How to Get EAV Attribute Group Details by ID in Magento 2.4.7
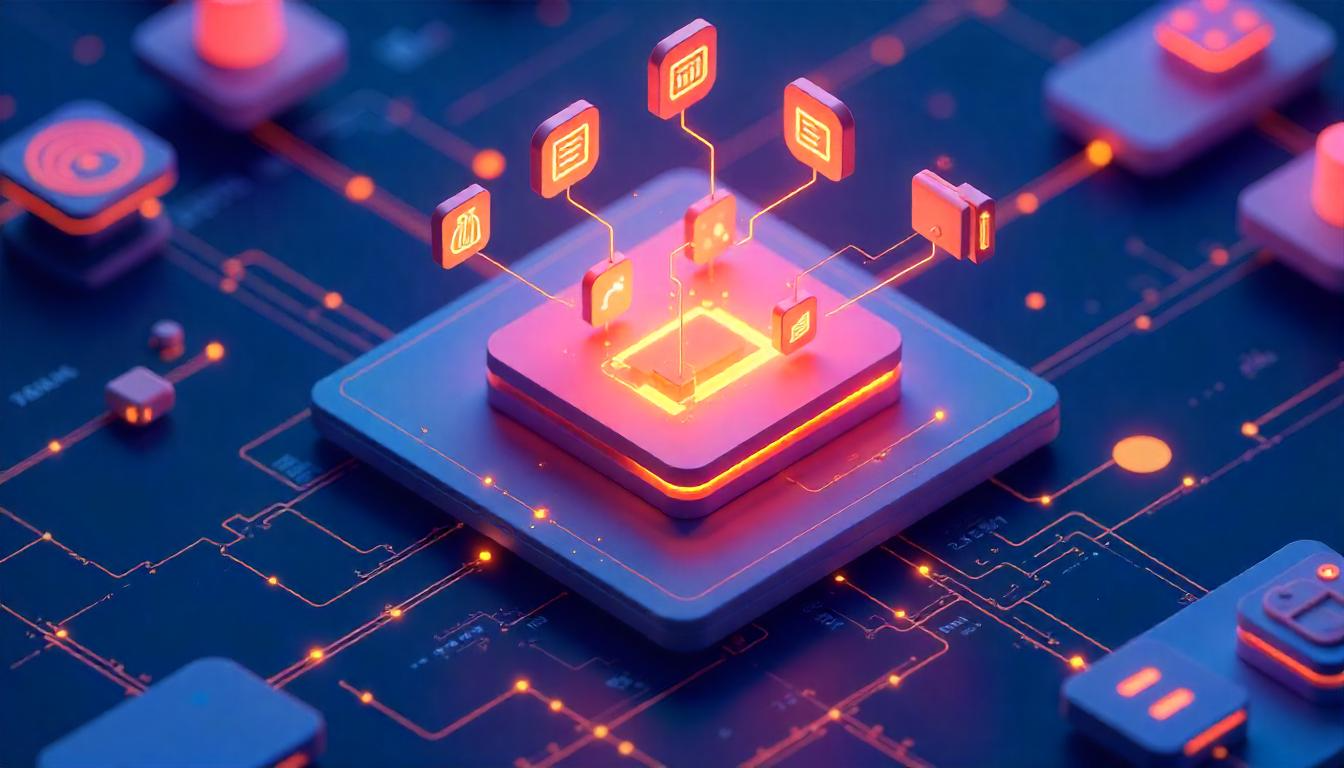
How to Get EAV Attribute Group Details by ID in Magento 2.4.7
Magento uses the EAV (Entity-Attribute-Value) model for flexible product data management. Retrieving attribute group details by its ID is useful when you need to inspect, debug, or customize how attribute groups are handled in your store. In this guide, we use the native method from ProductAttributeGroupRepositoryInterface to fetch details such as the attribute group ID, attribute set ID, group name, sort order, default ID, attribute group code, and tab group code.
Table Of Content
Understanding Magento 2 EAV Attribute Groups
Magento 2 follows the Entity-Attribute-Value (EAV) model, where attribute groups serve as logical containers to structure product attributes within an attribute set. Retrieving attribute group details is essential for various development and operational tasks, including:
Why Retrieve Attribute Group Data?
Purpose | Description |
---|---|
Debugging | Identify if the correct attribute group is assigned to products. |
Customization | Modify attribute groups for specific requirements or UI changes. |
Integration | Use attribute data in custom modules, APIs, or third-party systems. |
Performance Optimization | Ensure attributes are properly structured to improve database efficiency. |
Bulk Operations | Automate attribute assignments for multiple products. |
Key Components of Attribute Groups
Concept | Description |
---|---|
Attribute Group ID | Unique identifier that differentiates each attribute group. |
Attribute Set ID | Defines which attribute set the group belongs to. |
Attribute Sorting | Attributes within a group follow a specific sort order for better organization. |
System Groups | Certain groups, like “Product Details,” are mandatory and cannot be deleted. |
Custom Groups | Groups manually added via Admin Panel or programmatically. |
Fetching attribute group details in Magento 2 is essential for customization, debugging, and integration. Using the repository pattern ensures efficient data retrieval, while following best practices minimizes errors and improves performance.
