Implementing Cookies in Magento 2: A Practical Guide
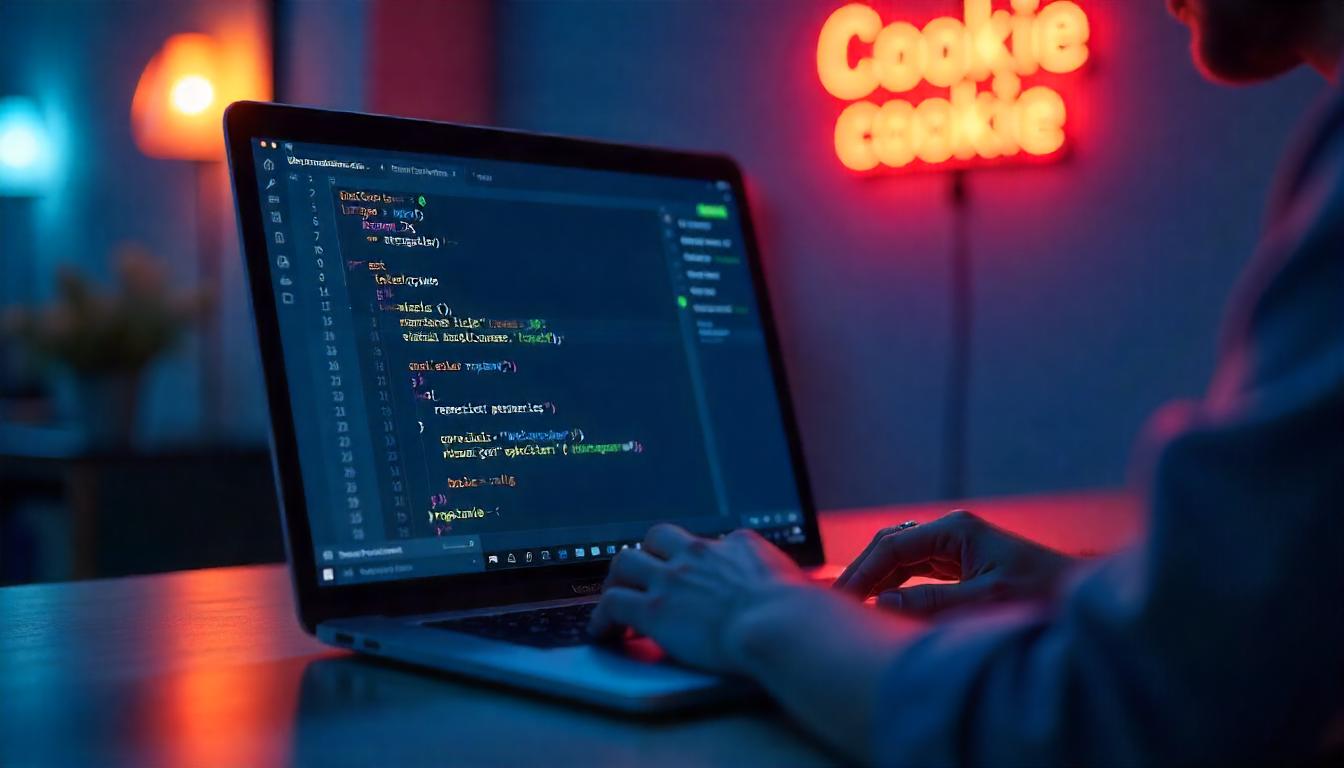
Implementing Cookies in Magento 2: A Practical Guide
Learn how to implement cookies in Magento 2 using the recommended CookieManagerInterface and CookieMetadataFactory approach. This guide covers setting and retrieving cookies, explains key metadata options like duration, path, and HttpOnly, and highlights best practices for secure cookie handling—without relying on discouraged methods like $_COOKIE.
Table Of Content
Implementing Cookies in Magento 2: A Practical Guide
In Magento 2, managing cookies is crucial for session handling, user preferences, and compliance with privacy regulations. Direct use of $_COOKIE is discouraged due to Magento's coding standards. Instead, utilize the CookieManagerInterface for setting and retrieving cookies.
Setting a Cookie in Magento 2
To set a cookie, create a model that injects CookieManagerInterface
and CookieMetadataFactory
:
<?php
declare(strict_types=1);
namespace Vendor\Module\Model;
use Magento\Framework\Stdlib\Cookie\CookieMetadataFactory;
use Magento\Framework\Stdlib\CookieManagerInterface;
class CookieManager
{
public function __construct(
private readonly CookieManagerInterface $cookieManager,
private readonly CookieMetadataFactory $cookieMetadataFactory
) {
}
public function setCookie(): void
{
$metadata = $this->cookieMetadataFactory->createPublicCookieMetadata()
->setDurationOneYear()
->setPath('/')
->setHttpOnly(false);
$this->cookieManager->setPublicCookie('cookie_name', 'cookie_value', $metadata);
}
}
This approach ensures compliance with Magento's standards and provides flexibility in cookie management.
Retrieving a Cookie
To retrieve the cookie value:
public function getCookie(): ?string
{
return $this->cookieManager->getCookie('cookie_name');
}
This method returns the cookie value or null if the cookie doesn't exist.
Cookie Metadata Explained
When setting cookies, it's essential to understand the metadata:
- Duration: Specifies how long the cookie remains valid.
- Path: Defines the URL path for which the cookie is valid.
- HttpOnly: If set to true, the cookie is inaccessible to JavaScript, enhancing security.
By default, HttpOnly is set to false, allowing JavaScript access. However, for security reasons, it's advisable to set it to true when possible.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What’s the Recommended Way to Set a Cookie in Magento 2?
Use Magento\Framework\Stdlib\CookieManagerInterface
along with CookieMetadataFactory
to safely set cookies. Avoid using $_COOKIE
directly as it's against Magento coding standards.
Why Should I Avoid Using $_COOKIE
Directly?
Direct access to $_COOKIE
can break Magento’s coding guidelines and create security risks. Always use Magento's cookie service classes for compatibility and safe handling.
How Do I Create a Public Cookie in Magento 2?
Create metadata using createPublicCookieMetadata()
. Then use setPublicCookie()
to add the cookie. This ensures it's accessible via JavaScript if HttpOnly
is false.
Can I Customize Cookie Lifetime and Path?
Yes, use methods like setDurationOneYear()
and setPath()
on metadata to control how long and where the cookie is valid.
What Is HttpOnly
and Should I Enable It?
HttpOnly
prevents JavaScript from accessing the cookie. Set it to true
if you want added security. It's false
by default for public cookies.
How Do I Retrieve a Cookie in Magento 2?
Use getCookie('cookie_name')
method from CookieManagerInterface
. It returns the cookie value or null
if not found.
Where Do I Configure Cookie Settings in Magento 2?
Go to Stores > Configuration > General > Web > Default Cookie Settings in the admin panel. You can manage lifetime, path, domain, and HttpOnly there.
Is It Safe to Let JavaScript Access Cookies?
If you don’t set HttpOnly
, the cookie is accessible via JavaScript. Only do this if needed and ensure the data isn’t sensitive.
Can I Set Extra Data in Cookies?
You can associate extra metadata like duration, path, and security settings using CookieMetadataFactory
. It helps maintain consistency and control.
Where Can I Find More About Magento 2 Cookies?
Check Magento's official developer documentation and trusted blogs. They cover cookie handling, examples, and secure practices in depth.