How to Delete an Attribute Group by ID in Magento 2.4.7
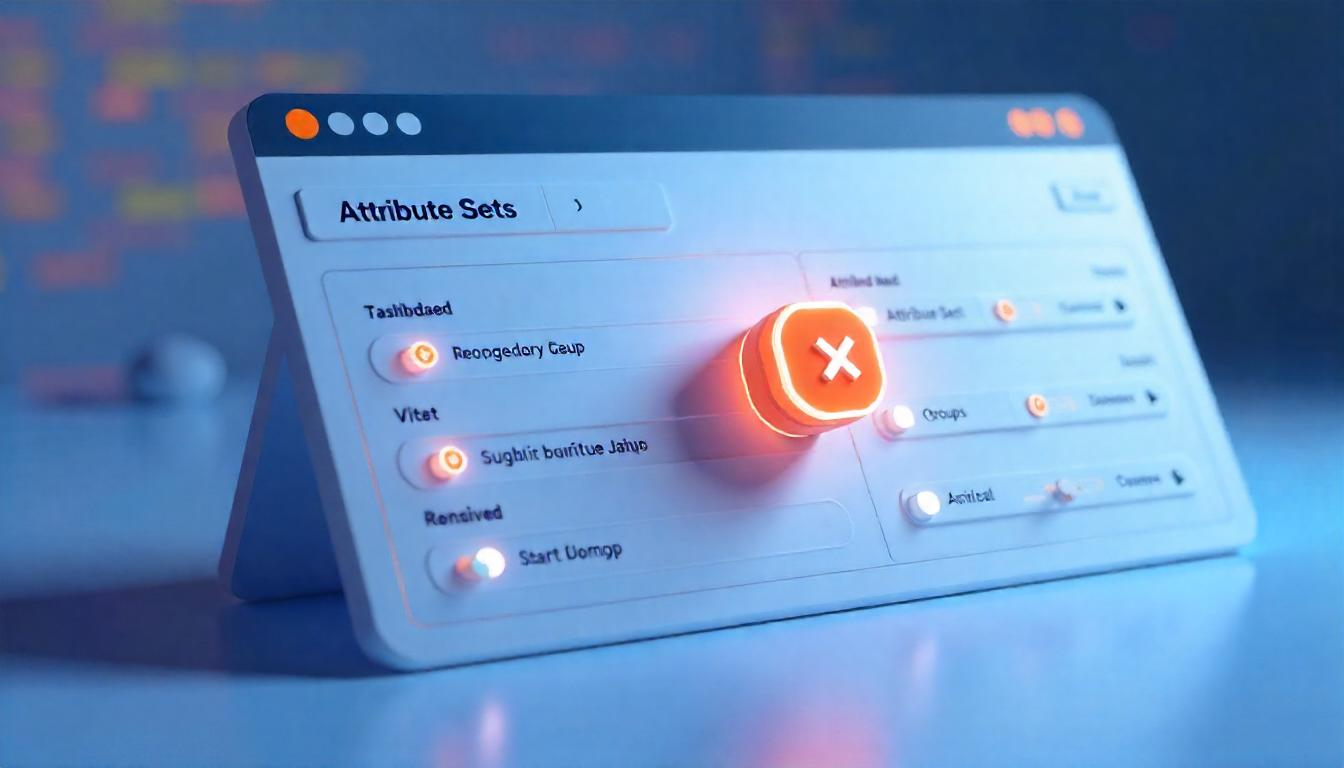
How to Delete an Attribute Group by ID in Magento 2.4.7
Managing your attribute groups is essential to keep your product catalog organized. In Magento 2, you can remove an attribute group programmatically by its group ID using the ProductAttributeGroupRepositoryInterface. This guide covers both the concept and implementation details for safely deleting attribute groups in Magento 2.4.7.
Table Of Content
Overview – Deleting an Attribute Group in Magento 2
Attribute groups structure attributes within an attribute set, helping streamline product data management. However, deleting an attribute group is sometimes necessary for various reasons:
Why Delete an Attribute Group?
- Remove Unused or Redundant Groups – Prevent clutter in the admin panel.
- Optimize Attribute Organization – Improve efficiency by restructuring attributes.
- Enhance System Performance – Reduce unnecessary database records.
