Understanding Magento 2 Indexer Modes: Checking if Scheduled Updates Are Enabled
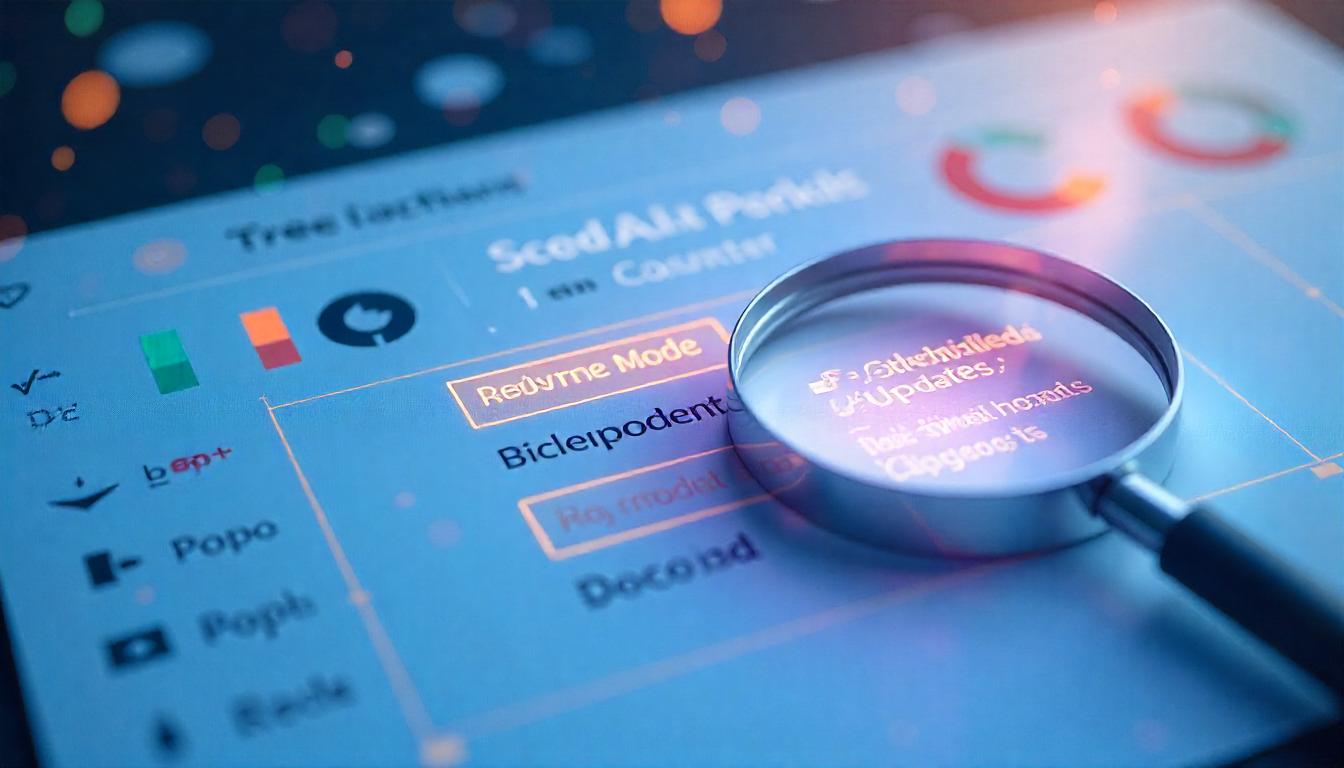
Understanding Magento 2 Indexer Modes: Checking if Scheduled Updates Are Enabled
In Magento 2, indexers play a crucial role in ensuring your store's data is structured and displayed optimally for performance. They can operate in two modes: Update on Save and Scheduled Updates.
Understanding Magento 2 Indexer Modes: Checking if Scheduled Updates Are Enabled
In Magento 2, indexers manage how data is organized for efficient retrieval. There are two primary modes:
- Update on Save: Indexes update immediately after data changes.
- Update by Schedule: Indexes update at set intervals via cron jobs.
To determine if a specific indexer uses the "Update by Schedule" mode, you can check its configuration programmatically.
