Extracting Entity Link Field Values in Magento 2
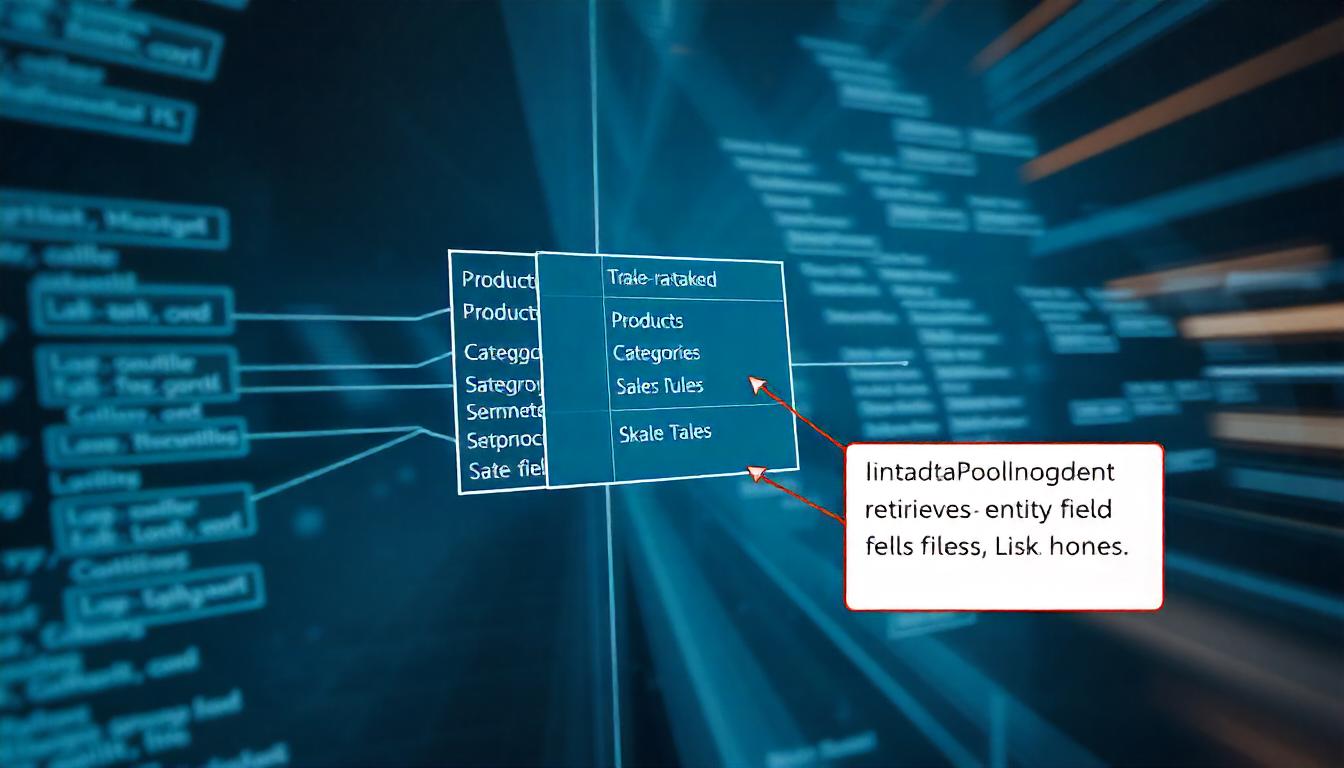
Extracting Entity Link Field Values in Magento 2
When working with Magento 2, it's essential to understand how to extract entity link field values, especially when you need to join database tables programmatically. The link field is used in place of the primary key (entity_id) to establish relationships between entities, such as products, categories, and sales rules.
Table Of Content
Extracting Entity Link Field Values in Magento 2
In Magento 2, retrieving the entity type's link field value programmatically is essential for tasks like joining database queries. Magento leverages the MetadataPool class to achieve this using the $metadata->getLinkField() method. This approach ensures seamless integration with various entity types.
When working with Magento 2, you often need the link field value instead of the primary key (entity_id) to correctly link tables in your queries. Let’s dive into the implementation.
