Get Sales Channels Data for a Stock in Magento 2.4.7 MSI
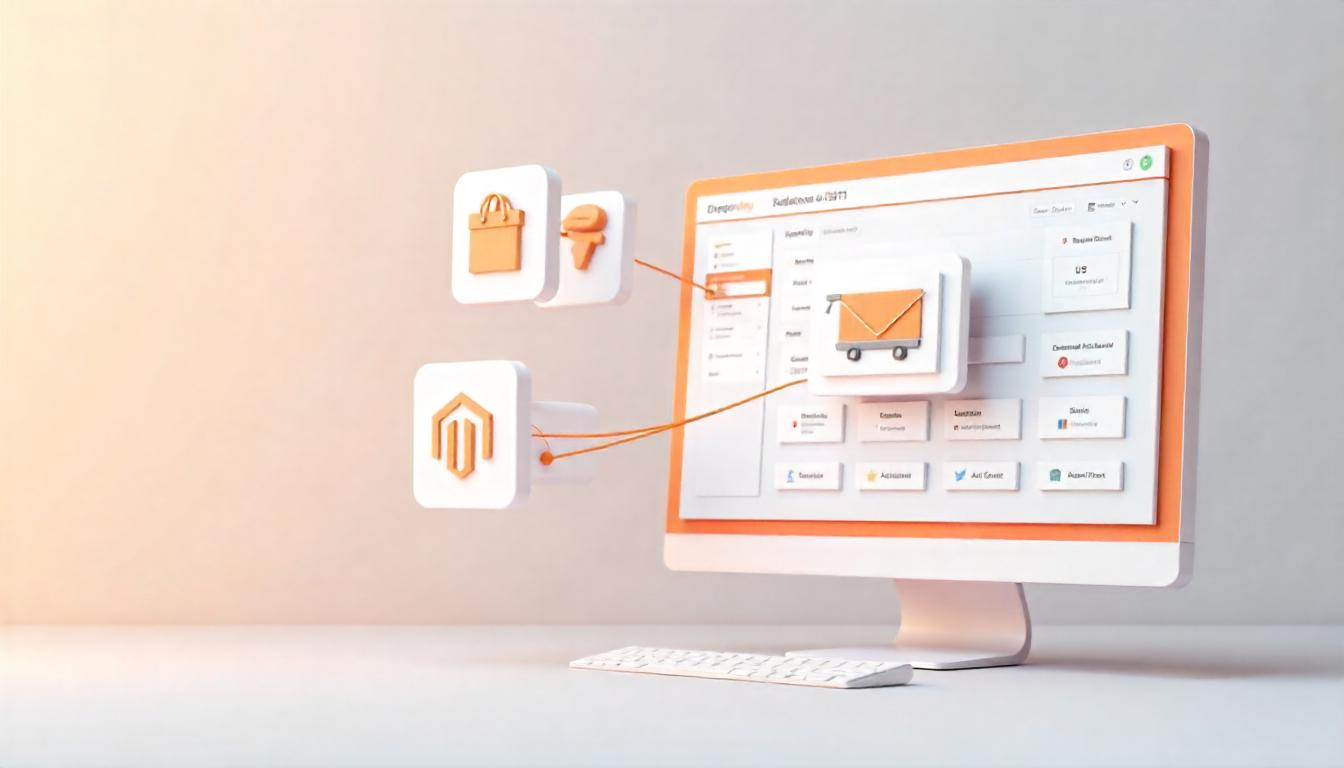
Get Sales Channels Data for a Stock in Magento 2.4.7 MSI
Magento 2's Multi-Source Inventory (MSI) supports assigning multiple websites (sales channels) to inventory stocks. In Magento 2.4.7, this functionality continues to power flexible inventory management across multiple storefronts.
Table Of Content
Understanding Sales Channels in Magento MSI
In Magento's Multi-Source Inventory (MSI) system, Sales Channels represent individual websites or storefronts that are linked to specific inventory Stocks. Each stock can serve one or more websites, enabling businesses to manage inventory visibility and availability per website.
This connection is crucial in scenarios where a business operates multiple storefronts with different inventory requirements. It ensures accurate inventory sourcing, fulfillment, and product availability for each sales channel.
