How to Convert CSV Data into Key-Value Pairs in Magento 2
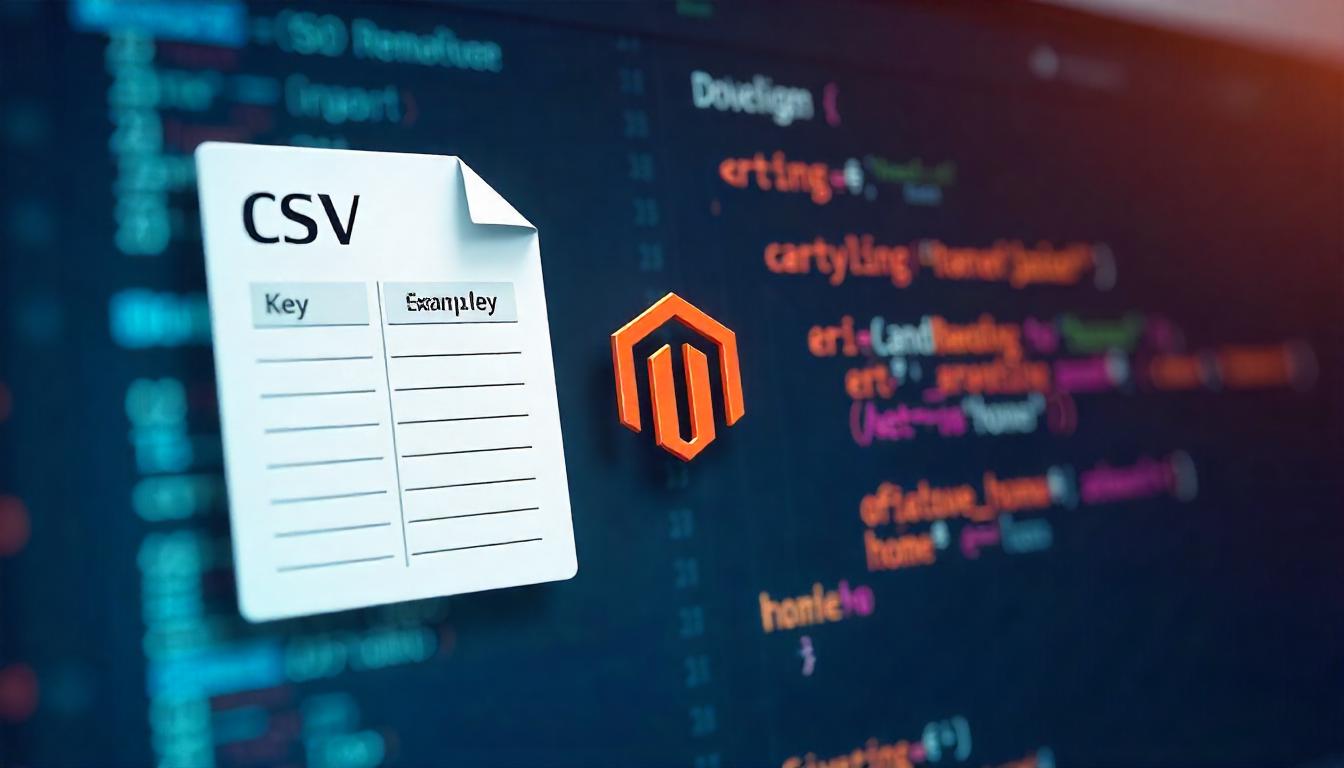
How to Convert CSV Data into Key-Value Pairs in Magento 2
Need to turn a two-column CSV file into a usable array in Magento 2? This guide shows you how to convert CSV data into key-value pairs using Magento’s built-in Csv class. You'll learn how to structure your file, load it securely, and extract the data with getDataPairs() for use in modules, configuration, or custom logic. Includes working PHP code and best practices.
Table Of Content
How to Convert CSV Data into Key-Value Pairs in Magento 2
If you're working with a CSV file that has two columns and want to convert it into a key-value pair array in Magento 2, the Magento\Framework\File\Csv
class provides a straightforward solution. This approach is particularly useful for handling configuration settings or language translations.
Step-by-Step Guide
Prepare Your CSV File
Ensure your CSV file is structured with two columns: the first column for keys and the second for values. For example:
"Sort By","Sorting"
"Homepage","home"
"cc_type","Credit Card type"
"Shopping Options","Shop By"
Implement the CSV Parsing Logic
Use the following PHP code to read the CSV file and convert it into a key-value pair array:
declare(strict_types=1);
namespace Vendor\Module\Model;
use Magento\Framework\Exception\FileSystemException;
use Magento\Framework\File\Csv;
use Magento\Framework\Filesystem\Driver\File;
class CsvToArray
{
public function __construct(
private readonly File $fileDriver,
private readonly Csv $csvParser
) {
}
/**
* Retrieve data from CSV file as key-value pairs
*
* @return string[]
* @throws FileSystemException
*/
public function getFileData(): array
{
$data = [];
$file = '/path/to/your/file.csv'; // Replace with your CSV file path
if ($this->fileDriver->isExists($file)) {
$this->csvParser->setDelimiter(',');
$data = $this->csvParser->getDataPairs($file);
}
return $data;
}
}
In this code:
- Replace '
/path/to/your/file.csv
' with the actual path to your CSV file. - The
getDataPairs()
method reads the CSV file and returns an associative array where the first column becomes the key and the second column becomes the value.
Handle Missing Files or Errors
Ensure that the CSV file exists at the specified path. If the file is missing or an error occurs during parsing, handle these situations gracefully to prevent application crashes.
Output Example
After executing the above code, the output will be an associative array like:
Array
(
[Sort By] => Sorting
[Homepage] => home
[cc_type] => Credit Card type
[Shopping Options] => Shop By
)
This array can now be used within your Magento 2 application for various purposes, such as setting configuration values or populating dropdown options.
Best Practices
- File Location: Store your CSV files in a secure and appropriate directory within your Magento 2 installation.
- Error Handling: Implement proper error handling to manage scenarios where the CSV file is missing or corrupted.
- Data Validation: Validate the data within your CSV files to ensure consistency and prevent potential issues during processing.
By following this approach, you can efficiently convert CSV data into key-value pairs in Magento 2, facilitating easier data management and integration within your application.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What is the simplest way to convert CSV data into key-value pairs in Magento 2?
Use the Magento\Framework\File\Csv
class and its getDataPairs()
method to convert a two-column CSV into an associative array.
How should my CSV file be structured for key-value conversion?
Make sure the file has exactly two columns. The first column will be used as the key, and the second as the value. For example: "Sort By","Sorting"
.
Which class handles CSV parsing in Magento 2?
The Magento\Framework\File\Csv
class is used to read and parse CSV files.
How do I check if the CSV file exists before reading it?
Use the Magento\Framework\Filesystem\Driver\File
class and call isExists($filePath)
to verify the file exists.
What’s the correct method to read key-value pairs from a CSV?
Call getDataPairs($filePath)
on the CSV parser instance. It returns an array with the first column as the key and the second as the value.
Can I use this method with more than two columns?
No. The getDataPairs()
method only works with two-column CSV files. Use getData()
for multi-column files.
What happens if the CSV file path is incorrect?
If the path is wrong or the file doesn’t exist, the method returns an empty array. Always check file existence first to avoid silent failures.
Can I use a relative path for the CSV file?
It’s safer to use an absolute path when calling getDataPairs()
, especially during development or in cron jobs.
Where should I place the CSV file in my Magento setup?
Place it in a secure directory like var/import
or app/code/YourVendor/YourModule/etc
, depending on your use case.