Invalidating Full-Page Cache in Magento 2
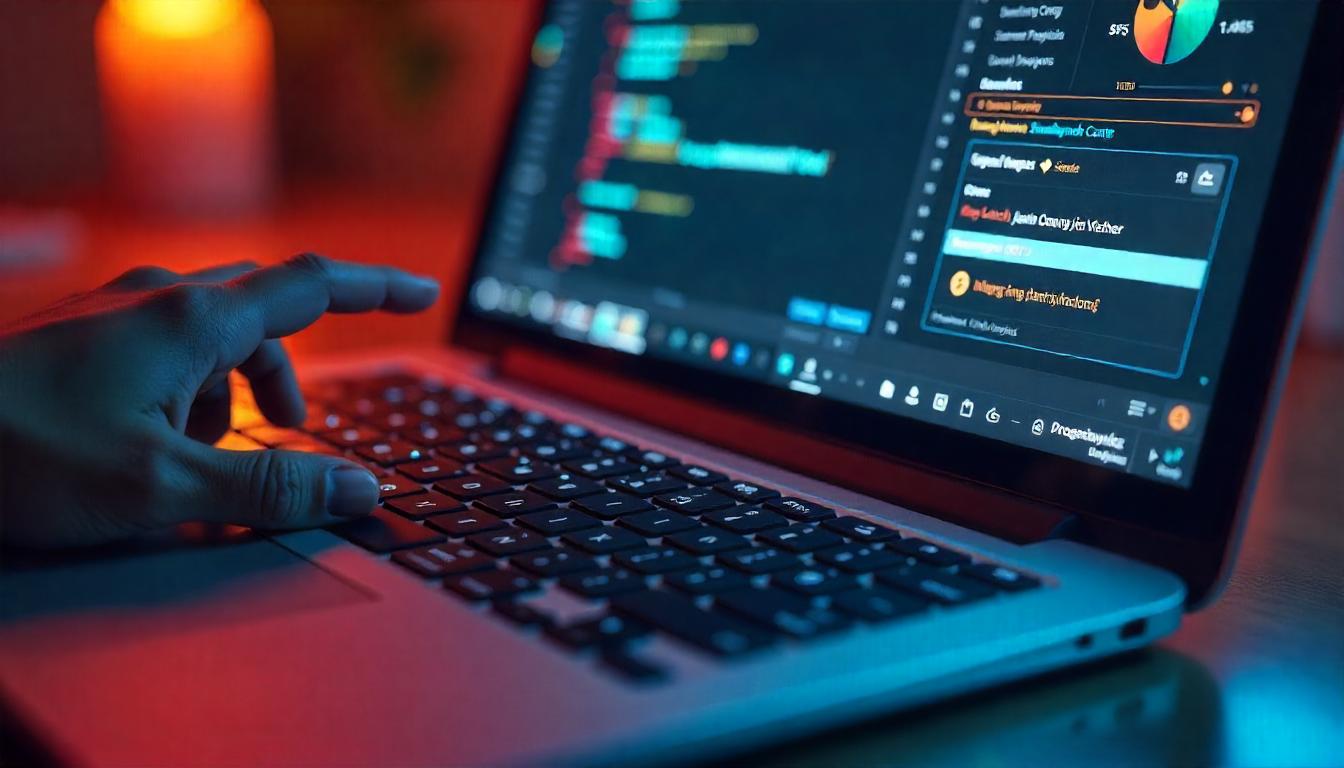
Invalidating Full-Page Cache in Magento 2
Invalidating the full-page cache in Magento 2 ensures that the most up-to-date content is served to customers. When cache data becomes outdated, it can cause issues such as displaying old information or a slower page load time. This process involves using Magento’s invalidate() method from the TypeListInterface to mark the full-page cache as invalid.
Table Of Content
Invalidating Full-Page Cache in Magento 2
To invalidate the full-page cache in Magento 2, utilize the invalidate() method from the TypeListInterface. This action is essential when you need to refresh the cache after specific events or code triggers. By invalidating the cache, you ensure that users receive the most up-to-date content.
Implementing Cache Invalidation
Here's how to programmatically invalidate the full-page cache:
- Define Dependencies: Include jQuery and the get-totals action in your module's JavaScript file.
