How to Update the Cart Total Summary in Magento 2 Using JavaScript
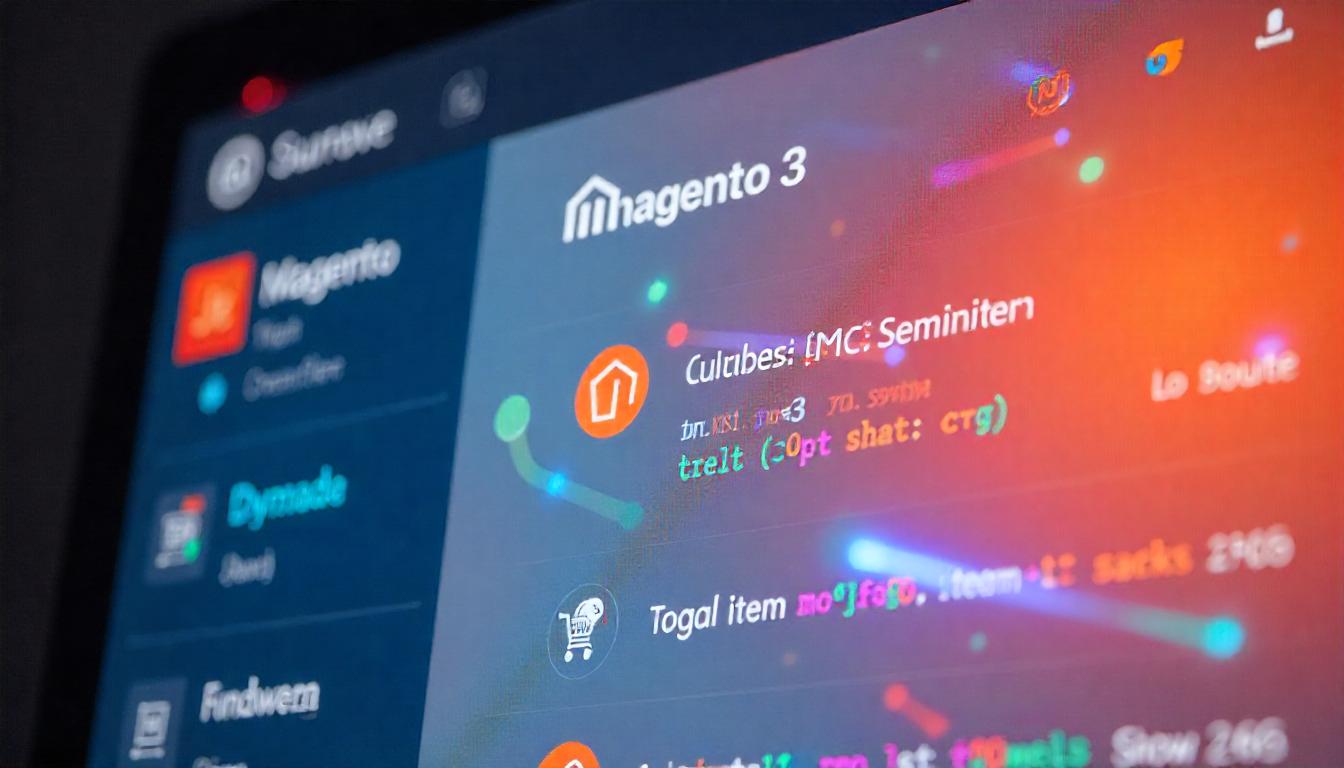
How to Update the Cart Total Summary in Magento 2 Using JavaScript
To update the cart total summary in Magento 2 using JavaScript, you can utilize built-in JavaScript actions that allow you to refresh the cart totals dynamically. This is particularly useful when updating cart items through AJAX and needing the total summary to reflect these changes without reloading the entire page. By calling the get-totals.js action, you can update the cart summary in real-time, ensuring that the totals are accurate and up-to-date. This process helps maintain a smooth and responsive user experience on the cart page by providing instant feedback on the cart's status.
Table Of Content
How to Update the Cart Total Summary in Magento 2 Using JavaScript
In Magento 2, you can refresh the cart total summary on the cart page using JavaScript. This is particularly useful when updating cart items via AJAX and needing the total summary to reflect these changes without a full page reload.
