How to Programmatically Check if a File Exists at a Specific Location in Magento 2?
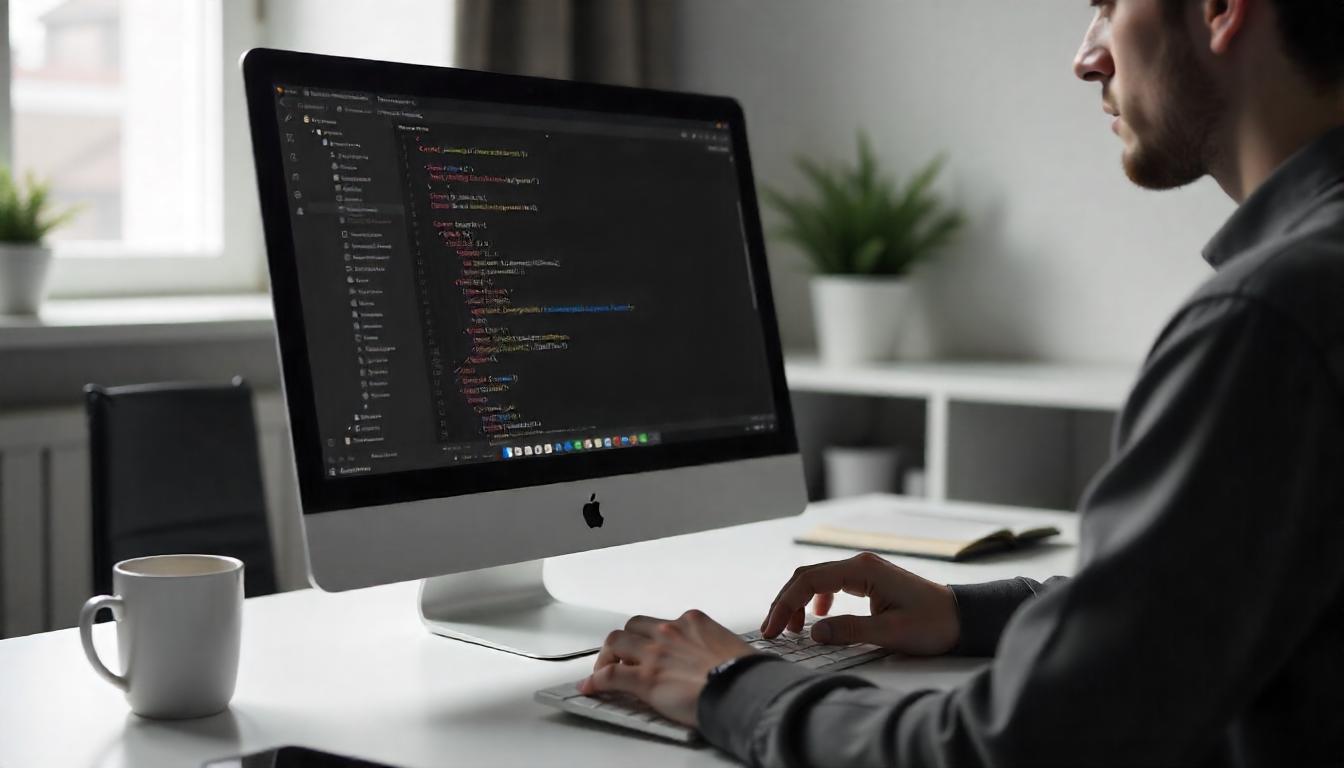
How to Programmatically Check if a File Exists at a Specific Location in Magento 2?
To check if a file exists at a specific location in Magento 2, you can use the Magento\Framework\Filesystem\Driver\File class. This method is more reliable than PHP’s native file_exists() function, as it integrates seamlessly with Magento's virtual file system.
Table Of Content
How to Programmatically Check if a File Exists at a Specific Location in Magento 2?
To check if a file exists at a specific path in Magento 2, use the Magento\Framework\Filesystem\Driver\File
class. This method is more reliable than PHP's native file_exists()
function, especially within Magento's virtual filesystem.
How to Check File Existence in Magento 2
Inject the File
driver into your class via dependency injection. Here's an example implementation in a custom block:
namespace Vendor\Module\Block;
use Magento\Framework\View\Element\Template;
use Magento\Framework\Filesystem\Driver\File;
class FileChecker extends Template
{
protected $fileDriver;
public function __construct(
Template\Context $context,
File $fileDriver,
array $data = []
) {
$this->fileDriver = $fileDriver;
parent::__construct($context, $data);
}
public function doesFileExist($filePath)
{
return $this->fileDriver->isExists($filePath);
}
}
In your template file, you can call this method:
$filePath = '/var/log/system.log';
if ($block->doesFileExist($filePath)) {
echo 'File exists.';
} else {
echo 'File does not exist.';
}
Best Practices
- Avoid Hardcoding Paths: Use Magento's directory list to get base paths, ensuring compatibility across environments.
Use Dependency Injection
: Always inject dependencies rather than instantiating them directly.- Handle Exceptions: Wrap file operations in try-catch blocks to handle potential exceptions gracefully.
Common Use Cases
Use Case | Description |
---|---|
Logging | Check if log files exist before writing to them. |
Media Management | Verify if media files exist before displaying them on the frontend. |
Configuration Files | Ensure configuration files are present before loading settings. |
By following these practices, you can reliably check for file existence in Magento 2, enhancing the robustness of your application.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
How can I programmatically check if a file exists in Magento 2?
You can use the Magento\Framework\Filesystem\Driver\File
class to check if a file exists at a specific location. This method is preferred over PHP’s native file_exists()
function within Magento.
How do I check if a file exists in the Magento directory?
Inject the File
driver class into your custom block and use its isExists($filePath)
method to check if a file exists at a given path.
Which class should I use to check if a file exists in Magento 2?
The Magento\Framework\Filesystem\Driver\File
class handles file system operations, including checking for file existence.
How do I check for file existence in a Magento 2 block?
You can create a method in your block, using dependency injection for File
, and call isExists()
to check if the file is present.
What method should I use to check if a file exists in Magento 2?
Use the isExists($filePath)
method of the File
class. It checks if the file exists at the given location.
How do I handle errors if a file does not exist?
If the file doesn’t exist, the method returns false
. Always handle the result by checking the return value and providing appropriate error handling.
Can I check if a file exists using a relative path?
Yes, you can use a relative path, but it’s recommended to use an absolute path for consistency and reliability, especially in different environments.
What should I do if the file path is incorrect?
Ensure the file path is correct before checking for existence. Incorrect paths may lead to failed file checks and silent errors. Always validate the path.
Where should I place the file for existence checking?
Place files in appropriate directories such as var/log
, app/code/YourVendor/YourModule/etc
, or other secure locations depending on your use case.