Code Implementation: Retrieving Attribute Group Details in Magento 2
In Magento 2, attribute groups help structure product attributes within attribute sets. Retrieving attribute group details can be useful for debugging, customization, integration, and automation. Below, we’ll walk through a programmatic approach to fetching attribute group data using a custom model.
1. Creating the Model
To retrieve attribute group details by ID, create a model file (e.g., ProductAttributeGroup.php
) in your custom module under Emmo\AttributeGroup\Model
. This model will interact with Magento’s ProductAttributeGroupRepositoryInterface
to fetch attribute group details.
2. Code Example
Below is a PHP class that demonstrates how to fetch attribute group details:
<?php
namespace Emmo\AttributeGroup\Model;
use Exception;
use Magento\Eav\Api\Data\AttributeGroupInterface;
use Magento\Catalog\Api\ProductAttributeGroupRepositoryInterface;
use Psr\Log\LoggerInterface;
class ProductAttributeGroup
{
/**
* @var ProductAttributeGroupRepositoryInterface
*/
private $productAttributeGroupRepository;
/**
* @var LoggerInterface
*/
private $logger;
/**
* Constructor with dependency injection.
*
* @param ProductAttributeGroupRepositoryInterface $productAttributeGroupRepository
* @param LoggerInterface $logger
*/
public function __construct(
ProductAttributeGroupRepositoryInterface $productAttributeGroupRepository,
LoggerInterface $logger
) {
$this->productAttributeGroupRepository = $productAttributeGroupRepository;
$this->logger = $logger;
}
/**
* Retrieve Product Attribute Group Details by ID.
*
* @param int $groupId
* @return AttributeGroupInterface
* @throws Exception
*/
public function getAttributeGroupById(int $groupId)
{
try {
if (!$groupId || !is_numeric($groupId)) {
throw new Exception('Invalid attribute group ID.');
}
$productAttributeGroup = $this->productAttributeGroupRepository->get($groupId);
$this->logger->info("Attribute Group ID {$groupId} retrieved successfully.");
return $productAttributeGroup;
} catch (Exception $exception) {
$this->logger->error("Error retrieving Attribute Group ID {$groupId}: " . $exception->getMessage());
throw new Exception($exception->getMessage());
}
}
}
Explanation of Code
Component | Description |
---|---|
Dependency Injection | The constructor injects ProductAttributeGroupRepositoryInterface, ensuring modular and testable code. |
getAttributeGroupById() Method | Uses $this->productAttributeGroupRepository->get($groupId) to fetch attribute group details. |
Validation | Checks if $groupId is a valid numeric value before proceeding. |
Logging | Uses LoggerInterface to log both success and failure cases. |
Error Handling | Catches any exception and logs an error message for debugging. |
Expected Output
When the method is executed, it returns an instance of
Magento\Eav\Api\Data\AttributeGroupInterface
. Below is a sample output in array format:
array (
'attribute_group_id' => '1',
'attribute_set_id' => '1',
'attribute_group_name' => 'General',
'sort_order' => '1',
'default_id' => '1',
'attribute_group_code' => 'general',
'tab_group_code' => null,
)
Using the Function in Your Code
You can use this method in another class or a template file to retrieve attribute group details dynamically:
$attributeDetails = $this->getAttributeGroupById(1);
var_dump($attributeDetails);
This will print the attribute group details, which can be used in custom modules, UI components, or API integrations.
Additional Considerations
Scenario | Solution |
---|---|
Invalid Group ID | Ensure the ID exists in eav_attribute_group before calling the method. |
Permission Issues | Verify user roles in Magento Admin under System → Permissions → User Roles . |
Caching Issues | Run php bin/magento cache:flush and php bin/magento indexer:reindex to reflect recent changes. |
Performance Optimization | If fetching multiple groups, consider using collection models for efficiency. |
Pro Tip:
- Use Dependency Injection Properly: Always inject repository interfaces instead of directly using object managers.
- Log Every Critical Operation: Logging helps track issues when working with attribute groups.
- Handle Large Data Efficiently: For bulk operations, retrieve groups using a collection rather than fetching one at a time.
- Ensure Attribute Set Integrity: Deleting or modifying groups incorrectly can disrupt attribute set configurations.
Attributes Details
Below is a detailed breakdown of key attributes returned when retrieving an attribute group in Magento 2.
