How to Check Product Stock Status Programmatically in Magento 2
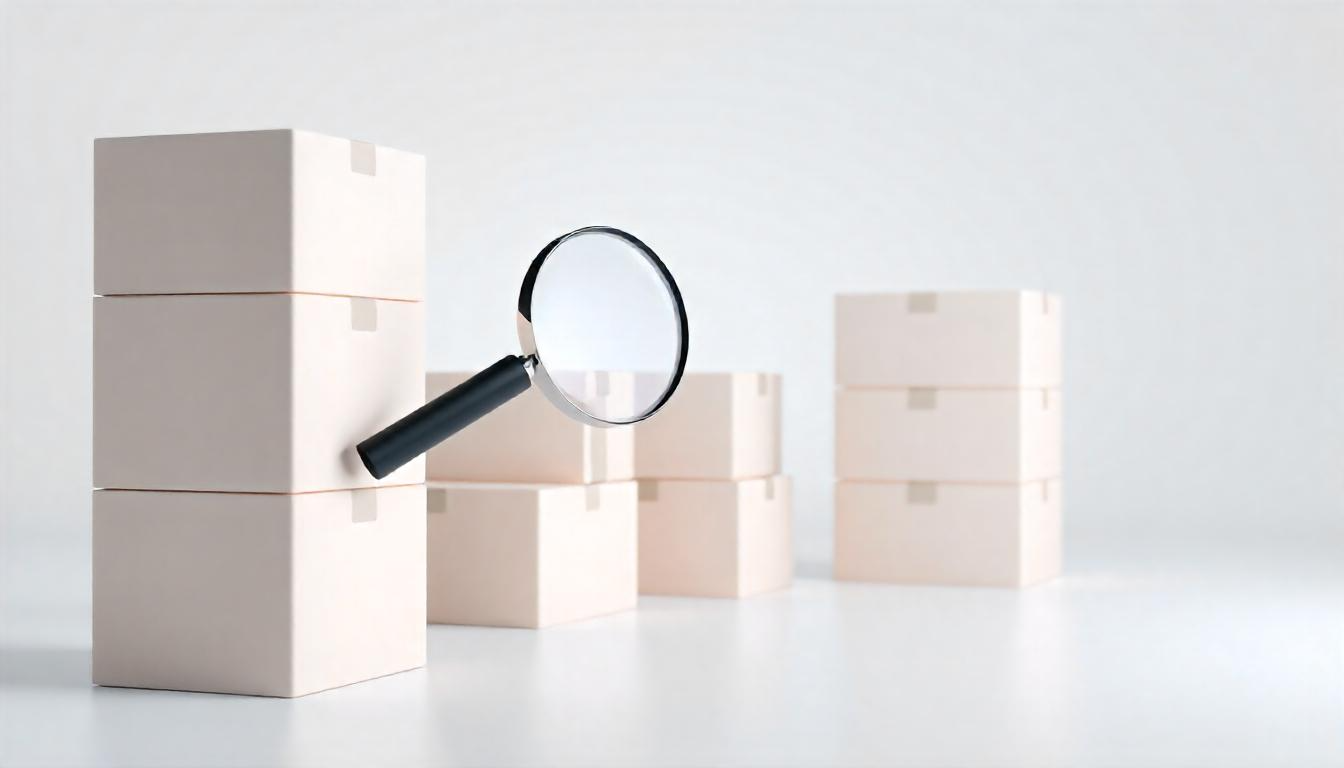
How to Check Product Stock Status Programmatically in Magento 2
When developing in Magento 2, you might need to programmatically determine whether a product is in stock or out of stock. This can be achieved using the StockRegistryInterface
class, which allows you to fetch product stock status effectively.
