Dropping or Removing Tables with DataPatchInterface in Magento 2
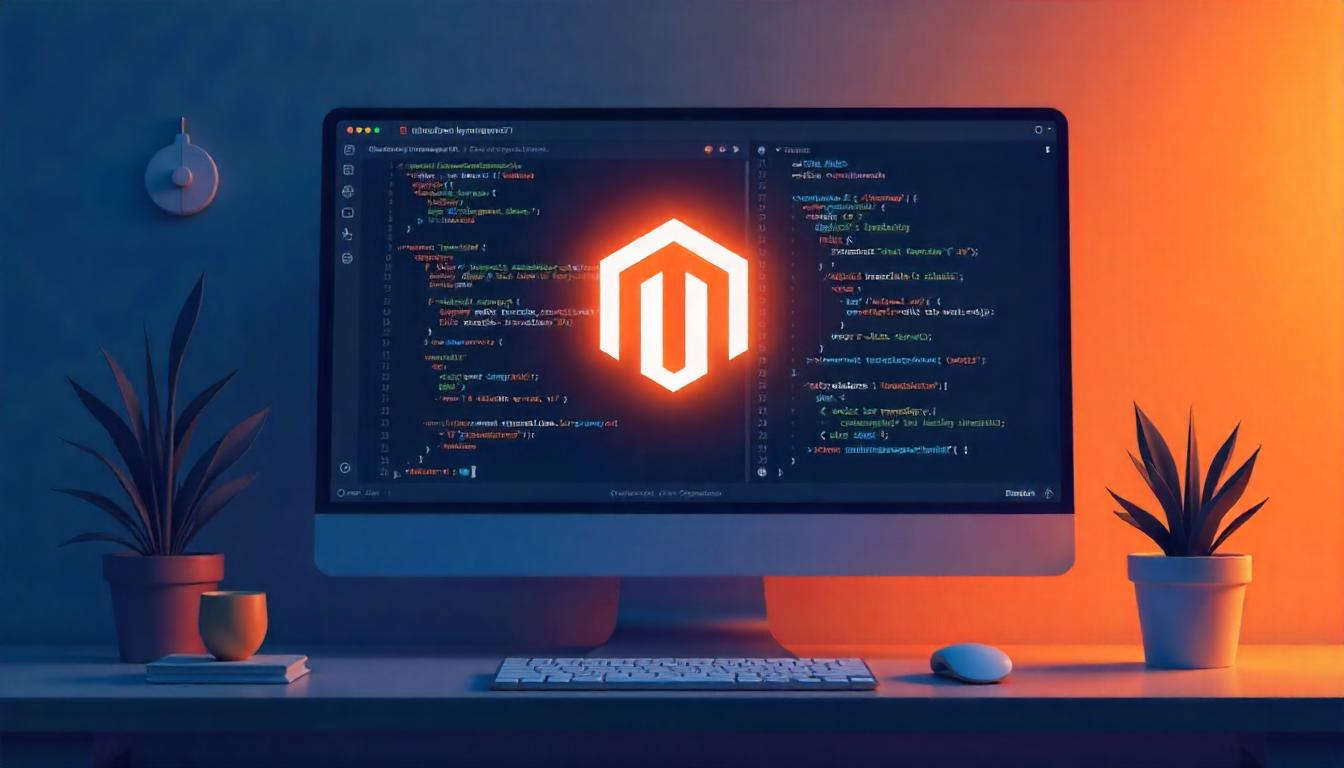
Dropping or Removing Tables with DataPatchInterface in Magento 2
In Magento 2, the introduction of Setup Patch Data in Magento 2.3 streamlined database schema updates, providing a better approach to modify or remove database tables. One of the most powerful tools for managing database changes is the DataPatchInterface, which allows developers to remove unnecessary tables without causing disruption to other operations.
This guide will walk you through how to drop or remove a database table using the DataPatchInterface in Magento 2.4.7, helping you keep your database clean and efficient.