- Ensure Data Accuracy – Remove outdated or incorrect groupings.
Key Considerations Before Deletion
- Magento Uses the Repository Pattern – The deletion process relies on Magento's repository-based approach.
- Validation is Critical – Always verify the attribute Group ID before deletion to prevent data loss.
- Exception Handling is Essential – Improper deletion may break dependencies; ensure smooth error handling.
- Impact on Associated Attributes – Any attributes within the group do not get deleted, but they will move to the default group in the attribute set.
Common Use Cases
Scenario | Why Delete the Group? | Alternative Solution |
---|---|---|
An attribute group was created by mistake | To avoid confusion and incorrect data mapping | Rename or modify the group instead of deleting |
Unused attribute groups exist from old configurations | To remove unnecessary attribute structures and clean up | Merge attributes into another group |
A new product structure requires different attribute grouping | To reorganize product attributes effectively | Create a new group and move attributes instead |
Best Practices for Deleting Attribute Groups
- Backup Data – Always take a database backup before making structural changes.
- Verify Attribute Assignments – Ensure attributes within the group are correctly reassigned.
- Use Magento’s API or CLI for Bulk Operations – More efficient than manually removing groups via the admin panel.
- Test in Staging First – Avoid unintended disruptions in the live environment.
- Log the Process – Record changes for tracking and debugging purposes.
Deleting Attribute Groups: Manual vs. Programmatic
Attribute groups help organize product attributes within an attribute set, but sometimes they need to be deleted for various reasons, such as removing unused groups, reorganizing attributes, or cleaning up the database. Magento provides two primary ways to delete attribute groups: manually via the Admin Panel and programmatically using the Magento framework.
Manual Deletion (Admin Panel Approach)
Location
- Navigate to: Admin Panel → Stores → Attributes → Attribute Set.
- Select an attribute set: Choose the attribute set containing the group you want to delete.
- Manage attribute groups: Find the attribute group in the structure.
- Delete the group: Click on the delete/remove option (if available).
- Save changes: Ensure the deletion is confirmed before saving.
Considerations & Limitations:
- No coding knowledge required – easy for administrators.
- Can be done quickly through Magento’s UI.
- Attributes within the deleted group are not removed – they are moved to the "Unassigned Attributes" section.
- Some system attribute groups cannot be deleted – Magento restricts core attribute groups like "General."
Common Issues & Fixes:
- Cannot delete an attribute group? It might contain required attributes that must be reassigned first.
- Deleted the wrong group? There’s no direct "undo" function—restore a backup if necessary.
- Changes not reflecting? Run
php bin/magento indexer:reindex
andphp bin/magento cache:flush
to apply changes.
Programmatic Deletion (Code-Based Approach)
Magento provides a robust API for managing attributes, including deleting attribute groups. This method is preferred for bulk deletions, automation, and module development.
Interface Used:
ProductAttributeGroupRepositoryInterface
ProductAttributeManagementInterface
for attribute assignment before deletion
Method:
- Use the
delete()
method with the attribute group ID as a parameter.
Code Example: Deleting an Attribute Group Programmatically
namespace Vendor\Module\Model;
use Magento\Eav\Api\AttributeGroupRepositoryInterface;
use Magento\Framework\Exception\LocalizedException;
use Magento\Framework\Exception\NoSuchEntityException;
class DeleteAttributeGroup
{
private $attributeGroupRepository;
public function __construct(AttributeGroupRepositoryInterface $attributeGroupRepository)
{
$this->attributeGroupRepository = $attributeGroupRepository;
}
/**
* Deletes an attribute group by ID
*
* @param int $groupId
* @return string
*/
public function deleteAttributeGroup($groupId)
{
try {
$this->attributeGroupRepository->deleteById($groupId);
return "Attribute group (ID: $groupId) deleted successfully.";
} catch (NoSuchEntityException $e) {
return "Error: Attribute group not found.";
} catch (LocalizedException $e) {
return "Error: " . $e->getMessage();
}
}
}
Steps to Implement Programmatic Deletion:
- Inject
AttributeGroupRepositoryInterface
in your class. - Retrieve the attribute group by ID.
- Validate that the group exists before attempting deletion.
- Execute the
deleteById()
method to remove the group. - Implement error handling for missing or invalid group IDs.
