Retrieving Child Product IDs for Configurable and Bundle Products in Magento 2
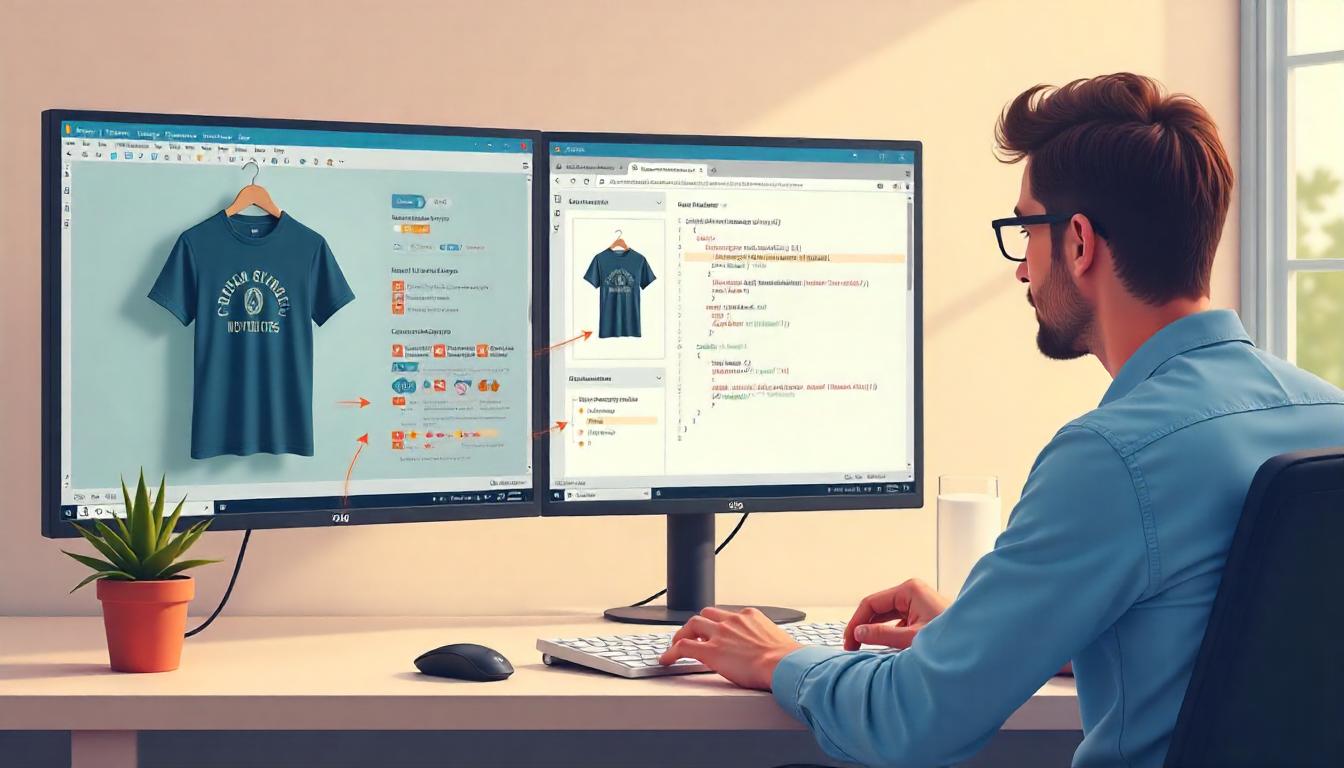
Retrieving Child Product IDs for Configurable and Bundle Products in Magento 2
Retrieving child product IDs in Magento 2 is essential for managing configurable and bundle products effectively. Configurable products link to multiple simple (child) products, while bundle products consist of multiple selectable items. This guide covers how to fetch child product IDs using Magento’s built-in methods, including the Product Repository Interface, Product Link Management Interface, and Type Instance methods.