Table Of Content
- Comprehensive Guide to Stock Management in Magento 2
- How to Check Product Stock Status Programmatically in Magento 2
- Practical Applications of Stock Status Checking in Magento 2
- Practical Examples for Fetching Stock Status in Magento 2
- Troubleshooting Common Stock Status Issues in Magento 2
- Conclusion
- FAQs
Comprehensive Guide to Stock Management in Magento 2
Magento 2 features a robust inventory management system designed to streamline stock tracking and ensure accurate product availability across your store. This system monitors stock status and quantity, ensuring customers have a seamless shopping experience.
Key Components of Stock Management
Stock Status
Stock status indicates whether a product is In Stock or Out of Stock. This status is automatically determined by various factors:
- Product Quantity: Ensures available stock is above the threshold set in configurations.
- Admin-Defined Status: Overrides automatic settings for specific scenarios.
- Backorder Settings: Allows products with zero quantity to be sold based on admin configurations.
Quantity Management
Magento 2 tracks the precise quantity for each product, updating it in real time as orders are placed, canceled, or modified. This ensures your inventory data remains accurate.
Advanced Configurations
Magento 2 offers flexible configuration settings to manage stock behavior:
- Backorders: Enable selling of out-of-stock products to maintain sales momentum.
- Threshold Limits: Define minimum stock levels to trigger notifications for restocking.
- Multi-Source Inventory (MSI): Manage stock across multiple warehouses and sales channels.
Why Stock Management Matters
- Improved Customer Experience: Real-time stock updates ensure customers only see products available for purchase.
- Operational Efficiency: Accurate stock tracking minimizes errors in order fulfillment and reduces operational costs.
- Scalability: Advanced features like MSI enable businesses to grow while maintaining seamless inventory management.
By leveraging Magento 2’s inventory management capabilities, you can optimize your store’s operations, enhance customer trust, and stay ahead in competitive markets.
How to Check Product Stock Status Programmatically in Magento 2
In Magento 2 development, it's common to programmatically check whether a product is in stock or out of stock. This functionality is essential for tasks like custom extensions, dynamic inventory updates, or even integrating third-party systems. The StockRegistryInterface
class is a powerful tool that allows developers to fetch stock-related data effectively and with minimal effort.
Why Programmatically Check Stock Status?
Checking stock status programmatically in Magento 2 is useful for:
- Custom Features: Build unique features like custom stock badges or notifications.
- Third-Party Integrations: Sync inventory status with external systems like CRMs or ERPs.
- Custom Cart Rules: Restrict the addition of out-of-stock products to the cart.
- Dynamic Updates: Create real-time updates in custom dashboards or frontend displays.
Using StockRegistryInterface to Fetch Stock Status
Magento provides the Magento\CatalogInventory\Api\StockRegistryInterface
class to retrieve product stock information. Here's a step-by-step guide:
Class | Purpose |
---|---|
StockRegistryInterface | Fetch stock details for specific products. |
StockItemInterface | Provides stock item data. |
1. Create a Custom Model
Define a model class that uses StockRegistryInterface
to get the stock status of a product.
<?php
namespace Jesadiya\StockStatus\Model;
use Magento\CatalogInventory\Api\StockRegistryInterface;
use Magento\CatalogInventory\Api\Data\StockItemInterface;
class Data
{
/**
* @var StockRegistryInterface|null
*/
private $stockRegistry;
/**
* Constructor
*
* @param StockRegistryInterface $stockRegistry
*/
public function __construct(
StockRegistryInterface $stockRegistry
) {
$this->stockRegistry = $stockRegistry;
}
/**
* Get Stock Status
*
* @param int $productId
* @return bool
*/
public function getStockStatus($productId)
{
/** @var StockItemInterface $stockItem */
$stockItem = $this->stockRegistry->getStockItem($productId);
$isInStock = $stockItem ? $stockItem->getIsInStock() : false;
return $isInStock;
}
}
Pro Tip: Optimize Your Custom Model for Scalability
When creating a custom model to retrieve stock status, consider adding error handling and logging mechanisms. This ensures your model remains robust in production and provides valuable insights during debugging.
2. Call the Method
You can call the getStockStatus
method by passing the product ID as a parameter.
