How to Disable Payment method for frontend only in Magento 2.4.x and Why?.
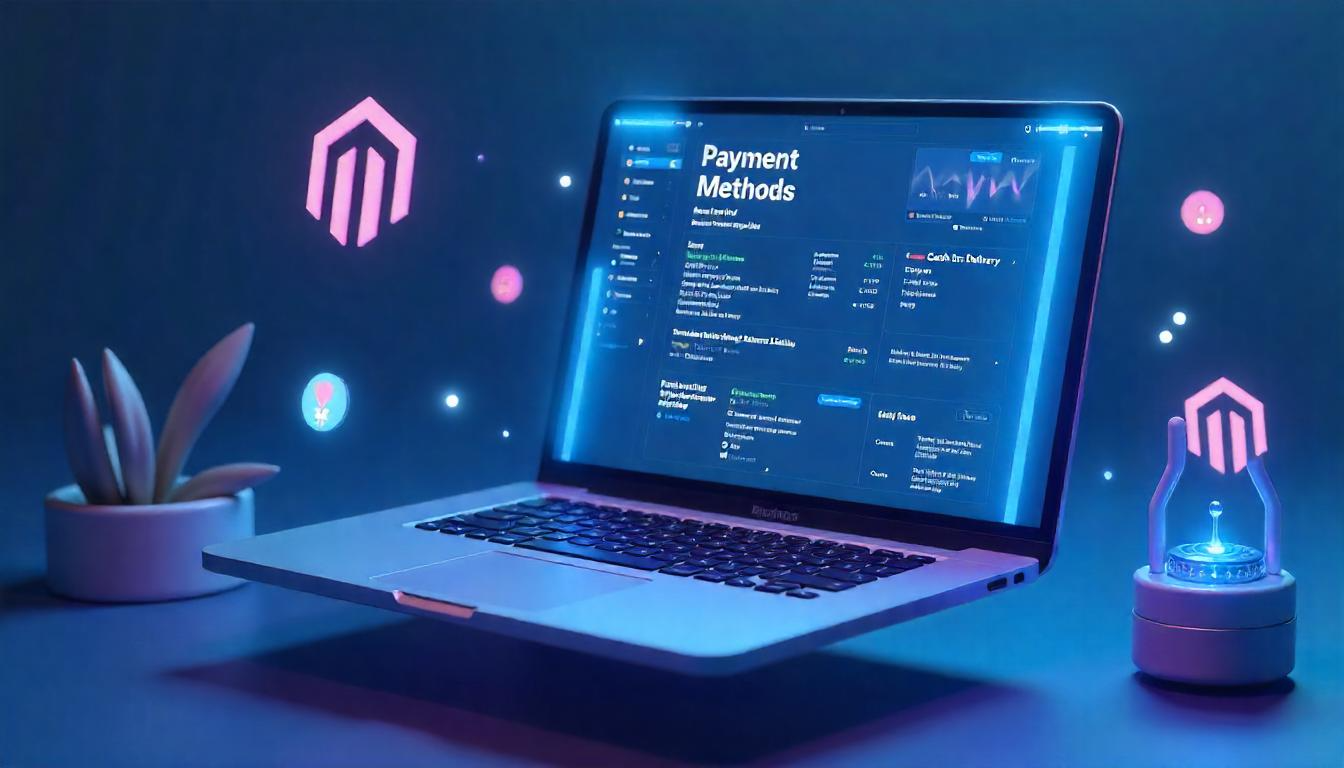
How to Disable Payment method for frontend only in Magento 2.4.x and Why?.
Magento 2.4.7 allows you to selectively disable payment methods on the storefront while keeping them active in the admin. By creating a lightweight module and using a plugin on the MethodList::getAvailableMethods() function, you can filter out any payment method—such as Cash on Delivery—before it’s presented to customers during checkout. This approach requires four files: registration.php to register the module, module.xml to declare dependencies, di.xml to configure the plugin, and the plugin class itself to remove the specified method code. The result is a clean, upgrade‑safe solution that preserves backend functionality and can be extended to any condition or payment method type.
Table Of Content
Customizing Payment Method Visibility in Magento 2.4.7
Magento 2.4.7 provides a flexible payment framework that allows developers to control the visibility of payment methods programmatically. This is especially useful when you want to restrict certain methods from appearing on the frontend checkout but still keep them available for admin order management.