Table Of Content
- Why Magento Developers Should Use DataPatchInterface for Data Changes
- Preparing Your Magento Module for Data Patch Execution
- Writing a Data Patch to Remove a Database Table in Magento 2
- Advanced Techniques and Best Practices for Managing Table Removals via Data Patches
- Essential Magento 2 Data Patch Operations
- Conclusion
- FAQs
Why Magento Developers Should Use DataPatchInterface for Data Changes
Magento 2.4 and above encourages the use of DataPatchInterface
as the preferred way to make data-level changes. This modern system replaces the older InstallData
and UpgradeData
scripts, aligning Magento development with best practices in modular programming, version control, and CI/CD compatibility.
What Is DataPatchInterface?
DataPatchInterface
is part of Magento’s declarative schema system. It allows developers to:
- Add or update data in the database during module installation or upgrade.
- Apply changes in a modular, versioned, and trackable way.
- Chain patches with dependencies and revert them if necessary.
Benefits of Using DataPatchInterface
Feature | Description | Why It Matters |
---|---|---|
Atomic Patches | Each patch is self-contained. | Avoids side effects and makes troubleshooting easier. |
One Patch = One Responsibility | Each patch targets a specific action. | Promotes clean, readable code. |
Track Execution | Patches are logged in the patch_list DB table. |
You know exactly which patches ran and when. |
Safe CI/CD Deployments | Works consistently in automated environments. | Supports DevOps workflows and Git-based deployment. |
Revertibility | Optional revert() method for undoing changes. |
Useful for QA, testing, or temporary data patches. |
Supports Dependencies | Use getDependencies() to run patches in a specific order. |
Ensures critical sequences are honored. |
Real Use Cases for DataPatchInterface
Using DataPatchInterface
allows developers to safely and cleanly insert, update, or configure data during module installation or upgrade. Below is a comprehensive table highlighting practical scenarios where this interface proves valuable.
Common Scenarios in Custom Modules
Use Case | Purpose |
---|---|
Add CMS pages or blocks | Create default CMS pages like “Home,” “Privacy Policy,” or insert reusable static blocks. |
Insert custom data rows | Programmatically insert default entries into your module-specific tables during setup. |
Set config values | Automatically configure system values in core_config_data , like enabling features by default. |
Create product attributes | Add EAV attributes such as dropdowns, text fields, or booleans tied to product or customer entities. |
Associate entities | Automatically link products to categories, assign customers to specific groups, or tags. |
Create roles and permissions | Setup custom admin user roles or ACL permissions required by your module. |
Advanced and Niche Scenarios
Use Case | Purpose |
---|---|
Seed third-party integrations | Pre-fill credentials or endpoints for external APIs your module connects to. |
Define cart price rules | Create default promotional rules like discounts or coupons on module install. |
Create URL rewrites | Add default SEO-friendly URL rewrites for custom frontend routes or pages. |
Configure shipping/payment settings | Setup custom shipping methods, fees, or payment gateways with predefined defaults. |
Set default tax rules | Load custom tax classes, rates, and rules needed for your store’s region. |
Insert default data for testing | Populate dev environments with sample data for products, categories, and customers. |
Why It Matters
- Automation: Reduces manual setup during fresh deployments.
- Reproducibility: Ensures consistent data across staging, testing, and production.
- Version Control Friendly: Patches are in code, not in the database directly—ideal for Git workflows.
- Easier QA: Helps QA teams test modules with predefined baseline data.
- Custom Module Reliability: Guarantees modules have the necessary data to function post-install or upgrade.
Modernizing Magento Setup: Data Patches vs. Traditional Scripts
When managing data-related logic in Magento modules, it’s essential to choose a method that promotes maintainability, clarity, and modularity. Below are key differences between traditional UpgradeData.php scripts and the modern DataPatchInterface approach, along with extended explanations and use cases.
1. Modularity and Structure
- Traditional Scripts:
All upgrade logic is typically bundled in a single UpgradeData.php file. Over time, this becomes a tangled mix of unrelated logic, making it hard to manage. - Data Patches:
Each patch lives in its own class. One patch does one thing. This makes it modular, focused, and much easier to test, maintain, and debug.
Example:
Want to add a default CMS page? Just create AddCmsHomePage.php. No need to touch a general upgrade file.
2. Trackability and Version Control
- Traditional Scripts:
It’s difficult to determine which updates ran and when, especially on larger projects or CI/CD pipelines. - Data Patches:
Every patch is automatically recorded in Magento’spatch_list
table. You can clearly see the execution status of each one.
