How to Get Product Canonical URL by ID Programmatically in Magento 2
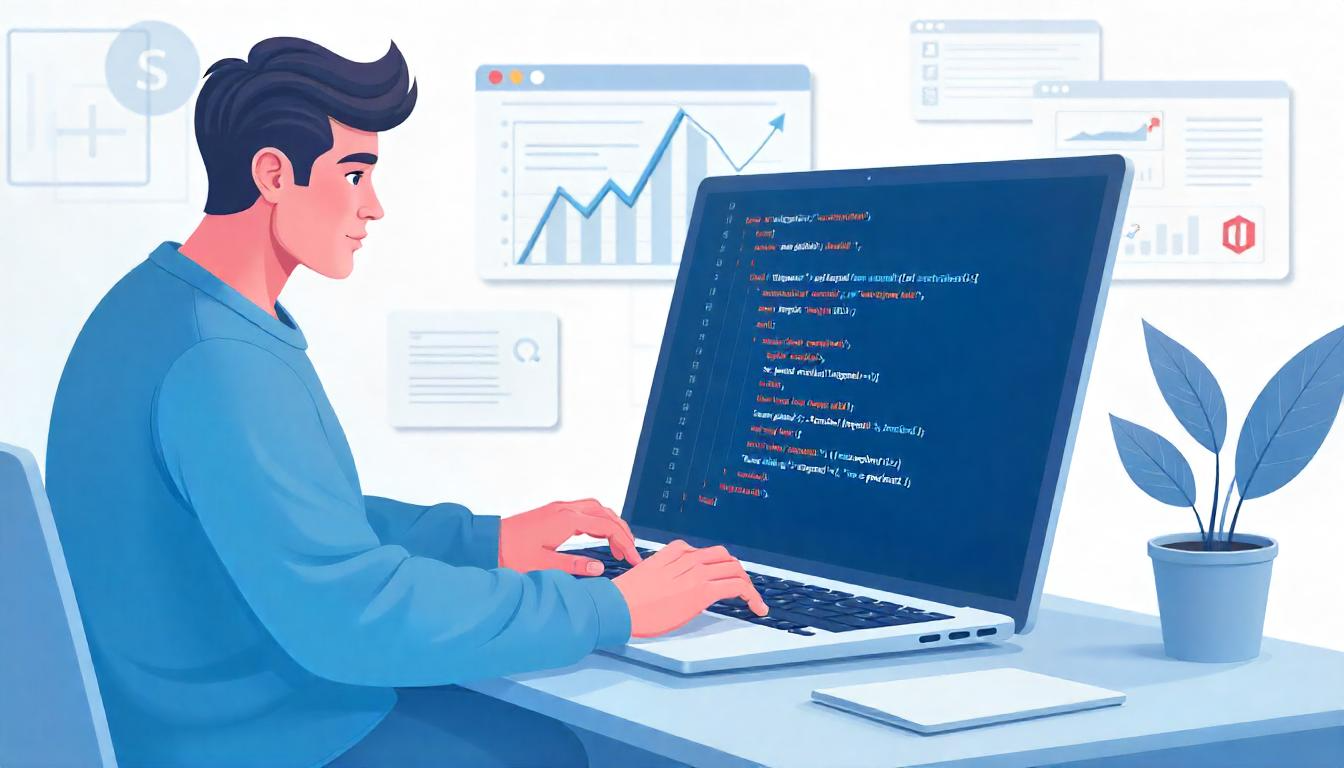
How to Get Product Canonical URL by ID Programmatically in Magento 2
In Magento 2, a Canonical URL helps prevent duplicate content issues by specifying the preferred URL for a product page. If you need to fetch the Product Canonical URL programmatically using a product ID, you can use Magento's repository methods.
This guide will show you how to retrieve the Canonical URL dynamically for a product in the current store.
Table Of Content
- Understanding Canonical URLs in Magento 2
- Fetching Product Canonical URL in Magento 2
- Alternative Approach: Using Object Manager (Not Recommended)
- Adding Canonical URLs in XML Layout for SEO (Good for SEO)
- Comparison of Methods for Fetching Canonical URLs in Magento 2
- Best Practices for Managing Canonical URLs in Magento 2
- Conclusion
- FAQs
Understanding Canonical URLs in Magento 2
Canonical URLs are a crucial part of Magento 2’s SEO framework. They define the preferred version of a page when multiple URLs lead to identical or similar content. This helps search engines consolidate ranking signals and prevents duplicate content issues, which can negatively impact SEO.