Key Concepts and Definitions
Term | Description |
---|---|
Sales Channel | A website or storefront in Magento that is connected to a stock. It defines where products from that stock can be purchased. |
Stock | A virtual group of sources, linked to one or more sales channels. Each stock handles inventory aggregation from multiple sources. |
Default Stock | The system-created stock that is used by default when no custom stock is created. Often used in single-website setups. |
Source | A physical or virtual inventory location (e.g., warehouse, store, drop-shipper). |
Website Code | The internal identifier used to associate a stock with a Magento website. |
When Are Sales Channels Important?
- Multi-Website Stores: Assign different inventory rules or sources per region, language, or currency.
- Store-specific Inventory Rules: Limit inventory visibility to specific websites.
- Localized Fulfillment Models: Use different fulfillment centers for different regions.
- B2B and B2C Separation: Offer different inventory per business type or customer group.
Programmatic Use of Sales Channels
To retrieve and display sales channels for a stock in Magento 2.4.7, you can use the Magento\InventorySalesApi\Api\StockResolverInterface
and Magento\InventoryApi\Api\StockRepositoryInterface
.
Goals:
- Fetch stock data programmatically
- Access assigned sales channels via extension attributes
- Map website codes to human-readable names
- Use this data in custom modules or reports
Example Code Overview
You can use the following components in your module to fetch the list of sales channels assigned to a stock:
Magento\InventoryApi\Api\StockRepositoryInterface:
Load stock data.Magento\InventorySalesApi\Api\Data\SalesChannelInterface:
Access extension attributes.Magento\Framework\Api\SearchCriteriaBuilder:
Build filters if needed.
Real-world Applications
Use Case | Benefit |
---|---|
Regional Store Inventory | Assign inventory per region or website to ensure localized stock display. |
Multi-brand or Multi-currency Sites | Separate inventory rules for different brand sites or currencies. |
B2B vs B2C Channel Customization | Allow different fulfillment logic depending on customer group. |
Testing or Staging Websites | Link isolated stocks to development or staging environments for safe testing. |
Admin Panel Path
To view assigned sales channels via the Magento Admin panel:
- Go to
Stores > Inventory > Stocks
- Select a stock
- Under the
Sales Channels
tab, you’ll see all websites linked to the selected stock
This UI provides a visual representation of the connection between inventory and storefronts, making it easier for merchants to manage fulfillment and visibility.
Understanding Magento’s Sales Channel Mapping Mechanism
Magento 2 with MSI (Multi-Source Inventory) introduces a flexible and robust system for connecting inventory stocks with specific sales channels (websites or store views). This system governs how products are displayed, sold, and fulfilled across various Magento storefronts.
What Are Sales Channels in Magento?
A Sales Channel in Magento refers to a website or storefront that is connected to a specific Stock. This connection controls which set of inventory sources the website can access for order processing and fulfillment.
How Sales Channel Assignment Works
When a website is assigned to a stock:
- Inventory Availability – All source-level quantities linked to that stock become available for that website.
- Catalog Visibility – The product catalog for that website reflects only the inventory associated with the connected stock.
- Checkout Logic – Magento uses the sources and their priorities under the assigned stock to determine inventory at checkout.
- Shipping Decisions – Fulfillment logic leverages the closest or prioritized source for shipping, based on assigned stock data.
- Backorder Rules – These follow the configurations set at source level within the linked stock.
Use Cases of Mapping Sales Channels to Stocks
Use Case | Purpose |
---|---|
Region-based Storefronts | Assign region-specific sources to show accurate inventory per location. |
Separate Brands Under One Store | Link different brands to separate stocks for isolated inventory control. |
Multilingual / Multicurrency | Manage independent inventories for each locale-based website. |
B2B vs B2C Segmentation | Customize stock availability per customer group or website type. |
Marketplace or Multi-Vendor | Allocate dedicated sources for each vendor with custom stock setups. |
Benefits of Correct Channel Mapping
- Improves fulfillment efficiency by linking stock close to customers
- Minimizes stockouts by isolating inventories by region or business model
- Simplifies inventory reporting and forecasting across distinct sales streams
- Provides flexibility for testing/staging without impacting live inventory
Important Notes for Admins
- Admin Path: To manage this in the admin panel, go to:
Admin Path: To manage this in the admin panel, go to:
- Default Stock Behavior: For single-website setups, Magento uses a default stock that includes all sources unless changed.
- Programmatic Access: You can retrieve this mapping using the StockRepositoryInterface and its extension attributes.