Overview
Magento’s core payment method retrieval is handled by the \Magento\Payment\Model\MethodList::getAvailableMethods()
method. It compiles all active and available payment methods for a specific quote context.
Plugin-Based Approach
Using an after-plugin on getAvailableMethods()
, you can safely filter out specific payment methods based on custom business logic (store view, customer group, quote contents, cart value, etc.).
This approach maintains separation of concerns, doesn't affect admin functionality, and is upgrade-friendly.
Common Use Cases
Use Case | Description |
---|---|
Hide methods for guest users | Only show certain payment methods for logged-in customers. |
Filter methods by customer group | Limit access to specific payment providers based on B2B or VIP tiers. |
Remove COD for virtual products | Prevent Cash on Delivery if the cart has only downloadable items. |
Exclude payment for certain regions | Block a payment method if the customer's billing/shipping region is not supported. |
Display method only on specific store views | Useful for multi-language or multi-brand sites using different payment vendors. |
Implementation Outline
Step 1: Define the Plugin
Create a plugin on the \Magento\Payment\Model\MethodList::getAvailableMethods()
class.
namespace Vendor\Module\Plugin;
use Magento\Payment\Model\MethodInterface;
use Magento\Quote\Api\Data\CartInterface;
class FilterPaymentMethods
{
public function afterGetAvailableMethods(
\Magento\Payment\Model\MethodList $subject,
array $methods,
CartInterface $quote = null
): array {
$filteredMethods = [];
foreach ($methods as $method) {
// Add your conditions to hide/show payment methods
if ($method->getCode() !== 'cashondelivery') {
$filteredMethods[] = $method;
}
}
return $filteredMethods;
}
}
Step 2: Register the Plugin in di.xml
<type name="Magento\Payment\Model\MethodList">
<plugin name="filter_payment_methods" type="Vendor\Module\Plugin\FilterPaymentMethods" />
</type>
Key Considerations
- Admin Order Unaffected: This plugin only filters the methods on the storefront. Admin order creation calls a separate method (getAvailableMethods() without quote context), which you don’t intercept here.
- Safe Filtering: Always validate the quote object before applying any filtering logic to avoid issues on pages where it’s not present.
- Scope Aware: Incorporate store, customer, and quote data when building your filtering logic to make it dynamic and relevant.
- Performance: Avoid heavy service calls or database queries within the plugin to keep checkout performance smooth.
Best Practices
Best Practice | Recommendation |
---|---|
Use after plugins for filtering | Ensures original method execution and lets you work with the full result set. |
Avoid filtering in adminhtml | Ensure that admin functionality remains intact and unaffected. |
Add config options | Allow control via admin configuration for easier management. |
Log filtered methods during testing | Helps troubleshoot and verify conditions during development. |
Respect dependencies | If other modules rely on specific payment methods, check compatibility before removal. |
By leveraging plugins in Magento 2.4.7, you can control payment method visibility on the frontend dynamically, without disrupting the admin workflow. This allows for a more tailored checkout experience that aligns with business logic, improves user experience, and ensures operational flexibility.
Strategic Frontend Payment Method Control in Magento 2.4.7
In a multi-market or feature-rich Magento 2.4.7 store, it's often necessary to restrict payment methods on the storefront while keeping them available in the admin area for backend operations. This selective visibility ensures a seamless customer experience and operational flexibility for store managers.
By leveraging Magento’s plugin architecture, especially through after
plugins on the payment method list, developers can dynamically control which payment options are presented to users without permanently disabling them system-wide.
Why Restrict Payment Methods Only on the Frontend?
Controlling frontend-only availability of payment methods ensures smoother transactions, improved user targeting, and compliance with business logic, all while preserving full payment method availability for backend users.
Key Benefits of Frontend‑Only Payment Method Filtering
Benefit | Description |
---|---|
Streamlined Checkout | Remove unsuitable or high-fee payment options for specific user segments or regional markets. |
Backend Operational Flexibility | Keep all methods active for staff use in admin panel for manual orders, refunds, or quotes. |
Targeted Display by Conditions | Apply filters based on customer groups, cart content, store views, or shipping preferences. |
Enhanced User Experience | Show only relevant and fast payment options to reduce confusion and boost conversion rates. |
Regional or Legal Compliance | Prevent offering unsupported payment methods in restricted countries or states. |
Additional Use Cases
Scenario | Application Example |
---|---|
B2B Customer Access | Enable offline payment or purchase order methods only for approved customer groups. |
Cart-Based Logic | Block Cash on Delivery when only virtual or subscription items are in the cart. |
Multi-Store Strategy | Use localized payment providers per store view or language setup. |
High-Risk Transaction Filtering | Disable high-fraud-risk methods for new or unverified accounts. |
Flash Sale Events | Temporarily limit available methods to speed up checkout during high traffic. |
Best Practice Highlights
- Use After Plugins: Preserve original logic while modifying the output list of methods.