What Are Canonical URLs?
A canonical URL is an HTML tag (<link rel="canonical" href="https://example.com/product-name" /> ) that tells search engines which version of a webpage should be indexed as the primary source. This is particularly useful for eCommerce websites where product pages may have multiple variations due to filters, sorting, and session-based parameters.
Example of Canonical URL Implementation
<link rel="canonical" href="https://example.com/product-name" />
Common URL Variations Without a Canonical Tag
URL Variant | Issue Without Canonical Tag |
---|---|
example.com/product-name | Preferred URL |
example.com/product-name?color=red | Treated as a duplicate by search engines |
example.com/category/product-name | Can dilute ranking authority |
Tip
Always set the canonical tag (<link rel="canonical" href="https://example.com/product-name" />
) on product pages to prevent search engines from indexing duplicate URLs. This helps consolidate ranking signals and improves SEO performance.
Without proper canonicalization, search engines might treat these as separate pages, leading to content duplication penalties and lower rankings.
Why Are Canonical URLs Important in Magento 2?
Key Benefits of Using Canonical URLs
Prevents Duplicate Content Issues
Search engines avoid indexing multiple versions of the same page, reducing penalties for duplicate content.
Improves Search Rankings
By consolidating link equity to a single authoritative URL, canonical tags help boost SEO rankings.
Ensures a Consistent URL Structure
Canonical tags direct users and search engines to the correct URL, providing a better user experience.
Optimizes Crawl Budget
Search engines avoid wasting crawl resources on duplicate pages, focusing on more valuable content instead.
Enhances Internal Linking Efficiency
All internal and external backlinks contribute to a single, preferred URL rather than being split among duplicate pages.
Fetching Product Canonical URL in Magento 2
Why Fetch Canonical URLs in Magento 2?
Canonical URLs help prevent duplicate content issues by directing search engines to the preferred URL version of a product page. Fetching these URLs programmatically allows you to manage SEO dynamically across your store.
Steps to Retrieve a Product’s Canonical URL via ProductRepositoryInterface (Recommended)
Step 1: Create a Custom Model Class
Create a custom model file to fetch the canonical URL of a product dynamically.
PHP Code:
<?php
namespace Vendor\Module\Model;
use Magento\Catalog\Api\ProductRepositoryInterface;
use Magento\Framework\Exception\NoSuchEntityException;
use Psr\Log\LoggerInterface;
class CanonicalUrl {
private $productRepository;
private $logger;
public function __construct(
ProductRepositoryInterface $productRepository,
LoggerInterface $logger
) {
$this->productRepository = $productRepository;
$this->logger = $logger;
}
public function getCanonicalUrl(int $productId): ?string {
try {
$product = $this->productRepository->getById($productId);
return $product->getUrlInStore();
} catch (NoSuchEntityException $exception) {
$this->logger->error("Product ID not found: " . $productId, [$exception]);
return null;
}
}
}
Step 2: Use the Canonical URL Function in Magento 2
After creating the model class, call it in a block, helper, or controller.
Example Usage in a Block or Helper
$canonicalUrl = $this->canonicalUrl->getCanonicalUrl(42);
echo "Canonical URL: " . $canonicalUrl;
This will return the canonical URL of the specified product.
Expected Output
Canonical URL: https://yourstore.com/sample-product
Comparison of URL Variants and Canonical Tag Impact
URL Variant | Issue Without Canonical Tag |
---|---|
example.com/product-name | Preferred URL |
example.com/product-name?color=red | Treated as a duplicate by search engines |
example.com/category/product-name | Can dilute ranking authority |
Tip
Regularly audit your product pages to check if the correct canonical URLs are implemented. This ensures that no unnecessary or duplicate pages are indexed by search engines, helping maintain the integrity of your SEO strategy.