Fetching Sales Channels Programmatically in Magento 2.4.7
Magento 2.4.7 uses extension attributes to enrich standard data entities like stock. These attributes allow developers to retrieve connected data (e.g., websites or sales channels) in a structured and flexible way.
In this guide, we will cover how to programmatically fetch the sales channels (websites) associated with a specific stock. This is essential when working with Magento's Multi-Source Inventory (MSI) system, especially in multi-website, multi-region, or segmented inventory setups.
Why Fetch Sales Channels?
Magento allows you to link different websites (storefronts) to a specific stock. This linkage determines:
- Which sources’ inventory appears on which website
- Which website can sell what product
- Fulfillment and backorder behavior during checkout
Fetching these programmatically is useful for integrations, audits, custom reports, and advanced business logic.
Service Class: SalesChannels
Below is an updated and production-ready code sample that demonstrates how to retrieve the sales channels for a given stock ID using Magento’s service contracts.
<?php
namespace Vendor\Module\Model;
use Exception;
use Psr\Log\LoggerInterface;
use Magento\InventoryApi\Api\StockRepositoryInterface;
class SalesChannels
{
protected StockRepositoryInterface $stockRepository;
protected LoggerInterface $logger;
public function __construct(
StockRepositoryInterface $stockRepository,
LoggerInterface $logger
) {
$this->stockRepository = $stockRepository;
$this->logger = $logger;
}
/**
* Fetch sales channels by stock ID
*
* @param int $stockId
* @return array|null
*/
public function getSalesChannelsByStockId(int $stockId = 1): ?array
{
$salesChannelsData = [];
try {
$stock = $this->stockRepository->get($stockId);
$extensionAttributes = $stock->getExtensionAttributes();
if ($extensionAttributes && $extensionAttributes->getSalesChannels()) {
foreach ($extensionAttributes->getSalesChannels() as $channel) {
$salesChannelsData[$channel->getType()][] = $channel->getCode();
}
}
} catch (Exception $e) {
$this->logger->error(__('Error fetching sales channels: %1', .$e->getMessage());
}
return $salesChannelsData;
}
}
Output Example
This code will return an associative array that groups sales channels by their type (e.g., "website"):
Array
((
[website] => Array
((
[0] => base
[1] => canada_website
)
)
Each channel is a Magento website code associated with the given stock ID.
Real-World Scenarios and Their Benefits
Scenario | Benefit |
---|---|
Multinational eCommerce | Assign region-specific websites to regional stocks for better accuracy |
B2B vs B2C Portals | Isolate wholesale and retail inventory to avoid cross-visibility |
Language-specific Storefronts | Bind language-based websites with local inventories for fast shipping |
Marketplace Multi-Vendor Setup | Enable separate inventories per vendor under dedicated website codes |
Inventory Audit Automation | Automatically report sales channel linkage for inventory compliance |
Key Concepts
Sales Channel
A website or storefront in Magento that connects to a stock. It controls what inventory is shown and sold on that storefront.
Stock
A logical grouping of inventory sources. A stock defines product availability by aggregating quantity across connected sources.
Extension Attributes
Magento's way of attaching additional, non-core data (such as sales channels) to data entities like Stock or Product.
Best Practices
- Always wrap your service calls in a try-catch to avoid breaking frontend or admin functionality.
- Use dependency injection
(DI)
to inject services likeStockRepositoryInterface
. - Do not hardcode website codes—use Magento's APIs to fetch and validate channel relationships dynamically.
Advanced Use Cases of Sales Channel Assignment in Magento 2.4.7
Magento 2 Multi-Source Inventory (MSI) enables powerful inventory segregation based on business models and market segmentation. Below is a comprehensive table showcasing key real-world scenarios, along with the benefits of assigning separate sales channels to inventory stocks.
