Avoiding ObjectManager in Magento 2 Unit Tests
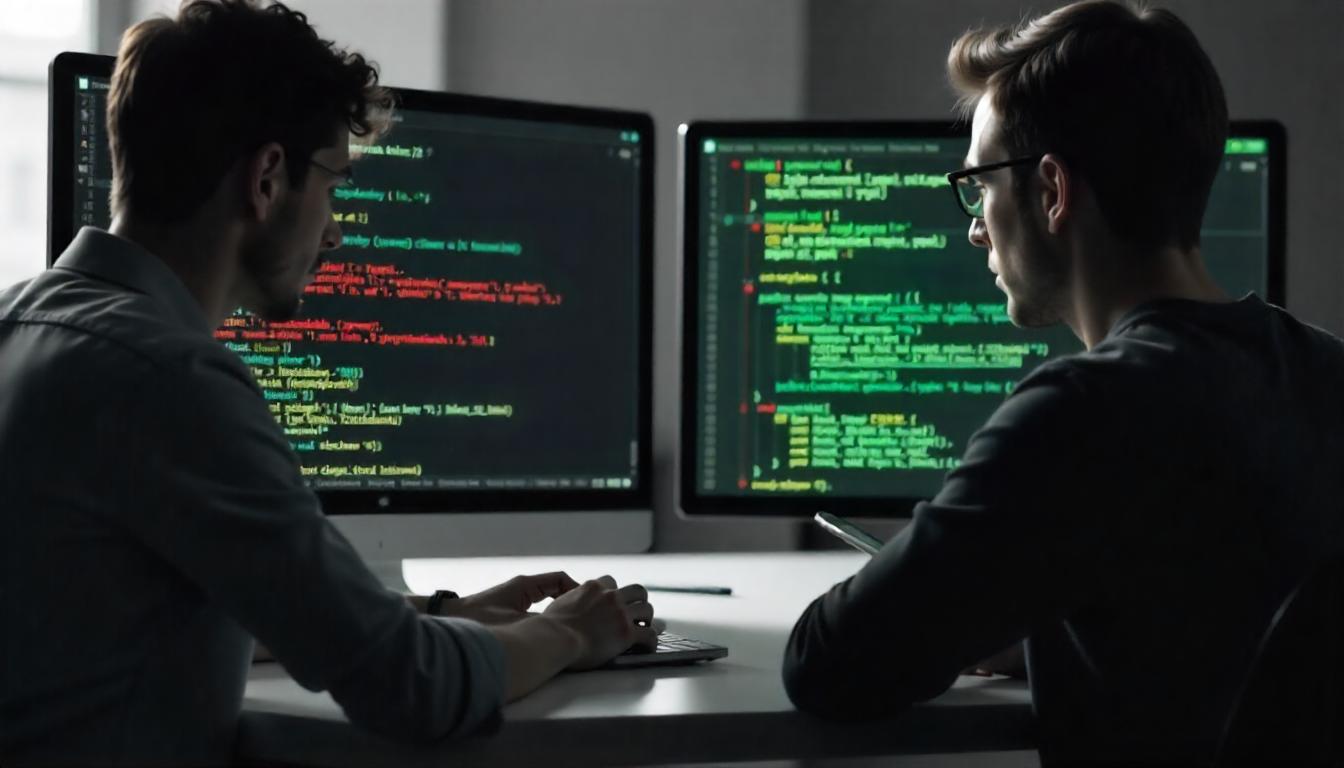
Avoiding ObjectManager in Magento 2 Unit Tests
Using ObjectManager in Magento 2 unit tests is considered bad practice because it bypasses dependency injection, making tests harder to maintain and debug. Instead, Magento recommends using mock objects and constructor injection to create testable code.