- FULL_PAGE_CACHE is the identifier for the full-page cache type.
- The invalidateFullPageCache method calls the invalidate function, marking the full-page cache as invalid.
<?php
namespace Vendor\Module\Model;
class InvalidateCache
{
const FULL_PAGE_CACHE = 'full_page';
protected $cacheTypeList;
public function __construct(TypeListInterface $cacheTypeList)
{
$this->cacheTypeList = $cacheTypeList;
}
public function invalidateFullPageCache(): void
{
$this->cacheTypeList->invalidate(self::FULL_PAGE_CACHE);
}
}
In this example:
Invalidating Multiple Cache Types
If you need to invalidate multiple cache types simultaneously, pass an array of cache type identifiers to the invalidate method:
public function invalidateMultipleCaches(): void
{
$cacheTypes = ['config', 'layout', 'block_html'];
foreach ($cacheTypes as $type) {
$this->cacheTypeList->invalidate($type);
}
}
Understanding Cache Invalidation
Invalidating the cache doesn't immediately clear stored data. Instead, it marks the cache as outdated, prompting Magento to refresh it upon the next request. This process ensures that users access the latest content without unnecessary delays.
Common Scenarios for Cache Invalidation
- Product Updates: When product information changes, invalidating the cache ensures that customers see the latest details.
- Category Modifications: After altering category structures or attributes, cache invalidation updates the storefront accordingly.
- CMS Page Edits: Changes to CMS pages require cache invalidation to reflect new content to users.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What Is Full-Page Cache Invalidation in Magento 2?
Full-page cache invalidation in Magento 2 is the process of marking cached content as outdated. It ensures that the latest content is displayed to users after specific updates or triggers occur on the website.
How Do You Invalidate Full-Page Cache Programmatically?
To programmatically invalidate the full-page cache in Magento 2, use the invalidate() method from the TypeListInterface. This method allows you to specify one or multiple cache types for invalidation.
Example Code:
namespace Vendor\Module\Model;
use Magento\Framework\App\Cache\TypeListInterface;
class InvalidateCache
{
const FULL_PAGE_CACHE = 'full_page';
protected $cacheTypeList;
public function __construct(TypeListInterface $cacheTypeList)
{
$this->cacheTypeList = $cacheTypeList;
}
public function invalidateFullPageCache(): void
{
$this->cacheTypeList->invalidate(self::FULL_PAGE_CACHE);
}
}
Can You Invalidate Multiple Cache Types Simultaneously?
Yes, you can invalidate multiple cache types at once by passing an array of cache type identifiers to the invalidate() method. For example:
public function invalidateMultipleCaches(): void
{
$cacheTypes = ['config', 'layout', 'block_html'];
foreach ($cacheTypes as $type) {
$this->cacheTypeList->invalidate($type);
}
}
What Happens When Cache Is Invalidated?
Invalidating the cache does not immediately clear the stored data. Instead, it marks the cache as outdated, prompting Magento to refresh it during the next request. This ensures users see updated content without unnecessary delays.
Why Should You Invalidate the Full-Page Cache?
Invalidating the full-page cache is crucial to maintaining accurate and updated content on your website. Common scenarios include:
- Product Updates: To ensure customers see the latest product details.
- Category Changes: To reflect updated category structures or attributes.
- CMS Page Edits: To show new content immediately after changes.
What Are the Benefits of Full-Page Cache Invalidation?
Invalidating the cache offers several benefits:
- Improved Accuracy: Ensures users see the latest updates.
- Optimized Performance: Avoids reloading unnecessary data.
- Better User Experience: Updates content dynamically without disrupting the browsing experience.
What Should You Do If Cache Invalidation Doesn't Work?
If cache invalidation doesn't work as expected, check the following:
- Ensure the invalidate() method is implemented correctly.
- Look for errors in the browser console or logs.
- Verify that the cache type identifiers are accurate and properly passed.
How Does Cache Invalidation Impact Performance?
Cache invalidation has minimal performance impact. Instead of reloading the entire page, it updates only outdated sections. This approach enhances both frontend performance and user satisfaction.