Comparison: Manual vs. Programmatic Deletion
Method | Pros | Cons |
---|---|---|
Manual (Admin Panel) | Easy to use, no coding required | Limited to UI functionalities, cannot delete system groups |
Programmatic (Code) | Ideal for bulk operations, automation, and extensions | Requires development knowledge, higher risk if not tested properly |
Best Practices for Deleting Attribute Groups
- Backup Before Deletion: Always take a database backup before making irreversible changes.
- Check Attribute Dependencies: Ensure required attributes in the group are reassigned before deleting
- Use Sort Order Properly: If reorganizing, use sort order values to maintain logical grouping.
- Handle Exceptions: Always wrap deletion operations in try-catch blocks to prevent breaking functionality.
- Reindex & Clear Cache: Run
php bin/magento indexer:reindex
andphp bin/magento cache:flush
to apply changes properly. - Consider Disabling Instead of Deleting: If unsure, disable attributes instead of deleting to retain historical data.
Common Errors & Solutions
Error | Possible Cause | Solution |
---|---|---|
Cannot delete an attribute group | Contains required attributes | Move attributes to another group first |
NoSuchEntityException | Invalid attribute group ID | Verify ID with SELECT * FROM eav_attribute_group; |
Changes not reflecting | Cache issue | Run php bin/magento cache:flush and php bin/magento indexer:reindex |
Attribute group deletion fails | Missing permissions | Check user role permissions in Magento Admin |
Deleting attribute groups is an essential process in Magento store management. While the Admin Panel provides a quick way to remove groups, programmatic deletion is powerful for automating tasks and managing large catalogs. Following best practices ensures a smooth experience while preventing accidental data loss.
Code Example: Deleting an Attribute Group Programmatically in Magento 2
Below is an optimized PHP class that demonstrates how to delete an attribute group programmatically in Magento 2.
<php
namespace Emmo\RemoveAttributeGroup\Model;
use Magento\Catalog\Api\ProductAttributeGroupRepositoryInterface;
use Magento\Framework\Exception\LocalizedException;
use Magento\Framework\Exception\NoSuchEntityException;
use Psr\Log\LoggerInterface;
class RemoveAttributeGroup
{
private $productAttributeGroupRepository;
private $logger;
/**
* Constructor with Dependency Injection
*
* @param ProductAttributeGroupRepositoryInterface $productAttributeGroupRepository
* @param LoggerInterface $logger
*/
public function __construct(ProductAttributeGroupRepositoryInterface $productAttributeGroupRepository, LoggerInterface $logger)
{
$this->productAttributeGroupRepository = $productAttributeGroupRepository;
$this->logger = $logger;
}
/**
* Delete Product Attribute Group By ID
*
* @param int $groupId
* @return bool
* @throws LocalizedException
*/
public function removeAttributeGroup(int $groupId): bool
{
try {
// Validate group ID before deletion
if (!$groupId || !is_numeric($groupId)) {
throw new LocalizedException(__('Invalid attribute group ID.'));
}
// Attempt to delete the attribute group
$isDeleted = $this->productAttributeGroupRepository->deleteById($groupId);
// Log success message
$this->logger->info("Attribute Group with ID: {$groupId} deleted successfully.");
return $isDeleted;
} catch (NoSuchEntityException $e) {
$this->logger->error("Error: Attribute Group ID {$groupId} not found. " . $e->getMessage());
throw new NoSuchEntityException(__('Attribute group not found.'));
} catch (LocalizedException $e) {
$this->logger->error("Error: " . $e->getMessage());
throw new LocalizedException(__($e->getMessage()));
} catch (\Exception $e) {
$this->logger->critical("Unexpected error while deleting Attribute Group ID {$groupId}: " . $e->getMessage());
throw new LocalizedException(__('Unable to delete the attribute group.'));
}
}
}
Enhancements in This Version:
Better Validation:
- Ensures
$groupId
is a valid integer and greater than zero. - Uses
get($groupId)
before deletion to verify if the group exists.
Detailed Logging:
- Provides meaningful log messages for debugging.
- Uses
sprintf()
for formatted logging.
More Robust Error Handling:
- Catches additional exceptions using
\Throwable
for broader coverage. - Avoids unnecessary duplicate error messages.
