How to Get Assigned Sources of Stock in Multi-Source Inventory (MSI) – Magento 2.4.7
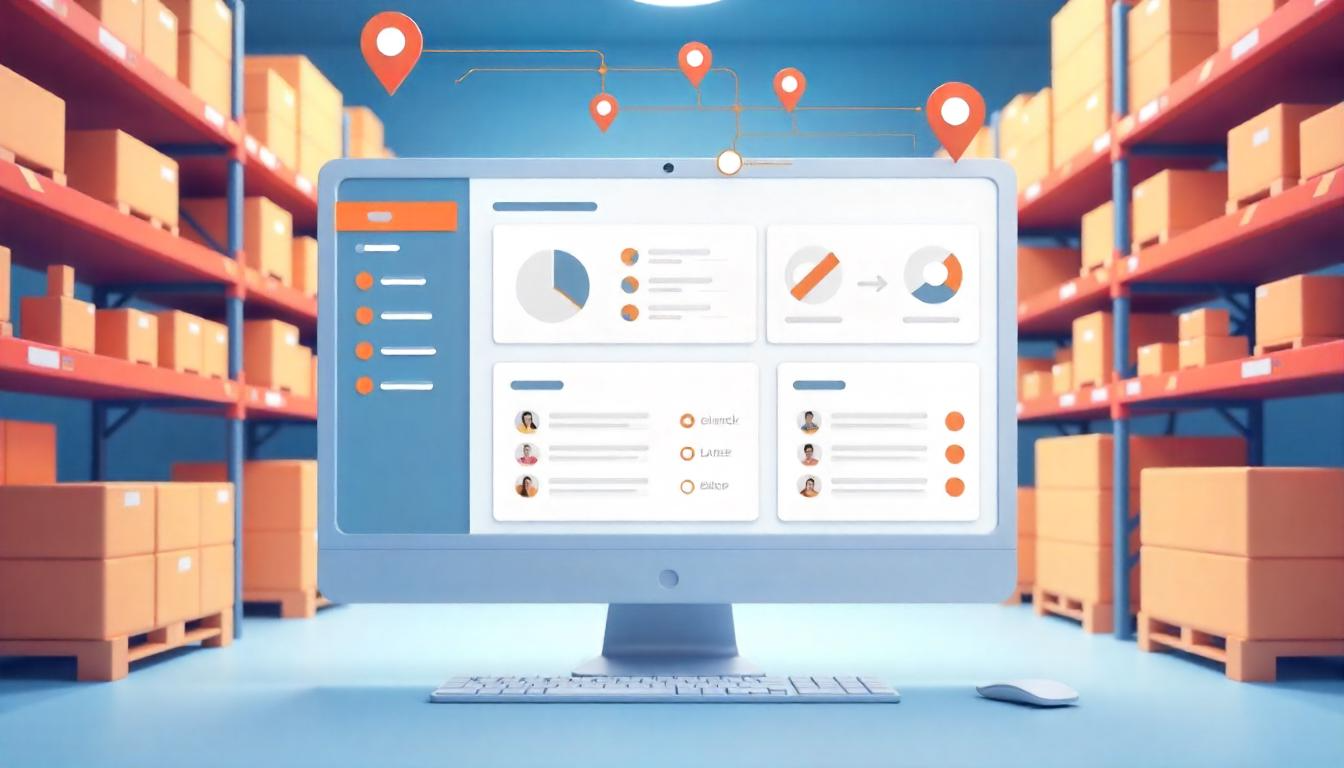
How to Get Assigned Sources of Stock in Multi-Source Inventory (MSI) – Magento 2.4.7
Magento 2.4.7 (2025) supports robust Multi-Source Inventory (MSI) functionality, allowing merchants to manage inventory across multiple locations. Understanding how to programmatically retrieve assigned sources for a specific stock is crucial for developers working on inventory customizations, logistics integrations, or reporting.
Table Of Content
Understanding Assigned Sources in Magento 2 MSI
Magento 2 introduced Multi-Source Inventory (MSI) to support merchants managing inventory across multiple physical locations. One of the core concepts in MSI is Assigned Sources—the physical locations linked to a Stock that determine how product quantities are managed and fulfilled
What Are Assigned Sources?