3. Execution Order and Dependencies
- Traditional Scripts:
Execution relies on hardcoded logic and version comparisons. There's no built-in way to enforce dependencies between changes. - Data Patches:
Patches can explicitly declare dependencies using thegetDependencies()
method. Magento will ensure they run in the correct order.
Example:
Create an attribute after its attribute set is available—just define that dependency in the patch.
4. Reusability and Testability
- Traditional Scripts:
Typically procedural, not reusable or test-friendly. Not easy to isolate logic for unit or integration tests. - Data Patches:Written as separate PHP classes, making them easier to reuse across environments or test suites.
You can inject services or repositories into patches, enabling cleaner logic.
5. Rollback and Revertibility
- Traditional Scripts:
No native rollback support. If you need to undo something, you have to manually reverse changes and re-deploy. - Data Patches:
You can optionally implement the\Magento\Framework\Setup\Patch\PatchRevertableInterface
and define arevert()
method.
Perfect for QA and development environments where data needs to be frequently reset.
Why Data Patches Are Preferred
- Cleaner codebase
- Easier collaboration across teams
- Ideal for agile, modular development
- Consistent across environments (local, staging, production)
- Promotes better deployment practices with fewer bugs
Preparing Your Magento Module for Data Patch Execution
Using DataPatchInterface
to handle database operations such as removing tables or modifying data structures is a modern and efficient way to manage module updates in Magento 2. Before writing the patch itself, your custom module must be properly structured to support Magento’s patching system.
This section explains how to set up your module if you're starting from scratch, and what the folder structure should look like to ensure Magento recognizes your data patches correctly.
Step 1: Create or Use a Custom Module
If you don’t already have a module, create one. Each Magento patch file must live within a valid module that’s registered and recognized by the system.
Step 2: Understand the Required Directory Structure
Magento expects data patches to be located in a specific directory inside your module. Below is an example directory layout for a module named Jesadiya_DropTable.
app/code/Emmo/DropTable/
├── etc/
│ └── module.xml
├── registration.php
├── Setup/
│ └── Patch/
│ └── Data/
│ └── RemoveExtraTable.php
Explanation:
- app/code/: Root folder where all custom modules are placed.
- Emmo/DropTable: Vendor and module name. You can replace
Emmo
with your own vendor name. - etc/module.xml: Describes the module name and version.
- registration.php: Tells Magento how to register this module.
- Setup/Patch/Data/: Magento scans this location to locate and run any data patches during
bin/magento setup:upgrade
.
Step 3: Register the Module
Ensure the registration.php
and module.xml
files exist and are properly configured.
registration.php
<?php
use Magento\Framework\Component\ComponentRegistrar;
ComponentRegistrar::register(
ComponentRegistrar::MODULE,
'Emmo_DropTable',
__DIR__
);
etc/module.xml
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:noNamespaceSchemaLocation="urn:magento:framework:Module/etc/module.xsd">
<module name="Emmo_DropTable" setup_version="1.0.0"/>
</config>
Step 4: Understand the Patch File Placement
Inside the Setup/Patch/Data/
directory, you’ll create patch classes that extend Magento's DataPatchInterface
. For example, RemoveExtraTable.php
will contain the logic to drop a custom table from your database schema.
Magento will automatically detect this class and execute it during the setup:upgrade
command—if it hasn't already been run.
Step 5: Run the Setup Upgrade Process
After the patch class is in place and your module is registered:
php bin/magento setup:upgrade
Magento will:
- Register your patch
- Execute it if it hasn’t already run
- Record its status in the patch_list database table
This ensures that it won't run again unless explicitly reverted or modified with a new class name.
Best Practices for Module and Patch Setup
- Keep patches atomic — do one thing in each patch file.
- Use namespaces consistently to avoid class name collisions.
- Use dependency injection instead of direct object manager usage.
- Make patches reversible by implementing the PatchRevertableInterface when appropriate.
- Log patch actions for better traceability in development environments.
Writing a Data Patch to Remove a Database Table in Magento 2
When maintaining or refactoring a Magento module, there are situations where you may need to remove unused or temporary tables from the database. Magento 2’s DataPatchInterface
provides a clean and trackable way to manage this kind of update.
In this section, you'll learn how to create a data patch
that removes a table named image_storage_tmp
using Magento’s patch system.
Overview: Why Use a Data Patch for Table Removal?