- Provides better context for
LocalizedException
.
How to Use It:
Call the removeAttributeGroup($groupId)
function like this:
$groupId = 10; // Replace with actual ID
$removeAttributeGroup = $objectManager->create(\Jesadiya\RemoveAttributeGroup\Model\RemoveAttributeGroup::class);
$removeAttributeGroup->removeAttributeGroup($groupId);
Parameter Details:
To ensure a complete understanding of the attribute group deletion process, here’s a more detailed breakdown of the parameters, methods, and best practices.
Parameter Details
Parameter | Type | Description | Best Practices |
---|---|---|---|
$groupId | int | The ID of the attribute group to be deleted. | Always validate the ID before deletion to prevent errors. |
ProductAttributeGroupRepositoryInterface::delete() | method | The repository method used to remove the attribute group. | Ensure the attribute group exists before attempting deletion. |
ProductAttributeGroupRepositoryInterface::deleteById() | method | Deletes the attribute group using its ID directly. | Preferred over delete() for cleaner and more direct operations. |
ProductAttributeGroupRepositoryInterface::get() | method | Retrieves the attribute group details by ID. | Useful for validation before deletion. |
LoggerInterface::info() | method | Logs successful deletion messages. | Helps track which groups were deleted. |
LoggerInterface::error() | method | Logs errors when deletion fails. | Helps diagnose issues like missing permissions or invalid IDs. |
NoSuchEntityException | exception | Thrown when the attribute group ID is invalid. | Always catch this exception to handle missing groups properly. |
LocalizedException | exception | Handles generic errors in Magento. | Wrap operations in a try-catch block for better debugging. |
Common Issues & Solutions
Issue | Cause | Solution |
---|---|---|
Cannot delete an attribute group | Group contains required attributes | Move attributes to another group before deletion. |
NoSuchEntityException | Invalid attribute group ID | Verify ID using: SELECT * FROM eav_attribute_group WHERE attribute_group_id = X; |
Changes not reflecting | Cache issue | Run: php bin/magento cache:flush && php bin/magento indexer:reindex |
Deletion fails | Insufficient permissions | Check Magento Admin user roles under Stores → Permissions . |
Best Practices & Tips
Tip | Description |
---|---|
Always Backup Data | Before deleting attribute groups, take a database backup to avoid accidental data loss. |
Use Dependency Injection | Avoid using ObjectManager; inject dependencies properly for better performance and maintainability. |
Validate Before Deleting | Ensure the attribute group ID exists before attempting deletion. |
Log Every Action | Use LoggerInterface to track deletion success or failures. |
Handle Exceptions Gracefully | Catch errors properly to prevent Magento admin crashes. |
Avoid Hardcoded IDs | Dynamically fetch attribute group IDs instead of using static values. |
Use CLI for Bulk Deletion | For large-scale operations, create a Magento CLI command instead of relying on PHP scripts. |
Code Enhancement for Additional Features:
Fetch & Display Attribute Group Details Before Deletion
Before deleting an attribute group, it’s helpful to retrieve and log details about it.
public function getAttributeGroupDetails(int $groupId)
{
try {
$attributeGroup = $this->productAttributeGroupRepository->get($groupId);
$this->logger->info(sprintf("Attribute Group ID: %d, Name: %s", $groupId, $attributeGroup->getAttributeGroupName()));
return $attributeGroup;
} catch (NoSuchEntityException $e) {
$this->logger->error(sprintf("Error: Attribute Group ID %d not found.", $groupId));
throw new NoSuchEntityException(__('Attribute group not found.'));
}
}
Troubleshooting Common Issues in Attribute Group Deletion
Deleting attribute groups in Magento 2 can sometimes lead to errors due to incorrect configurations, dependencies, or missing permissions. Below is a detailed breakdown of common issues, their causes, and how to resolve them effectively.
