Retrieve Order Status Comments by Comment ID in Magento 2
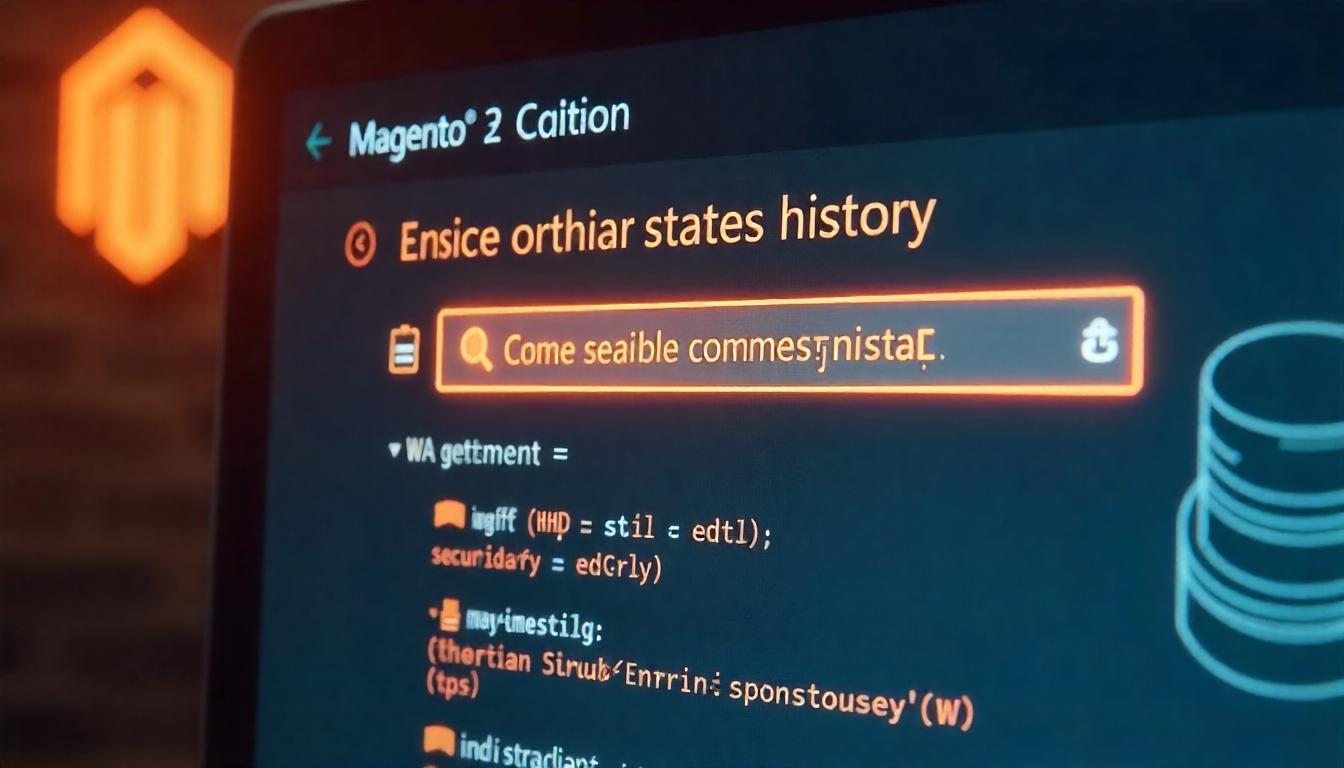
Retrieve Order Status Comments by Comment ID in Magento 2
To retrieve an order status comment using its comment ID in Magento 2, use the OrderStatusHistoryRepositoryInterface. This method allows you to fetch specific status history details, including the comment text, status, and creation time.
Table Of Content
Retrieve Order Status Comments by Comment ID in Magento 2
To fetch a specific order status comment in Magento 2 using its comment ID, utilize the get($commentId)
method from the OrderStatusHistoryRepositoryInterface.
This approach ensures you access the desired status history data efficiently.
Implementation Steps:
Set Up Dependencies:
- Inject the OrderStatusHistoryRepositoryInterface into your class.
- Incorporate a logger to handle potential exceptions.
Create the Method:
- Develop a function that accepts the comment ID as a parameter.
- Use the repository's get method to retrieve the order status comment.
- Implement exception handling to manage errors gracefully.
