Using SQL Group By and Having Clauses in Magento 2
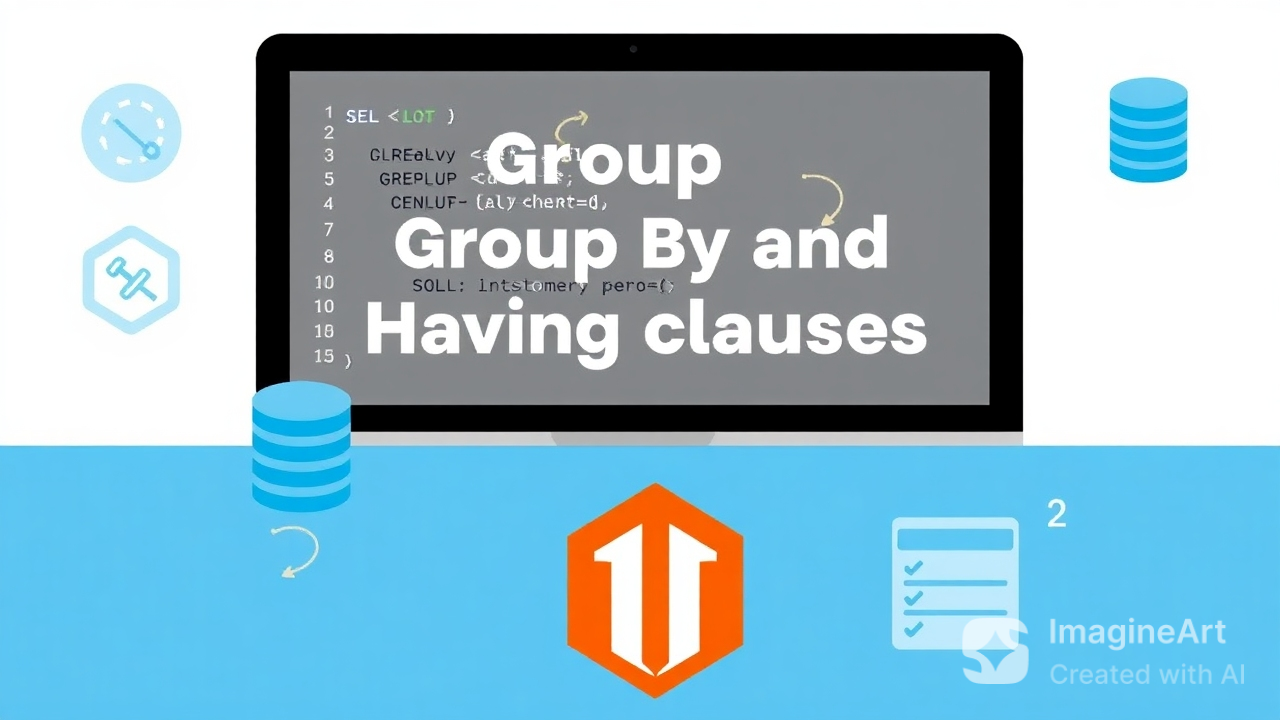
Using SQL Group By and Having Clauses in Magento 2
To identify duplicate customer emails in Magento 2, use SQL's GROUP BY and HAVING clauses. These clauses help group identical values and filter results based on conditions.
Table Of Content
Understanding GROUP BY and HAVING in Magento 2
In Magento 2, the GROUP BY clause groups rows sharing a specified column's value, while aggregate functions like COUNT, SUM, AVG, MIN, and MAX perform calculations on these groups. The HAVING clause then filters these grouped results based on a condition.
Example: Finding Duplicate Emails
To find duplicate emails in the customer_entity table, follow these steps:
Set Up the Customer Collection Factory: Inject the CollectionFactory into your class to access customer data.
use Magento\Customer\Model\ResourceModel\Customer\CollectionFactory;
class CustomerData
{
private $customerCollectionFactory;
public function __construct(CollectionFactory $customerCollectionFactory)
{
$this->customerCollectionFactory = $customerCollectionFactory;
}
}
Create a Method to Find Duplicate Emails: Use the GROUP BY clause on the 'email' field and the HAVING clause to filter groups with more than one entry.
public function findDuplicateEmails()
{
$customerCollection = $this->customerCollectionFactory->create();
$customerCollection->getSelect()
->columns(['email_count' => 'COUNT(e.entity_id)'])
->group('email')
{
->having('email_count > ?', 1);
return $customerCollection;
}
Explanation:
group('email'):
Groups customers by their email addresses.columns(['email_count' => 'COUNT(e.entity_id)']):
Counts the number of customers in each email group.- having('email_count > ?', 1): Filters to include only those email groups with more than one customer, indicating duplicates.
Common Aggregate Functions in SQL:
Function | Description |
---|---|
COUNT |
Returns the number of items in a group. |
SUM |
Adds up the numeric values in a group. |
AVG |
Calculates the average value of a numeric column. |
MIN |
Finds the smallest value in a group. |
MAX |
Finds the largest value in a group. |
Using these functions with GROUP BY and HAVING allows for efficient data analysis in Magento 2.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What Is the Purpose of the GROUP BY Clause in Magento 2?
The GROUP BY
clause is used to group identical values from a specified column in the database. It helps in organizing data and performing aggregate functions like COUNT
, SUM
, AVG
, etc.
When Should I Use the HAVING Clause Instead of WHERE?
Use the HAVING
clause when you need to filter grouped results after applying aggregate functions. The WHERE
clause cannot be used for this purpose as it filters rows before grouping.
How Can I Find Duplicate Customer Emails in Magento 2?
To find duplicate emails in the customer_entity
table, use the GROUP BY
clause to group by email and the HAVING
clause to filter groups with more than one entry.
SELECT email, COUNT(*) as emailCount
FROM customer_entity
GROUP BY email
HAVING emailCount > 1;
What Are Some Common SQL Aggregate Functions?
Common aggregate functions include:
COUNT()
: Returns the number of rows in a group.SUM()
: Adds up numeric values.AVG()
: Calculates the average value.MIN()
: Finds the smallest value.MAX()
: Finds the largest value.
What Are the Common Mistakes When Using GROUP BY and HAVING?
Common mistakes include:
- Using
WHERE
instead ofHAVING
with aggregate functions. - Grouping by the wrong column, leading to incorrect results.
- Not specifying the aggregate function correctly.
How Can I Use GROUP BY in Magento 2 Code?
Here’s an example of using GROUP BY
in Magento 2:
$customerCollection = $this->customerCollectionFactory->create();
$customerCollection->getSelect()
->columns(['emailCount' => 'COUNT(e.entity_id)'])
->group('email')
->having('emailCount > ?', 1);
This groups customers by email and finds duplicate emails.