Retrieving Request Parameters in Magento 2 REST API
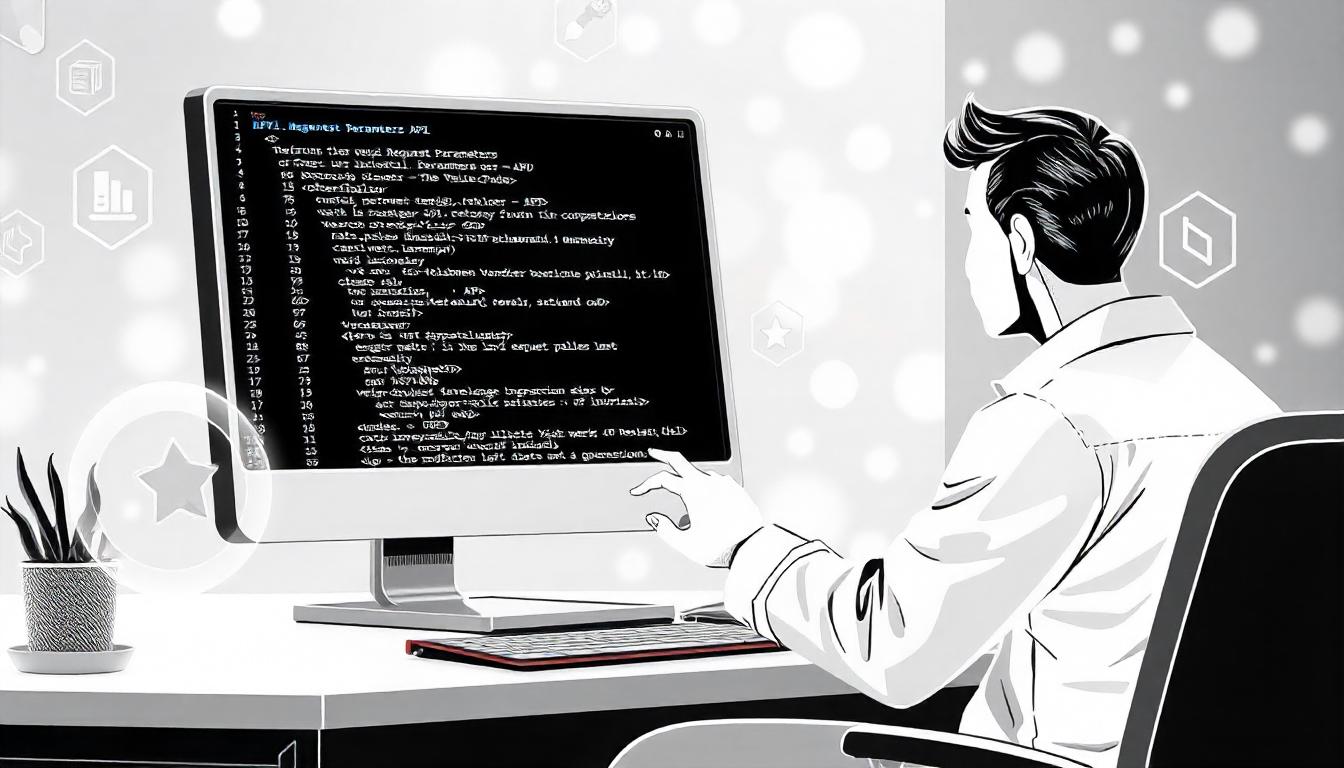
Retrieving Request Parameters in Magento 2 REST API
Learn how to efficiently access and manage request parameters when working with Magento 2's REST API. This guide explains how to retrieve specific parameters, fetch all parameters, and extract data from the HTTP request body using the Magento\Framework\Webapi\Rest\Request class.
Table Of Content
Retrieving Request Parameters in Magento 2 REST API
When working with Magento 2's REST API, it's essential to access request parameters efficiently. Magento provides the Magento\Framework\Webapi\Rest\Request class to facilitate this.
Accessing Specific Request Parameters
To retrieve a particular parameter from a REST API request, utilize the getParam method:
<?php
namespace Vendor\Module\Model;
use Magento\Framework\Webapi\Rest\Request;
class GetRestParam
{
protected $request;
public function __construct(Request $request)
{
$this->request = $request;
}
public function getParamValue()
{
$parameterValue = $this->request->getParam('parameter_name');
return $parameterValue;
}
}
Replace 'parameter_name' with the actual name of the parameter you intend to fetch.
Retrieving All Request Parameters
To obtain all parameters from a REST API request, use the getParams method:
$allParams = $this->request->getParams();
This method returns an associative array containing all key-value pairs from the request.
Fetching Data from the HTTP Request Body
For data sent in the HTTP request body, especially in POST requests, use the getBodyParams method:
Common Scenarios for Cache Invalidation
$bodyParams = $this->request->getBodyParams();
This retrieves all parameters present in the request body as an associative array.
Summary of Methods
Method | Description |
---|---|
getParam('param_name') |
Retrieves the value of a specific parameter. |
getParams() |
Retrieves all request parameters as an array. |
getBodyParams() |
Retrieves parameters from the request body. |
By effectively using these methods, you can manage request parameters in Magento 2's REST API with ease.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What Are Request Parameters in Magento 2 REST API?
Request parameters in Magento 2 REST API are the data sent by the client to the server during an API call. These parameters allow specific information to be retrieved or actions to be performed based on the request.
How Do You Retrieve a Specific Request Parameter?
You can retrieve a specific request parameter using the getParam() method from the Magento\Framework\Webapi\Rest\Request class. This method fetches the value of a specified parameter.
Example Code:
request = $request;
}
public function getParamValue()
{
return $this->request->getParam('parameter_name');
}
}
How Can You Retrieve All Request Parameters?
To retrieve all request parameters, use the getParams() method. This returns an associative array of all key-value pairs from the request.
Example Code:
$allParams = $this->request->getParams();
How Do You Access Data from the HTTP Request Body?
Use the getBodyParams() method to access data sent in the HTTP request body. This is particularly useful for POST requests.
Example Code:
$bodyParams = $this->request->getBodyParams();
Why Is Fetching Request Parameters Important?
Fetching request parameters is essential for building dynamic APIs. It allows you to process client-specific data and deliver personalized responses efficiently.
What Issues Should You Look Out for When Handling Request Parameters?
Common issues include:
- Ensuring the correct parameter names are used.
- Handling cases where parameters are missing or null.
- Validating and sanitizing input to prevent security vulnerabilities.
How Can You Debug Request Parameter Issues?
If request parameters are not working as expected:
- Print getParams() or getBodyParams() output to verify the data received.
- Check API documentation to ensure the correct parameter structure.
- Review server logs for errors or unexpected behavior.
Does Fetching Parameters Impact API Performance?
Fetching parameters has minimal performance impact. Magento 2’s architecture ensures that parameter handling is efficient and optimized for scalability.