How to Remove a Specific Order Status Comment in Magento 2++
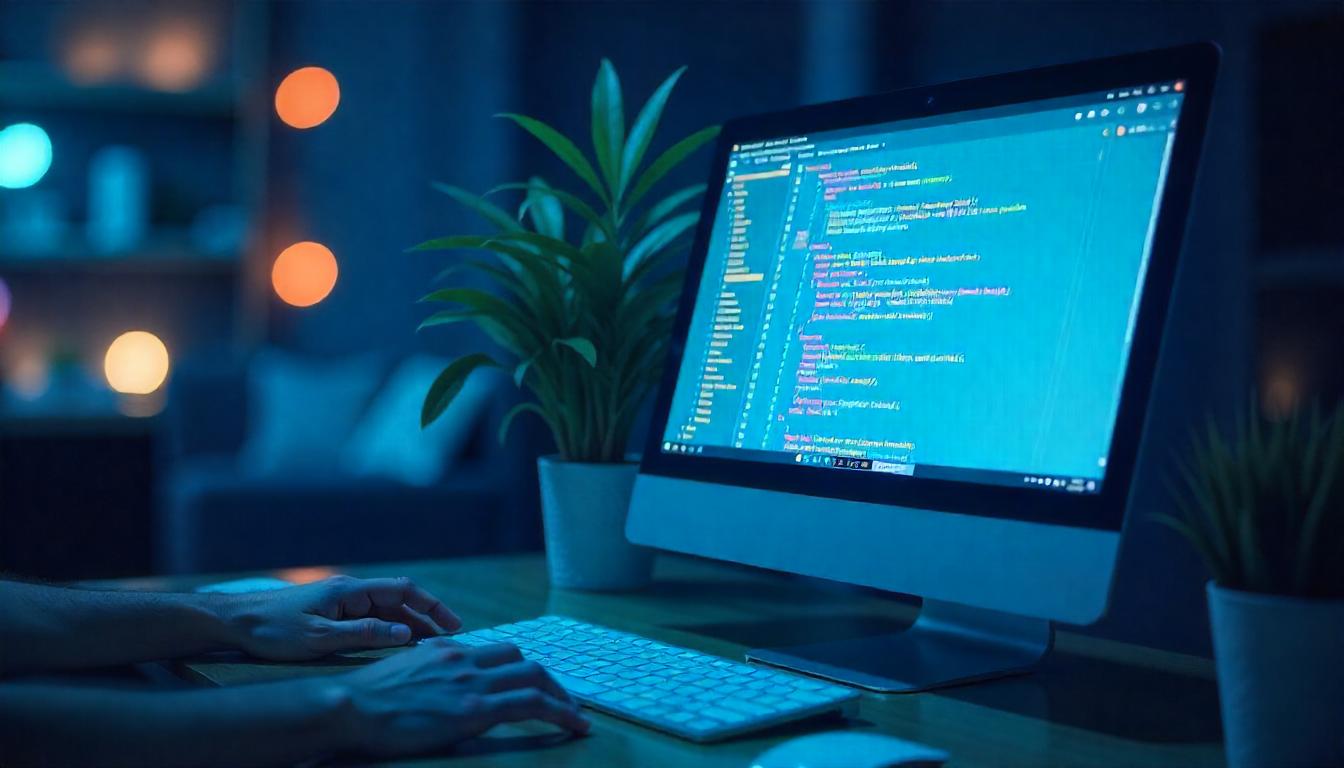
How to Remove a Specific Order Status Comment in Magento 2++
Removing unnecessary order status comments in Magento 2 helps maintain clean and organized order histories. Whether you're cleaning up old comments or correcting errors, Magento provides multiple ways to delete specific order status comments.
Table Of Content
How to Remove a Specific Order Status Comment in Magento 2++
In Magento 2, order status comments help track order progress. Sometimes, you might need to delete a specific comment to keep the order history clean. Here's how to do it:
Using a Custom PHP Script
- Create the Script: In your Magento root directory, create a file named
DeleteOrderStatusComment.php.
- Add the Code: Insert the following code into the file:
- Run the Script: Execute this script from the command line or through a browser to remove the specified comment.
