Unit Testing a Collection Factory in Magento 2
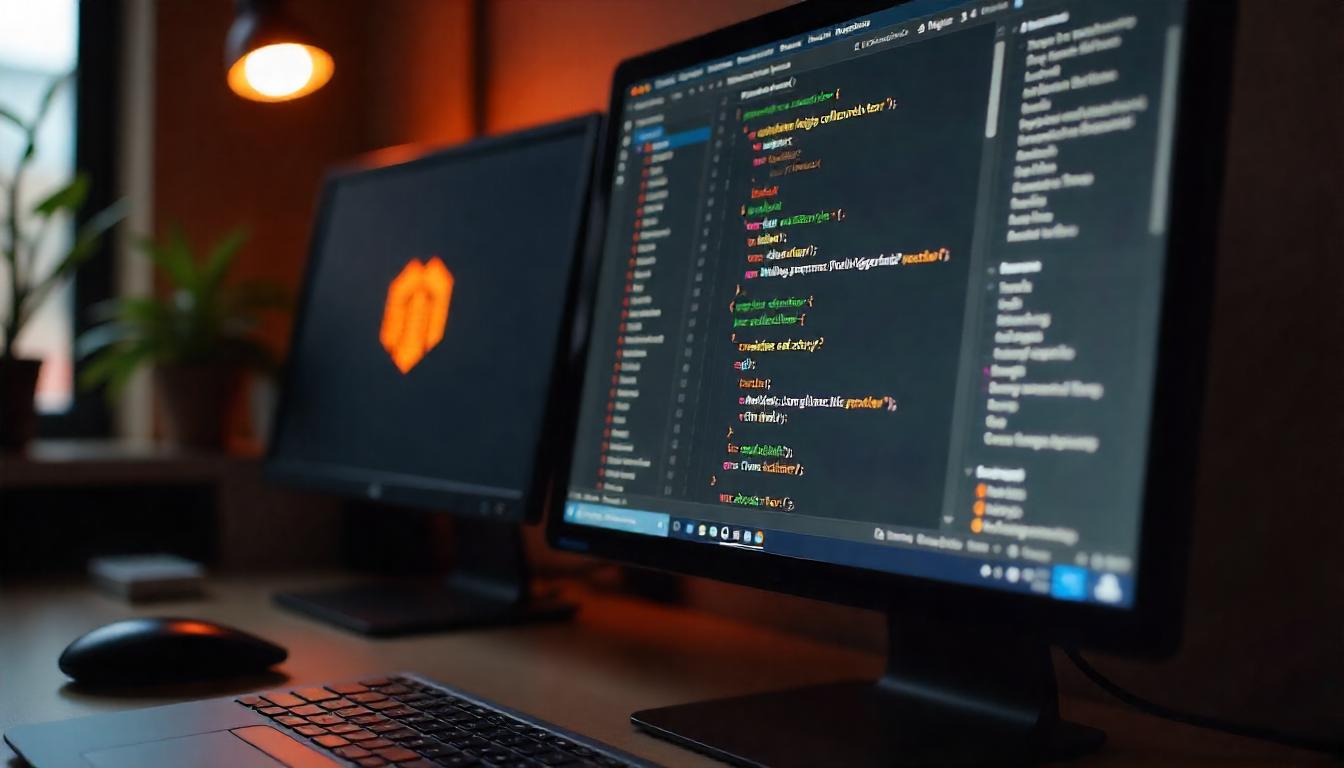
Unit Testing a Collection Factory in Magento 2
Learn how to properly unit test a Collection Factory in Magento 2. This guide walks you through mocking dependencies, testing data retrieval logic, and verifying expected results without hitting the database. Improve your code quality and ensure your collections behave as intended with PHPUnit best practices tailored for Magento 2 development.
Table Of Content
Unit Testing a Collection Factory in Magento 2
In Magento 2, unit testing with PHPUnit is essential for verifying the functionality of your code. This guide focuses on writing a unit test for a model that retrieves a product collection using a factory pattern.
Creating the Product Collection Model
First, define a model that fetches a product collection. This model uses ProductFactory
to create product instances and retrieve their collections.