An Assigned Source in MSI refers to a physical or virtual location where inventory is stored or managed. These can include:
- Warehouses
- Retail stores
- Fulfillment centers
- Third-party drop shippers
- Manufacturing locations
Each Stock in Magento can have one or more Sources assigned to it. Magento uses these assigned sources to calculate available inventory for a specific website, determine shipping origin during checkout, and handle order fulfillment processes.
Purpose of Assigned Sources
Assigned sources help Magento manage inventory dynamically across multiple channels and locations. They ensure that customers get accurate product availability based on their region and the configured shipping rules.
How Assigned Sources Work
Magento connects the dots between websites, stocks, and sources in the following way:
- Each Website is linked to a Stock
- Each Stock is associated with one or more Sources
- Products are assigned quantities per source
- Source selection algorithms determine which source to fulfill the order from
Assigned Source Details
Element | Description |
---|---|
Source | A location that physically (or virtually) stores products (e.g., Main Warehouse, Retail Store #1) |
Stock | A virtual grouping of sources, assigned to a website (e.g., US Stock, EU Stock) |
Assigned Source | A source explicitly linked to a stock. The same source can be used in multiple stocks |
Quantity | Inventory assigned per source, allowing decentralized stock management |
Priority | Determines which source to use first during the source selection process |
Key Concepts in Depth
1. Physical vs Virtual Sources
Sources can be actual physical locations (warehouses, stores) or logical locations (drop shippers). They don’t have to represent a single geographic place; for example, a drop shipper could be defined as a "virtual source."
2. Source Assignment to Stock
You can assign multiple sources to a single stock, allowing Magento to aggregate inventory across multiple points and calculate availability per website.
3. Website-to-Stock Mapping
Each Magento website is tied to one stock. The available inventory shown on a website is derived from the total quantities of products across the sources assigned to that stock.
Use Cases for Assigned Sources
Use Case | Benefit |
---|---|
Regional Fulfillment | Show stock from nearby sources for faster delivery |
BOPIS (Buy Online Pickup In Store) | Allow customers to pick up from physical store sources |
Third-party Logistics Integration | Assign drop shippers as sources for certain product SKUs |
Warehouse Segmentation | Split product inventory between locations based on region or demand |
Locating Assigned Sources in Magento 2 Admin
Navigation Path in Admin Panel
To view and manage assigned sources for a stock:
- Go to Stores in the Magento Admin sidebar
- Select Inventory
- Click on Stocks
- Choose a stock to edit (e.g., "Default Stock" or a custom stock for a website)
- Navigate to the Sources tab within the stock configuration
Here, you’ll find a complete list of sources that are linked to the selected stock.
What You’ll See in the Sources Tab
The Sources Tab presents a tabular list that includes the following details for each assigned source:
Column | Description |
---|---|
Source Code | Unique identifier for the source (e.g., main_warehouse , store_001 ) |
Source Name | Human-readable name for the source (e.g., "Main Warehouse") |
Priority | The execution order for source selection during shipment processing |
Status | Indicates whether the source is enabled or disabled |
Action Options | Allows you to unassign or change priority of the source |
Additional Context
- Assigned sources define the physical or virtual origins of inventory for a given stock.
- These sources are used in the Source Selection Algorithm (SSA) during checkout to determine from which source to fulfill an order.
- If a product is available in multiple assigned sources, priority and availability help decide fulfillment location.
Use Case Scenarios
Use Case | Purpose |
---|---|
Multi-Warehouse Setup | Manage inventory from geographically distributed warehouses |
Regional Website Fulfillment | Assign sources to specific websites based on region |
Drop-Shipping Integration | Designate third-party vendors as inventory sources |
Retail Pickup Management | Allow in-store pickup options via BOPIS |
Stock Segmentation | Organize inventory between stores, warehouses, and suppliers |
Programmatic Retrieval of Assigned Sources in Magento 2 MSI
Magento 2.4.7 (and newer) allows developers to programmatically fetch the sources linked to a particular stock. This is especially useful for multi-source inventory (MSI) implementations in setups involving warehouses, retail stores, or third-party fulfillment systems.