Using MetadataPool to Retrieve Link Field Values
The MetadataPool class fetches metadata for a specific entity type. Here's a concise implementation:
namespace Jesadiya\LinkField\Model;
use Magento\Catalog\Api\Data\ProductInterface;
use Magento\Framework\EntityManager\MetadataPool;
class GetLinkField
{
/**
* @var MetadataPool
*/
private $metadataPool;
/**
* Constructor
*
* @param MetadataPool $metadataPool
*/
public function __construct(MetadataPool $metadataPool)
{
$this->metadataPool = $metadataPool;
}
/**
* Retrieve link field for the entity type.
*
* @return string|null
* @throws \Magento\Framework\Exception\LocalizedException
*/
public function getLinkField()
{
$metadata = $this->metadataPool->getMetadata(ProductInterface::class);
return $metadata ? $metadata->getLinkField() : null;
}
}
// Example usage
$objectManager = \Magento\Framework\App\ObjectManager::getInstance();
$metadataPool = $objectManager->get(MetadataPool::class);
$getLinkField = new GetLinkField($metadataPool);
echo $linkFieldValue = $getLinkField->getLinkField();
Steps to Retrieve a Link Field for Other Entity Types
To fetch the link field for other entity types, pass the respective entity interface to the getMetadata() method. Examples:
Entity Type | Interface | Example Link Field |
---|---|---|
Product | Magento\Catalog\Api\Data\ProductInterface |
row_id |
Category | Magento\Catalog\Api\Data\CategoryInterface |
row_id |
Sales Rule | Magento\SalesRule\Api\Data\RuleInterface |
rule_id |
Example code for Sales Rules:
$this->metadataPool->getMetadata(\Magento\SalesRule\Api\Data\RuleInterface::class)->getLinkField();
Important Notes
- Content Staging: The row_id link field is critical for Content Staging functionality in Magento 2 Commerce.
- Avoid Errors: Always use the link field instead of entity_id when dealing with multi-version tables like catalog_product_entity.
By following this method, you can ensure proper handling of database queries, especially in scenarios requiring Content Staging or versioned entities.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What Does Extracting Entity Link Field Values in Magento 2 Mean?
Extracting entity link field values in Magento 2 allows you to retrieve specific link field data for various entity types. This is essential when joining database queries or working with multi-versioned tables, ensuring seamless data relationships between tables.
How Can I Extract the Entity Link Field Value Programmatically in Magento 2?
You can extract the entity link field value using the MetadataPool
class. The getMetadata
method accepts an entity type interface and returns the associated link field for that entity.
What Is the Code for Extracting Entity Link Field Values in Magento 2?
Here’s an example code for extracting the entity link field value for a product entity:
namespace Jesadiya\LinkField\Model;
use Magento\Catalog\Api\Data\ProductInterface;
use Magento\Framework\EntityManager\MetadataPool;
class GetLinkField
{
private $metadataPool;
public function __construct(MetadataPool $metadataPool)
{
$this->metadataPool = $metadataPool;
}
public function getLinkField()
{
return $this->metadataPool
->getMetadata(ProductInterface::class)
->getLinkField();
}
}
// Usage
echo $linkFieldValue = $this->getLinkField();
How Do I Extract Link Field Values for Other Entity Types?
To extract link field values for other entities like categories or sales rules, pass the respective entity interface to the getMetadata
method. Here’s an example for a sales rule:
$this->metadataPool->getMetadata(\Magento\SalesRule\Api\Data\RuleInterface::class)->getLinkField();
What Link Field Is Used for Content Staging in Magento 2 Commerce?
The row_id
field is used as the link field for Content Staging functionality in Magento 2 Commerce. It helps connect different versions of entities in the staging environment.
Why Is It Important to Retrieve the Link Field Value in Magento 2?
Retrieving the link field value ensures that you can join tables correctly when performing database queries. It’s especially important when working with multi-versioned entities or integrating with features like Content Staging, as using the link field ensures data consistency.
How Can I Ensure Accuracy When Retrieving Link Field Values?
To ensure accuracy, always make sure:
- The correct entity interface is passed to
getMetadata
. - The link field is correctly defined in the metadata for each entity type.
- You’re using the correct class for each entity type (e.g.,
ProductInterface
for products).
What Should I Do If an Error Occurs When Retrieving Link Field Values?
If an error occurs, ensure the entity interface is correct and that the metadata is properly injected into your class. Logging errors using a LoggerInterface
can help identify the problem for easier debugging.