<php
namespace Vendor\Module\Model;
use Magento\Catalog\Model\ProductFactory;
use Magento\Framework\Model\AbstractModel;
class ProductCollection extends AbstractModel
{
protected $productFactory;
public function __construct(
ProductFactory $productFactory
) {
$this->productFactory = $productFactory;
}
public function getProductsCollection()
$product = $this->productFactory->create();
$collection = $product->getCollection();
$collection->addAttributeToSelect('name')
->addAttributeToFilter('status', ['eq' => 1])
->setOrder('name');
return $collection;
}
}
Writing the PHPUnit Test
Next, create a unit test to verify the behavior of the getProductsCollection
method. This involves mocking dependencies and asserting that the method returns the expected collection.
<?php
namespace Vendor\Module\Test\Unit\Model;
use PHPUnit\Framework\TestCase;
use Magento\Catalog\Model\ProductFactory;
use Magento\Catalog\Model\Product;
use Magento\Catalog\Model\ResourceModel\Product\Collection;
use Vendor\Module\Model\ProductCollection;
class ProductCollectionTest extends TestCase
{
public function testGetProductsCollection()
{
$collectionMock = $this->createMock(Collection::class);
$collectionMock->expects($this->once())
->method('addAttributeToSelect')
->with('name')
->willReturnSelf();
$collectionMock->expects($this->once())
->method('addAttributeToFilter')
->with('status', ['eq' => 1])
->willReturnSelf();
$collectionMock->expects($this->once())
->method('setOrder')
->with('name')
->willReturnSelf();
$productMock = $this->createMock(Product::class);
$productMock->expects($this->once())
->method('getCollection')
->willReturn($collectionMock);
$productFactoryMock = $this->createMock(ProductFactory::class);
$productFactoryMock->expects($this->once())
->method('create')
->willReturn($productMock);
$productCollection = new ProductCollection($productFactoryMock);
$result = $productCollection->getProductsCollection();
$this->assertSame($collectionMock, $result);
}
}
Running the Test
Execute the unit test using the following command from the Magento root directory:
php vendor/bin/phpunit -c dev/tests/unit/phpunit.xml.dist app/code/Vendor/Module/Test/Unit/Model/ProductCollectionTest.php
Summary
This unit test ensures that the getProductsCollection
method in your model behaves as expected, retrieving a product collection with the specified attributes and filters. By mocking dependencies, you isolate the unit of work and verify its correctness without relying on the actual Magento framework or database.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What Is a Collection Factory in Magento 2?
A collection factory in Magento 2 is used to create and manage collections of model objects, enabling easy querying and filtering of data from the database.
Why Should I Unit Test a Collection Factory?
Unit testing a collection factory ensures that your collection logic behaves as expected, and helps catch bugs early in the development process. It also allows you to validate that data retrieval and manipulation are correct.
How Do I Create a Unit Test for a Collection Factory in Magento 2?
Create a test class in the Test
folder of your module, extending the Magento\TestFramework\TestCase\AbstractController
. Use the ObjectManager
to instantiate your collection factory, and assert the expected outcomes with PHPUnit methods.
What Dependencies Should I Mock When Testing a Collection Factory?
You should mock dependencies like the Magento\Framework\Model\ResourceModel\Db\Collection\AbstractCollection
, database models, and any other injected services used by the factory to isolate and test the collection logic.
How Can I Test If the Factory Returns the Correct Collection?
Use assertions in your unit test to verify that the factory method returns an instance of the expected collection type, and that the collection contains the expected data after applying filters and criteria.
How Do I Handle Collection Filtering in Unit Tests?
In your unit test, you can mock the filtering methods, like addFieldToFilter()
, to simulate filtering behavior and assert that the collection contains the correct results based on the applied filters.
What Tools Should I Use for Unit Testing a Collection Factory in Magento 2?
Use PHPUnit as the testing framework, Magento’s Magento\TestFramework
for setting up tests, and mock objects for simulating dependencies like database connections and model classes.
How Do I Test Database Interactions in a Collection Factory?
In unit tests, avoid interacting with the actual database. Instead, mock the database calls and use assertions to verify that the correct SQL queries are being executed, or that the right methods are being called on the database connection.
Where Can I Learn More About Unit Testing in Magento 2?
Check out Magento’s official documentation on unit testing, explore community blog posts, and review the Magento 2 test framework on GitHub for detailed examples and best practices.