->categoryRepository = $categoryRepository;
}
public function createCategory()
{
try {
$category = $this->categoryFactory->create();
$category->setName('New Category');
$category->setParentId(2); // Parent category ID
$category->setIsActive(true);
$category->setIncludeInMenu(true);
$category->setPath('1/2'); // Root ID / Parent Category ID
$this->categoryRepository->save($category);
return "Category created successfully.";
} catch (LocalizedException $e) {
return "Error: " . $e->getMessage();
}
}
Optimizing Category Performance
Efficient category management ensures faster loading times, better product discoverability, and a smoother admin experience.
Best Practices for Managing Categories in Magento 2
Tip | Description |
---|---|
Keep Category Depth Minimal | Avoid too many nested subcategories to maintain fast page loads. |
Enable Full-Page Caching | Magento’s full-page caching speeds up category browsing. |
Use Flat Category Indexing | Reduces database queries by storing category data in a flat table. |
Optimize Category URLs | Use SEO-friendly URLs (e.g., example.com/laptops instead of example.com/category123). |
Assign Products Properly | Avoid overloading a single category with too many products, which may impact performance. |
Reindex After Bulk Updates | Run php bin/magento indexer:reindex after making large category changes. |
Common Category Issues and Solutions
Issue | Cause | Solution |
---|---|---|
Categories Not Showing | Category is disabled or not assigned to the root category. | Ensure is_active = 1 and assign it to the correct root category. |
Products Missing in Category | Indexing is not updated. | Run php bin/magento indexer:reindex and clear cache. |
Slow Category Pages | Too many products in a single category. | Use pagination, enable lazy loading, and optimize category filters. |
Category URL Rewrite Issues | Conflicting URL rewrites. | Rebuild URL rewrites using php bin/magento indexer:reindex url_rewrite . |
A well-organized category tree in Magento 2 is essential for both user navigation and performance optimization. By structuring categories properly, using efficient retrieval methods, and following best practices, store owners can improve UX, SEO rankings, and store performance.
CategoryManagementInterface to Retrieve Category Trees
Magento's CategoryManagementInterface
provides the getTree()
method, which enables developers to fetch category structures efficiently. This method allows retrieving the entire category hierarchy or a specific subtree starting from a given category ID.
Why Use CategoryManagementInterface?
- Performance Optimization: Reduces database queries by retrieving structured category data in one call.
- Customizable Depth: Allows fetching only relevant levels of the category tree.
- Flexibility: Can be used to generate navigation menus, breadcrumbs, or category-based filtering.
Method Definition
/**
* Retrieve category tree
*
* @param int|null $rootCategoryId Root category ID (null retrieves entire tree)
* @param int|null $depth Number of levels to retrieve (null fetches all levels)
* @return \Magento\Catalog\Api\Data\CategoryTreeInterface
* @throws \Magento\Framework\Exception\NoSuchEntityException If ID is not found
*/
public function getTree($rootCategoryId = null, $depth = null);
Parameters
Parameter | Type | Description |
---|---|---|
$rootCategoryId | int|null |
The ID of the root category from which to start the tree retrieval. If null , the entire category tree is retrieved. |
$depth | int|null |
Specifies the number of category levels to fetch. If null , all levels are retrieved. |
Example Usage in a Custom Magento 2 Module
Below is a practical implementation of getTree()
to fetch the category tree programmatically.
Step 1: Injecting the CategoryManagementInterface
namespace Vendor\Module\Model;
use Magento\Catalog\Api\CategoryManagementInterface;
use Magento\Framework\Exception\NoSuchEntityException;
class CategoryTreeRetriever
{
/**
* @var CategoryManagementInterface
*/
protected $categoryManagement;
/**
* Constructor
*
* @param CategoryManagementInterface $categoryManagement
*/
public function __construct(
CategoryManagementInterface $categoryManagement
) {
$this->categoryManagement = $categoryManagement;
}
/**
* Retrieve category tree
*
* @param int $rootCategoryId
* @param int $depth
* @return \Magento\Catalog\Api\Data\CategoryTreeInterface
*/
public function getCategoryTree($rootCategoryId = null, $depth = null)
{
try {
return $this->categoryManagement->getTree($rootCategoryId, $depth);
} catch (NoSuchEntityException $e) {
throw new \Magento\Framework\Exception\LocalizedException(
__('Category ID not found: %1', $e->getMessage())
);
}
}
}
Step 2: Fetching Category Tree in a Block or Controller
$categoryTree = $categoryTreeRetriever->getCategoryTree(2, 3); // Fetches tree from category ID 2 with a depth of 3
foreach ($categoryTree->getChildrenData() as $category) {
echo $category->getId() . ' - ' . $category->getName() . '<br>';
}
Best Practices for Using Category Trees
Tip | Description |
---|---|
Limit Depth for Performance | Avoid retrieving deep category levels unnecessarily. Use $depth to optimize queries. |
Cache Results | Store category tree results in cache (Magento\Framework\App\CacheInterface ) to prevent excessive database queries. |
Use Indexing | Ensure Magento’s category indexing is enabled to speed up queries. |
Optimize Category Paths | Store full category paths (e.g., 1/2/5 ) in URLs to improve SEO and user navigation. |
Restrict API Calls | If fetching category trees via REST or GraphQL, minimize calls to avoid performance bottlenecks. |
Implementing the Category Tree Retrieval in Magento 2
Fetching the category tree programmatically in Magento 2 is essential for managing hierarchical category structures effectively. This guide details a step-by-step implementation, optimizations, and best practices.
