How the listens Property in Magento 2 UI Components Works
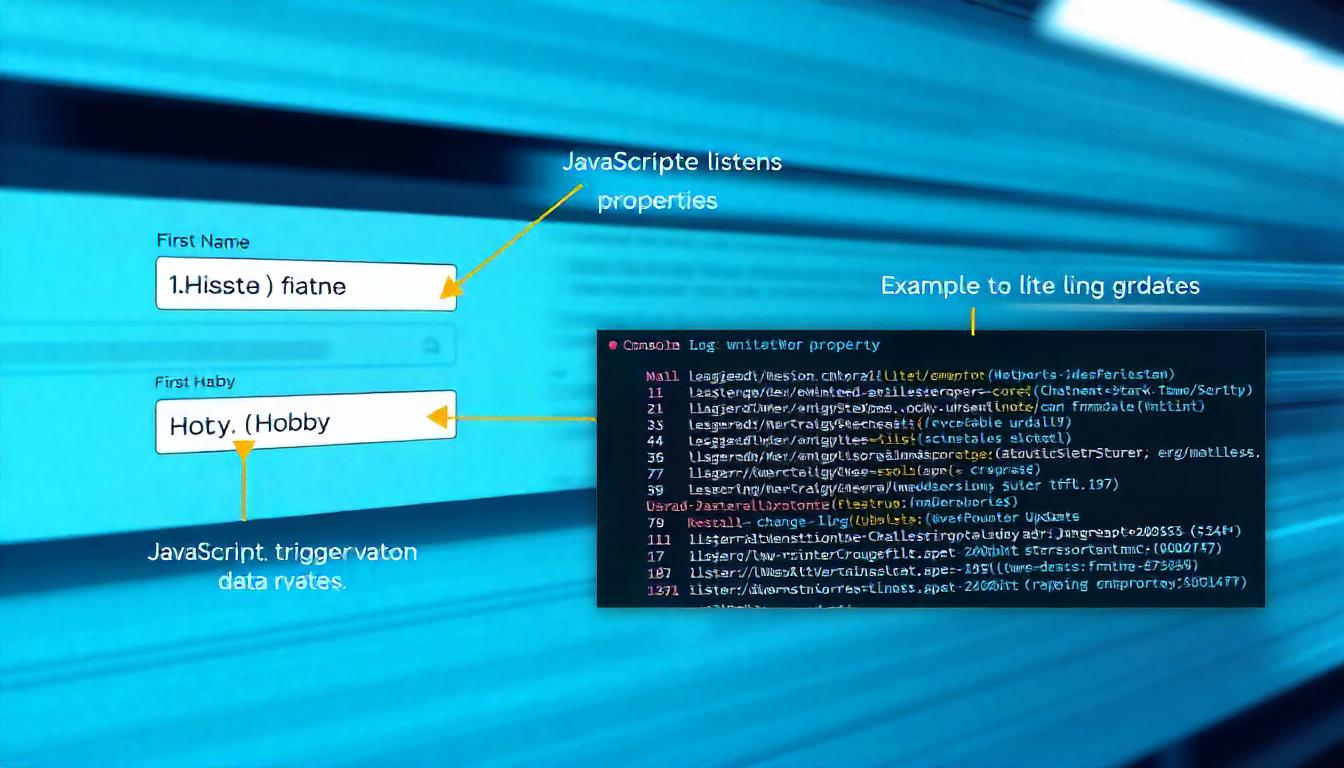
How the listens Property in Magento 2 UI Components Works
The listens property in Magento 2 UI Components is a mechanism for monitoring changes to observable properties. It triggers specified functions automatically when the associated properties are updated. This functionality enables developers to create interactive and dynamic user interfaces by responding to real-time data changes. Commonly used in forms and checkout pages, the listens property ensures seamless integration and responsive behavior in Magento applications.
Table Of Content
How the listens Property in Magento 2 UI Components Works
The listens property in Magento 2 UI Components allows developers to track changes to specific observable properties. When a property's value changes, the function linked to that property via the listens configuration is triggered. This functionality is particularly useful for creating dynamic, interactive components.
Key Points About the listens Property
- Tracks Observable Properties: The listens property works exclusively with observable fields.