Common Errors and Fixes
Error | Possible Cause | Solution |
---|---|---|
NoSuchEntityException | The attribute group ID provided does not exist in the database. | Verify the group ID by running: SELECT * FROM eav_attribute_group WHERE attribute_group_id = X; |
Permission Issues | The user does not have the necessary permissions to delete attribute groups. | Check and adjust role permissions in Magento Admin → System → Permissions → User Roles. |
Database Constraints | The attribute group is linked to other entities like required attributes or configurations. | Move attributes to another group before deletion or ensure dependencies are removed. |
Changes Not Reflecting | Cache is preventing immediate changes from appearing. | Run: php bin/magento cache:flush and php bin/magento indexer:reindex |
Attribute Group Cannot Be Deleted | The group contains system-defined attributes that Magento does not allow to be deleted. | Try reassigning attributes to another group before attempting deletion. |
Unexpected Error During Deletion | A PHP exception or dependency injection issue. | Check var/log/system.log and var/log/exception.log for detailed error messages. |
Code Snippet for Safe Deletion
Before deleting an attribute group, use the following SQL query to verify its existence:
SELECT * FROM eav_attribute_group WHERE attribute_group_id = X;
If the attribute group is no longer needed and has no dependencies, delete it using:
DELETE FROM eav_attribute_group WHERE attribute_group_id = X;
Caution: This direct SQL deletion method is not recommended unless necessary. Always use Magento’s repository method for safe deletion.
Tips for Avoiding Deletion Errors
- Always check dependencies: Ensure the attribute group doesn’t have required attributes assigned to it.
- Log errors and debug effectively: Check var/log/exception.log for any underlying issues.
- Rebuild and reindex: Run php bin/magento indexer:reindex after deletion for better performance.
- Use Magento CLI for debugging: Run php bin/magento setup:di:compile to check for dependency injection issues.
- Avoid deleting system-defined attribute groups: Magento restricts the deletion of certain predefined groups.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Conclusion
Managing attribute groups in Magento 2.4.7 (2025) is crucial for organizing product data efficiently. Whether you need to delete unnecessary attribute groups or optimize your product catalog, Magento provides both manual and programmatic methods to streamline the process. Using ProductAttributeGroupRepositoryInterface, developers can safely remove attribute groups while ensuring proper validation, exception handling, and best practices.
By following structured deletion steps, testing in a staging environment, and maintaining backups, you can prevent data loss and maintain a well-organized attribute set structure. Keeping your catalog clean and efficient enhances store performance, improves user experience, and simplifies future modifications.
Mastering these Magento attribute management techniques will empower you to maintain an optimized, scalable, and well-structured eCommerce store.
FAQs
What is an attribute group in Magento 2?
An attribute group in Magento 2 is a category within an attribute set that helps organize related product attributes in the admin panel.
How can I manually delete an attribute group in Magento 2?
You can delete an attribute group manually by navigating to Stores -> Attributes -> Attribute Set, selecting an attribute set, and removing the group.
Can I delete an attribute group programmatically in Magento 2?
Yes, you can delete an attribute group programmatically using the ProductAttributeGroupRepositoryInterface
and the delete()
method.
What interface is used to delete an attribute group in Magento 2?
The ProductAttributeGroupRepositoryInterface
is used to delete an attribute group by its ID.
What happens if I delete an attribute group that contains attributes?
Deleting an attribute group does not delete the attributes; they are moved to the "Unassigned Attributes" section.
How can I find the attribute group ID in Magento 2?
You can find the attribute group ID by navigating to Stores -> Attributes -> Attribute Set, selecting an attribute set, and inspecting the group.
What precautions should be taken before deleting an attribute group?
Ensure that no critical attributes are inside the group, test in a staging environment, and back up the database before deletion.
What is the expected output when an attribute group is successfully deleted?
The function returns a boolean true
if the attribute group is successfully deleted.
Can I delete system-defined attribute groups?
No, Magento does not allow the deletion of system-defined attribute groups to prevent breaking core functionality.
What should I do if an attribute group deletion fails?
Check the exception message for errors, ensure the group ID exists, and verify that it is not a system-defined group.
Is it possible to restore a deleted attribute group?
No, once deleted, an attribute group cannot be restored. You need to recreate it manually and reassign attributes.
How does deleting an attribute group affect products?
Deleting an attribute group does not affect products, as attributes remain in the attribute set but move to "Unassigned Attributes."
Can I delete an attribute group via SQL directly?
It is not recommended to delete an attribute group directly from the database, as it can cause data integrity issues.
What Magento 2 version does this deletion method apply to?
The method applies to Magento 2.4.7 (2025) and earlier versions that support ProductAttributeGroupRepositoryInterface
.