Fetching Payment Transaction Details in Magento 2
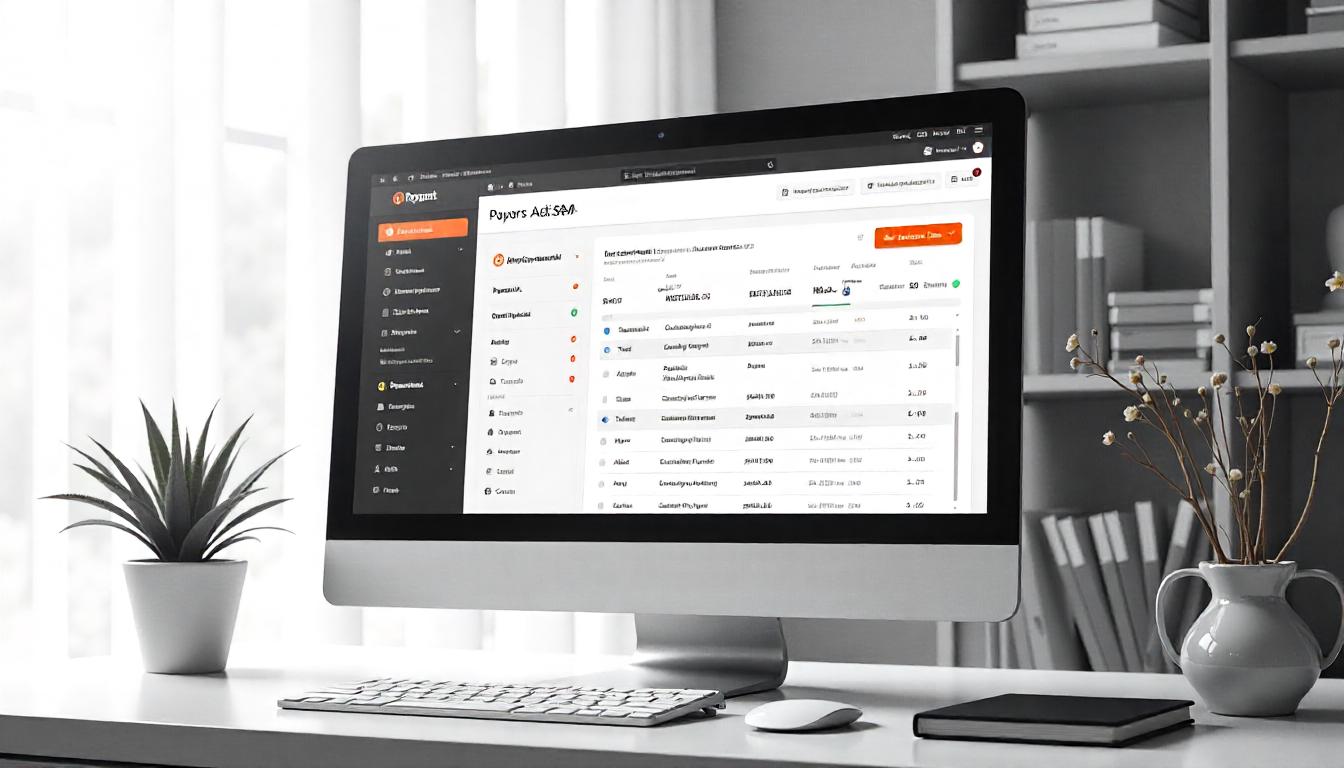
Fetching Payment Transaction Details in Magento 2
Retrieving payment transaction details in Magento 2 is essential for tracking orders and managing payment data. Using the TransactionRepositoryInterface, you can fetch details like transaction ID, parent transaction ID, order ID, payment ID, transaction type, and additional information stored in the sales_payment_transaction database table.
Table Of Content
Fetching Payment Transaction Details in Magento 2
To retrieve payment transaction details in Magento 2, use the TransactionRepositoryInterface. This interface allows you to access transaction data such as order ID, transaction ID, payment ID, transaction type, and additional information stored in the sales_payment_transaction table.
