Comprehensive Guide to Using the wishlist_add_product Event in Magento 2
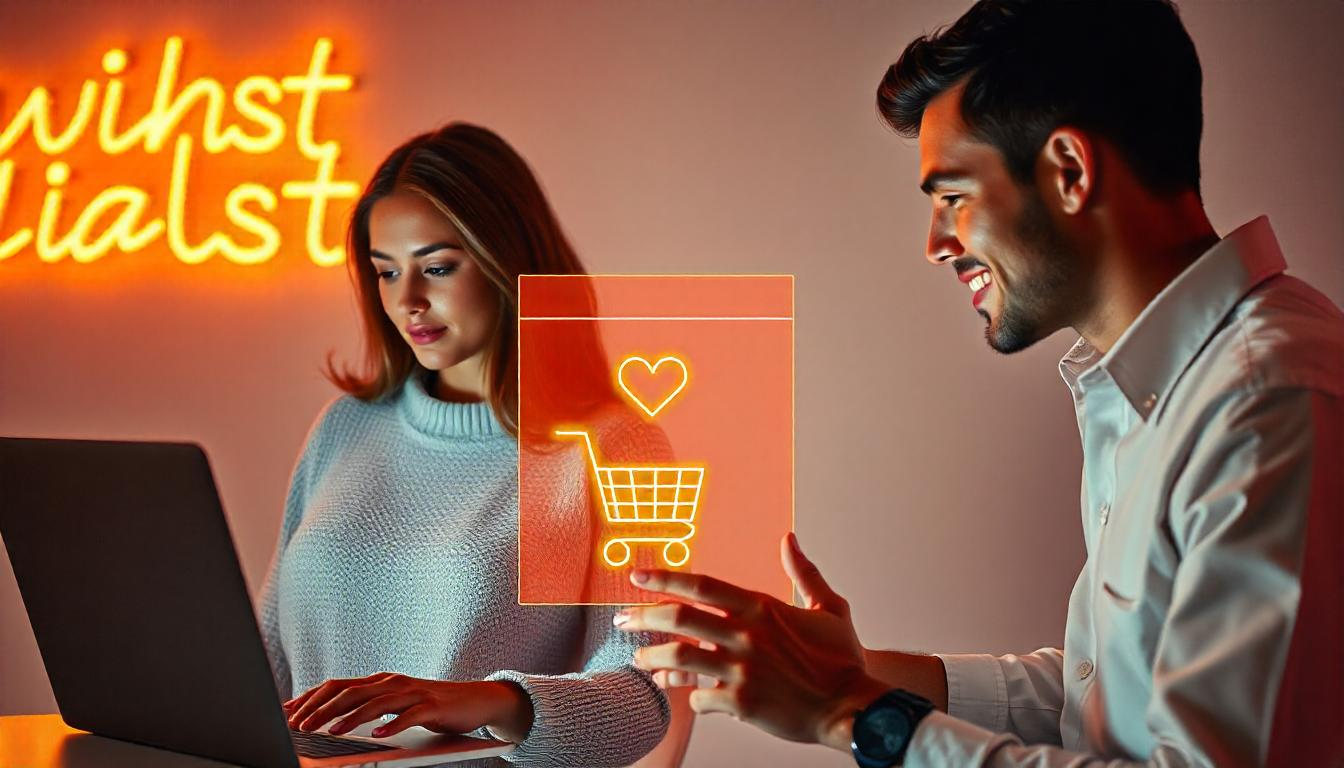
Comprehensive Guide to Using the wishlist_add_product Event in Magento 2
The wishlist_add_product
event in Magento 2 is a powerful tool for developers, enabling them to trigger custom functionality whenever a customer adds a product to their wishlist. Whether it's for logging, sending notifications, or executing business logic, this event provides flexibility and control. Here's a detailed guide to using this event effectively.
Table Of Content
- What is the wishlist_add_product Event?
- Exploring the Benefits of Using wishlist_add_product in Magento 2
- Key Components of the wishlist_add_product Event in Magento 2
- Steps to Implement the wishlist_add_product Event
- Core Magento References for wishlist_add_product
- Advantages of Using wishlist_add_product Event
- Conclusion
- FAQs
What is the wishlist_add_product Event?
This event is triggered during the process of adding a product to the wishlist. Developers can use this hook to implement custom functionalities such as logging, sending notifications, or modifying wishlist data.