- Avoid Filtering in Admin Area: Ensure admin users can access full functionality when processing orders manually.
- Add Configuration Controls: Allow easy toggling of frontend filtering from system configuration.
- Enable Logging for Testing: Track filtered methods and related conditions during development or debugging.
- Respect Payment Method Dependencies: Verify that filtering doesn't interfere with extensions or modules that depend on specific payment methods.
Module Setup and Declaration for Disabling Frontend Payment Methods in Magento 2.4.7
Prerequisites for Module Creation
Before you dive into creating your custom module to disable frontend payment methods, ensure that you meet the following prerequisites:
- Magento 2.4.7 Installation: You must have a working installation of Magento 2.4.7. If you haven’t already, follow the official Magento documentation to install or update Magento to this version.
- Command-Line Interface (CLI) Access: You’ll need access to Magento’s CLI to run necessary commands like setup:upgrade, cache:flush, and others during the module creation and deployment process.
- Basic Knowledge of Dependency Injection (DI) and Plugins: A basic understanding of Magento’s DI system and plugin architecture will be helpful as these are the core concepts we’ll use to intercept and modify Magento's payment methods.
- Magento E-Commerce Solutions Knowledge: An understanding of how Magento 2 handles payments and how methods are displayed on both the frontend and backend will ensure a smooth implementation.
Step 1: Module Declaration
In this first step, you’ll need to declare your custom module in Magento. This process involves creating the registration.php
and module.xml
files.
1.1 registration.php
Create the registration.php
file in the directory app/code/Vendor/DisableFrontPayment/
. This file will register your custom module with Magento:
<?php
\Magento\Framework\Component\ComponentRegistrar::register(
\Magento\Framework\Component\ComponentRegistrar::MODULE,
'Vendor_DisableFrontPayment',
__DIR__
);
<?php
The registration.php
file ensures that Magento recognizes your module as part of its application.
1.2 module.xml
Next, create the module.xml
file in app/code/Vendor/DisableFrontPayment/etc/
. This configuration file declares the module and ensures that it loads after Magento’s payment functionality.
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:noNamespaceSchemaLocation="urn:magento:framework:Module/etc/module.xsd">
<module name="Vendor_DisableFrontPayment">
<sequence>
<module name="Magento_Payment"/>
</sequence>
</module>
</config>
The sequence
tag ensures that Magento's core payment module is loaded before your custom module.
Step 2: Configure the Plugin
Magento uses plugins to intercept methods and modify their behavior. In this step, you’ll configure the plugin that will allow you to filter out specific payment methods on the frontend.
2.1 di.xml
The di.xml
file will define the plugin and specify that it should execute after the getAvailableMethods()
method of the Magento\Payment\Model\MethodList
class.
Create the di.xml
file in app/code/Vendor/DisableFrontPayment/etc/di.xml
:
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:noNamespaceSchemaLocation="urn:magento:framework:ObjectManager/etc/config.xsd">
<type name="Magento\Payment\Model\MethodList">
<plugin name="disable_frontend_methods"
type="Vendor\DisableFrontPayment\Plugin\MethodListPlugin"
sortOrder="10" />
</type>
</config>
This configuration tells Magento to apply your plugin after the getAvailableMethods()
method is called. The sortOrder="10"
defines the order in which multiple plugins are applied. You can adjust this value based on your needs.
Step 3: Implement the Plugin Logic
Now, you’ll implement the logic that filters the available payment methods. The plugin will check each available method and remove certain ones from the frontend list.
3.1 MethodListPlugin.php
Create the MethodListPlugin.php
file in
app/code/Vendor/DisableFrontPayment/Plugin/MethodListPlugin.php
. This file will implement the logic to remove specific payment methods from the list:
namespace Vendor\DisableFrontPayment\Plugin;
class MethodListPlugin
{
/**
* @param \Magento\Payment\Model\MethodList $subject
* @param array $result
* @return array
*/
public function afterGetAvailableMethods(
\Magento\Payment\Model\MethodList $subject,
array $result
): array {
// Filter out 'cashondelivery' from the list of available methods
foreach ($result as $key => $method) {
if ($method->getCode() === 'cashondelivery') {
unset($result[$key]);
}
}
return $result;
}
}
In this example, the plugin removes the "Cash on Delivery" payment method by checking its method code using $method->getCode()
. You can extend this logic to remove other payment methods or apply more complex conditions.