Retrieving Child Product IDs for Configurable and Bundle Products in Magento 2
In Magento 2, obtaining the IDs of child products associated with configurable and bundle products is straightforward. Here's how you can achieve this:
1. Fetching Child Product IDs for Configurable Products
Configurable products are parent items that link to simple (child) products. To retrieve the IDs of these child products:
Using the Product Repository Interface:
<?php
namespace YourNamespace\YourModule\Model;
use Magento\Catalog\Api\ProductRepositoryInterface;
class ConfigurableProductHelper
{
/**
* @var ProductRepositoryInterface
*/
private $productRepository;
public function __construct(ProductRepositoryInterface $productRepository)
{
$this->productRepository = $productRepository;
}
/**
* Get child product IDs for a configurable product
*
* @param int $configurableProductId
* @return array
*/
public function getChildProductIds(int $configurableProductId): array
{
$configurableProduct = $this->productRepository->getById($configurableProductId);
return $configurableProduct->getExtensionAttributes()->getConfigurableProductLinks();
}
}
</div>
In this code, replace YourNamespace\YourModule
with your actual namespace and module name. The getChildProductIds
method returns an array of child product IDs associated with the specified configurable product.
2. Fetching Child Product IDs for Bundle Products
Bundle products consist of multiple child items. To retrieve their IDs:
Using the Product Link Management Interface:
<?php
namespace YourNamespace\YourModule\Model;
use Magento\Bundle\Api\ProductLinkManagementInterface;
class BundleProductHelper
{
/**
* @var ProductLinkManagementInterface
*/
private $productLinkManagement;
public function __construct(ProductLinkManagementInterface $productLinkManagement)
{
$this->productLinkManagement = $productLinkManagement;
}
/**
* Get child product IDs for a bundle product
*
* @param int $bundleProductId
* @return array
*/
public function getChildProductIds(int $bundleProductId): array
{
return $this->productLinkManagement->getChildren($bundleProductId);
}
}
Here, getChildProductIds
returns an array of child product IDs for the specified bundle product.
3. Fetching Child Product IDs for Bundle Products (Alternative Method)
Alternatively, you can use the product's type instance to get child product IDs:
Using the Product Type Instance:
<?php
namespace YourNamespace\YourModule\Model;
use Magento\Catalog\Model\Product;
use Magento\Bundle\Model\Product\Type as BundleType;
class BundleProductHelper
{
/**
* Get child product IDs for a bundle product
*
* @param Product $bundleProduct
* @return array
*/
public function getChildProductIds(Product $bundleProduct): array
{
if (!$bundleProduct->getTypeId() === BundleType::TYPE_CODE) {
return [];
}
/** @var \Magento\Bundle\Model\Product\Type $typeInstance */
$typeInstance = $bundleProduct->getTypeInstance();
return $typeInstance->getChildrenIds($bundleProduct->getId()) ?? [];
}
}
In this approach, getChildProductIds
retrieves child product IDs for the given bundle product.
Note: Ensure that the product IDs you provide are valid and correspond to existing products in your Magento store.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
How Can I Retrieve Child Product IDs for a Configurable Product in Magento 2?
You can retrieve child product IDs of a configurable product using the getConfigurableProductLinks
method from the configurable product's extension attributes.
What Code Can I Use to Get Child Product IDs for a Configurable Product?
Here’s a PHP snippet to fetch child product IDs of a configurable product:
namespace YourNamespace\YourModule\Model;
use Magento\Catalog\Api\ProductRepositoryInterface;
class ConfigurableProductHelper
{
private $productRepository;
public function __construct(ProductRepositoryInterface $productRepository)
{
$this->productRepository = $productRepository;
}
public function getChildProductIds(int $configurableProductId): array
{
$configurableProduct = $this->productRepository->getById($configurableProductId);
return $configurableProduct->getExtensionAttributes()->getConfigurableProductLinks();
}
}
How Can I Retrieve Child Product IDs for a Bundle Product in Magento 2?
You can retrieve child product IDs of a bundle product using the ProductLinkManagementInterface
service in Magento 2.
Is There an Alternative Method to Get Child Product IDs for a Bundle Product?
Yes, you can also use the product type instance method getChildrenIds
to retrieve child product IDs for a bundle product.
What Is the Code to Fetch Child Product IDs Using the Product Type Instance?
Here’s a PHP snippet to fetch child product IDs using the product type instance:
namespace YourNamespace\YourModule\Model;
use Magento\Catalog\Model\Product;
class BundleProductHelper
{
public function getChildProductIds(Product $bundleProduct): array
{
$typeInstance = $bundleProduct->getTypeInstance();
return $typeInstance->getChildrenIds($bundleProduct->getId());
}
}
Where Are Child Product IDs Stored in Magento 2?
Child product IDs for configurable and bundle products are stored in different database tables. Configurable product links are found in catalog_product_relation
, while bundle product links are stored in catalog_product_bundle_selection
.