- Event-Based Execution: Functions defined in listens are called automatically when the corresponding property's value changes.
- Flexible Use Cases: It can handle different events, such as real-time updates (textInput) or blur events (value).
Practical Example: Using listens
Below is an example of implementing the listens property in a custom Magento 2 module.
Template File
This .phtml file defines a UI Component with an input form bound to observable properties:
<script type="text/x-magento-init">
{
"*" : {
"Magento_Ui/js/core/app": {
"components": {
"listensExample": {
"component": "Custom_Module/js/listener-example"
}
}
}
}
}
</script>
<div data-bind="scope: 'listensExample'">
<label>First Name</label>
<input data-bind="textInput: firstname" />
<label>Hobby</label>
<input data-bind="value: hobby" />
</div>
- Scope Binding: The listensExample component is linked to the DOM using data-bind="scope".
- Input Binding: The firstname and hobby fields are tied to observables using Knockout.js bindings.
JavaScript File
This script defines the behavior of the listens property:
define(['uiComponent'], function (Component) {
'use strict';
return Component.extend({
defaults: {
firstname: 'Enter First Name',
hobby: 'Cricket',
listens: {
firstname: 'handleFirstnameChange',
hobby: 'handleHobbyChange'
}
},
handleFirstnameChange: function (value) {
console.log('First name updated:', value);
},
handleHobbyChange: function (value) {
console.log('Hobby updated:', value);
},
initObservable: function () {
this._super().observe(['firstname', 'hobby']);
return this;
}
});
});
- Dynamic Observables: The firstname and hobby properties are declared as observables using initObservable.
- Listeners: The handleFirstnameChange and handleHobbyChange methods execute when their respective properties are updated.
Example from Magento Core
In Magento’s guest checkout, the listens property is used to validate email fields. When users update their email address, the listens property triggers a function to check if the email is already registered.Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What Is the `listens` Property in Magento 2 UI Components?
The `listens` property in Magento 2 UI Components tracks changes in observable properties. It allows developers to specify methods that should be triggered whenever a specific property changes.
How Does the `listens` Property Work with Observables?
The `listens` property works by associating specific properties with functions. These functions are executed whenever the corresponding observable property changes. For example:
define(['uiComponent'], function (Component) {
'use strict';
return Component.extend({
defaults: {
firstname: 'Default Name',
hobby: 'Reading',
listens: {
firstname: 'updateFirstname',
hobby: 'updateHobby'
}
},
updateFirstname: function (value) {
console.log('First Name Updated:', value);
},
updateHobby: function (value) {
console.log('Hobby Updated:', value);
}
});
});
What Are the Requirements for Using the `listens` Property?
The `listens` property requires the associated fields to be defined as observables. If the properties are not observables, the `listens` property will not work. Example initialization:
initObservable: function () {
this._super().observe(['firstname', 'hobby']);
return this;
}
How Does the `listens` Property Improve UI Responsiveness?
The `listens` property improves UI responsiveness by automatically triggering methods when data changes. This ensures that updates to observable properties are reflected in the UI without requiring manual event handling.
Can the `listens` Property Be Used with Data Binding?
Yes, the `listens` property is commonly used alongside Knockout data bindings like textInput
and value
. For instance, typing in a text field bound to an observable triggers the corresponding `listens` method:
Each keypress updates the property and calls the method specified in `listens`.
What Are Common Mistakes When Using the `listens` Property?
Some common mistakes include:
- Failing to declare properties as observables.
- Not initializing observables with
observe()
. - Misnaming methods referenced in the `listens` property.
- Incorrectly binding UI elements to non-observable properties.
How Is the `listens` Property Used in Magento Core Features?
In Magento, the `listens` property is widely used in core features like the guest checkout page. For instance, when users enter their email, the `listens` property triggers a function to verify whether the email is registered.
How Can You Debug Issues with the `listens` Property?
To debug issues with the `listens` property:
- Use
console.log()
to verify that methods are triggered as expected. - Ensure that properties are correctly defined as observables.
- Check for typos or misconfigurations in the `listens` property.
- Inspect the browser developer console for JavaScript errors.