$productId = 1; // Replace with the actual product ID
$result = $this->getStockStatus($productId);
if ($result) {
echo "Product is in stock.";
} else {
echo "Product is out of stock.";
}
Pro Tip: Ensure Accurate and Reliable Method Calls
When calling the getStockStatus
method, ensure the process is streamlined for accurate results and better performance.
How Stock Status Works
Condition | Stock Status |
---|---|
Quantity > 0, Stock Status = "In Stock" | true |
Quantity ≤ 0, Stock Status = "Out of Stock" | false |
StockRegistryInterface:
This interface retrieves the stock item details for the given product ID.getStockItem($productId):
Returns theStockItemInterface
object, which contains stock-related details.- Check Stock Status:
- If the product quantity is greater than 0, or the stock status is explicitly set to "In Stock" in the admin panel, the method will return
true
. - Otherwise, it will return
false
.
- If the product quantity is greater than 0, or the stock status is explicitly set to "In Stock" in the admin panel, the method will return
Practical Applications of Stock Status Checking in Magento 2
Effectively managing stock information programmatically can unlock numerous possibilities for enhancing your store's functionality and user experience. Here are some common use cases:
1. Validate Stock Before Orders
Ensure that customers can only place orders for products with sufficient inventory. This is particularly useful for:
- Preventing Backorders: Automatically restrict orders for out-of-stock products.
- Bulk Order Management: Validate stock quantities for bulk orders before they are processed.
- Custom Cart Rules: Block the addition of out-of-stock products to the cart and display appropriate error messages.
2. Real-Time Stock Display on the Front-End
Leverage stock data to create a dynamic shopping experience:
- Stock Status on Product Pages: Show "In Stock," "Low Stock," or "Out of Stock" indicators dynamically.
- Category Listings: Add stock badges or labels to product cards in category views.
- Urgency Features: Display messages like "Only 3 left in stock" to encourage faster purchases.
3. Enhance Custom APIs for Third-Party Integrations
Utilize stock data in APIs to sync inventory with external systems, such as:
- Enterprise Resource Planning (ERP): Ensure consistent inventory updates between Magento and ERP systems.
- Marketplace Integrations: Synchronize stock levels with platforms like Amazon, eBay, or Walmart.
- Dropshipping Services: Share accurate stock data with dropshipping vendors in real-time.
4. Generate Advanced Inventory Reports
Programmatically analyze stock data to drive business decisions:
- Trend Analysis: Identify fast-moving or slow-moving products.
- Restocking Alerts: Automatically generate reports for products that need to be restocked.
- Custom Dashboards: Build dashboards to monitor stock levels across multiple categories or locations.
- Profitability Insights: Correlate stock trends with sales data to identify high-performing products.
Summary of Applications
Application Area | Use Case | Key Benefits |
---|---|---|
Order Validation | Prevent backorders, validate bulk orders, and enforce cart rules. | Ensures a seamless checkout experience and minimizes customer dissatisfaction. |
Front-End Display | Show real-time stock status, add urgency messages, and dynamic labels. | Enhances user experience and drives conversions by creating a sense of urgency. |
Custom API Enhancements | Sync stock data with ERP systems, marketplaces, or dropshipping platforms. | Enables accurate and real-time inventory synchronization across multiple channels. |
Inventory Reports | Generate trends, restocking alerts, and profitability insights. | Provides actionable insights for better inventory management and business decisions. |
Warehouse Management | Optimize storage and reduce inventory costs by using stock data in warehouse planning. | Improves operational efficiency and reduces overhead costs. |
Multi-Source Inventory | Manage inventory across multiple warehouses with Magento's MSI feature. | Ensures accurate stock allocation and faster delivery for multi-location businesses. |
By programmatically accessing and utilizing stock information, you can enhance the efficiency and capabilities of your Magento 2 store while providing a better shopping experience for customers.