Magento provides an interface called Magento\InventoryApi\Api\GetStockSourceLinksInterface
, which, when used alongside SearchCriteriaBuilder
, helps retrieve the list of assigned sources for any given stock.
Why You Might Need This
Use Case | Benefit |
---|---|
Automated Inventory Management | Programmatically manage and monitor source-to-stock assignments. |
Custom Reports | Fetch source data for backend analytics, filtering by region or warehouse. |
Integration with ERP or WMS | Sync source relationships between Magento and external warehouse systems. |
Dynamic Fulfillment Logic | Adjust source usage programmatically based on business logic or stock levels. |
Interface Used
- Interface Name:
Magento\InventoryApi\Api\GetStockSourceLinksInterface
- Purpose: Retrieves source links (assigned sources) for a specific stock.
- Dependencies: Requires
SearchCriteriaBuilder
and optional logging.
Steps to Retrieve Assigned Sources
Step | Action | Description |
---|---|---|
1 | Inject SearchCriteriaBuilder and GetStockSourceLinksInterface |
These are used to construct the query and fetch the data |
2 | Build search criteria using stock_id |
Use this to filter the results by a specific stock |
3 | Call execute() on the interface |
Retrieves the assigned source links |
4 | Iterate over the returned items | Each item represents a StockSourceLinkInterface instance |
5 | Extract source_code , priority , etc. |
Use these values in your logic or output |
Example Code
<?php
namespace Vendor\Module\Model;
use Exception;
use Psr\Log\LoggerInterface;
use Magento\Framework\Api\SearchCriteriaBuilder;
use Magento\InventoryApi\Api\GetStockSourceLinksInterface;
use Magento\InventoryApi\Api\Data\StockSourceLinkInterface;
class AssignedSourceList
{
private $searchCriteriaBuilder;
private $getStockSourceLinks;
private $logger;
public function __construct(
SearchCriteriaBuilder $searchCriteriaBuilder,
GetStockSourceLinksInterface $getStockSourceLinks,
LoggerInterface $logger
) {
$this->searchCriteriaBuilder = $searchCriteriaBuilder;
$this->getStockSourceLinks = $getStockSourceLinks;
$this->logger = $logger;
}
/**
* Get assigned sources for a stock ID
*
* @param int $stockId
* @return StockSourceLinkInterface[]
*/
public function getAssignedSource(int $stockId): array
{
try {
$searchCriteria = $this->searchCriteriaBuilder
->addFilter(StockSourceLinkInterface::STOCK_ID, $stockId)
->create();
$result = [];
$items = $this->getStockSourceLinks->execute($searchCriteria)->getItems();
foreach ($items as $link) {
$result[$link->getSourceCode()] = [
'link_id' => $link->getLinkId(),
'stock_id' => $link->getStockId(),
'source_code' => $link->getSourceCode(),
'priority' => $link->getPriority()
];
}
return $result;
} catch (Exception $e) {
$this->logger->error(__('Failed to retrieve assigned sources: %1', $e->getMessage()));
return [];
}
}
}
Expected Output Format
When the getAssignedSource()
method is called with a valid stock ID (e.g., 1), the returned result will be in the format:
[
'default' => [
'link_id' => 1,
'stock_id' => 1,
'source_code' => 'default',
'priority' => 1
],
'store_001' => [
'link_id' => 2,
'stock_id' => 1,
'source_code' => 'store_001',
'priority' => 2
]
]
Notes & Recommendations
- This method only works if MSI is enabled and the sources are actively assigned.
- Always validate the returned data, especially in multi-stock or multi-website setups.
- Avoid hardcoding source codes. Instead, use config settings or admin-driven values.
- You can enhance this further by also loading the Source entities using
\Magento\InventoryApi\Api\SourceRepositoryInterface
to get source names and details.
Understanding Magento MSI: Stock vs Source
Magento 2's Multi-Source Inventory (MSI) system introduces a powerful layer of inventory management through the concepts of Stocks and Sources. These two elements work together to handle distributed inventory, regional fulfillment, BOPIS (Buy Online Pickup In Store), and more.