Alternative Approach: Using Object Manager (Not Recommended)
In Magento 2, it is possible to use the Object Manager to retrieve objects like the ProductRepositoryInterface
. However, this approach is not recommended because it breaks best practices and violates key principles of object-oriented design, such as dependency injection. The Object Manager is primarily intended for internal use by the Magento framework, and its use in custom code can lead to several issues.
Example Using Object Manager
$objectManager = \Magento\Framework\App\ObjectManager::getInstance();
$productRepository = $objectManager->get(\Magento\Catalog\Api\ProductRepositoryInterface::class);
$product = $productRepository->getById(42);
echo $product->getUrlInStore();
Why Avoid Using the Object Manager?
The Object Manager should be avoided for the following reasons:
Hard to Test in Unit Tests
Since it bypasses dependency injection, it makes your code harder to unit test, which can lead to difficulties during development and maintenance.
Breaks Dependency Injection Principles
Magento 2 heavily relies on dependency injection (DI) for managing class dependencies. Using the Object Manager goes against this core principle, making your code less maintainable and more prone to errors.
Slower Performance
Using the Object Manager can introduce performance overhead because it dynamically resolves dependencies during runtime, unlike DI, which resolves them at compile time.
Reduced Code Readability and Flexibility
Code that uses the Object Manager is harder to read and extend. It also creates tight coupling between classes, making it more difficult to modify your code in the future.
Best Practice
Instead of using the Object Manager, always use dependency injection. Magento 2 encourages the use of constructor-based injection, which leads to cleaner, more maintainable code.
Tip
Always use dependency injection to retrieve services like the ProductRepositoryInterface
. This promotes better code maintainability, improves performance, and ensures that your code is testable. Avoiding the Object Manager ensures your code adheres to Magento 2’s best practices and can be more easily extended in the future.
Why Object Manager Is Not Recommended
Reason | Explanation |
---|---|
Hard to Test | Code becomes difficult to test in unit tests due to lack of control over object instantiation. |
Breaks Dependency Injection | Violates the core principle of dependency injection, leading to tightly coupled and harder-to-maintain code. |
Slower Performance | Using the Object Manager can slow down the application, as dependencies are resolved at runtime instead of compile time. |
Reduced Readability and Flexibility | Code using the Object Manager is harder to read and modify, reducing the flexibility for future changes. |
Adding Canonical URLs in XML Layout for SEO (Good for SEO)
To ensure Canonical URLs are automatically included in the <head>
section of product pages, modify the catalog_product_view.xml
file. This method ensures the correct product page URL is referenced, preventing search engines from indexing duplicate content across various URL variants.
XML Code Example:
You can add the following code snippet in the catalog_product_view.xml
layout file to include the canonical tag:
<referenceBlock name="head.additional">
<block class="Magento\Framework\View\Element\Template"
name="canonical.url"
template="Magento_Catalog::seo/canonical.phtml"/>
</referenceBlock>
Generated Output in Page Source:
After implementing the code, the canonical URL will appear in the page's HTML source code as shown below:
<link rel="canonical" href="https://yourstore.com/sample-product"/>
This automatically sets the canonical URL for the product page and helps search engines index the correct version of the page, preventing duplicate content penalties
Tip
When implementing canonical URLs, make sure the tag is present for all pages that may have multiple URL variations due to parameters like sorting, filtering, or session IDs. This is particularly useful for product pages that can be accessed via different URLs due to these parameters.
For example:
- If a product page is accessible with various parameters like color, size, or stock level, the canonical tag should point to the main product page to ensure proper indexing.
By doing this, you prevent search engines from treating different product URL variants as separate pages, which could dilute the page’s SEO value.
Comparison of Methods for Fetching Canonical URLs in Magento 2
When dealing with Canonical URLs in Magento 2, it's essential to choose the most efficient and best-practice method for fetching and rendering these URLs. Depending on the method you choose, there are differences in performance, ease of use, and whether it's considered a best practice for your Magento store.