Using get-totals.js to Refresh the Cart Summary
Magento provides a built-in JavaScript action, get-totals.js, to reload the cart summary. Here's how to implement it:
- Define Dependencies: Include jQuery and the get-totals action in your module's JavaScript file.
- Invoke the Action: Call getTotalsAction to refresh the cart totals.
define([
'jquery',
'Magento_Checkout/js/action/get-totals'
], function ($, getTotalsAction) {
'use strict';
// Your code here
});
define([
'jquery',
'Magento_Checkout/js/action/get-totals'
], function ($, getTotalsAction) {
'use strict';
// Create a deferred object
var deferred = $.Deferred();
// Call the getTotalsAction to refresh the cart totals
getTotalsAction([], deferred);
});
By invoking getTotalsAction, the cart total summary will update to reflect the latest cart items.
Alternative Method: Using totals-processor.js
Another approach involves using totals-processor.js to estimate and update the totals.
- Define Dependencies: Include the necessary modules.
- Update Totals: Use the estimateTotals method to refresh the totals.
define([
'ko',
'jquery',
'Magento_Checkout/js/model/cart/totals-processor/default',
'Magento_Checkout/js/model/cart/cache'
], function (ko, $, defaultTotal, cartCache) {
'use strict';
// Your code here
});
define([
'ko',
'jquery',
'Magento_Checkout/js/model/cart/totals-processor/default',
'Magento_Checkout/js/model/cart/cache'
], function (ko, $, defaultTotal, cartCache) {
'use strict';
// Function to update the cart totals
function updateCartTotals() {
// Clear the cached totals
cartCache.set('totals', null);
// Estimate and update the totals
defaultTotal.estimateTotals();
}
// Call the function to update the totals
updateCartTotals();
});
This method clears the cached totals and estimates the new totals, ensuring the cart summary is up-to-date.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
How to Update the Cart Total Summary in Magento 2 Using JavaScript?
To update the cart total summary dynamically in Magento 2, you can use the get-totals.js action. This method is useful when updating cart items through AJAX, ensuring the cart totals are automatically refreshed without reloading the entire page.
- Real-time Update: Refreshes the cart summary after each update.
- AJAX-Driven: Updates happen via AJAX, so users experience seamless updates.
What Is the Role of the get-totals.js Action?
The get-totals.js action is used to refresh the cart total summary block on the cart page. It is triggered after a successful cart update, ensuring the total matches the latest cart items.
How Do You Implement get-totals.js to Update the Cart Summary?
To use get-totals.js in your custom JavaScript module:
- Define dependencies: jQuery and get-totals action.
- Invoke the action to refresh the cart totals after changes are made.
Example code:
define([
'jquery',
'Magento_Checkout/js/action/get-totals'
], function ($, getTotalsAction) {
'use strict';
var deferred = $.Deferred();
getTotalsAction([], deferred);
});
Can You Update the Cart Summary Without Reloading the Page?
Yes, by using the get-totals.js action in combination with AJAX, the cart total summary can be updated dynamically. This ensures that the latest total is shown without a full page reload, improving the user experience.
What Are the Benefits of Using JavaScript for Cart Total Updates?
Using JavaScript for cart total updates offers several advantages:
- Faster User Experience: No page reloads are needed, making the process faster.
- Improved Performance: Reduces the load on the server by only updating the cart total block.
- Seamless Updates: Users can see changes in real-time without disruption.
What Should You Do If the Cart Summary Doesn’t Update?
If the cart summary doesn't update, check the following:
- Ensure the get-totals.js action is correctly included and triggered.
- Check for JavaScript errors in the browser console that might prevent the action from executing.
- Verify that the AJAX response correctly updates the cart total values.
Can You Use Other Methods to Update the Cart Total Summary?
Yes, besides get-totals.js, you can use other methods such as:
- totals-processor.js: For more complex scenarios involving re-estimating totals.
- Custom AJAX: If you want more control over the cart updates, you can implement your custom AJAX solution.
What Is the Impact of Updating the Cart Total Summary on Performance?
Using JavaScript to update the cart total summary provides a minimal impact on performance, as it updates only the affected section of the page. This method reduces the need for full-page reloads, improving both frontend performance and user experience.