Attribute Group Fields
Attribute Key | Description |
---|---|
attribute_group_id | Unique identifier for the attribute group. Used for referencing the group programmatically. |
attribute_set_id | The ID of the attribute set that this group belongs to. Helps in organizing attributes under a specific set. |
attribute_group_name | The human-readable name of the attribute group (e.g., "General"). This appears in the Magento Admin. |
sort_order | Determines the order in which attribute groups appear within an attribute set. Lower numbers appear first. |
default_id | Internal system identifier, used by Magento for managing default groups. |
attribute_group_code | A machine-readable code assigned to the attribute group. Useful for programmatic access. |
tab_group_code | Defines the tab where the attribute group appears in the admin panel (nullable). |
Use Cases of Attribute Groups
Understanding attribute groups is essential for structuring product data effectively. Here are some common use cases:
1. Product Management
- Helps in organizing product attributes logically.
- Makes it easier for store admins to manage product data.
2. Customization and Theming
- Developers can create custom attribute groups for better UI/UX in the admin panel.
3. API Integration
- Attribute groups are used in API calls when fetching or updating product attributes programmatically.
4. Bulk Operations
- Allows bulk modifications of attributes using scripts or Magento’s admin panel.
Best Practices for Managing Attribute Groups
- Use Meaningful Names: Choose clear names like "Technical Specifications" instead of generic ones like "Group 1."
- Sort Logically: Assign sort orders to maintain a structured display in the admin panel.
- Keep Groups Small: Large groups can slow down data entry; categorize attributes efficiently.
- Use Codes Consistently: Follow a naming convention for
attribute_group_code
values to maintain consistency.
Troubleshooting Common Issues
Issue | Cause | Solution |
---|---|---|
Attribute Group Not Found | The attribute_group_id does not exist in the database. | Verify the group ID using: SELECT * FROM eav_attribute_group WHERE attribute_group_id = X; |
Cannot Delete Group | Contains system-required attributes. | Move the attributes to another group before attempting deletion. |
Changes Not Visible | Cache is preventing updates from appearing. | Run: php bin/magento cache:flush php bin/magento indexer:reindex |
Attribute Group Name Not Updating | Admin changes may not be saved properly. | Re-save and check the eav_attribute_group table to confirm updates. |
Tip: Fetching Attribute Group Details Programmatically
If you need to fetch attribute group details in Magento 2 programmatically, use the following method:
$groupId = 1; // Replace with the actual group ID
$attributeGroup = $this->productAttributeGroup->get($groupId);
var_dump($attributeGroup);
This will return an array with the attributes mentioned in the table above.
Best Practices and Tips for Handling Attribute Groups in Magento 2
Tip | Description |
---|---|
Use Dependency Injection (DI) | Always inject interfaces via the constructor rather than instantiating objects directly. This ensures modular, testable, and maintainable code. |
Dynamic Group ID | Instead of hardcoding the group ID, pass it dynamically to make the function more reusable and adaptable to different scenarios. |
Error Logging | Utilize Magento’s LoggerInterface to log errors and warnings, helping diagnose issues if an attribute group is not found. |
Caching Data | If attribute group details are frequently used, consider caching them to improve performance and reduce database queries. |
Validate Input | Always validate the provided group ID to ensure it is numeric and exists in the eav_attribute_group table before performing operations. |
Follow Magento Coding Standards | Adhere to Magento's best practices, using service contracts instead of direct model calls for better maintainability. |
Troubleshooting Common Issues
Issue | Cause | Solution |
---|---|---|
Attribute Group Not Found | The attribute_group_id does not exist in the database. |
Verify the group ID using:SELECT * FROM eav_attribute_group WHERE attribute_group_id = X;
|
Cannot Delete Group | The group contains system-required attributes that cannot be deleted. | Move the attributes to another group before attempting deletion. |
Changes Not Visible | Cache is preventing updates from appearing. | Run:php bin/magento cache:flush php bin/magento indexer:reindex
|
Attribute Group Name Not Updating | Admin changes may not be saved properly. | Re-save and check the eav_attribute_group table to confirm updates. |
Performance Issues | Fetching attribute groups frequently can slow down performance. | Use a collection model for bulk retrieval instead of multiple single queries. |
Permission Errors | The user lacks necessary permissions. | Check and adjust role permissions in Magento Admin: System → Permissions → User Roles |
Advanced Optimizations for Attribute Group Handling
1. Use Collection Models for Bulk Data Retrieval
Instead of fetching attribute groups one by one, use Magento’s Collection
models to retrieve multiple records efficiently:
use Magento\Eav\Model\ResourceModel\Entity\Attribute\Group\CollectionFactory;
class AttributeGroupList
{
private $collectionFactory;
public function __construct(CollectionFactory $collectionFactory)
{
$this->collectionFactory = $collectionFactory;
}
public function getAttributeGroupsBySetId($attributeSetId)
