Programmatically Adding Order Comments in Magento 2
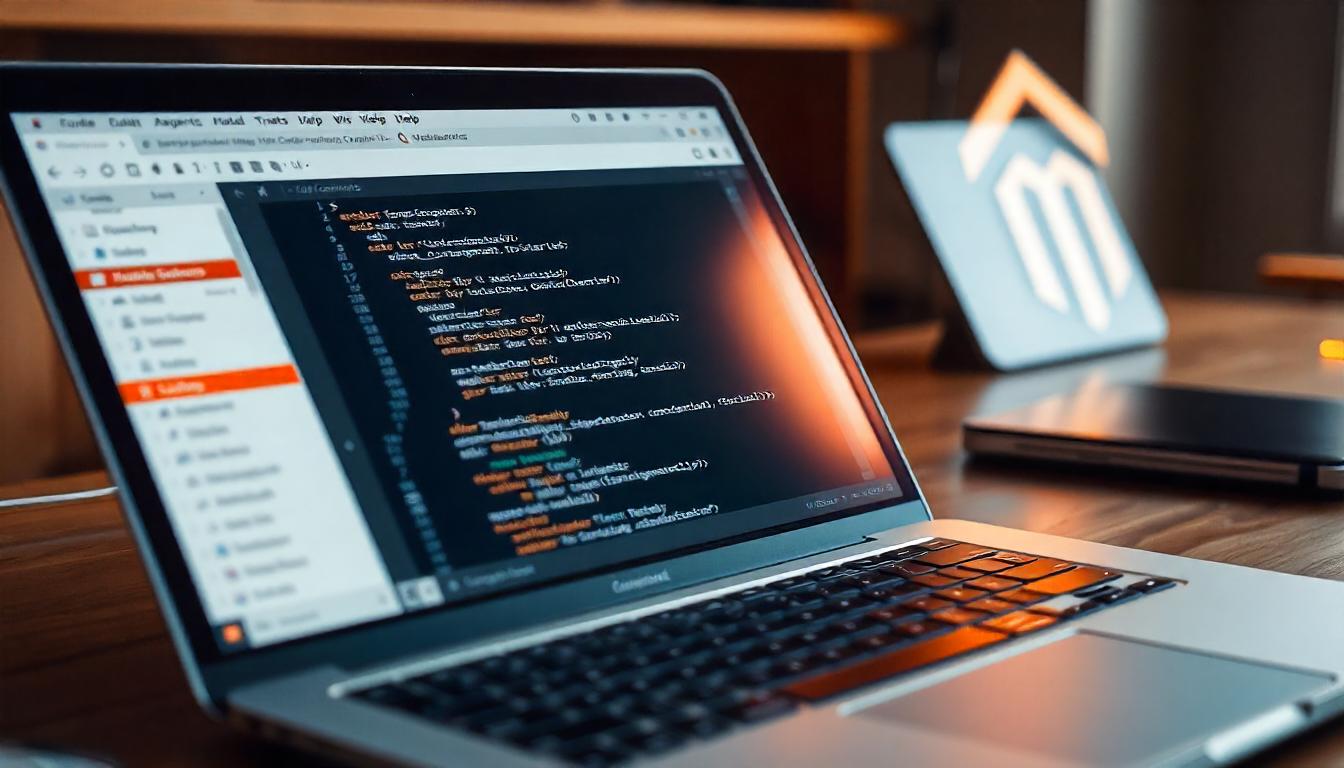
Programmatically Adding Order Comments in Magento 2
Adding order comments programmatically in Magento 2 helps store owners track order updates efficiently. Using the OrderRepositoryInterface and OrderStatusHistoryRepositoryInterface, you can retrieve an order, add a status history comment, and save it.
Table Of Content
Programmatically Adding Order Comments in Magento 2
To add a comment to an order in Magento 2 programmatically, follow these steps:
- Retrieve the Order: Use the OrderRepositoryInterface to load the order by its ID.
- Add the Comment: Utilize the addCommentToStatusHistory method to append your comment.
- Save the Comment: Employ the OrderStatusHistoryRepositoryInterface to save the comment.