Using DataPatchInterface
for schema-related tasks like dropping a table ensures:
- Your logic is version-controlled and reproducible
- It's automatically integrated into Magento's upgrade process
- You can easily manage execution order and dependencies
- The action is logged in the
patch_list
table for transparency
Step-by-Step: Creating the Patch Class
Follow the steps below to safely remove the image_storage_tmp
table as part of your module update.
Step 1: File Path and Structure
Create a new file named RemoveExtraTable.php in the following path:
app/code/Emmo/DropTable/Setup/Patch/Data/RemoveExtraTable.php
Ensure your module is already registered and follows the correct directory structure.
Step 2: The Complete Patch Class
Here’s the full code to define the data patch:
<?php declare(strict_types=1);
namespace Jesadiya\DropTable\Setup\Patch\Data;
use Magento\Framework\Setup\SchemaSetupInterface;
use Magento\Framework\Setup\Patch\DataPatchInterface;
class RemoveExtraTable implements DataPatchInterface
{
const IMAGE_STORAGE = 'image_storage_tmp';
private $schemaSetup;
public function __construct(
SchemaSetupInterface $schemaSetup
) {
$this->schemaSetup = $schemaSetup;
}
public static function getDependencies(): array
{
return [];
}
public function getAliases(): array
{
return [];
}
public function apply(): void
{
$installer = $this->schemaSetup;
$installer->startSetup();
if ($installer->tableExists(self::IMAGE_STORAGE)) {
$installer->getConnection()->dropTable(
$installer->getTable(self::IMAGE_STORAGE)
);
}
$installer->endSetup();
}
}
Detailed Breakdown of Key Components
SchemaSetupInterface
This object provides access to Magento’s setup tools and database connection. It handles things like verifying table existence and performing schema changes within setup transactions.
startSetup()
and endSetup()
These methods ensure that Magento handles transactions properly, allowing changes to be rolled back if needed during upgrade processes.
tableExists()
A safeguard method to prevent errors if the specified table is already removed or missing.
dropTable()
Executes the SQL command to delete the specified table from the database schema.
Running the Patch
Once the patch file is in place, execute the following command from your Magento root directory:
php bin/magento setup:upgrade
This will trigger Magento’s patch system to:
- Register your
RemoveExtraTable
patch - Run it if it hasn’t been previously executed
- Drop the
image_storage_tmp
table if it exists
You can confirm the patch ran by checking the patch_list
table.
Verifying That the Table Was Removed
You can confirm successful removal by checking directly in the database.
Use the following SQL command:
SHOW TABLES LIKE 'image_storage_tmp';
If the patch was applied and the table removed successfully, this command will return no results.
Best Practices When Using Data Patches for Table Cleanup
- Always check for table existence before attempting to drop it
- Keep your patch logic isolated—one patch per change
- Document the purpose of your patch with clear naming and inline comments
- Register patches in a consistent namespace and module path
- Consider implementing
PatchRevertableInterface
if you want to add rollback support
Advanced Techniques and Best Practices for Managing Table Removals via Data Patches
Data patches in Magento 2 are powerful tools for handling one-time, versioned changes in a reliable and trackable way. While typically used for inserting data or modifying configuration, they can also handle advanced database schema operations like removing multiple tables.
This section walks through multi-table handling, rollback strategies, and how to safely include schema changes within your data patch workflow.
Handling Multiple Table Removals in One Patch
If your module cleanup or upgrade involves removing more than one database table, it’s better to consolidate them into a single patch rather than creating multiple patches for each table. This approach ensures efficiency and reduces unnecessary overhead in patch registration.
You can modify the apply()
method of your DataPatchInterface
implementation to handle multiple table names in an array, then loop through and drop each if it exists.
This technique is useful when cleaning up legacy modules, test data tables, or deprecated feature tables.
Key Tips
- Always wrap schema modifications within
startSetup()
andendSetup()
to ensure consistency. - Log or comment on each table name to track what you're deleting.
- Use descriptive names for patch classes to reflect multiple actions, like
CleanObsoleteTables
.
Suggested Code Behavior for Multiple Table Deletions
Inside your patch class, build an array of target tables. Iterate through it, checking for existence before dropping each one using $installer->getConnection()->dropTable()
.