Table Of Content
Avoiding ObjectManager in Magento 2 Unit Tests
In Magento 2 unit testing, it's best to avoid using the ObjectManager
directly. Initially, developers relied on Magento\Framework\TestFramework\Unit\Helper\ObjectManager
due to unstable constructor dependencies. However, with stabilized dependencies, direct instantiation is now preferred.
Previous Approach Using ObjectManager
Previously, tests often utilized the ObjectManager
to create class instances:
<?php
use PHPUnit\Framework\TestCase;
use Magento\Framework\App\Action\Context;
use Magento\Contact\Model\ConfigInterface;
use Rbj\TestModule\Controller\Index\Index;
use Magento\Framework\TestFramework\Unit\Helper\ObjectManager;
class IndexTest extends TestCase
{
private $context;
private $configMock;
private $controller;
protected function setUp(): void
{
$objectManager = new ObjectManager($this);
$this->context = $this->getMockBuilder(Context::class)
->disableOriginalConstructor()
->getMock();
$this->configMock = $this->getMockBuilder(ConfigInterface::class)
->getMockForAbstractClass();
$this->controller = $objectManager->getObject(
Index::class,
[
'context' => $this->context,
'configMock' => $this->configMock
]
);
}
}
In this setup, the ObjectManage
r facilitated object creation with mocked dependencies.
Recommended Direct Instantiation Method
The current best practice is to instantiate classes directly:
<?php
use PHPUnit\Framework\TestCase;
use Magento\Framework\App\Action\Context;
use Magento\Contact\Model\ConfigInterface;
use Rbj\TestModule\Controller\Index\Index;
class IndexTest extends TestCase
{
private $context;
private $configMock;
private $controller;
protected function setUp(): void
{
$this->context = $this->getMockBuilder(Context::class)
->disableOriginalConstructor()
->getMock();
$this->configMock = $this->getMockBuilder(ConfigInterface::class)
->getMockForAbstractClass();
$this->controller = new Index(
$this->context,
$this->configMock
);
}
}
Here, the Index class is instantiated directly with its dependencies, aligning with Magento 2's updated testing practices.
Advantages of Direct Instantiation
- Clarity: Tests are more readable and maintainable without the additional layer of the ObjectManager.
- Isolation: Direct instantiation ensures that tests focus solely on the unit under test without unintended interactions.
- Performance: Reducing reliance on the ObjectManager can lead to faster test execution.
For effective Magento 2 unit testing, avoid using the ObjectManager. Instead, directly instantiate classes with mocked dependencies to ensure clear, efficient, and reliable tests.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
Why Should I Avoid Using ObjectManager in Magento 2 Unit Tests?
Using ObjectManager
directly in unit tests is discouraged because it adds unnecessary complexity, makes tests harder to maintain, and can lead to unexpected dependencies.
What Is the Recommended Way to Instantiate Objects in Magento 2 Unit Tests?
Instead of using ObjectManager
, you should instantiate objects directly with constructor dependencies using mock objects.
How Can I Set Up a Unit Test Without ObjectManager?
You can use PHPUnit’s getMockBuilder()
method to create mock dependencies and pass them to the class constructor.
$this->context = $this->getMockBuilder(Context::class)
->disableOriginalConstructor()
->getMock();
What Are the Benefits of Avoiding ObjectManager in Unit Tests?
- Improved Test Clarity: Tests are easier to read and maintain.
- Better Isolation: Ensures dependencies are properly mocked.
- Faster Execution: Reduces overhead compared to ObjectManager.
What Issues Might Occur When Using ObjectManager in Tests?
Common problems include:
- Unexpected Dependencies: Tests may rely on actual class dependencies instead of mocks.
- Unstable Code: Changes in class constructors can break tests using ObjectManager.
How Can I Debug Unit Test Issues Without ObjectManager?
Use PHPUnit’s built-in assertion methods to check expected results and ensure proper object mocking.
Where Can I Learn More About Magento 2 Unit Testing?
Check Magento’s official developer documentation and community forums for best practices and examples.