Understanding and Retrieving Admin Session Lifetime in Magento 2
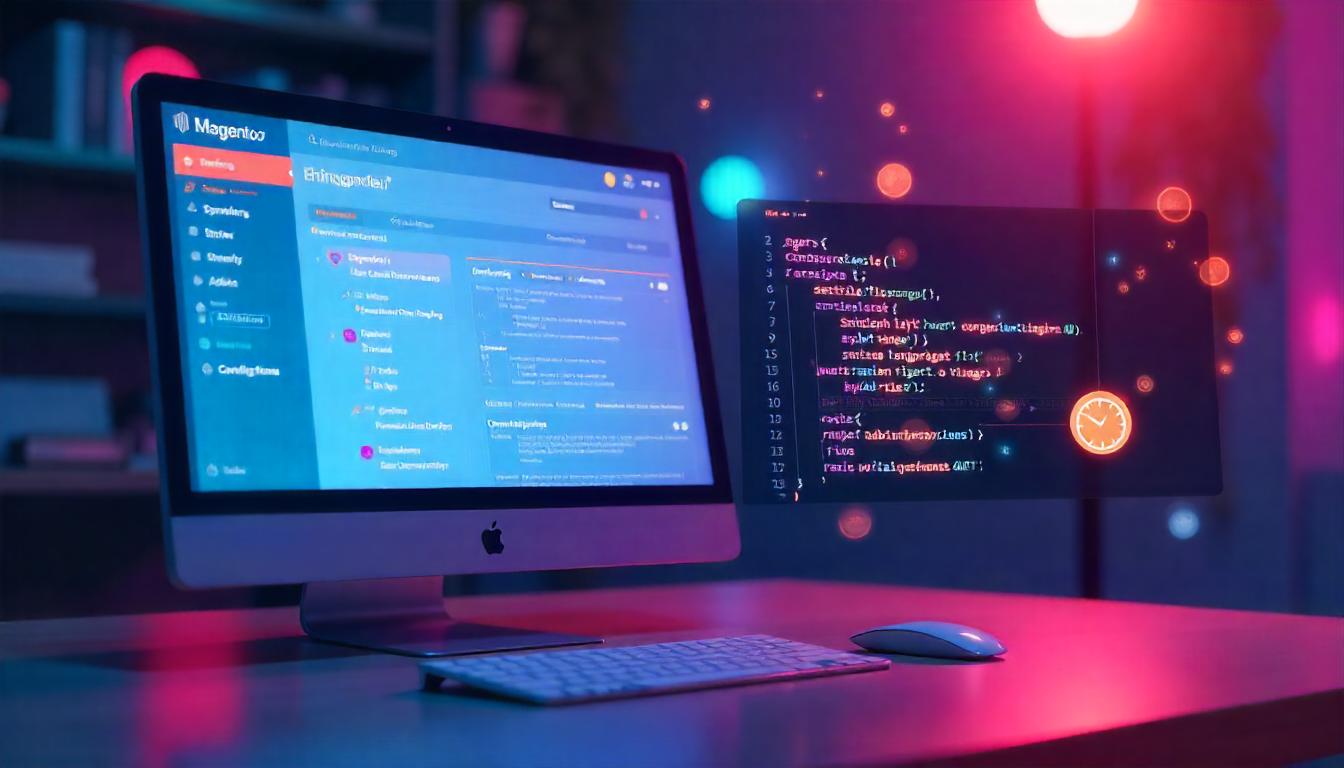
Understanding and Retrieving Admin Session Lifetime in Magento 2
Learn how to manage and retrieve the admin session lifetime in Magento 2. This setting controls how long an admin stays logged in before being logged out due to inactivity. Adjust it through the admin panel or fetch it programmatically using ConfigInterface. This guide provides step-by-step instructions and code examples to help you configure session lifetime securely and efficiently.
Table Of Content
Understanding and Retrieving Admin Session Lifetime in Magento 2
The admin session lifetime in Magento 2 determines how long an admin stays logged in before being automatically logged out due to inactivity. You can configure this setting through the Magento admin panel or programmatically.
Admin Panel Configuration
To adjust the admin session lifetime through the admin panel:
You can customize this value based on your security needs, but setting it too high might increase security risks.
Retrieving the Value Programmatically
<?php
namespace Custom\AdminSessionLifetime\Model;
use Magento\Backend\App\ConfigInterface;
class AdminSessionLifetime
{
/**
* Admin session lifetime XML path
*/
const XML_PATH_SESSION_LIFETIME = 'admin/security/session_lifetime';
/**
* @var ConfigInterface
*/
private $config;
public function __construct(ConfigInterface $config)
{
$this->config = $config;
}
/**
* Get the admin session lifetime value
*
* @return int|null
*/
public function getSessionLifetime()
{
return $this->config->getValue(self::XML_PATH_SESSION_LIFETIME);
}
}
?>
Notes and Recommendations
- The session lifetime value is stored in the core_config_data table in the database.
- Be cautious about setting extremely high values, as this can make your admin panel less secure.
Setting | Value Range | Default Value |
---|---|---|
Admin Session Lifetime | 60 seconds to 31,536,000 | 900 seconds |
By following these steps, you can easily manage and retrieve the admin session lifetime value in Magento 2. If past attempts at customization caused confusion, this guide simplifies the process.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What Is the Admin Session Lifetime in Magento 2?
The admin session lifetime in Magento 2 defines the duration of inactivity after which an admin user is automatically logged out.
How Can I Configure the Admin Session Lifetime?
You can configure the admin session lifetime in the Magento admin panel. Navigate to:
Stores > Configuration > Admin > Security
Locate the "Admin Session Lifetime (seconds)" field and set a value between 60 and 31,536,000 seconds.
How Do I Retrieve the Admin Session Lifetime Programmatically?
Use the ConfigInterface class to fetch the session lifetime programmatically. Here’s an example:
namespace Custom\AdminSessionLifetime\Model;
use Magento\Backend\App\ConfigInterface;
class AdminSessionLifetime
{
const XML_PATH_SESSION_LIFETIME = 'admin/security/session_lifetime';
private $config;
public function __construct(ConfigInterface $config)
{
$this->config = $config;
}
public function getSessionLifetime()
{
return $this->config->getValue(self::XML_PATH_SESSION_LIFETIME);
}
}
What Are the Minimum and Maximum Values for Session Lifetime?
The session lifetime can be set to a minimum of 60 seconds and a maximum of 31,536,000 seconds (1 year).
What Commands Should I Run After Changing the Session Lifetime?
After making changes, clear the cache using these commands:
php bin/magento cache:flush
php bin/magento cache:clean
You can also clear the cache via the admin panel under System > Tools > Cache Management.
What Common Issues May Arise While Modifying the Session Lifetime?
Potential issues include:
- Changes not reflecting due to uncleared cache.
- Conflicts with third-party modules affecting the security settings.
- Customizations in the theme overriding the configuration.
Why Should You Customize the Admin Session Lifetime?
Customizing the admin session lifetime enhances security by limiting session duration. However, longer durations can improve convenience for admins working on time-intensive tasks.
How Can I Verify That the Changes Were Applied?
Test the configuration by logging in as an admin and waiting for the set session duration to expire. If the session ends after the configured time, the changes were successful.