New Title: Verifying Admin User Login in Magento 2: A Practical Guide
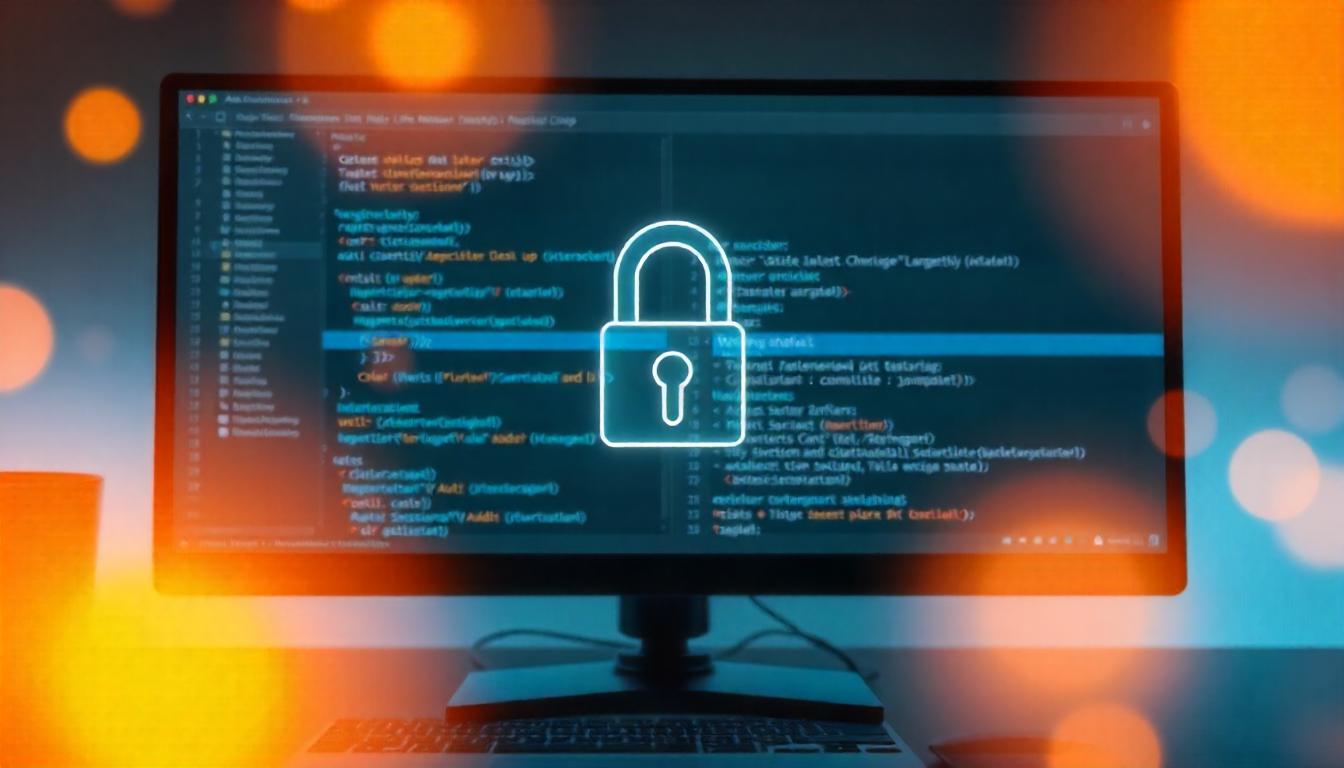
New Title: Verifying Admin User Login in Magento 2: A Practical Guide
Learn how to verify if an admin user is logged into Magento 2 using the Backend Session class. This guide provides a practical, step-by-step approach with clear explanations and a working code example. Understand why verifying admin login is essential for secure access and custom functionality. Whether you're managing user authentication or building advanced features, this guide equips you with the tools to ensure seamless admin verification in your Magento 2 projects.
New Title: Verifying Admin User Login in Magento 2: A Practical Guide
Introduction
Checking if an admin user is logged in Magento 2 is straightforward with the Backend Session class. This ensures secure access and helps you build custom functionalities based on user authentication. Below, we’ll walk you through the process step-by-step.
Why It Matters
Knowing the admin login status is crucial for tasks like restricting unauthorized actions, personalizing dashboards, or implementing advanced controls. This process uses the Magento\Backend\Model\Auth\Session class to handle authentication.
Updated Code Example
Here’s how to create a method to verify admin user login:
namespace Vendor\Module\Model;
use Magento\Backend\Model\Auth\Session;
class AdminLoginCheck
{
/**
* @var Session
*/
private $backendSession;
public function __construct(
Session $backendSession
) {
$this->backendSession = $backendSession;
}
/**
* Check if admin user is logged in
*
* @return bool
*/
public function isAdminLoggedIn(): bool
{
$user = $this->backendSession->getUser();
return $user && $user->getId() !== null;
}
}
Explanation
- Dependency Injection: The Session class is injected into the constructor.
- Method Logic: The isAdminLoggedIn() method checks if a user exists and retrieves their ID.
- Output: Returns true if logged in, false otherwise.
Implementation Use Case
You can use this class wherever you need to verify admin login, such as in controllers or custom modules.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What Does the Admin Login Check Do in Magento 2?
The admin login check in Magento 2 verifies whether an admin user is currently authenticated, returning a boolean value for the login status.
How Can I Programmatically Check If an Admin User Is Logged In?
You can programmatically verify the admin login status using the Magento\Backend\Model\Auth\Session class. Implement a method to check the login status as shown in this example:
namespace Vendor\Module\Model;
use Magento\Backend\Model\Auth\Session;
class AdminLoginCheck
{
private $backendSession;
public function __construct(Session $backendSession)
{
$this->backendSession = $backendSession;
}
public function isAdminLoggedIn(): bool
{
$user = $this->backendSession->getUser();
return $user && $user->getId() !== null;
}
}
What Dependencies Are Required for Checking Admin Login?
You need to inject the Magento\Backend\Model\Auth\Session class using dependency injection in your constructor to access admin session data.
How Can I Use the Admin Login Check in Magento 2?
The admin login check can be integrated into controllers, custom modules, or helper classes to restrict access or implement custom logic based on login status.
What Issues May Arise When Checking Admin Login?
Potential issues include:
- Missing or incorrect dependency injection configuration.
- Customizations that override default session behavior.
- Third-party module conflicts affecting the session class.
Why Is Verifying Admin Login Important?
It ensures secure access by validating that only authenticated admin users can perform sensitive actions. It’s also useful for customizing admin experiences and workflows.
How Can I Test the Admin Login Check?
Create a test script or integrate the method into a controller, then log in to the admin panel and call the method. Verify that it returns the expected boolean value.