Here's a concise code example:
namespace Vendor\Module\Model;
use Magento\Sales\Api\OrderRepositoryInterface;
use Magento\Sales\Api\OrderStatusHistoryRepositoryInterface;
use Psr\Log\LoggerInterface;
class OrderComment
{
private $orderRepository;
private $orderStatusHistoryRepository;
private $logger;
public function __construct(
OrderRepositoryInterface $orderRepository,
OrderStatusHistoryRepositoryInterface $orderStatusHistoryRepository,
LoggerInterface $logger
) {
$this->orderRepository = $orderRepository;
$this->orderStatusHistoryRepository = $orderStatusHistoryRepository;
$this->logger = $logger;
}
public function addCommentToOrder(int $orderId, string $comment, string $status = null)
{
try {
$order = $this->orderRepository->get($orderId);
$history = $order->addStatusHistoryComment($comment, $status);
$this->orderRepository->save($order);
} catch (\Exception $e) {
$this->logger->error('Error adding comment to order: ' . $e->getMessage());
}
}
}
Usage Example:
$orderId = 123;
$comment = 'Order processed successfully.';
$status = 'processing'; // Optional: specify the order status
$orderComment = $objectManager->create(\Vendor\Module\Model\OrderComment::class);
$orderComment->addCommentToOrder($orderId, $comment, $status);
Key Points:
- Order Retrieval: The
OrderRepositoryInterface
is used to fetch the order by its ID. - Adding Comments: The
addCommentToStatusHistory
method appends the comment to the order's history. You can optionally specify the order status. - Saving Comments: The
OrderStatusHistoryRepositoryInterface
ensures the comment is saved to the database.
This approach allows you to programmatically add comments to orders in Magento 2, enhancing order management and communication.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
How Can I Add an Order Status Comment Programmatically in Magento 2?
You can add an order status comment programmatically using the addCommentToStatusHistory
method. This allows you to append a custom message to an order's history.
Which Magento 2 Interface Is Used to Add Order Comments?
The OrderStatusHistoryRepositoryInterface
is used to save order status history comments programmatically. You also need the OrderRepositoryInterface
to retrieve the order object.
What Is the Code to Add an Order Status Comment in Magento 2?
Here’s a PHP snippet to add an order status comment:
namespace Vendor\Module\Model;
use Magento\Sales\Api\OrderRepositoryInterface;
use Magento\Sales\Api\OrderStatusHistoryRepositoryInterface;
use Psr\Log\LoggerInterface;
class OrderComment
{
private $orderRepository;
private $orderStatusHistoryRepository;
private $logger;
public function __construct(
OrderRepositoryInterface $orderRepository,
OrderStatusHistoryRepositoryInterface $orderStatusHistoryRepository,
LoggerInterface $logger
) {
$this->orderRepository = $orderRepository;
$this->orderStatusHistoryRepository = $orderStatusHistoryRepository;
$this->logger = $logger;
}
public function addCommentToOrder(int $orderId, string $comment, string $status = null)
{
try {
$order = $this->orderRepository->get($orderId);
$history = $order->addCommentToStatusHistory($comment, $status);
$this->orderStatusHistoryRepository->save($history);
} catch (\Exception $e) {
$this->logger->error('Error adding comment to order: ' . $e->getMessage());
}
}
}
How Do I Specify an Order Status When Adding a Comment?
You can pass the order status as a second parameter when calling addCommentToStatusHistory
. For example:
$order->addCommentToStatusHistory('Order updated.', 'processing');
Can I Add an Order Comment Without Changing the Status?
Yes, the status parameter is optional. If omitted, the comment is added without affecting the order status.
How Do I Handle Errors When Adding an Order Comment?
Wrap the code inside a try-catch
block to handle exceptions. If an error occurs, log it using LoggerInterface
to debug the issue.
Where Are Order Comments Stored in Magento 2?
Order comments are stored in the sales_order_status_history
table in the Magento database.
How Can I View Added Order Comments?
Order comments can be viewed in the Magento Admin Panel under Sales > Orders > Order View. They are listed in the order history section.
Can I Modify an Existing Order Comment?
No, once an order comment is saved, Magento does not provide a direct way to edit it. However, you can add a new comment to provide updated information.
How Do I Delete an Order Status Comment?
Magento does not provide a built-in way to delete order status comments from the admin panel. However, you can remove them manually from the database or create a custom module to delete them programmatically.