{
return $this->collectionFactory->create()
->addFieldToFilter('attribute_set_id', $attributeSetId)
->setOrder('sort_order', 'ASC');
}
}
2. Implement Proper Logging and Debugging
Logging errors can help diagnose issues when fetching or deleting attribute groups:
use Psr\Log\LoggerInterface;
class AttributeGroupHandler
{
private $logger;
public function __construct(LoggerInterface $logger)
{
$this->logger = $logger;
}
public function logError($message)
{
$this->logger->error("Attribute Group Error: " . $message);
}
}
3. Use Magento’s Cache for Performance Improvements
If you frequently retrieve the same attribute group data, consider caching it using Magento’s cache system:
use Magento\Framework\App\CacheInterface;
class AttributeGroupCache
{
private $cache;
private $cacheKey = 'custom_attribute_group_cache';
public function __construct(CacheInterface $cache)
{
$this->cache = $cache;
}
public function saveToCache($data)
{
$this->cache->save(serialize($data), $this->cacheKey);
}
public function loadFromCache()
{
return unserialize($this->cache->load($this->cacheKey));
}
}
Final Recommendations
- Regularly Monitor Logs: Keep track of logs in
var/log/system.log
andvar/log/exception.log
to detect issues early. - Optimize Queries: Avoid unnecessary database queries by using caching and collection models.
- Ensure Role-Based Access: Restrict access to attribute group modifications based on user roles in Magento Admin.
- Use REST or GraphQL APIs for External Integrations: If you need to fetch or modify attribute groups externally, use Magento’s APIs instead of direct database manipulation.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Conclusion
Retrieving EAV attribute group details by ID in Magento 2.4.7 is a crucial process for developers working with attribute management and customization. By leveraging ProductAttributeGroupRepositoryInterface, you can efficiently access important attribute group details, such as attribute set ID, group name, sort order, and attribute group code. This allows for better debugging, customization, and integration with third-party extensions.
Implementing this solution following Magento’s best practices, such as dependency injection, error handling, caching, and performance optimization, ensures scalability and maintainability. Additionally, dynamically handling attribute group IDs and logging errors can help prevent issues and improve system stability.
By following this guide, you now have a structured approach to fetching and utilizing attribute group data effectively. Whether you are optimizing your store’s attribute structure, debugging EAV issues, or integrating with external systems, this method provides a reliable, Magento-standard approach to working with attribute groups. Keep refining your Magento development skills, and happy coding!
FAQs
What is an attribute group in Magento 2?
An attribute group in Magento 2 is a category within an attribute set that helps organize related product attributes in the admin panel.
How do I retrieve attribute group details by ID in Magento 2?
You can retrieve attribute group details using the ProductAttributeGroupRepositoryInterface and the get($groupId) method in Magento 2.
Which interface is used to fetch attribute group details?
The ProductAttributeGroupRepositoryInterface is used to retrieve attribute group details in Magento 2.
What details can be retrieved using attribute group ID?
You can retrieve details such as attribute_group_id, attribute_set_id, group name, sort order, default ID, attribute group code, and tab group code.
What is the default attribute group ID in Magento 2?
The default attribute group ID varies based on the attribute set. The "General" group is commonly assigned ID 1.
Can I modify an attribute group in Magento 2 programmatically?
Yes, you can modify attribute groups programmatically using the Magento APIs, specifically the AttributeGroupRepositoryInterface.
How do I get all attribute groups for an attribute set?
You can retrieve all attribute groups by calling the getList() method of ProductAttributeGroupRepositoryInterface with the attribute set ID.
What happens if an invalid attribute group ID is provided?
If an invalid attribute group ID is provided, Magento will throw a "No such entity" exception.
Where are attribute groups used in Magento 2?
Attribute groups are used in the Magento 2 admin panel under product attributes to organize attributes in a structured manner.
Can I create a new attribute group programmatically?
Yes, you can create a new attribute group programmatically using the AttributeGroupRepositoryInterface and the save() method.
How do I remove an attribute group in Magento 2?
You can delete an attribute group programmatically using the deleteById() method of ProductAttributeGroupRepositoryInterface.
Can attribute groups be assigned to multiple attribute sets?
No, each attribute group is specific to a single attribute set and cannot be shared across multiple sets.
How can I fetch attribute groups using GraphQL?
Currently, Magento 2 does not provide direct GraphQL queries for attribute groups, but you can extend GraphQL schema to include custom queries.
Is caching required when working with attribute groups?
Yes, to improve performance, caching attribute group details is recommended, especially when frequently retrieving them.
What is the purpose of the tab group code in attribute groups?
The tab group code determines under which tab the attribute group appears in the admin product edit form.