This ensures you avoid errors and maintain clean execution logs.
Recommended Strategy for Patch Rollback
Magento’s DataPatchInterface
does not natively support automatic rollback. However, there are practical strategies you can follow to implement manual or assisted rollbacks.
Strategies to Handle Rollback
Version Control Rollbacks
>Use Git branches or commit checkpoints to revert changes and patch classes.
Backup First, Patch Later
Always create a backup of your database before running any destructive patch. This is especially important in staging or production environments.
Patch Flags or Custom Logs
Store a custom flag or record in a tracking table when a destructive patch is applied. This lets your team verify patch execution status and take informed rollback actions.
Separate Revert Patch
If needed, create another patch class that recreates the removed tables with their original structure. This allows you to manually undo a patch for QA or testing purposes.
Using Data Patches for Schema-Level Changes
Although DataPatchInterface
is primarily used for data operations, it is flexible enough to support schema-level changes when used carefully with SchemaSetupInterface
.
What You Can Do in Schema-Level Patches
- Add or drop columns in existing tables
- Create new tables or indexes
- Rename tables or fields
- Modify data types or constraints
- Drop foreign keys or add new ones
When implementing schema-level changes, always:
- Include proper checks for existing structures
- Use safe default values for new columns
- Avoid large operations in production without downtime or maintenance mode
Pro Tips for Reliable Patch Management
- Keep patches atomic: One patch should ideally serve one purpose. Use multiple small patches over one large one unless performance demands otherwise.
- Use dependencies wisely: If one patch depends on another, declare it using getDependencies() to enforce execution order.
- Avoid irreversible operations in data patches: Especially if working in shared environments, ensure all destructive operations are reviewed and agreed upon.
- Maintain logs: Custom modules can log patch executions or insert into an audit table for long-term reference.
- Test in isolated environments first: Always validate schema patches in a sandbox or local environment to confirm no side effects exist.
Removing tables via DataPatchInterface is a clean, maintainable way to eliminate legacy data structures from your Magento 2 database. When done properly, it integrates smoothly with the bin/magento setup:upgrade process, keeps your deployment safe, and supports versioned changes in a CI/CD workflow.
Make sure to:
- Use loop-based logic for multiple table removals
- Implement rollback strategies or backups
- Take advantage of the flexibility of
SchemaSetupInterface
for schema modifications
Let me know if you'd like a sample rollback patch, schema modification example, or CI-compatible patch management strategies.
Essential Magento 2 Data Patch Operations
Magento 2’s DataPatchInterface
enables developers to perform controlled, versioned database changes such as table creation, column modification, index handling, and more. These operations are particularly valuable during module installation, upgrades, or structural adjustments in CI/CD workflows.
This guide breaks down the most common operations, their Magento methods, and practical examples, while also offering key insights into usage patterns and best practices.
Overview: When to Use Schema-Level Data Patches
While data patches are often used for inserting default values or creating entities like CMS pages and attributes, they can also safely handle schema changes when wrapped within the patch lifecycle.
Use schema-level operations within a DataPatchInterface
only when:
- The module requires custom database structures
- You need to remove deprecated tables or fields
- The patch has no dependencies on external modules not yet initialized
- Your CI/CD pipeline supports non-interactive patch execution
Most Common Schema Operations in Data Patches
The table below outlines the primary database operations typically used in Magento patches and the relevant PHP methods provided by Magento’s DB\Adapter\AdapterInterface:
Operation | Magento Method | Usage Example |
---|---|---|
Create a Table | $installer->getConnection()->createTable() |
Used to define a brand-new table with all required columns and constraints |
Drop a Table | $installer->getConnection()->dropTable() |
Deletes a table if it exists |
Add a Column | $installer->getConnection()->addColumn() |
Adds a new column to an existing table |
Modify a Column | $installer->getConnection()->changeColumn() |
Changes an existing column’s type, name, or definition |
Drop a Column | $installer->getConnection()->dropColumn() |
Removes a specific column from a table |
Add an Index | $installer->getConnection()->addIndex() |
Adds indexes to columns to improve performance or enforce uniqueness |
Drop an Index | $installer->getConnection()->dropIndex() |
Removes indexes that are no longer needed |
Add a Foreign Key | $installer->getConnection()->addForeignKey() |
Links two tables through primary/foreign key relationship |
Drop a Foreign Key | $installer->getConnection()->dropForeignKey() |
Removes foreign key constraint from a table |
Rename a Table | $installer->getConnection()->renameTable() |
Changes the name of an existing table |
Rename a Column | $installer->getConnection()->changeColumn() |
Renames or changes the definition of a column using the same method |
Create an Auto-Increment Column | $installer->getConnection()->addColumn() |
Specify IDENTITY => true for auto-incrementing IDs |
Tips for Writing Schema-Safe Data Patches
Wrap Every Operation in Setup Start/End
Always begin with $installer->startSetup()
and finish with $installer->endSetup()
to avoid inconsistent behavior in patch execution.