Key Characteristics of the Event
Attribute | Details |
---|---|
Event Name | wishlist_add_product |
Trigger Point | When a product is successfully added to the wishlist. |
Parameters Available | Product, Wishlist, Customer Information. |
Primary Use Cases | Logging, sending notifications, triggering workflows, and analytics. |
Scope | Global, affecting all wishlist additions unless scoped programmatically. |
Workflow of the wishlist_add_product Event
1. Triggering the Event
The event is triggered whenever a customer adds a product to their wishlist, either from the frontend or via AJAX requests.
2. Observing the Event
Magento allows developers to observe this event and define custom logic in an observer class.
3. Processing Custom Logic
The observer processes custom logic, such as sending an email, logging data, or updating a custom database table.
Exploring the Benefits of Using wishlist_add_product in Magento 2
The wishlist_add_product
event is a powerful tool that provides flexibility and customization for Magento 2 developers. By utilizing this event, you can implement targeted functionalities to enhance user engagement, streamline backend operations, and gather actionable insights.
Why Use wishlist_add_product?
1. Custom Notifications
- Notify admins or customers about wishlist updates to keep them informed.
- Examples:
- Send customers an email notification with details about the product added.
- Alert admins when a high-value product is frequently added to wishlists.
2. Data Analysis
- Track frequently added products to gain insights into customer preferences.
- Examples:
- Identify trending products.
- Analyze data for inventory planning or promotional strategies.
3. Custom Actions
- Execute business-specific logic tailored to your operational needs.
- Examples:
- Apply targeted promotions to products in the wishlist.
- Update external systems, such as CRM or ERP software.
Practical Use Cases of wishlist_add_product
Use Case | Description | Benefit |
---|---|---|
Personalized Email Campaigns | Send customized emails to customers when a product is added to their wishlist. | Enhances customer engagement and increases sales. |
Trend Monitoring | Log wishlist activity to identify products that are popular among customers. | Helps in making informed decisions about inventory and marketing campaigns. |
Dynamic Discounts | Automatically apply discounts to frequently wishlisted products. | Encourages customers to proceed to checkout. |
CRM Integration | Sync wishlist activity with a CRM system for targeted customer segmentation. | Enables personalized marketing strategies. |
Reward Programs | Award loyalty points or rewards to customers for using the wishlist feature. | Promotes brand loyalty and repeat purchases. |
Advanced Features Enabled by wishlist_add_product
Feature | Description | Implementation Strategy |
---|---|---|
Product Cross-Selling | Suggest complementary products based on wishlist items. | Use the observer to trigger cross-sell product recommendations. |
Stock Alerts | Notify customers when wishlisted items are back in stock. | Integrate with stock management systems to send alerts. |
Wishlist Sharing | Enable customers to share their wishlist with friends or family via email or social media. | Use the observer to track shared wishlists and log activities. |
Key Components of the wishlist_add_product Event in Magento 2
The wishlist_add_product
event exposes key objects that developers can use to create custom logic. Each component represents a specific aspect of the wishlist functionality, enabling precise and meaningful customizations.
Key Components Overview
Component | Purpose | Accessible Via |
---|---|---|
Wishlist Object | Represents the current wishlist instance. | $observer->getEvent()->getWishlist() |
Product Object | Represents the product being added to the wishlist. | $observer->getEvent()->getProduct() |
Item Object | Represents the wishlist item created after the product is added. | $observer->getEvent()->getItem() |
Detailed Breakdown of Components
1. Wishlist Object
The wishlist object provides access to the customer’s wishlist details and allows developers to modify or interact with the wishlist data programmatically.
Method | Description | Example Code |
---|---|---|
getId() |
Retrieves the ID of the wishlist. | $wishlistId = $wishlist->getId(); |
getItems() |
Retrieves all items in the wishlist. | $items = $wishlist->getItems(); |
addNewItem($product) |
Adds a new product to the wishlist. | $wishlist->addNewItem($product); |
save() |
Saves changes made to the wishlist. | $wishlist->save(); |
2. Product Object
The product object represents the product being added to the wishlist. It is useful for extracting product-specific information or triggering product-related actions.
Attribute | Description | Example Code |
---|---|---|
getName() |
Retrieves the product name. | $productName = $product->getName(); |
getSku() |
Retrieves the product SKU. | $productSku = $product->getSku(); |
getPrice() |
Retrieves the product price. | $productPrice = $product->getPrice(); |
getTypeId() |
Retrieves the product type (e.g., simple, configurable). | $productType = $product->getTypeId(); |
3. Item Object
The item object provides information about the specific wishlist item created after adding a product. It is ideal for adding custom attributes or processing item-level logic.