Here's a straightforward example:
namespace Vendor\Module\Model;
use Psr\Log\LoggerInterface;
use Magento\Framework\Exception\NoSuchEntityException;
use Magento\Sales\Api\TransactionRepositoryInterface;
class TransactionInfo
{
private $logger;
private $transactionRepository;
/**
* Constructor
*
* @param TransactionRepositoryInterface $transactionRepository
* @param LoggerInterface $logger
*/
public function __construct(
TransactionRepositoryInterface $transactionRepository,
LoggerInterface $logger
) {
$this->transactionRepository = $transactionRepository;
$this->logger = $logger;
}
/**
* Get Transaction by ID
*
* @param int $transactionId
* @return \Magento\Sales\Api\Data\TransactionInterface|null
*/
public function getTransactionById(int $transactionId)
{
try {
return $this->transactionRepository->get($transactionId);
} catch (NoSuchEntityException $exception) {
$this->logger->error(__('Transaction not found: %1', $exception->getMessage()));
return null;
} catch (\Exception $exception) {
$this->logger->critical(__('An error occurred while retrieving the transaction: %1', $exception->getMessage()));
return null;
}
}
}
To use this method:
$transactionId = 1;
$transactionInfo = $this->getTransactionById($transactionId);
if ($transactionInfo) {
echo 'Transaction ID: ' . $transactionInfo->getTxnId();
echo 'Parent Transaction ID: ' . $transactionInfo->getParentTxnId();
echo 'Transaction Type: ' . $transactionInfo->getTxnType();
echo 'Order ID: ' . $transactionInfo->getOrderId();
}
This approach ensures you can efficiently access and display transaction details in Magento 2.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What Does Fetching Payment Transaction Details in Magento 2 Mean?
Fetching payment transaction details in Magento 2 allows you to retrieve information such as transaction ID, order ID, payment ID, and transaction type. This is crucial for managing and debugging payment data in the system.
How Can I Fetch Payment Transaction Details Programmatically in Magento 2?
You can fetch payment transaction details using the TransactionRepositoryInterface
. By calling the get()
method and providing a transaction ID, you can retrieve the associated transaction data.
What Is the Code for Fetching Payment Transaction Details in Magento 2?
Here’s an example of code to fetch transaction details using the TransactionRepositoryInterface
:
namespace Vendor\Module\Model;
use Magento\Sales\Api\TransactionRepositoryInterface;
use Psr\Log\LoggerInterface;
class TransactionInfo
{
private $transactionRepository;
private $logger;
public function __construct(
TransactionRepositoryInterface $transactionRepository,
LoggerInterface $logger
) {
$this->transactionRepository = $transactionRepository;
$this->logger = $logger;
}
public function getTransactionById(int $transactionId)
{
try {
return $this->transactionRepository->get($transactionId);
} catch (\Exception $e) {
$this->logger->error('Error fetching transaction: ' . $e->getMessage());
return null;
}
}
}
// Usage
$transactionId = 1;
$transactionInfo = $this->getTransactionById($transactionId);
if ($transactionInfo) {
echo 'Transaction ID: ' . $transactionInfo->getTxnId();
echo 'Order ID: ' . $transactionInfo->getOrderId();
}
How Do I Fetch Transaction Details for Specific Payment Methods?
To fetch transaction details for specific payment methods, you can use the same TransactionRepositoryInterface
and filter by transaction type or payment ID based on your requirements.
Why Is Fetching Payment Transaction Details Important in Magento 2?
Fetching payment transaction details ensures you have accurate data for order processing, debugging, and resolving disputes. It also helps maintain consistency across payment records and system logs.
How Can I Handle Errors When Fetching Payment Transactions?
If an error occurs, ensure that the transaction ID exists and that the TransactionRepositoryInterface
is correctly injected into your class. Using a LoggerInterface
can help track and debug issues efficiently.
What Should I Do If a Transaction ID Is Not Found?
If a transaction ID is not found, verify that the ID is valid and exists in the database. If the issue persists, check the transaction table (`sales_payment_transaction`) and ensure the database integrity for missing records.
Can I Delete Payment Transactions in Magento 2?
Yes, you can delete payment transactions programmatically by using the repository’s delete method. However, be cautious when deleting transactions, as this can affect order history and payment records.