Practical Examples for Fetching Stock Status in Magento 2
To demonstrate the various ways of utilizing Magento 2's stock management system programmatically, here are a few practical examples that you can use to integrate stock status fetching into your store's functionality.
Example 1: Fetch Stock for Multiple Products
To check the stock status for a list of products, you can loop through the product IDs and fetch their stock status dynamically. This is particularly useful for bulk stock checks in custom modules or reports.
$productIds = [1, 2, 3, 4, 5]; // Array of product IDs
foreach ($productIds as $productId) {
$status = $this->getStockStatus($productId); // Fetch stock status for each product
echo "Product ID: $productId is " . ($status ? "In Stock" : "Out of Stock") . "<br>";
}
Explanation:
- This example demonstrates a simple loop where stock status for each product ID is fetched and displayed.
- You can easily modify the
$productIds
array to include any number of products. - The
getStockStatus()
method (which can be custom-defined) retrieves whether the product is in stock (true
) or out of stock (false
).
Example 2: Extend API Response with Stock Data
You can add the stock status to an API response for custom integrations. For example, when building an API that returns product details, including the stock status is useful for external systems like mobile apps, marketplaces, or ERP systems.
$response = [
'product_id' => $productId,
'product_name' => $product->getName(), // Fetch the product name
'price' => $product->getPrice(), // Fetch the product price
'is_in_stock' => $this->getStockStatus($productId), // Add stock status to the response
];
return $response;
Explanation:
- This example showcases how to include stock information within an API response.
- The response not only contains the
product_id
., but alsoproduct_name
,price
, andis_in_stock
status. - You can extend this further by adding more product attributes like SKU, description, and category.
Example 3: Dynamic Front-End Display of Stock Status
You can use stock status dynamically on the front-end to notify customers whether a product is available. This example fetches the stock status of a product and displays it on a product page.
$productId = 101; // Replace with actual product ID
$status = $this->getStockStatus($productId);
if ($status) {
echo "This product is in stock!";
} else {
echo "Sorry, this product is currently out of stock.";
}
Explanation:
- This is a simple PHP script to dynamically display stock availability on product pages.
- It can be enhanced by adding styling and functionality, such as displaying "Low Stock" when there are only a few units remaining.
Example 4: Stock Validation Before Adding to Cart
Before allowing a customer to add a product to their cart, you might want to check its stock status. Here's how to validate stock before adding it to the cart.
$productId = 202; // Replace with actual product ID
$quantity = 2; // Desired quantity for the cart
$status = $this->getStockStatus($productId);
if ($status && $this->checkProductStock($productId, $quantity)) {
echo "Product added to cart!";
} else {
echo "Sorry, this product is out of stock or insufficient quantity.";
}
Explanation:
- This example checks both if the product is in stock and if there is enough inventory available for the requested quantity before adding it to the cart.
- The method
checkProductStock()
would verify if the desired quantity is available.
Example 5: Generate Custom Inventory Report with Stock Status
You can generate a custom report that lists product IDs, names, and stock statuses for inventory analysis.
$productCollection = $this->productRepository->getList($searchCriteria); // Fetch all products
foreach ($productCollection->getItems() as $product) {
$stockStatus = $this->getStockStatus($product->getId()); // Fetch stock status for each product
echo "Product: " . $product->getName() . " | Stock Status: " . ($stockStatus ? "In Stock" : "Out of Stock") . "<br>";
}
Explanation:
- In this example, we fetch all products using a repository or model and then check the stock status for each product.
- The custom report can be extended by including more product details or exporting the data to a CSV or PDF format for business use.
These practical examples demonstrate how to leverage Magento 2's stock management system in various scenarios, including bulk stock checks, API responses, front-end displays, cart validation, and custom reports. By programmatically fetching stock status, you can enhance user experiences and ensure smoother workflows in your store.
Troubleshooting Common Stock Status Issues in Magento 2
When dealing with stock status programmatically in Magento 2, you might run into a few common issues. Below is a detailed breakdown of these issues along with their possible causes and resolutions.