Attribute/Method | Description | Example Code |
---|---|---|
getId() |
Retrieves the wishlist item ID. | $itemId = $item->getId(); |
getWishlistId() |
Retrieves the ID of the wishlist containing the item. | $wishlistId = $item->getWishlistId(); |
setCustomAttribute($key, $value) |
Sets a custom attribute for the wishlist item. | $item->setCustomAttribute('source', 'event')->save(); |
How These Components Interact
The wishlist_add_product
event passes these components to the observer, enabling seamless integration into custom workflows. Here's how they interact in a typical scenario:
Component | Interaction | Example Use Case |
---|---|---|
Wishlist Object | The wishlist is fetched to add additional details or update it dynamically. | Add promotional messages or synchronize with external systems. |
Product Object | The product is used to extract details or validate conditions before adding it to the wishlist. | Prevent adding restricted products to the wishlist. |
Item Object | The wishlist item is customized with additional data or attributes. | Link the wishlist item to loyalty points or external tracking systems. |
Comparison of Magento Wishlist Events
Event Name | Trigger Point | Primary Use Case |
---|---|---|
wishlist_add_product |
When a product is added to the wishlist. | Custom notifications, logging, or analytics. |
wishlist_items_add |
Adding multiple items to the wishlist. | Batch processing or syncing actions. |
wishlist_items_update |
Updating existing wishlist items. | Custom logic for modified wishlist items. |
wishlist_items_delete |
Removing items from the wishlist. | Cleanup tasks or logging removal actions. |
Steps to Implement the wishlist_add_product Event
1. Register the Event in events.xml
The events.xml
file is essential for defining the event and linking it to an observer. This configuration file tells Magento which class should handle the custom logic for the event.
Sample events.xml Configuration
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:Event/etc/events.xsd">
<event name="wishlist_add_product">
<observer name="wishlist_add_product_observer" instance="Vendor\Module\Observer\WishlistAddItemObserver" />
</event>
</config>
File Path:
app/code/Vendor/Module/etc/frontend/events.xml
Key Elements in events.xml
Element | Description | Example |
---|---|---|
<event name> |
Specifies the event to listen for. | wishlist_add_product |
<observer name> |
Unique identifier for the observer. | wishlist_add_product_observer |
instance |
Specifies the observer class responsible for handling the event. | Vendor\Module\Observer\WishlistAddItemObserver |
2. Create the Observer Class
The observer class contains the custom logic that runs whenever the wishlist_add_product
event is triggered.
Sample Observer Class
<?php
namespace Vendor\Module\Observer;
use Magento\Framework\Event\ObserverInterface;
use Magento\Framework\Event\Observer;
class WishlistAddItemObserver implements ObserverInterface
{
/**
* Execute custom logic after a product is added to the wishlist.
*
* @param Observer $observer
* @return void
*/
public function execute(Observer $observer)
{
// Fetch objects from the event
$wishlist = $observer->getEvent()->getWishlist();
$product = $observer->getEvent()->getProduct();
$item = $observer->getEvent()->getItem();
// Example: Logging the wishlist addition
$logger = \Magento\Framework\App\ObjectManager::getInstance()
->get(\Psr\Log\LoggerInterface::class);
$logger->info("Product '{$product->getName()}' (ID: {$product->getId()}) added to Wishlist ID {$wishlist->getId()}");
// Custom logic here...
}
}
File Path:
app/code/Vendor/Module/Observer/WishlistAddItemObserver.php
Key Elements in the Observer Class
Component | Purpose | Example Code |
---|---|---|
$observer->getEvent() |
Fetches the event data passed during the trigger. | $observer->getEvent()->getProduct() |
LoggerInterface |
Logs activity for debugging or auditing purposes. | $logger->info("Message"); |
Custom Logic |
Add your own logic to handle wishlist events. | Update an external CRM or apply special discounts. |
3. Example Use Case: Notify Admins About Wishlist Additions
Let’s extend the observer to send an email notification to admins when a product is added to a wishlist.
