Defining Knockout Observables in Magento 2: A Step-by-Step Guide
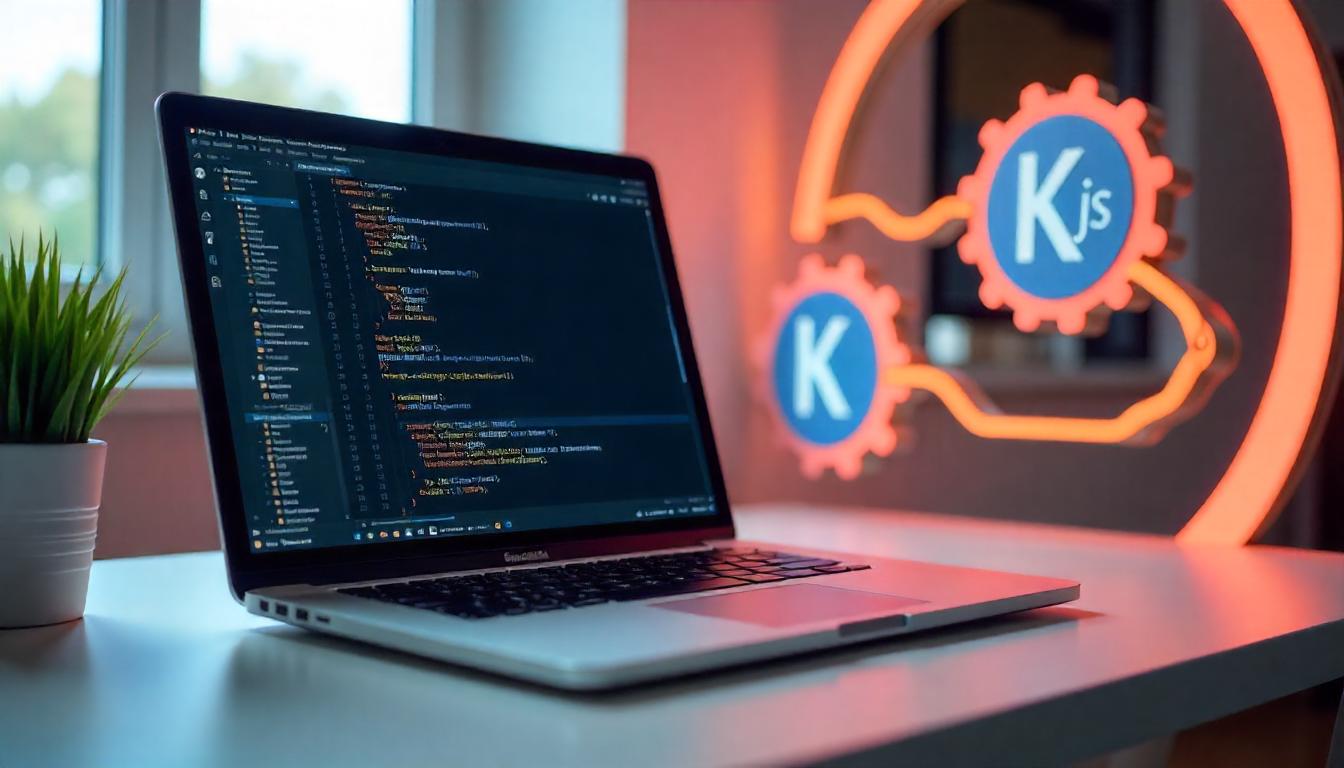
Defining Knockout Observables in Magento 2: A Step-by-Step Guide
In Magento 2, integrating Knockout.js observables allows your application to respond dynamically to user interactions. Observables track changes in your view model properties, ensuring the UI stays updated. Here's how to define observable properties in Magento 2:
Table Of Content
Using Knockout.js as a Dependency
By including Knockout.js (ko) in your module's define method, you can create observables directly:
define(['uiComponent', 'ko'], function (Component, ko) {
'use strict';
return Component.extend({
name: ko.observable('My Product Name'),
total: ko.observable(7),
items: ko.observableArray([
{ name: 'Product 1', price: 2 },
{ name: 'Product 2', price: 5 }
])
});
});
In this example, name and total are observables, while items is an observable array. This setup ensures that any changes to these properties automatically reflect in the UI.
2. Utilizing the tracks Property
Alternatively, you can define observables using the tracks property within the defaults section:define(['uiComponent'], function (Component) {
'use strict';
return Component.extend({
defaults: {
tracks: {
name: true,
total: true,
items: true
}
}
});
});
Here, setting properties in tracks to true designates them as observables. This method simplifies the declaration process.
3. Implementing initObservable
Method
Another approach involves the initObservable method to define observables:
define(['uiComponent'], function (Component) {
'use strict';
return Component.extend({
defaults: {
name: 'Default Name',
total: 10,
items: [
{ name: 'Product 1', price: 2 },
{ name: 'Product 2', price: 5 }
]
},
initObservable: function () {
this._super().observe(['name', 'total', 'items']);
return this;
}
});
});
In this setup, observe converts the specified properties into observables during initialization. This approach is beneficial for managing multiple observables efficiently.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What Are Knockout Observables in Magento 2?
Knockout observables in Magento 2 are used to track changes to a property in the view model. They allow you to create dynamic, responsive user interfaces by automatically updating when the data changes.
How Do You Define Knockout Observables in Magento 2?
In Magento 2, you can define Knockout observables by using the ko.observable()
function for single properties and ko.observableArray()
for arrays. Here’s an example:
define(['uiComponent', 'ko'], function (Component, ko) {
'use strict';
return Component.extend({
name: ko.observable("My Product Name"),
total: ko.observable(7),
items: ko.observableArray([
{name: "Product 1", price: 2},
{name: "Product 2", price: 5}
])
});
});
What Is the Purpose of Using the `tracks` Property for Knockout Observables?
The `tracks` property allows you to designate specific properties as observables in Magento 2 without explicitly using ko.observable()
. This method simplifies defining observables for properties:
define(['uiComponent'], function (Component) {
'use strict';
return Component.extend({
defaults: {
tracks: {
name: true,
total: true,
items: true
}
},
});
});
How Does the `initObservable` Method Work for Knockout Observables?
The initObservable()
method is used to initialize observables by calling observe()
on an array of properties. This approach is useful when you want to define observables after the component’s initialization:
define(['uiComponent'], function (Component) {
'use strict';
return Component.extend({
defaults: {
name: 'Default Name',
total: 10,
items: [
{name: "Product 1", price: 2},
{name: "Product 2", price: 5}
]
},
initObservable: function () {
this._super().observe(['name', 'total', 'items']);
return this;
}
});
});
What Are Common Issues When Working with Knockout Observables in Magento 2?
Common issues include:
- Incorrectly initialized observables or missing observable declarations.
- Not updating the UI after changes to observable data.
- Overusing observables, which can affect performance.
- Issues with data binding between the view model and UI components.
How Can You Debug Knockout Observables in Magento 2?
To debug Knockout observables:
- Use
console.log()
to log the observable values and check changes. - Ensure that observables are properly initialized and bound to the UI.
- Inspect the JavaScript and HTML to ensure correct data flow.
- Use browser developer tools to track changes in the view model.
Can Knockout Observables Be Customized in Magento 2?
Yes, you can customize observables in several ways:
- Define custom observable methods for data processing.
- Bind observables to different UI components or actions.
- Combine multiple observables for complex data structures.