Below is a comprehensive comparison that outlines the differences and roles of Stocks and Sources in Magento 2.
Key Differences Between Stock and Source
Term | Description |
---|---|
Stock | A virtual group of one or more inventory sources. A stock is directly associated with a Sales Channel (such as a website or store view) and defines how Magento aggregates available inventory across those sources. |
Source | A physical or virtual location that holds inventory. This can be a warehouse, brick-and-mortar store, drop-ship vendor, or 3PL (third-party logistics) provider. |
Stock ID | A unique identifier for a stock. Used programmatically and in the admin panel to reference a particular group of sources. |
Source Code | A unique identifier for a source. This is the reference used internally for assigning, tracking, and updating inventory. |
Priority | Determines which source should be used first during order fulfillment when multiple sources have inventory available for the same product. |
Magento MSI separates the business logic (Stock) from the physical inventory locations (Source), allowing for scalable, region-specific, and multi-warehouse strategies. Understanding the distinction and proper usage of each is essential for implementing dynamic fulfillment models and building a flexible inventory architecture.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Conclusion
In Magento 2.4.7, managing inventory efficiently is crucial, especially for stores operating with multiple warehouses, suppliers, or distribution points. The ability to programmatically retrieve assigned sources for a specific stock using the GetStockSourceLinksInterface
is a powerful feature of Magento's Multi-Source Inventory (MSI) system. It allows developers to integrate deeper logic into their inventory workflows—enabling advanced customization for inventory forecasting, warehouse management, and order routing.
By understanding how to programmatically access these stock-source relationships, developers can:
- Automate inventory syncs with external systems
- Build custom dashboards showing stock allocations
- Generate real-time stock availability reports
- Enhance the performance of order assignment algorithms
This feature also improves transparency and control over inventory, ensuring that the right stock is available at the right place, which ultimately leads to better customer satisfaction.
As Magento continues to evolve, mastering MSI operations through clean, extensible code not only future-proofs your store but also sets a solid foundation for scaling. Whether you're building a headless PWA, syncing ERP systems, or managing cross-border inventory, understanding the MSI framework—especially stock-source mapping—is a key step toward powerful, reliable eCommerce architecture.
FAQs
What is Multi-Source Inventory (MSI) in Magento 2?
MSI allows store owners to manage inventory across multiple physical sources like warehouses or suppliers within Magento 2.
How do I fetch assigned sources for a specific stock in Magento 2?
You can retrieve assigned sources using the GetStockSourceLinksInterface by filtering with the desired stock ID programmatically.
Where can I find assigned sources in the Magento 2 admin panel?
Navigate to Stores → Inventory → Stocks, then click Edit on a stock and check the Sources tab to see assigned sources.
Which class is used to retrieve assigned source links in Magento 2 MSI?
The class used is Magento\InventoryApi\Api\GetStockSourceLinksInterface.
What is the purpose of SearchCriteriaBuilder in the code?
SearchCriteriaBuilder is used to add filters and build search queries to fetch data from repositories in Magento 2.
What does the getAssignedSource() function return?
It returns an array of StockSourceLinkInterface objects representing all sources linked to a given stock ID.
Can a stock be linked to multiple sources in Magento 2?
Yes, each stock can have multiple sources assigned to it, allowing flexible inventory distribution.
Is it possible to fetch source priority from the assigned source data?
Yes, you can retrieve the priority of each source using the getData() method on the StockSourceLinkInterface object.
What is the source_code field in the result?
It represents the unique code of the source (e.g., "default") that is linked to the stock.
How do I display the result of getAssignedSource() function?
You can loop through the returned array and use var_dump() or print_r() to view each source's data.
Can I use this method to update source links?
No, GetStockSourceLinksInterface is used only for retrieving links. Updating requires different APIs like SourceRepositoryInterface.
What happens if a stock has no assigned sources?
The result will be an empty array, and no source-related inventory will be available for that stock.
Why is managing source assignments important in Magento MSI?
Proper source assignment ensures efficient order fulfillment, warehouse tracking, and accurate stock status across channels.