Extended Observer Code
<?php
namespace Vendor\Module\Observer;
use Magento\Framework\Event\ObserverInterface;
use Magento\Framework\Event\Observer;
use Magento\Framework\Mail\Template\TransportBuilder;
use Magento\Store\Model\StoreManagerInterface;
class WishlistAddItemObserver implements ObserverInterface
{
protected $transportBuilder;
protected $storeManager;
public function __construct(
TransportBuilder $transportBuilder,
StoreManagerInterface $storeManager
) {
$this->transportBuilder = $transportBuilder;
$this->storeManager = $storeManager;
}
public function execute(Observer $observer)
{
$product = $observer->getEvent()->getProduct();
// Prepare email data
$emailVars = [
'product_name' => $product->getName(),
'product_url' => $product->getProductUrl(),
];
// Send email
$transport = $this->transportBuilder
->setTemplateIdentifier('wishlist_notification_template') // Email template ID
->setTemplateOptions(['area' => 'frontend', 'store' => $this->storeManager->getStore()->getId()])
->setTemplateVars($emailVars)
->setFrom('general') // Sender (defined in Magento admin)
->addTo('[email protected]') // Admin email
->getTransport();
$transport->sendMessage();
}
}
4. Test the Implementation
To ensure your observer works correctly:
- Add a product to the wishlist from the frontend.
- Check your logs or email notifications for the custom logic output.
Core Magento References for wishlist_add_product
To gain deeper insights into how Magento implements the wishlist_add_product
event, it is crucial to review specific core files and modules. These references showcase how Magento handles the event and provide examples for extending its functionality.
1. Magento_Wishlist Add Controller
This file is the backbone of the wishlist addition process. It manages the logic for adding products to a customer's wishlist and triggers the wishlist_add_product
event.
- Purpose: Handles the addition of products to the wishlist.
- File Path:
vendor/magento/module-wishlist/Controller/Index/Add.php
Key Details:
- This controller checks whether the product is valid for adding to the wishlist.
- It dispatches the
wishlist_add_product
event after successfully adding a product. - You can extend or override this file to implement custom validation or additional business logic.
2. Magento_Reports Example
Magento uses the wishlist_add_product
event in its Reports module to track wishlist activity. Reviewing this implementation provides a practical example of how the event is leveraged for analytics.
- Purpose: Demonstrates how Magento uses the event for reporting and analytics.
- File Path:
vendor/magento/module-reports/Observer/WishlistAddProductObserver.php
Key Details:
- The observer captures the event to log wishlist activity for reporting purposes.
- It serves as a template for creating custom observers for analytics or similar functionality.
- This implementation highlights how data from the event can be processed and stored for future analysis.
How These References Help Developers
By studying the core files mentioned above:
- You can understand how Magento natively triggers and utilizes the
wishlist_add_product
event. - These files serve as blueprints for developing custom observers or extending the wishlist functionality.
- They demonstrate best practices for implementing event-based logic within Magento 2.
For additional customization, developers can override or extend these files while adhering to Magento's module development standards.
Advantages of Using wishlist_add_product Event
The wishlist_add_product
event in Magento 2 offers a range of advantages that can enhance both the functionality and customer experience of your store. By hooking into this event, developers can execute custom logic, track user behavior, and improve business operations. Here are some key benefits:
1. Custom Business Logic
One of the major advantages of using the wishlist_add_product
event is the ability to implement custom business logic tailored to your store's specific needs. Whether it's applying special promotions, handling inventory updates, or triggering actions in external systems, this event provides a flexible hook to extend Magento's native functionality.
Examples of Custom Logic:
- Apply special discounts when customers add certain products to their wishlist.
- Update stock levels or trigger restocking alerts when popular items are frequently added.
- Sync wishlist additions with external systems, such as customer relationship management (CRM) software.
By integrating these custom actions, you can automate key workflows that improve operational efficiency and customer satisfaction.
2. Improved Customer Experience
The wishlist_add_product
event enables you to create a more personalized experience for your customers. You can offer tailored recommendations, provide custom notifications, or even adjust product availability based on their wishlist actions. Personalization is crucial in building long-term customer loyalty, and this event allows you to create engaging experiences that resonate with your shoppers.
Ways to Improve Customer Experience:
- Send personalized email notifications when an item on the wishlist goes on sale or is back in stock.
- Offer customers incentives, such as exclusive discounts or early access to promotions, for products they add to their wishlist.
- Provide dynamic recommendations based on the contents of a customer’s wishlist.
These personalized interactions can drive engagement and enhance the likelihood of converting wishlist items into purchases.
3. Enhanced Analytics
Tracking customer behavior is key to understanding preferences and improving product offerings. By using the wishlist_add_product
event, you can capture data on which products are added to wishlists, providing valuable insights into customer interests. Analyzing this data can help identify trends, optimize product offerings, and even shape future marketing campaigns.