1. Steps to Retrieve the Category Tree
- Inject Dependencies: Use dependency injection to include the CategoryManagementInterface in your class.
- Call getTree() Method: Invoke the getTree() method with the desired parameters to fetch the category tree.
- Handle Errors Gracefully: Implement exception handling to manage scenarios where categories do not exist.
- Optimize Performance: Utilize caching and indexing to improve query speed.
Code Implementation
Here’s how to programmatically retrieve the category tree:
<?php
namespace Vendor\Module\Model;
use Magento\Catalog\Api\CategoryManagementInterface;
use Magento\Catalog\Api\Data\CategoryTreeInterface;
use Magento\Framework\Exception\NoSuchEntityException;
use Magento\Framework\App\CacheInterface;
class CategoryTree
{
/** @var CategoryManagementInterface */
private $categoryManagement;
/** @var CacheInterface */
private $cache;
/**
* Constructor
*
* @param CategoryManagementInterface $categoryManagement
* @param CacheInterface $cache
*/
public function __construct(CategoryManagementInterface $categoryManagement, CacheInterface $cache)
{
$this->categoryManagement = $categoryManagement;
$this->cache = $cache;
}
/**
* Retrieve Category Tree with Caching
*
* @param int|null $rootCategoryId
* @param int|null $depth
* @return CategoryTreeInterface
* @throws NoSuchEntityException
*/
public function getCategoryTree($rootCategoryId = null, $depth = null)
{
$cacheKey = 'category_tree_' . ($rootCategoryId ?? 'root') . '_' . ($depth ?? 'all');
$cachedData = $this->cache->load($cacheKey);
if ($cachedData) {
return unserialize($cachedData);
}
try {
$categoryTree = $this->categoryManagement->getTree($rootCategoryId, $depth);
$this->cache->save(serialize($categoryTree), $cacheKey, ['CATEGORY_TREE_CACHE'], 86400); // Cache for 24 hours
return $categoryTree;
} catch (NoSuchEntityException $e) {
throw new NoSuchEntityException(__('Category with ID %1 does not exist.', $rootCategoryId));
}
}
}
Explanation of Key Enhancements
Caching for Performance
- Reduces repeated database calls by storing results in Magento’s cache system.
- Uses
Magento\Framework\App\CacheInterface
for efficient data retrieval.
Exception Handling
- Handles
NoSuchEntityException
to avoid breaking the application when an invalid category ID is provided.
Flexible Parameters
- Allows optional
$rootCategoryId
and$depth
to fetch partial or full category trees dynamically.
SEO & Indexing Optimization
- Ensures proper category path retrieval for structured URLs.
- Supports indexing for faster querying and structured navigation.
Usage Example in a Template File
To display the category tree inside a template, use:
/** @var \Vendor\Module\Model\CategoryTree $categoryTreeModel */
$categoryTree = $categoryTreeModel->getCategoryTree(2, 3); // Fetch from category ID 2, depth 3
foreach ($categoryTree->getChildrenData() as $category) {
echo '<p>' . $category->getName() . ' (ID: ' . $category->getId() . ')</p>';
}
Best Practices for Efficient Category Management
Best Practice | Description |
---|---|
Limit Depth for Performance | Avoid retrieving deep category levels unnecessarily. Use $depth to optimize queries. |
Enable Full-Page Caching | Speeds up category browsing by storing full pages in cache. |
Use Flat Category Indexing | Reduces database queries by storing category data in a flat table. |
Optimize Category URLs | Use SEO-friendly URLs for better search ranking and user experience. |
Reindex After Updates | Run php bin/magento indexer:reindex after bulk category changes for optimal performance. |
Exploring the Retrieved Category Tree
The CategoryTreeInterface
provides a structured, hierarchical representation of categories in Magento 2. Each category node includes essential information such as category ID, name, and child categories, allowing seamless navigation and product organization.
