Suppressing 'Line Exceeds Maximum Limit' Warnings in PHP_CodeSniffer for Magento 2
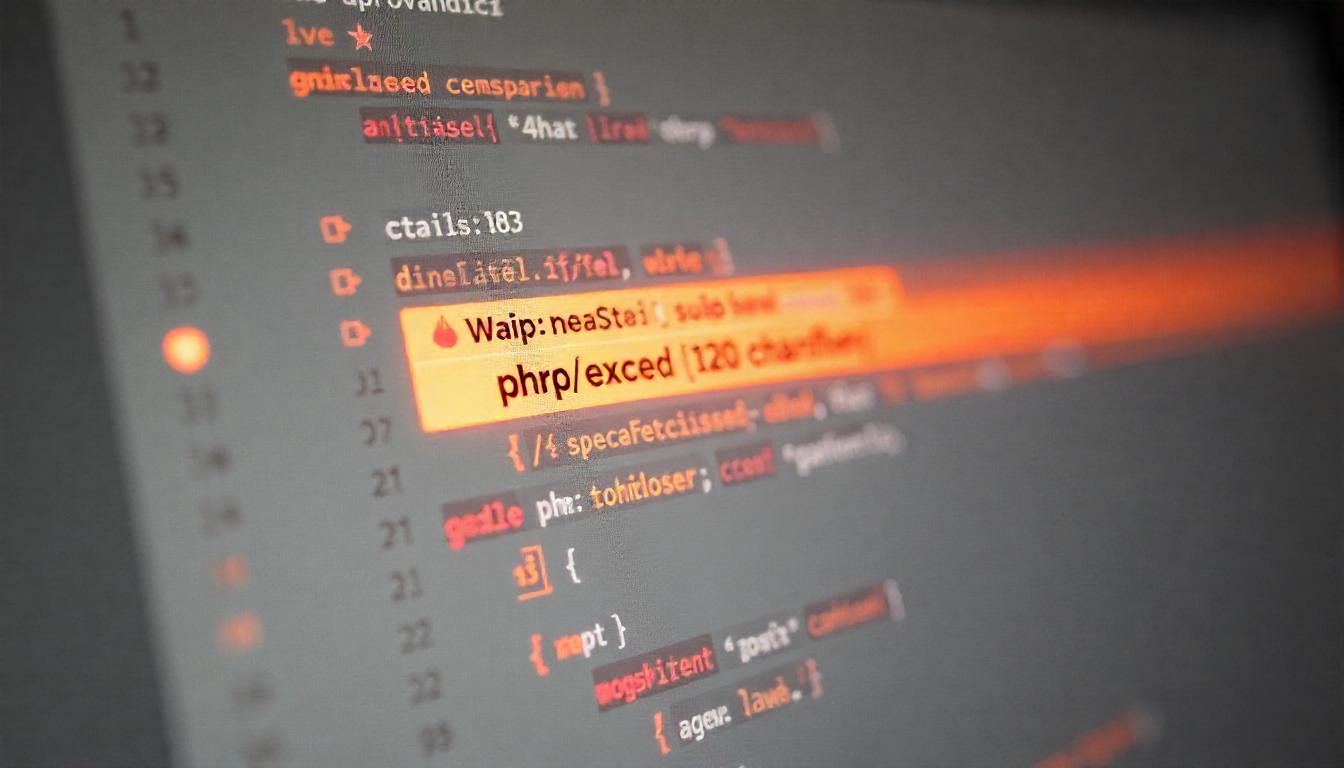
Suppressing 'Line Exceeds Maximum Limit' Warnings in PHP_CodeSniffer for Magento 2
When working with Magento 2, PHP_CodeSniffer often flags lines that exceed a specific character limit—typically 120 characters—as a warning. These warnings can clutter your reports, especially when long strings or URLs are involved.