Steps to Check if an Indexer Uses Scheduled Updates
- Obtain the Indexer ID: Each indexer has a unique identifier. For example:
- Access the Indexer Registry: Magento's IndexerRegistry service allows interaction with indexers.
- Check the Indexer's Scheduled Status: Use the isScheduled() method to determine if the indexer operates in scheduled mode.
Sample Code Implementation
Here's how you can implement this check:
<?php
namespace YourNamespace\Indexer\Model;
use Magento\Framework\Indexer\IndexerRegistry;
class IndexerModeChecker
{
/**
* @var IndexerRegistry
*/
protected $indexerRegistry;
}
public function __construct(
IndexerRegistry $indexerRegistry
) {
$this->indexerRegistry = $indexerRegistry;
}
/**
* Check if the specified indexer uses scheduled updates.
*
* @param string $indexerId
* @return bool
*/
public function isIndexerScheduled($indexerId)
{
try {
$indexer = $this->indexerRegistry->get($indexerId);
return $indexer->isScheduled();
} catch (\InvalidArgumentException $e) {
// Handle exception if indexer ID is invalid
return false;
}
}
}
Usage Example
To check if the catalog_category_product indexer uses scheduled updates:
$indexerModeChecker = new \YourNamespace\Indexer\Model\IndexerModeChecker($indexerRegistry);
$isScheduled = $indexerModeChecker->isIndexerScheduled('catalog_category_product');
The $isScheduled variable will be true if the indexer uses scheduled updates, and false otherwise.
Additional Information
- Changing Indexer Modes: To switch an indexer to scheduled updates, use the following command:
- Listing All Indexers: To view all indexers and their current modes:
bin/magento indexer:set-mode schedule [indexer-name]
Replace [indexer-name] with the actual indexer ID.
bin/magento indexer:show-mode
This command displays each indexer's name and its mode.
By following these steps, you can programmatically determine if a specific indexer in Magento 2 is set to update by schedule.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What Are Indexer Modes in Magento 2?
Indexer modes in Magento 2 determine how and when the index is updated. There are two modes:
- Real-time (Update on Save): Automatically updates the index when changes are made.
- Scheduled (Update by Schedule): Updates the index through scheduled cron jobs.
How Can You Check If Scheduled Updates Are Enabled?
You can check the indexer mode using the Magento CLI. Run the following command:
php bin/magento indexer:status
This will display the current mode for each indexer.
What Are the Advantages of Using Scheduled Updates?
Scheduled updates provide the following benefits:
- Improved performance for large catalogs as updates are processed in batches.
- Reduced load on the server during real-time operations.
- Flexibility in scheduling updates during off-peak hours.
How Can You Switch to Scheduled Update Mode?
To switch an indexer to scheduled update mode, use the following CLI command:
php bin/magento indexer:set-mode schedule
This will set all indexers to update by schedule. To update a specific indexer, include its name:
php bin/magento indexer:set-mode schedule catalog_product_price
What Are Common Issues with Scheduled Updates?
Some common issues include:
- Missing or misconfigured cron jobs, preventing updates from running.
- Indexers stuck in
processing
status due to server errors. - Incorrect file or directory permissions affecting the cron job.
Regularly check the cron job logs and indexer status to ensure smooth operations.
How Can You Debug Indexer Mode Issues?
To debug issues with indexer modes:
- Verify cron jobs are set up correctly using the
cron:run
command. - Use
php bin/magento indexer:reindex
to manually reindex data and check for errors. - Inspect system and exception logs for detailed error messages.
How Does Indexer Mode Impact Store Performance?
The indexer mode directly impacts the performance and responsiveness of your Magento store:
- Real-time: Provides immediate updates but may slow down the admin and frontend during large data changes.
- Scheduled: Improves performance for high-traffic or large-scale stores by deferring updates to scheduled times.
What Are Best Practices for Managing Indexer Modes?
Follow these best practices for managing indexer modes:
- Use scheduled mode for large catalogs to avoid real-time performance issues.
- Ensure cron jobs are configured and tested regularly.
- Monitor indexer status frequently to avoid stuck processes.
- Reindex manually during development or significant data updates.