Here’s a detailed comparison of the most common methods for fetching Canonical URLs:
Method | Performance | Best Practice | Ease of Use | Notes |
---|---|---|---|---|
Using ProductRepositoryInterface | Fast | Recommended | Easy | This is the most efficient and recommended method for retrieving Canonical URLs. It ensures good performance and follows Magento’s dependency injection principles. |
Using Object Manager | Slow | Not Recommended | Simple but poor practice | Although it's simple to implement, using the Object Manager violates Magento's dependency injection principles and can lead to poor performance and hard-to-test code. |
Modifying XML Layout | Medium | Good for Frontend SEO | Requires XML Knowledge | This approach is beneficial for automatically adding Canonical URLs to product pages. It requires knowledge of XML layout customization but is a solid SEO practice. |
Explanation of Methods:
1. Using ProductRepositoryInterface (Recommended)
- Performance: This method is fast because it uses Magento’s built-in
ProductRepositoryInterface
, which is optimized for fetching product data. - Best Practice: It’s the most efficient method as it follows Magento's dependency injection system and ensures code maintainability.
- Ease of Use: This method is easy to implement, making it ideal for developers who need to fetch the canonical URL programmatically.
Example Code:
$productRepository = $objectManager->get(\Magento\Catalog\Api\ProductRepositoryInterface::class);
$product = $productRepository->getById($productId);
$canonicalUrl = $product->getUrlInStore();
2. Using Object Manager (Not Recommended)
- Performance: The performance is slower because the Object Manager resolves dependencies at runtime, making it less efficient than dependency injection.
- Best Practice: This is not considered a best practice in Magento, as it breaks the principles of dependency injection.
- Ease of Use: It’s simple to use but should be avoided in favor of more structured methods like dependency injection.
Why Avoid Object Manager?
- Hard to Test: Using Object Manager makes unit testing difficult because object instantiation is not controlled.
- Breaks Dependency Injection: Violates Magento's core dependency injection principles.
- Slower Performance: Performance is slower as dependencies are resolved at runtime instead of compile-time.
3. Modifying XML Layout (Good for SEO)
- Performance: This method has a medium performance impact since it relies on XML layout modifications to inject canonical tags.
- Best Practice: It's a solid choice for SEO, as it automatically adds canonical URLs to product pages without requiring additional PHP logic.
- Ease of Use: Requires XML knowledge and familiarity with Magento’s layout structure. It’s not as easy as the previous methods but offers a structured and automated solution.
Example Code:
<referenceBlock name="head.additional">
<block class="Magento\Framework\View\Element\Template"
name="canonical.url"
template="Magento_Catalog::seo/canonical.phtml"/>
</referenceBlock>
Tip
If you’re aiming for the best SEO and long-term maintainability, always use the ProductRepositoryInterface
to fetch Canonical URLs. While modifying XML layouts is a good practice for SEO, retrieving URLs programmatically via the ProductRepositoryInterface allows you to handle edge cases, dynamic products, and other customizations more flexibly.
Troubleshooting Common Issues with Canonical URLs in Magento 2
Issue | Cause | Solution |
---|---|---|
Canonical URL returns NULL | Product ID does not exist or is incorrect | Verify the product ID exists in the database before calling the function. Use ProductRepositoryInterface to check if the product is available. |
Incorrect Canonical URL | Multiple store views or wrong store ID affecting URLs | Ensure you are using the correct storeId when calling getUrlInStore(). For multiple store views, specify the storeId parameter. |
Duplicate Canonical Tags | Theme override or conflicting modules | Check the layout.xml files for multiple canonical tag entries. Look for conflicts between custom theme and third-party modules overriding the <head.additional> block. |
Canonical URL not updated | Missing or outdated product URL rewrite | Clear Magento's URL rewrites cache and reindex data to ensure the latest canonical URL is being fetched. |
Canonical Tag not rendering | Incorrect template or block override | Ensure that the canonical tag is correctly added to the <head> section using the right layout XML files. Validate if Magento_Catalog::seo/canonical.phtml is being called properly. |
Canonical Tag pointing to homepage | Product page incorrectly configured | Check that the product’s URL is correctly configured. If it's pointing to the homepage, verify product URL path and ensure correct category associations. |
Canonical URL includes parameters | Parameters in URL affecting canonical URL | Ensure the canonical URL is clean and doesn’t include unnecessary query parameters like ?color=red. Use Magento’s getUrlInStore() method with the no_cache and no_redirect options to ensure a clean URL. |
Canonical Tag missing for specific products | Product type or template issue | Ensure the product type (like downloadable or virtual products) is properly configured to include the canonical URL. Check for missing XML or PHTML template overrides for these types. |
Tip
Always check for conflicting third-party extensions or custom modules that could affect how canonical tags are rendered on the frontend. Regularly update Magento to ensure compatibility with SEO best practices.