Check Before Acting
Always verify existence using helper methods like tableExists()
and tableColumnExists()
before attempting destructive operations.
Use Constants for Table and Column Names
Avoid hardcoding table or column names. Define constants or use $installer->getTable('your_table')
for portability.
Structure Patches Clearly
Break patches into small, focused responsibilities. One patch per logical change is ideal for traceability and maintainability.
Avoid Complex Joins or Transactions Inside Patches
Use setup patches only for schema or safe data initialization, not business logic or batch processing.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Conclusion
Dropping a database table using Magento 2.4.7’s DataPatchInterface
isn’t just a technical task — it’s a best practice in keeping your store’s backend lean, efficient, and maintainable. Whether you're cleaning up leftover tables from old modules, streamlining your schema, or just tightening up performance, using DataPatchInterface
ensures you’re doing it the Magento way — safely and version-controlled.
In this tutorial, we built a complete example using a real-world scenario: removing the image_storage_tmp
table with a custom module and patch class. We followed Magento's modern setup system, leveraged SchemaSetupInterface
, and safely dropped the table only if it existed. Along the way, we covered advanced tips, rollback ideas, and troubleshooting advice to give you full control.
Magento 2.4.7 continues to support developers with more structured upgrade flows, and using data patches instead of legacy setup scripts is a major part of that improvement. From creating your patch to verifying it with setup:upgrade
, the entire process remains flexible, reliable, and scalable.
FAQs
What is DataPatchInterface in Magento 2?
DataPatchInterface is part of Magento's declarative schema system introduced in Magento 2.3+. It allows developers to perform data-related operations like inserting or deleting data during setup upgrades.
How can I drop a table using a patch in Magento 2.4.7?
You can use the DataPatchInterface in combination with SchemaSetupInterface to safely check if the table exists and drop it using the dropTable() method.
Where should I place my Data Patch class to remove a table?
Your patch class should go inside Setup/Patch/Data
within your custom module directory.
What is the correct way to check if a table exists before dropping it?
Use $installer->tableExists('table_name')
to ensure the table exists before executing dropTable()
.
Do I need to run any command after creating the patch?
Yes, run bin/magento setup:upgrade
to apply the patch and drop the table.
Will the dropped table come back after cache refresh or reindexing?
No, once dropped via DataPatchInterface, the table will be permanently removed unless recreated in another patch or install schema.
Is dropping a table via patch reversible?
No, the DataPatchInterface doesn’t support automatic rollback. You’ll need to create a separate patch if you wish to recreate the table.
What is SchemaSetupInterface used for in the patch?
SchemaSetupInterface allows interaction with the Magento database setup process, including executing schema changes like dropping tables.
Can I drop multiple tables in one patch file?
Yes, you can check and drop multiple tables within the same apply()
method in your patch file.
Is it safe to run setup:upgrade in production?
While it is generally safe, it's recommended to run setup:upgrade
during a maintenance window on production environments.
How do I know if my patch has been applied?
Magento stores patch status in the patch_list
database table. You can also verify manually if the table was removed.
What is the naming convention for a table constant in a patch?
Use all uppercase letters with underscores, like IMAGE_STORAGE_TMP
, for defining table names as class constants.
Why is my patch not being executed?
Ensure your patch implements DataPatchInterface
, is placed correctly, and that setup:upgrade
has been executed.
Is this patch method available in Magento 2.4.7?
Yes, Magento 2.4.7 fully supports Data Patches and the use of SchemaSetupInterface for structural operations like table removal.
What should I do before removing a table?
Always back up your database and ensure the table is not being used by any active module or feature before deleting it.