<?php
use Magento\Framework\App\Bootstrap;
require __DIR__ . '/app/bootstrap.php';
$bootstrap = Bootstrap::create(BP, $_SERVER);
$objectManager = $bootstrap->getObjectManager();
$state = $objectManager->get('Magento\Framework\App\State');
$state->setAreaCode('frontend');
try {
$orderID = 71; // Replace with the desired order ID
$commentID = 22; // Replace with the desired comment ID
$order = $objectManager->create('Magento\Sales\Model\Order')->load($orderID);
$commentsCollection = $order->getStatusHistoryCollection();
foreach ($commentsCollection as $comment) {
if ($comment->getId() == $commentID) {
$comment->delete();
break;
}
}
$order->save();
echo 'Order status comment deleted successfully!';
} catch (\Exception $e) {
echo "Error: " . $e->getMessage();
}
Using Magento's API
Alternatively, you can use Magento's API to delete a comment:
- Load the Comment: Retrieve the comment by its ID.
- Delete the Comment: Use the delete method from the
OrderStatusHistoryRepositoryInterface
to remove the comment.. - Call the Method: Invoke the removeStatusFromOrder method with the comment ID to delete the comment.
namespace YourNamespace\DeleteStatusComment\Model;
use Magento\Sales\Api\OrderStatusHistoryRepositoryInterface;
use Psr\Log\LoggerInterface;
class DeleteStatusComment
{
private $orderStatusRepository;
private $logger;
public function __construct(
OrderStatusHistoryRepositoryInterface $orderStatusRepository,
LoggerInterface $logger
) {
$this->orderStatusRepository = $orderStatusRepository;
$this->logger = $logger;
}
public function removeStatusFromOrder(int $commentId)
{
try {
$orderStatusCommentObject = $this->orderStatusRepository->get($commentId);
$this->orderStatusRepository->delete($orderStatusCommentObject);
return true;
} catch (\Exception $exception) {
$this->logger->error($exception->getMessage());
return false;
}
}
}
'; ?>
By following these steps, you can effectively remove specific order status comments in Magento 2, keeping your order history organized.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What Is the Purpose of Removing Order Status Comments in Magento 2?
Removing order status comments helps keep the order history clean and relevant. It’s useful for eliminating outdated or unnecessary comments that don’t add value to the order’s progress.
Why Should I Remove Order Status Comments in Magento 2?
Managing order status comments is important for maintaining a clear and organized order history. Removing irrelevant comments ensures that customers and admin staff can focus on the most important updates.
How Can I Delete Specific Order Status Comments Programmatically in Magento 2?
In Magento 2, you can delete order status comments using the OrderStatusHistoryRepositoryInterface
API. This allows you to load a specific comment by ID and delete it to keep the order status history clean.
What Is the Code for Deleting an Order Status Comment in Magento 2?
Here’s an example of how to delete a specific order status comment programmatically:
namespace YourNamespace\DeleteOrderStatusComment\Model;
use Magento\Sales\Api\OrderStatusHistoryRepositoryInterface;
use Magento\Sales\Api\Data\OrderStatusHistoryInterface;
use Psr\Log\LoggerInterface;
class DeleteStatusComment
{
private $orderStatusRepository;
private $logger;
public function __construct(
OrderStatusHistoryRepositoryInterface $orderStatusRepository,
LoggerInterface $logger
) {
$this->orderStatusRepository = $orderStatusRepository;
$this->logger = $logger;
}
public function removeStatusFromOrder(int $commentId)
{
try {
$orderStatusCommentObject = $this->orderStatusRepository->get($commentId);
$this->orderStatusRepository->delete($orderStatusCommentObject);
return true;
} catch (\Exception $exception) {
$this->logger->error($exception->getMessage());
return false;
}
}
}
How Do I Remove an Order Status Comment Using an API in Magento 2?
To remove an order status comment using Magento’s API, load the comment by ID, and then use the delete
method from the OrderStatusHistoryRepositoryInterface
to remove it.
Can I Prevent Order Status Comments from Being Created in Magento 2?
While it’s not possible to fully prevent order status comments from being created, you can implement filters and validation to ensure only relevant comments are added in the first place.
How Can I Troubleshoot Issues When Deleting Order Status Comments in Magento 2?
If you encounter issues while deleting order status comments, check the Magento logs for errors. Ensure the comment ID is valid and the API is properly configured. Also, verify that you have the correct permissions to delete the comment.
What Should I Do If I Cannot Delete an Order Status Comment?
If you’re unable to delete an order status comment, ensure that the comment exists and hasn’t been archived. You may also need to review permissions, or check for any system issues or conflicts that could be affecting the deletion process.
How Can I Delete Multiple Order Status Comments in Magento 2?
To delete multiple order status comments, you can loop through the comment IDs and delete each one using a similar approach as deleting a single comment. This can be done programmatically by iterating through a collection of comments.