Sample Code:
<?php
namespace YourNamespace\YourModule\Model;
use Magento\Sales\Api\Data\OrderStatusHistoryInterface;
use Magento\Sales\Api\OrderStatusHistoryRepositoryInterface;
use Psr\Log\LoggerInterface;
class OrderStatusCommentLoader
{
private $historyRepository;
private $logger;
public function __construct(
OrderStatusHistoryRepositoryInterface $historyRepository,
LoggerInterface $logger
) {
$this->historyRepository = $historyRepository;
$this->logger = $logger;
}
/**
* Load order status comment by comment ID.
*
* @param int $commentId
* @return OrderStatusHistoryInterface|null
*/
public function loadCommentById(int $commentId): ?OrderStatusHistoryInterface
{
try {
return $this->historyRepository->get($commentId);
} catch (\Exception $exception) {
$this->logger->error('Error loading order status comment: ' . $exception->getMessage(), [
'exception' => $exception->getTraceAsString()
]);
return null;
}
}
}
Usage:
$commentId = 1;
$orderStatusComment = $this->loadCommentById($commentId);
if ($orderStatusComment !== null) {
// Access methods from OrderStatusHistoryInterface
$comment = $orderStatusComment->getComment() ?? 'No comment';
$status = $orderStatusComment->getStatus() ?? 'Unknown status';
// Additional processing as needed
} else {
// Handle the case where the comment was not found
echo 'Order status comment not found.';
}
Key Considerations:
Alternatively, you can use Magento's API to delete a comment:
- Ensure the $commentId provided is valid and exists in your database.
- Handle exceptions appropriately to maintain system stability.
- This method retrieves the OrderStatusHistoryInterface object, allowing access to various details like comment text, status, creation time, and more.
By following this approach, you can effectively retrieve specific order status comments in Magento 2 using their comment IDs.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
How Can I Retrieve an Order Status Comment by Comment ID in Magento 2?
To fetch a specific order status comment using its comment ID, use the get($commentId)
method from OrderStatusHistoryRepositoryInterface
. This retrieves the status history data efficiently.
Why Would I Need to Load an Order Status Comment by Comment ID?
Loading an order status comment by its ID is useful when you need to display specific order history details, track order updates, or troubleshoot issues related to order status changes.
What Magento 2 Interface Is Used to Retrieve Order Status Comments?
The OrderStatusHistoryRepositoryInterface
in Magento 2 provides methods to retrieve order status comments programmatically using their comment ID.
What Is the Code to Retrieve an Order Status Comment by Comment ID?
Here’s an example of how to retrieve an order status comment using its ID in Magento 2:
namespace YourNamespace\YourModule\Model;
use Magento\Sales\Api\Data\OrderStatusHistoryInterface;
use Magento\Sales\Api\OrderStatusHistoryRepositoryInterface;
use Psr\Log\LoggerInterface;
class OrderStatusCommentLoader
{
private $historyRepository;
private $logger;
public function __construct(
OrderStatusHistoryRepositoryInterface $historyRepository,
LoggerInterface $logger
) {
$this->historyRepository = $historyRepository;
$this->logger = $logger;
}
public function loadCommentById(int $commentId): ?OrderStatusHistoryInterface
{
try {
return $this->historyRepository->get($commentId);
} catch (\Exception $exception) {
$this->logger->error('Error loading order status comment: ' . $exception->getMessage());
return null;
}
}
}
How Can I Use the Retrieved Order Status Comment Data?
Once retrieved, you can access various details from the OrderStatusHistoryInterface
, such as comment text, order status, and creation date.
What Happens If the Comment ID Is Invalid?
If the comment ID doesn’t exist, Magento throws an exception. To prevent this, wrap the retrieval code in a try-catch block and handle errors appropriately.
Can I Retrieve Multiple Order Status Comments at Once?
Yes, you can fetch multiple order status comments by loading them as a collection instead of fetching them one by one using individual IDs.
How Do I Debug Issues with Order Status Comment Retrieval?
Check the Magento logs for errors. Ensure that the provided comment ID exists in the database and that the repository is correctly injected and used in your code.
Is It Possible to Modify Retrieved Order Status Comments?
Yes, after retrieving the comment object, you can modify its values and save the changes using the save
method of OrderStatusHistoryRepositoryInterface
.