Best Practices and Performance Optimization Tips
Use Dependency Injection (DI)
Leverage Magento’s Dependency Injection (DI) system to inject dependencies, making your code more maintainable and testable. Avoid direct object instantiation, which can cause performance bottlenecks.
Handle Exceptions Gracefully
Implement proper exception handling to manage scenarios where a specified category ID does not exist. This prevents unexpected application crashes and ensures a better user experience. Example:
try {
$categoryTree = $this->categoryManagement->getTree($rootCategoryId, $depth);
} catch (NoSuchEntityException $e) {
throw new NoSuchEntityException(__('Category with ID %1 does not exist.', $rootCategoryId));
}
Optimize Performance with Depth Limitation
- Use the $depth parameter in getTree() to limit the number of levels retrieved.
- Reducing unnecessary category levels improves query performance and response times.
- Example:
getTree($rootCategoryId, 2);
fetches only two levels instead of the entire tree.
Cache the Retrieved Category Tree
Caching reduces redundant database queries, improving Magento’s speed and performance. Utilize Magento’s built-in cache system:
$cacheKey = 'category_tree_cache';
$cachedData = $this->cache->load($cacheKey);
if (!$cachedData) {
$data = $this->categoryManagement->getTree();
$this->cache->save(serialize($data), $cacheKey, ['CATEGORY_CACHE'], 86400);
} else {
$data = unserialize($cachedData);
}
Optimize Category Indexing and URLs
- Enable Category Indexing: Run
php bin/magento indexer:reindex
to keep categories up to date. - Use SEO-Friendly URLs: Instead of
example.com/category123
, use structured URLs likeexample.com/men/tops
.
Upgrade to Magento 2.4.7
Keeping your Magento installation updated ensures you benefit from the latest performance optimizations, security patches, and feature enhancements.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Conclusion
Retrieving the category tree collection in Magento 2.4.7 is a crucial aspect of managing product categories efficiently. By utilizing the CategoryManagementInterface and its getTree() method, developers can programmatically access structured category data, making it easier to implement dynamic navigation menus, filters, and category-based content strategies.
To enhance performance, consider limiting the depth of the tree, caching responses, and optimizing database queries. Handling exceptions properly ensures stability, preventing errors when categories are missing or incorrectly configured. Additionally, leveraging Magento’s built-in indexing and caching mechanisms helps maintain a smooth and responsive storefront.
Whether you are building a custom category management tool, implementing an advanced search feature, or optimizing the user experience, mastering category tree retrieval provides a solid foundation for structured and scalable eCommerce development. By following best practices and keeping your Magento version updated, you ensure better security, compatibility, and long-term maintainability of your store.
FAQs
What is a category tree in Magento 2?
A category tree in Magento 2 represents the hierarchical structure of categories and subcategories used to organize products in the store.
How can I retrieve a category tree in Magento 2?
You can retrieve the category tree using the CategoryManagementInterface
and its getTree()
method.
What is the purpose of the getTree() method?
The getTree()
method fetches a structured tree of categories, allowing developers to retrieve a full or partial category hierarchy.
What parameters does getTree() accept?
The method accepts two optional parameters: $rootCategoryId
(to specify a starting category) and $depth
(to limit the depth of retrieval).
What happens if I don’t provide a root category ID?
If no root category ID is provided, Magento returns the complete category tree for the store.
Where does Magento store category data?
Magento stores category data in the catalog_category_entity
table in the database.
Can I retrieve child categories of a specific category?
Yes, by passing the parent category’s ID to getTree()
, you can retrieve its child categories.
How do I display the category tree in a template file?
You can call the custom model’s getCategoryTree()
method in your template file and print the results.
What is the output format of getTree()?
The method returns a CategoryTreeInterface
object containing hierarchical category data, including child categories.
How can I optimize category tree retrieval performance?
To optimize performance, consider caching the category tree, limiting depth levels, and using Magento’s indexing mechanism.
How do I handle errors when fetching category trees?
Use a try-catch block to handle exceptions and ensure a fallback mechanism in case the category tree retrieval fails.
Can I modify the category tree programmatically?
Yes, you can programmatically modify category trees using the CategoryRepositoryInterface
to update, add, or delete categories.
Is there a way to retrieve only active categories?
Yes, by applying a filter condition to exclude disabled categories when querying the category tree.
How can I fetch category attributes along with the tree?
Use CategoryRepositoryInterface
in combination with getTree()
to retrieve additional category attributes.
Why is my category tree not displaying correctly?
Ensure that your categories are properly assigned, indexed, and enabled in the Magento admin panel.