Suppressing 'Line Exceeds Maximum Limit' Warnings in PHP_CodeSniffer for Magento 2
IWhen working with Magento 2, you might encounter PHP_CodeSniffer (PHPCS)
warnings indicating that a line exceeds the maximum character limit, such as:
Warning: Line exceeds maximum limit of 120 characters; contains 230 characters
To address these warnings, you have several options:
1. Ignoring Specific Lines
To suppress the warning for a particular line, append // phpcs:ignore
at the end of that line:
<?php
$messageContent[] = [
'title' => 'Create an Account',
'identifier' => 'create-an-account',
'content' => 'Account with this email address already exists. If you are sure that it is your email address, click the link to get your password.', // phpcs:ignore
'is_active' => 1
];
2. Disabling and Enabling PHPCS Checks Around Code Blocks
To ignore warnings for an entire block of code, you can disable PHPCS before the block and enable it afterward:
<?php
/**
* @return string[]
* phpcs:disable
*/
public function cmsContent()
{
$messageContent[] = [
'title' => 'Create an Account',
'identifier' => 'create-an-account',
'content' => 'Account with this email address already exists. If you are sure that it is your email address, click the link to get your password.',
'is_active' => 1
];
}
/** phpcs:enable */
This approach disables PHPCS checks for the enclosed code, allowing you to bypass multiple warnings within that section.
3. Excluding the Line Length Rule in PHPCS Configuration
If you prefer to exclude the line length rule globally or for a specific project, you can modify your PHPCS configuration:
- Using Command-Line Exclusion:
Run PHPCS with the --exclude flag
to exclude the Generic.Files
.LineLength rule:
vendor/bin/phpcs --standard=PSR2 --exclude=Generic.Files.LineLength app/
This command tells PHPCS to apply the PSR2 standard but exclude line length checks.
- Customizing the Ruleset XML:
Create or modify a phpcs.xml file in your project's root directory to exclude the line length rule:
<?xml version="1.0"?>
<ruleset name="Custom">
<description>Custom coding standard excluding line length check.</description>
<rule ref="PSR2">
<exclude name="Generic.Files.LineLength"/>
</rule>
</ruleset>
This configuration applies the PSR2 standard while excluding line length warnings.
Considerations
While it's possible to suppress line length warnings, it's generally advisable to adhere to coding standards for readability and maintainability. Before ignoring these warnings, consider refactoring your code to comply with the recommended line length. Suppress warnings only when necessary, such as when dealing with long strings or URLs that cannot be broken down.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What Does "Line Exceeds Maximum Limit" Mean in PHP_CodeSniffer?
This warning means a line in your PHP file has more characters than allowed by the coding standard—commonly 120 characters in Magento 2.
How Can I Suppress the Line Length Warning in Magento 2?
You can suppress it by adding // phpcs:ignore
at the end of the long line, or by wrapping the code block between phpcs:disable
and phpcs:enable
comments.
Can I Ignore the Warning for a Specific Line Only?
Yes. Just add // phpcs:ignore
after the line that exceeds the character limit. This suppresses the warning for that line only.
How Do I Ignore a Whole Block of Code in PHPCS?
Wrap the block of code with phpcs:disable
before and phpcs:enable
after. PHPCS will skip the entire section when checking.
Is There a Way to Disable the Rule in PHP_CodeSniffer Settings?
Yes. You can create or edit a phpcs.xml
file to exclude Generic.Files.LineLength
from your coding ruleset.
Can I Skip the Line Length Rule via Command Line?
Yes. Use the --exclude=Generic.Files.LineLength
flag with your PHPCS command to skip the check for line length violations.
Should I Always Ignore Line Length Warnings?
No. It’s better to refactor your code to fit the line length limit. Suppress only when necessary, like when handling long strings or URLs.
What Are the Risks of Suppressing Line Length Warnings?
Ignoring these warnings too often can lead to unreadable code. Stick to Magento coding standards whenever possible for consistency and clarity.
Where Can I Learn More About PHPCS in Magento 2?
Refer to the official Magento DevDocs and community blogs. The Magento 2 GitHub repo and developer forums also provide examples and best practices.