Retrieving Tier Prices by Product SKU in Magento 2
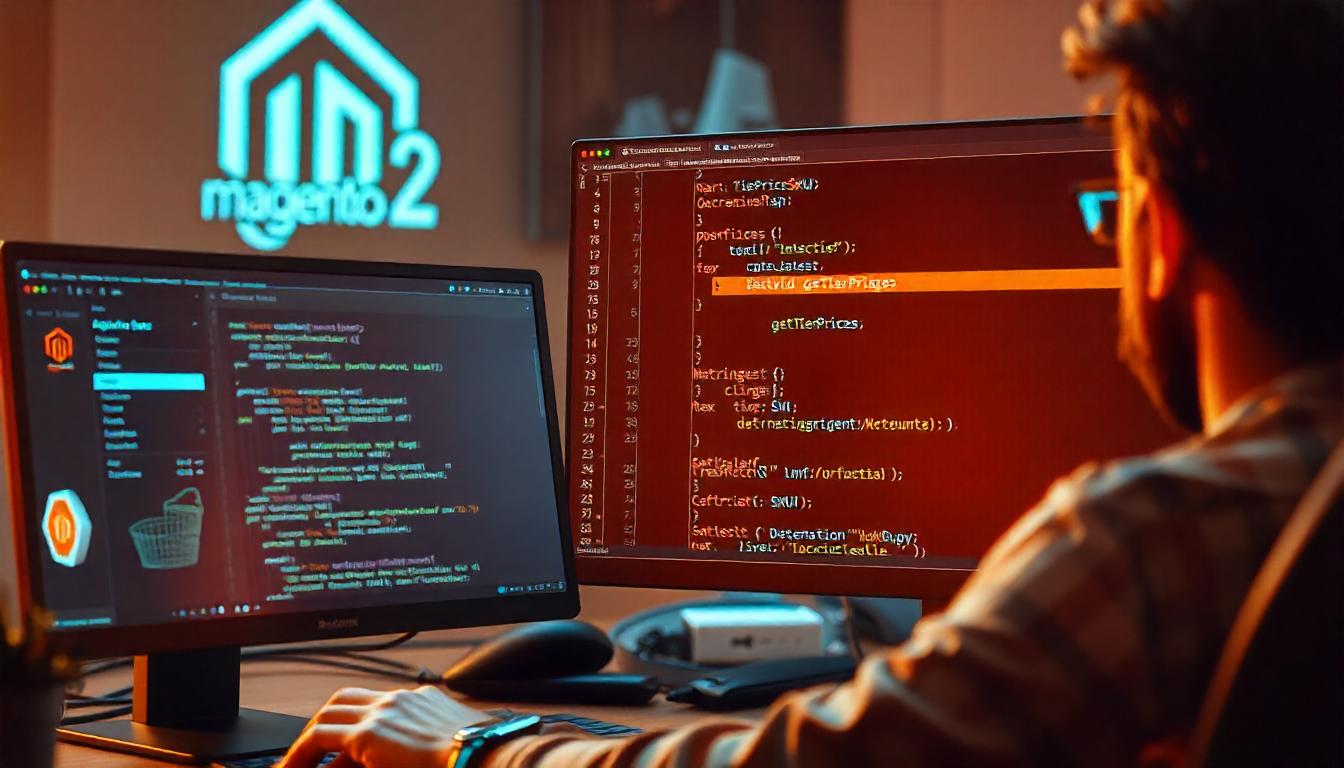
Retrieving Tier Prices by Product SKU in Magento 2
In Magento 2, retrieving tier prices for products by their SKU is a straightforward process using the TierPriceStorageInterface. This method allows developers to efficiently fetch tier pricing data based on product SKUs, making it easier to manage discounts and pricing structures for specific customer groups and quantities.
Table Of Content
Retrieving Tier Prices by Product SKU in Magento 2
In Magento 2, you can fetch tier prices for products using the TierPriceStorageInterface. This interface allows you to retrieve tier pricing information based on product SKUs.
Using the TierPriceStorageInterface
The get method of TierPriceStorageInterface accepts an array of SKUs and returns the corresponding tier pricing data.