Use Case Scenarios and Business Benefits
Scenario | Benefit |
---|---|
Multinational eCommerce | Assign websites representing different countries to separate regional stocks. This ensures accurate product availability, shipping timelines, and tax rules based on each location. |
B2B vs B2C Portals | Maintain separate inventory pools for wholesale and retail channels. Prevents accidental cross-ordering and allows fulfillment rules that differ based on customer groups. |
Language-Specific Storefronts | Link localized websites (e.g., English, Spanish, French) to unique inventory stocks. This speeds up delivery and allows better regional stock tracking. |
Marketplace / Multi-Vendor Setup | Assign vendors or sellers their own stock and sources. This simplifies order routing, vendor-specific stock audits, and performance tracking. |
Inventory Compliance & Auditing | Automate reports that match stock data to websites. Useful during compliance checks or for syncing data with ERP systems. |
Understanding Magento MSI Entity Relationships
Magento MSI is powered by a set of interconnected entities that store and manage inventory data. Here's how they relate:
Inventory Entity Reference Table
Entity | Class / Interface | Description |
---|---|---|
Stock | Magento\InventoryApi\Api\Data\StockInterface | Represents a logical group of inventory sources connected to one or more websites (sales channels). |
Sales Channel | Magento\InventorySalesApi\Api\Data\SalesChannelInterface | Refers to Magento websites or store views that are assigned to stocks for inventory access. |
Source | Magento\InventoryApi\Api\Data\SourceInterface | Defines physical or virtual inventory locations like warehouses, retail stores, or 3PL services. |
Developer Guidelines for Clean MSI Code Integration
To ensure stable and scalable Magento code when working with inventory and sales channels, follow these Magento best practices:
Key Tips for Developers
Tip | Insight |
---|---|
Use Interfaces Over Concrete Classes | Promotes better unit testing and flexible code architecture. |
Always Check for Nulls in Extension Attributes | Prevents errors when data isn't fully populated—especially during partial saves or import operations. |
Use PSR-3 Logger | Enables standardized error logging. Use $logger->error() to capture critical failures without breaking flow. |
Rely on Dependency Injection | Avoid using ObjectManager directly. Define dependencies in constructors for better control and testing. |
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Conclusion
Understanding and managing Sales Channels in Magento 2.4.7’s Multi-Source Inventory (MSI) is critical for running a scalable, multi-store eCommerce operation. Whether you're handling a single store or a complex multi-website architecture, knowing how to retrieve and configure stock-to-website relationships ensures accurate inventory visibility and smooth customer experience across regions, languages, and business models.
By using the latest StockRepositoryInterface and leveraging extension attributes, developers can efficiently fetch sales channel data programmatically, while store admins can easily configure assignments from the backend. With practical examples, updated code, admin navigation, real-world scenarios, and developer tips, you're now equipped to fully control and audit how stock flows across your sales websites.
In short, sales channels act as the bridge between your inventory and the frontend of your store. Mastering them means mastering Magento MSI.
Stay updated, test frequently, and apply best practices to keep your inventory ecosystem robust and flexible.
FAQs
What are sales channels in Magento 2?
Sales channels in Magento 2 are websites assigned to a stock, allowing inventory to be available on those specific sites.
Where can I manage sales channels in the admin panel?
Go to Stores ➝ Inventory ➝ Stocks, edit a stock, and navigate to the "Sales Channels" tab to manage assignments.
Can one stock be linked to multiple websites?
Yes, a single stock can be assigned to multiple websites, making its inventory visible on all of them.
Can one website be linked to multiple stocks?
No, Magento only allows a website to be assigned to one stock at a time.
What is the default stock and sales channel in Magento 2?
The default stock is created by Magento out of the box and is typically assigned to the ‘base’ website by default.
How do I programmatically fetch sales channels for a stock?
Use the StockRepositoryInterface and call getExtensionAttributes()->getSalesChannels() on the stock object.
Which interface provides access to stock data?
Use Magento\InventoryApi\Api\StockRepositoryInterface to retrieve stock data.
What happens if I don't assign a website to a stock?
The website will not show products tied to that stock, and checkout will fail for those items.
Can I fetch sales channels via GraphQL in Magento 2?
Not by default. You’ll need to create a custom GraphQL resolver to expose sales channels.
Is it safe to use ObjectManager for stock retrieval?
No, always use dependency injection to access services like StockRepositoryInterface for clean and testable code.
How do I assign a website to a stock programmatically?
You need to update the stock entity's extension attributes using Magento\InventoryApi\Api\StockRepositoryInterface and save it.
Can I assign different product quantities to different websites?
Yes, by assigning different sources to stocks and linking those stocks to different websites (sales channels).
Are sales channels limited to websites only?
Currently, Magento defines sales channels as websites, but future expansions may allow additional channel types.
How do I check current sales channel mappings for all stocks?
You can loop through all stock items using StockRepositoryInterface and fetch extension attributes for each.
Is this functionality compatible with Magento Open Source and Adobe Commerce?
Yes, sales channels and MSI are available in both Magento Open Source and Adobe Commerce editions.