Step 4: Enable the Module and Verify Changes
Once the module and plugin are in place, you need to enable the module and run Magento’s setup commands to apply the changes.
4.1 Run Setup and Cache Commands
Use the following commands to enable the module and clear Magento’s cache:
php bin/magento setup:upgrade
php bin/magento cache:flush
This will apply the module configuration, enable the plugin, and clear any cached data.
4.2 Verification
- Frontend Checkout: Visit the frontend checkout page. You should now see that the "Cash on Delivery" payment method is no longer available.
- Admin Panel: Navigate to the admin panel, and verify that "Cash on Delivery" is still available for order creation. This ensures that the method is only disabled on the frontend, not in the backend.
Best Practices for Disabling Frontend Payment Methods
When creating custom plugins and modules to disable payment methods, it’s essential to follow best practices to ensure smooth operation and prevent conflicts. Here are some key recommendations:
Best Practice | Recommendation |
---|---|
Use After Plugins for Filtering | By using an "after" plugin, you ensure the original method executes first, allowing you to modify the result without disrupting the default behavior. |
Avoid Filtering in Admin Panel | Admin functionality should remain intact. Avoid removing methods from the admin order creation screens unless absolutely necessary. |
Add Configurable Options in Admin | Make the filtering rules configurable in the Magento Admin panel. This allows administrators to control which payment methods are available without modifying code. |
Log Filtered Methods During Testing | Enable logging to track which methods are being filtered out during testing. This can help debug issues and ensure the correct methods are excluded. |
Respect Module Dependencies | Ensure that other third-party modules or custom solutions do not rely on the payment methods you are disabling. Verify compatibility before applying changes. |
By adhering to these best practices, you ensure that your Magento store’s payment processing remains flexible, reliable, and efficient.
Additional Considerations and Use Cases
As your store grows, the need for more sophisticated payment method management may arise. Consider implementing additional logic for more complex use cases, such as:
- Custom Payment Method Filters: You can create conditions based on customer attributes, such as whether they are logged in, their purchase history, or their geographical location.
- Multiple Payment Methods Per Customer Group: Tailor the payment options available to specific customer groups, such as offering different payment methods for wholesale versus retail customers.
- Support for Multiple Store Views: If you operate multiple stores or store views (e.g., for different countries or regions), you can implement payment method filtering specific to each store view.
These advanced strategies can improve the user experience and streamline payment processing by providing only the most relevant and secure payment methods for your customers.
Advanced Techniques for Dynamic Payment Method Control in Magento 2.4.7
Magento 2.4.7 offers the flexibility to dynamically filter payment methods based on various storefront and customer-specific conditions. These advanced customization techniques help deliver a more tailored checkout experience while retaining backend operational efficiency.
Key Scenarios for Payment Method Filtering
Magento allows you to intercept and modify the payment method list using a plugin on MethodList::getAvailableMethods()
. The logic can be extended with business-specific rules, such as customer group segmentation, cart contents, shipping preferences, and more.
Scenario-Based Filtering Techniques
Scenario | Technical Approach |
---|---|
Restrict by Customer Group |
Inject the \Magento\Customer\Model\Session class into your plugin constructor. Within the
afterGetAvailableMethods() method, retrieve the logged-in customer's group ID. Filter payment
methods based on group-specific logic, such as hiding certain methods for B2C users and allowing them for B2B customers.
|
Apply Cart-Based Conditions |
Use \Magento\Quote\Model\Quote to retrieve the items in the cart. Loop through each item to determine
attributes like product type, SKU, or category. This is useful to block methods like Cash on Delivery for virtual
or downloadable products.
|
Depend on Shipping Method |
Access the shipping method selected by the customer using \Magento\Quote\Model\Quote\Address\Rate .
You can filter out payment methods based on specific shipping carriers or methods (e.g., disable Cash on Delivery
for international shipping options).
|
Additional Custom Use Cases
To extend the flexibility further, Magento’s plugin system allows combining multiple conditions to deliver refined payment method behavior.