Benefits of Enhanced Analytics:
- Track which products are most frequently added to wishlists, allowing you to make data-driven inventory decisions.
- Identify potential cross-sell or up-sell opportunities by analyzing product pairings in wishlists.
- Use wishlist data to segment customers based on preferences for more targeted marketing efforts.
With robust analytics, you can fine-tune your business strategy and deliver more effective marketing, ultimately boosting sales and customer retention.
By leveraging the wishlist_add_product
event, you can unlock numerous opportunities for enhancing business logic, improving customer engagement, and gaining valuable insights into your store’s performance.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Conclusion
The wishlist_add_product
event in Magento 2 is a powerful tool that allows developers to enhance the default functionality of the platform by executing custom actions when products are added to a customer's wishlist. By leveraging this event, you can introduce personalized business logic, improve the customer experience, and gain deeper insights into your customers' preferences and shopping habits.
From sending custom notifications and applying special discounts to tracking trends and optimizing marketing strategies, the event offers a versatile framework for creating a more engaging and efficient eCommerce experience. Integrating custom actions based on wishlist additions can not only streamline your business operations but also foster customer loyalty by making their shopping experience more personalized.
Moreover, understanding the core components of the event, such as the wishlist, product, and item objects, enables you to build sophisticated workflows that align with your business goals. With the ability to create tailored solutions through events, Magento 2 empowers store owners and developers to meet the evolving demands of the eCommerce landscape.
Incorporating the wishlist_add_product
event into your Magento store ensures that your platform remains dynamic, customer-centric, and aligned with industry best practices, ultimately contributing to a more profitable and seamless shopping experience.
FAQs
What is the "wishlist_add_product" event in Magento 2?
The "wishlist_add_product" event in Magento 2 is triggered when a product is successfully added to a customer's wishlist. Developers can hook into this event to execute custom actions, such as sending notifications or applying discounts.
Why would I use the "wishlist_add_product" event?
Using the "wishlist_add_product" event allows you to extend Magento’s functionality by adding custom business logic, improving customer experience, and enhancing analytics to track customer preferences.
How can I implement the "wishlist_add_product" event in Magento 2?
To implement the "wishlist_add_product" event, register it in the `events.xml` file, then create an observer class that contains the custom logic you want to execute when the event is triggered.
What files do I need to modify to implement the "wishlist_add_product" event?
You need to modify the `events.xml` file to register the event and create an observer class that handles the custom logic triggered by the event. The observer class will be where your custom actions are executed.
Can I send notifications when a product is added to a wishlist?
Yes, you can send notifications, such as email alerts to customers or admins, when a product is added to a wishlist. This can be configured through the observer class hooked to the "wishlist_add_product" event.
How can I track customer behavior using the "wishlist_add_product" event?
By observing the "wishlist_add_product" event, you can track which products are added to customer wishlists. This data can be used for analytics, reporting, and customer behavior analysis.
What custom business logic can be implemented with the "wishlist_add_product" event?
Custom business logic could include applying discounts, triggering restocking alerts, syncing with external systems, or updating customer profiles with wishlist information.
How does the "wishlist_add_product" event improve customer experience?
The event allows you to personalize the shopping experience by offering special discounts, notifications for price drops, or product restocks based on the customer's wishlist actions.
How can I personalize customer notifications based on wishlist activity?
By using the "wishlist_add_product" event, you can send personalized notifications such as price drop alerts, back-in-stock messages, or exclusive offers based on the customer's wishlist products.
How do I use the "wishlist_add_product" event for enhanced analytics?
You can track the products most frequently added to wishlists, analyze customer preferences, and identify cross-sell or up-sell opportunities to improve your sales strategy.
Can I trigger a custom workflow when a product is added to the wishlist?
Yes, you can trigger custom workflows such as applying specific promotions, syncing the wishlist data with third-party systems, or initiating internal processes like inventory checks using the "wishlist_add_product" event.
Where can I find Magento’s implementation of the "wishlist_add_product" event?
You can find Magento’s implementation of the event in the `vendor/magento/module-wishlist` module, specifically in the `Controller/Index/Add.php` file, which handles the wishlist addition process.
What Magento core modules use the "wishlist_add_product" event?
Magento’s core modules, such as `Magento_Wishlist` and `Magento_Reports`, utilize the "wishlist_add_product" event for managing and analyzing wishlist activities. You can refer to these modules for implementation examples.