Implementation Example
Here's how you can implement this in your Magento 2 module:
<?php
namespace YourNamespace\YourModule\Model;?>
use Magento\Catalog\Api\Data\TierPriceInterface;
use Magento\Catalog\Api\TierPriceStorageInterface;
use Psr\Log\LoggerInterface;
class TierPriceFetcher
private $tierPriceStorage;
private $logger;
public function __construct(
TierPriceStorageInterface $tierPriceStorage,
LoggerInterface $logger
) {
$this->tierPriceStorage = $tierPriceStorage;
$this->logger = $logger;
}
public function getTierPrices(array $skus): array
{
try {
return $this->tierPriceStorage->get($skus);
} catch (\Exception $exception) {
$this->logger->error('Error fetching tier prices: ' . $exception->getMessage());
return [];
}
}
Usage
To use this method, pass an array of SKUs:
<?php $skus = ['product-sku-1', 'product-sku-2']; ?>
<?php $fetcher = new \YourNamespace\YourModule\Model\TierPriceFetcher($tierPriceStorage, $logger); ?>
<?php $tierPrices = $fetcher->getTierPrices($skus); ?>
foreach ($tierPrices as $tierPrice) {
// Process each tier price
$data = $tierPrice->getData();
// Example: var_dump($data);
}
Expected Output
The getData() method will return an array with details such as:
price:
The discounted price.price_type:
The type of discount (e.g., 'discount').website_id:
The website ID.sku:
The product SKU.customer_group:
The customer group applicable.quantity:
The quantity threshold for the discount.
Ensure that the TierPriceStorageInterface is properly injected into your class. This approach allows you to efficiently retrieve tier pricing information for multiple products by their SKUs.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What Does Retrieving Tier Prices by Product SKU Mean in Magento 2?
Retrieving tier prices by product SKU in Magento 2 allows you to fetch specific pricing details for products based on their SKU. This helps you apply discounts or pricing strategies efficiently for individual products without affecting others.
How Can I Retrieve Tier Prices for Products Using SKU in Magento 2?
You can retrieve tier prices by using the TierPriceStorageInterface
in Magento 2. The get
method accepts an array of SKUs and returns tier pricing information for those products.
What Is the Code for Retrieving Tier Prices by SKU?
Here is an example code to retrieve tier prices for products by SKU:
namespace YourNamespace\YourModule\Model;
use Magento\Catalog\Api\Data\TierPriceInterface;
use Magento\Catalog\Api\TierPriceStorageInterface;
use Psr\Log\LoggerInterface;
class TierPriceFetcher
{
private $tierPriceStorage;
private $logger;
public function __construct(
TierPriceStorageInterface $tierPriceStorage,
LoggerInterface $logger
) {
$this->tierPriceStorage = $tierPriceStorage;
$this->logger = $logger;
}
public function getTierPrices(array $skus): array
{
try {
return $this->tierPriceStorage->get($skus);
} catch (\Exception $exception) {
$this->logger->error('Error fetching tier prices: ' . $exception->getMessage());
return [];
}
}
}
How Do I Retrieve Tier Prices for Multiple Products by SKU?
You can retrieve tier prices for multiple products by passing an array of SKUs. Here's an example:
$skus = ['product-sku-1', 'product-sku-2'];
$tierPrices = $fetcher->getTierPrices($skus);
foreach ($tierPrices as $tierPrice) {
// Process each tier price
$data = $tierPrice->getData();
// Example: var_dump($data);
}
What Information Will I Get When Retrieving Tier Prices?
The tier price data includes:
- price: The discounted price.
- price_type: The type of discount (e.g., 'discount').
- website_id: The website ID where the price applies.
- sku: The SKU of the product.
- customer_group: The customer group eligible for the tier price.
- quantity: The quantity required to apply the tier price.
What Is the Benefit of Retrieving Tier Prices by SKU?
Retrieving tier prices by SKU allows you to target specific products and apply custom pricing strategies or discounts. This is especially useful when managing large catalogs with varying pricing tiers for different customer groups.
How Can I Ensure I Retrieve Accurate Tier Price Data?
To ensure accurate tier price retrieval, make sure that:
- The product SKUs are correct and match the catalog data.
- The tier prices are properly set up in the admin panel for each product.
- The
TierPriceStorageInterface
is correctly injected into your class.
How Do I Handle Errors When Retrieving Tier Prices?
If an error occurs when retrieving tier prices, ensure to log the error for troubleshooting. This can be done by using a LoggerInterface
and capturing the exception message. Make sure the SKUs passed are valid and exist in your product catalog.