Use Case | Implementation Notes |
---|---|
Region-Specific Restrictions | Use the customer's billing or shipping address data to restrict specific payment methods in unsupported countries or regions. This approach ensures compliance with local regulations and avoids transaction issues during checkout. |
Store View-Specific Control |
Retrieve the current store view using \Magento\Store\Model\StoreManagerInterface .
Enable or disable payment methods based on the store's branding, language, or regional audience.
Useful for multi-store setups targeting different customer segments.
|
Time-Sensitive Method Visibility |
Leverage \Magento\Framework\Stdlib\DateTime\TimezoneInterface to implement time-based logic.
This allows you to activate or hide payment options during specific campaigns, flash sales, or outside business hours.
|
Best Practices for Implementation
-
Always Use After Plugins: Use
afterGetAvailableMethods()
instead of before or around to avoid disrupting the original logic and preserve the integrity of method execution. -
Avoid Admin Area Filtering: Use
\Magento\Framework\App\State
to check if the area code isadminhtml
. Skip filtering to avoid unintended effects in backend operations like manual order creation or refunds. -
Introduce Config Settings: Add configuration options in the Admin UI (
system.xml
) so store managers can control payment method visibility rules without code changes. -
Log Filtered Methods During Testing: Enable debugging by logging removed methods to validate filtering logic. Use
\Psr\Log\LoggerInterface
for logging during development. - Validate Module Dependencies: Ensure compatibility if your store uses third-party modules that depend on specific payment methods. Improper removal may break workflows.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Conclusion
Managing payment visibility in Magento 2.4.7 is no longer just a developer trick—it's a best practice for modern, scalable eCommerce. By creating a lightweight custom module and using a safe plugin method, you can disable payment options like Cash on Delivery from the storefront while keeping them active in the backend for admins. This flexibility enhances your checkout flow, aligns with business strategies, and reduces unnecessary customer choices.
The beauty of this approach? It's upgrade-safe, modular, and fully customizable. You can apply advanced logic like filtering based on customer groups, shipping methods, or cart contents—all without touching core files.
Whether you're simplifying the frontend for a better user experience or tightening control for specific regions or B2B workflows, this method keeps your store clean, professional, and easy to manage.
Take full control of your Magento store’s payment system—one plugin at a time.
FAQs
How do I disable a payment method only on the frontend in Magento 2.4.7?
You can disable a payment method on the frontend by creating a custom module and using a plugin on Magento\Payment\Model\MethodList to remove specific methods during storefront rendering.
Will the disabled payment method still be available in the admin panel?
Yes, the payment method remains fully accessible in the Magento admin for manual order creation and management.
Which Magento class is used to fetch available payment methods?
Magento\Payment\Model\MethodList is used to fetch available payment methods during checkout on the frontend.
Is this approach upgrade-safe for future Magento versions?
Yes, this plugin-based approach doesn’t override core files, so it’s considered upgrade-safe and aligns with Magento development best practices.
Where should I place the plugin file for disabling frontend payment methods?
The plugin should be placed in Plugin/Model/Method/MethodAvailable.php
inside your module directory.
What is the purpose of di.xml in this module?
The di.xml
file defines the plugin and attaches it to Magento\Payment\Model\MethodList to intercept and modify available methods.
Which payment method is being disabled in the sample code?
The sample code disables the "Cash on Delivery" method (code: cashondelivery
) from the storefront only.
Can I disable multiple payment methods using the same plugin?
Yes, you can add multiple conditions in the plugin to unset multiple payment methods by their method codes.
Do I need to clear cache after module creation?
Yes, run php bin/magento cache:flush
after setup upgrade to ensure changes take effect properly.
Will this plugin affect payment methods during API-based checkout?
No, this plugin targets storefront rendering; API-based or admin checkout flows are unaffected unless customized further.
Can I use configuration settings to toggle frontend visibility dynamically?
Yes, you can enhance the plugin to check custom system config settings and conditionally disable methods at runtime.
Is it possible to disable payment method only for specific customer groups?
Yes, by injecting customer session in your plugin, you can filter payment methods based on group ID or login state.
What command is used to enable the new module?
Use php bin/magento setup:upgrade
to register and enable your custom module in Magento.
Will this customization impact the performance of the checkout page?
No, the plugin executes lightweight logic during payment method filtering and doesn’t affect checkout performance.
Can I log disabled payment methods for debugging?
Yes, inject a logger and add debug logs inside the plugin to track which methods are being removed during execution.