Best Practices for Managing Canonical URLs in Magento 2
Canonical URLs are a critical part of SEO strategy in Magento 2, helping search engines understand which version of a page should be indexed. Below are some of the best practices for managing canonical URLs to ensure optimal SEO performance:
Always Fetch Canonical URLs Dynamically Using getUrlInStore()
- Avoid hardcoding canonical URLs in your templates. Use the
getUrlInStore()
method to fetch the correct canonical URL dynamically. This ensures that the canonical tag always points to the most accurate version of the page, based on the store view, product, and any URL parameters.
$product = $this->productRepository->getById($productId);
$canonicalUrl = $product->getUrlInStore();
Leverage Magento’s Built-In SEO Features to Avoid Duplicate Content
- Magento 2 has powerful built-in tools for managing SEO and duplicate content. Make sure you use them effectively:
- Canonical Tags: Magento automatically includes canonical URLs in the section of product pages if configured correctly.
- URL Rewrites: Set up proper URL rewrites to manage different URLs for products, categories, and store views.
- Noindex/NoFollow Tags: Use noindex tags to prevent low-value or duplicate pages from being indexed by search engines.
Verify Canonical URLs Using Google Search Console
- After implementing canonical tags, it’s essential to check how Google is interpreting them. Use Google Search Console> to verify that your canonical URLs are being properly recognized. Look under the "URL Inspection" tool to see if the correct canonical version is being assigned.
- Action Tip: Regularly monitor Google Search Console for any errors or inconsistencies related to your canonical tags.
Ensure Canonical URLs Match the Preferred URL Structure Across Multiple Store Views
- If you have multiple store views (e.g., for different languages or regions), ensure that your canonical URLs point to the correct version of the product page, with the appropriate store ID or locale. This is essential for both user experience and SEO.
Tip
For multilingual stores, avoid using query parameters like ?lang=en in your canonical URLs. Instead, ensure that the URLs are clean and reflect the appropriate language or region.
Avoid Canonical URL Conflicts Between Themes and Extensions
- Check for any conflicts between your custom theme and third-party extensions that might override the canonical tag. Conflicts can lead to multiple canonical tags on the same page, which can confuse search engines and negatively impact SEO.
- Solution: Always ensure that only one canonical URL is outputted in the
<head>
section of your pages. Inspect yourlayout.xml
andhead.additional
blocks to avoid duplication.
Regularly Update Canonical URLs for Updated Product Data
- As your product data evolves, ensure that the canonical URL is updated to reflect the latest changes in product categories, descriptions, and URLs. Missing or outdated canonical URLs can hurt your SEO performance.
Tip
Implement a cache-clear and reindex process to ensure the latest canonical URL is being fetched and rendered after any updates.
Use Canonical Tags for Pagination and Faceted Navigation
- Pagination and faceted navigation (such as filtering by size, color, or price) can create duplicate content that harms SEO. Use the canonical tag to point back to the main page instead of having multiple versions of the same page indexed.
- Example: On category pages, you can set the canonical URL for page 2 or filtered pages to point to the main category page to avoid duplicate content.
Optimize Canonical Tags for Product Variants
- If your store sells product variants (such as different sizes or colors), make sure that the canonical URL points to the main product page, rather than the variant-specific URL.
- Example: If you sell a product in multiple colors, the canonical tag should point to the main product URL (e.g.,
example.com/product-name
) rather than a URL with parameters like?color=red
.
Monitor for Canonical URL Issues After Site Migration
- If you’ve recently migrated your store to a new domain or changed the URL structure, it’s crucial to ensure that all canonical URLs are pointing to the new, correct URLs. Broken or incorrect canonical links can lead to SEO penalties.
- Action Tip: After migration, perform a comprehensive SEO audit to ensure that all canonical tags are correct and functional.
Canonical URL for Images and Other Non-HTML Assets
- Canonical URLs should not only be used for product and category pages, but also for important non-HTML assets like images or downloadable files. Ensure that these assets don’t create duplicate content issues by setting appropriate canonical URLs.