Common Stock Issues and Their Resolutions
Issue | Possible Causes | Resolution |
---|---|---|
Product Always Returns False Stock Status |
|
|
Stock Data Not Updating Immediately |
|
|
API Returns Null for Stock Data |
|
|
Stock Status Inaccurate on Front-End |
|
|
Magento Admin Shows Correct Stock, API Shows Incorrect Data |
|
|
API Returns Incorrect Stock Quantity |
|
|
Pro Tip:
Ensure accurate stock management in Magento 2 by regularly auditing settings, automating tasks like reindexing and cache flushing, and validating APIs. Use Magento CLI tools to debug and resolve issues quickly. Periodically review database tables to detect and correct stock discrepancies.
Magento 2 stores rely on accurate stock management to ensure smooth operations. By understanding and addressing these common stock-related issues, you can maintain accurate inventory data across your store and APIs.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Conclusion
Mastering how to programmatically check a product's stock status in Magento 2 is a fundamental skill for developers building robust and scalable eCommerce solutions. By utilizing the StockRegistryInterface
and StockItemInterface
, you gain direct access to critical stock data, empowering you to create more dynamic and responsive functionalities tailored to your store's needs.
The ability to programmatically determine if a product is "In Stock" or "Out of Stock" has numerous applications, such as:
- Improving User Experience: Display real-time stock information to customers, ensuring transparency and reducing cart abandonment.
- Automating Inventory Management: Streamline backend workflows by triggering specific actions based on stock status.
- Personalized Marketing: Create alerts, recommendations, or promotions for low-stock or back-in-stock products.
- Optimizing Store Performance: Efficiently integrate stock status checks in custom modules to minimize manual overhead and errors.
With clear steps and practical examples provided in this guide, implementing these features in Magento 2 becomes a seamless task. By coupling technical precision with strategic planning, you can ensure your store operates efficiently, supports customer satisfaction, and drives better business outcomes.
Incorporating programmatic stock checks is not just about development—it’s about aligning your technical solutions with business goals to create a truly effective Magento 2 store.
FAQs
What is the purpose of StockRegistryInterface in Magento 2?
StockRegistryInterface in Magento 2 is used to fetch stock details for specific products programmatically.
What does StockItemInterface provide in Magento 2?
StockItemInterface provides detailed stock item data, such as quantity and stock status, for a product.
How can I check the stock status of a product programmatically in Magento 2?
You can use the StockRegistryInterface to retrieve the stock status of a product by passing its product ID to the getStockItem method.
What is the output of the getStockStatus method if a product is in stock?
If a product is in stock, the getStockStatus method will return "true".
What conditions determine a product's stock status in Magento 2?
If the product quantity is greater than 0 and the stock status is set to "In Stock", the product will be considered in stock.
Can I use the stock status programmatically in custom modules?
Yes, the stock status can be integrated into custom modules to automate inventory management and enhance store functionality.
What happens if a product quantity is 0 in Magento 2?
If a product's quantity is 0, its stock status will be set to "Out of Stock" by default unless overridden.
Where can I use the stock status information in a Magento 2 store?
Stock status information can be used on the storefront, in backend workflows, or for personalized marketing campaigns.
What are the benefits of programmatically checking stock status?
Programmatically checking stock status helps automate inventory management, improve user experience, and optimize performance.
Is it possible to override the stock status programmatically?
Yes, you can override stock status programmatically by customizing the logic within the StockRegistryInterface or related methods.
What is the significance of using StockRegistryInterface in custom Magento 2 modules?
Using StockRegistryInterface ensures precise and real-time stock information, which is essential for accurate inventory management in custom modules.
How does stock status impact customer experience?
Displaying accurate stock status improves customer trust, reduces frustration, and enhances the overall shopping experience.
What command should I run after making changes to stock status settings?
You should run the bin/magento cache:clear
command to reflect the changes on the frontend of the store.