Reindexing Product Prices by IDs in Magento 2
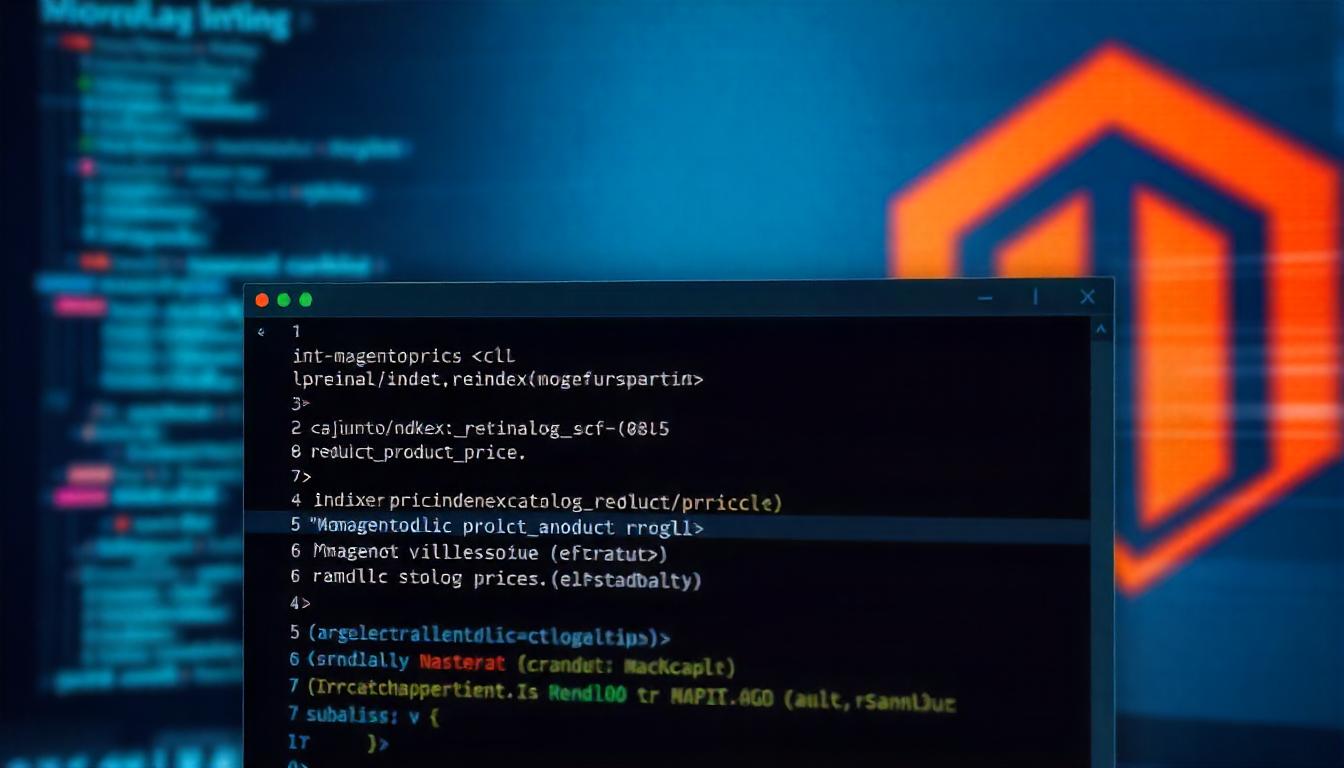
Reindexing Product Prices by IDs in Magento 2
Reindexing product prices by IDs in Magento 2 allows you to update price data for specific products without reindexing the entire catalog. This targeted approach improves performance and ensures price changes reflect accurately on the frontend. You can achieve this programmatically using the catalog_product_price indexer.
Table Of Content
Reindexing Product Prices by IDs in Magento 2
To reindex product prices by their IDs in Magento 2, you can use the following approach:
- Create a Helper Class: This class will handle the reindexing process.
<?php
namespace YourNamespace\YourModule\Helper;
use Magento\Indexer\Model\IndexerFactory;
use Magento\Indexer\Model\Indexer\CollectionFactory;
class Data extends \Magento\Framework\App\Helper\AbstractHelper
{
protected $indexerFactory;
protected $indexerCollectionFactory;
public function __construct(
\Magento\Framework\App\Helper\Context $context,
IndexerFactory $indexerFactory,
CollectionFactory $indexerCollectionFactory
) {
parent::__construct($context);
$this->indexerFactory = $indexerFactory;
$this->indexerCollectionFactory = $indexerCollectionFactory;
}
public function reindexPrice(array $productIds)
{
$indexer = $this->indexerFactory->create()->load('catalog_product_price');
$indexer->reindexList($productIds);
}
}
- Call the Method with Product IDs: Invoke the reindexPrice method with the specific product IDs you want to reindex.
$productIds = [1, 2, 3];
$helper = $this->_objectManager->get('YourNamespace\YourModule\Helper\Data');
$helper->reindexPrice($productIds);
This method ensures that only the prices for the specified products are reindexed, improving performance by avoiding a full reindex.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What Does Reindexing Product Prices by IDs Mean in Magento 2?
Reindexing product prices by IDs in Magento 2 allows you to update and apply price changes to specific products without reindexing all product data. It improves efficiency by targeting only the required products.
How Can I Programmatically Reindex Product Prices by IDs in Magento 2?
You can programmatically reindex product prices by creating a custom class and using the catalog_product_price
indexer. This involves calling the reindexList
method with the desired product IDs.
What Is the Command-Line Method for Reindexing Prices?
The command-line method for reindexing prices involves using the following command:
php bin/magento indexer:reindex catalog_product_price
This updates the prices for all products in the catalog.
How Do I Create a Custom Class for Reindexing Prices by IDs?
To create a custom class for reindexing prices by IDs, you can follow these steps:
- Define a custom helper or model class in your module.
- Inject the
Price\Processor
class for thecatalog_product_price
indexer. - Call the
reindexList
method with an array of product IDs.
What Is an Example of Code for Reindexing Prices?
Here is an example:
namespace YourNamespace\YourModule\Model;
use Magento\Catalog\Model\Indexer\Product\Price\Processor;
class PriceIndexer
{
public function __construct(private readonly Processor $priceProcessor) {}
public function reindexPrice(array $productIds): void
{
$this->priceProcessor->reindexList($productIds);
}
}
What Challenges Might You Face When Reindexing Prices?
Some challenges include:
- Ensuring proper file and directory structure for custom code.
- Handling cache issues that might delay updates.
- Verifying the indexer status to avoid incomplete reindexing.
Why Is It Important to Reindex Prices Efficiently?
Efficient price reindexing ensures that customers see accurate prices promptly. It minimizes system resource usage and enhances the user experience by avoiding delays in updates.
How Can You Test Price Reindexing Customizations?
You can test price reindexing by:
- Running the custom code with test product IDs.
- Verifying the updated prices in the frontend and backend.
- Monitoring system logs for any errors during the reindexing process.