Common Canonical URL Issues and Solutions
Issue | Cause | Solution |
---|---|---|
Duplicate Canonical Tags | Multiple entries in <head> | Ensure that only one canonical URL is added to the <head> section. |
Incorrect Canonical URL | Using URL parameters for filtering | Remove URL parameters from the canonical tag using clean URLs (e.g., ?color=red). |
Canonical URL Not Rendering | Theme override or template issue | Ensure canonical tag is implemented correctly in layout XML and templates. |
Canonical URL Not Updated | Missing URL rewrite or cache issue | Rebuild URL rewrites and clear Magento cache to fetch the latest canonical URL. |
Product Variants Not Covered | Canonical URL points to variant | Ensure canonical URL points to the main product page instead of variant-specific URLs. |
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Conclusion
Canonical URLs are essential for managing SEO and content duplication in Magento 2. By implementing the best practices outlined in this guide, you can ensure that search engines index the correct version of your product pages, which helps maintain optimal SEO performance. Whether you choose to fetch canonical URLs using ProductRepositoryInterface, modify XML layouts, or avoid the Object Manager, understanding when and how to implement these methods is critical for maintaining clean, efficient code.
By adhering to best practices like verifying canonical URLs in Google Search Console, removing unnecessary query parameters, and ensuring consistent URL structures across multiple store views, you can avoid common issues like duplicate tags and incorrect canonical URLs. Additionally, troubleshooting common issues and knowing how to handle them effectively will help keep your Magento store’s SEO in top shape.
In conclusion, managing canonical URLs in Magento 2 requires a combination of technical understanding, practical implementation, and SEO knowledge. By following the steps and best practices outlined, you can effectively optimize your Magento store, improve indexing, and avoid penalties due to duplicate content. Always remember to stay updated on Magento’s SEO capabilities and continue refining your practices to maintain a high-quality, SEO-optimized store.
FAQs
What is a Canonical URL in Magento 2?
A canonical URL in Magento 2 helps prevent duplicate content issues by specifying the preferred URL for a product page. It guides search engines to index the correct version.
How do I fetch a product’s Canonical URL in Magento 2?
You can fetch a product’s canonical URL using the getUrlInStore()
method from the ProductRepositoryInterface
, ensuring it dynamically retrieves the correct URL.
Where should I add the Canonical Tag in Magento 2?
Add the canonical tag in the catalog_product_view.xml
file under the <head.additional>
block, linking to Magento_Catalog::seo/canonical.phtml
.
Why is my Canonical URL returning NULL?
This issue occurs when the product ID is invalid or does not exist. Ensure the product ID is correct and the product is available in the database.
How do I fix duplicate Canonical Tags in Magento 2?
Check your theme’s layout.xml
files for multiple entries of the canonical tag. Ensure only one canonical URL is added in the <head>
section.
Can I set different Canonical URLs for multiple store views?
Yes, use the storeId
parameter in getUrlInStore()
to ensure the correct canonical URL is fetched for each store view.
Why should I avoid using Object Manager to fetch Canonical URLs?
Using Object Manager directly leads to poor coding practices, breaks dependency injection, makes code harder to test, and reduces performance.
How do I verify if my Canonical URLs are working?
Use Google Search Console’s URL Inspection tool or check the page source for the <link rel="canonical">
tag.
Why is my Canonical URL pointing to the homepage?
This happens when the product URL is misconfigured. Ensure the correct product URL is set and verify category associations.
How do I remove URL parameters from Canonical URLs?
Use Magento’s getUrlInStore()
method to ensure a clean URL without unnecessary parameters like ?color=red
.
Can I programmatically update Canonical URLs in Magento 2?
Yes, you can modify the canonical URL dynamically using a custom module that updates the <link rel="canonical">
tag based on product attributes.
How do I clear the cache for Canonical URLs in Magento 2?
Run php bin/magento cache:clean
and php bin/magento cache:flush
to remove outdated canonical URLs and ensure changes are reflected.
What is the best practice for managing Canonical URLs in Magento 2?
Always use getUrlInStore()
to fetch canonical URLs dynamically, avoid hardcoding URLs, and verify them using Google Search Console for proper indexing.