Implementing Conditional Logic in Knockout.js for Magento 2
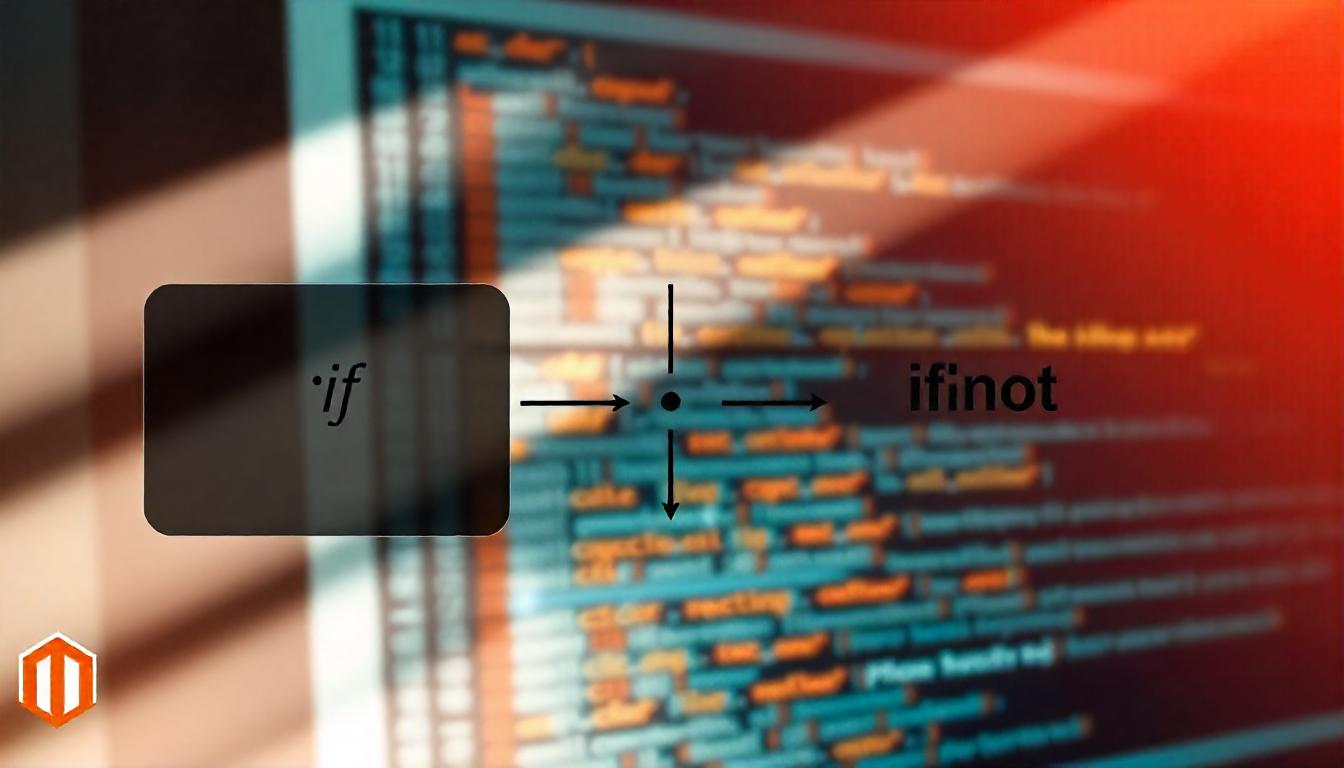
Implementing Conditional Logic in Knockout.js for Magento 2
Learn how to efficiently use if and ifnot bindings in Knockout.js to control the display of elements in Magento 2 templates. This guide explains how to implement conditional logic using both Knockout.js's default syntax and Magento 2's standard approaches.
Table Of Content
Implementing Conditional Logic in Knockout.js for Magento 2
In Magento 2, you can manage conditional logic in your templates using Knockout.js's if and ifnot bindings. These bindings control the display of elements based on the truthiness of expressions.
Using Knockout.js Bindings
To display content when a condition is true:
<!-- ko if: condition -->
<!-- Your content here -->
<!-- /ko -->
To display content when a condition is false:
<!-- ko ifnot: condition -->
<!-- Your content here -->
<!-- /ko -->
For example, to show a price when a product is available:
<!-- ko if: isProductAvailable() -->
<span class="price">100</span>
<!-- /ko -->
<!-- ko ifnot: isProductAvailable() -->
<span class="price">Out of Stock</span>
<!-- /ko -->
In this example, isProductAvailable is a function that returns a boolean indicating the product's availability.
Using Magento 2's Standard Approach
Magento 2 offers a standard syntax for conditional logic:
<if args="condition">
<!-- Your content here -->
</if>
<ifnot args="condition">
<!-- Your content here -->
</ifnot>
For instance, to display a price when a product is available:
<if args="isProductAvailable()">
<span class="price">100</span>
</if>
<ifnot args="isProductAvailable()">
<span class="price">Out of Stock</span>
</ifnot>
Here, isProductAvailable is a function that returns a boolean indicating the product's availability.
Handling Multiple Conditions
To handle multiple conditions, you can combine them using logical operators:
<if args="isEnabled() && isProductAvailable()">
<span class="price">100</span>
</if>
<ifnot args="isEnabled() && isProductAvailable()"">
<span class="price">Unavailable</span</span>
</ifnot>
In this example, the price is displayed only if both isEnabled and isProductAvailable return true.
Key Points
- Use
if
to display content when a condition is true. - Use
ifnot
to display content when a condition is false. - Combine conditions with logical operators for complex logic.
- Ensure that the functions used in conditions return boolean values.
By applying these methods, you can effectively manage conditional content in your Magento 2 templates using Knockout.js.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What Is Conditional Logic in Knockout.js for Magento 2?
Conditional logic in Knockout.js for Magento 2 allows you to control the visibility of elements in the UI based on certain conditions using if and ifnot bindings.
How Do I Use the if and ifnot Bindings?
Use if to display elements when a condition is true and ifnot when it’s false. Here’s an example:
100
Out of Stock
What Is the Magento 2 Standard for Conditional Logic?
Magento 2 offers its own syntax for conditional logic using <if> and <ifnot> tags. Example:
100
Out of Stock
Can I Use Multiple Conditions in Knockout.js?
Yes, you can use logical operators like && to combine conditions. For example:
100
What Are the Common Challenges When Using Conditional Logic?
Some common challenges include:
- Incorrect syntax for Knockout.js or Magento 2 bindings.
- Logical errors in condition definitions.
- Performance issues if conditions rely on complex computations.
Why Is Conditional Logic Important in Magento 2?
Conditional logic enhances user experience by dynamically displaying relevant content. It also optimizes workflows by adapting the UI to specific scenarios or data states.
How Can I Test Conditional Logic in Knockout.js?
You can test conditional logic by:
- Setting up a sample template with if and ifnot bindings.
- Logging the conditions to ensure correct evaluation.
- Verifying the UI changes based on dynamic data updates.