Implementing Left Joins in Magento 2 Resource Models
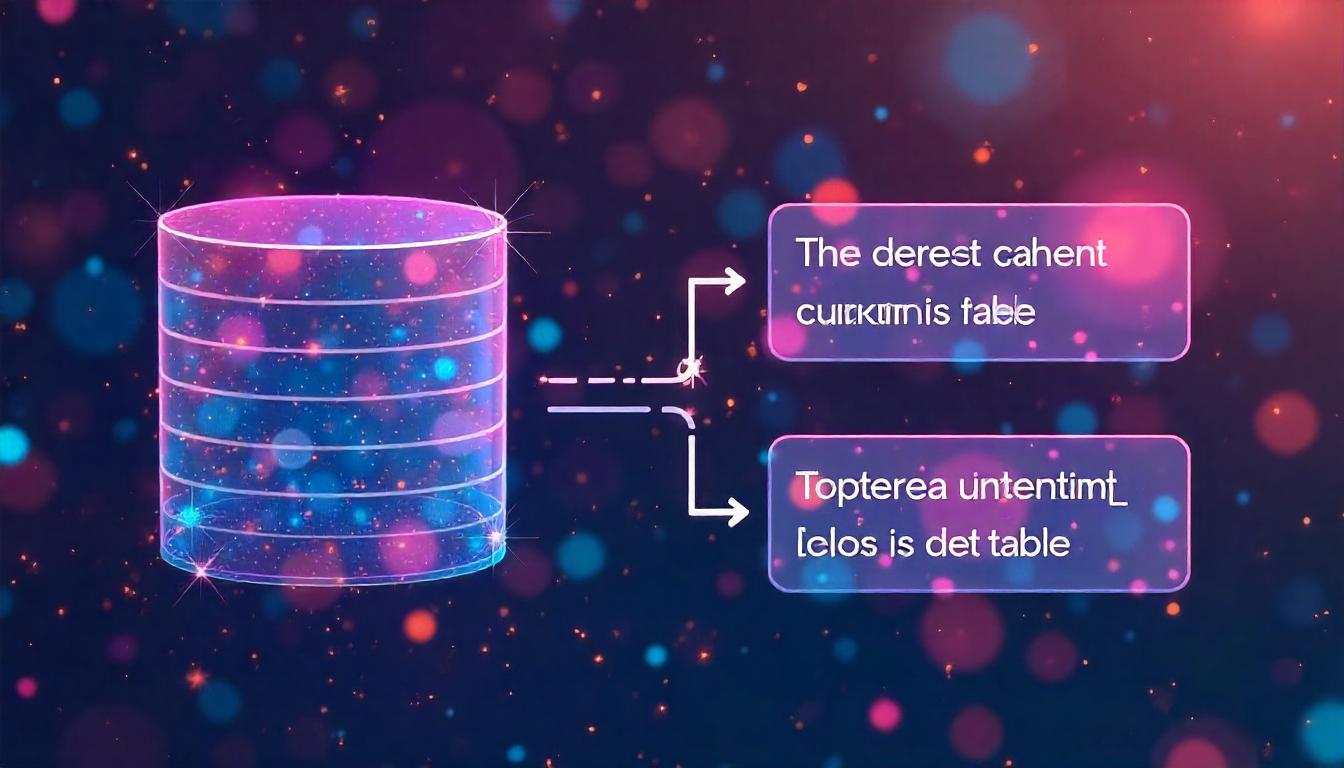
Implementing Left Joins in Magento 2 Resource Models
In Magento 2, working with custom SQL queries is common when retrieving data from multiple tables. One of the most efficient ways to achieve this is by using left joins within resource models. Left joins allow you to combine data from different tables, ensuring that all records from the primary table are included in the results, even if there are no matching records in the joined table.
Table Of Content
Implementing Left Joins in Magento 2 Resource Models
In Magento 2, you can use the joinLeft() method within a resource model's collection to perform a left join between tables. This allows you to retrieve all records from the primary table and the matching records from a secondary table.
Understanding the joinLeft() Method:
The joinLeft() method has the following signature:
joinLeft($name, $cond, $cols = '*', $schema = null)
$name:
The name of the table to join. This can be an array, string, or Zend_Db_Expr.$cond:
The join condition as a string.$cols:
The columns to select from the joined table. This can be an array or string.- $schema: The database schema, if applicable.
Example: Fetching Customer Data with Address Details
Here's how you can fetch customer data along with their city and postcode from the customer_address_entity table using a left join:
<?php
declare(strict_types=1);
namespace Vendor\Module\Model;
use Magento\Customer\Model\ResourceModel\Customer\CollectionFactory;
use Magento\Framework\App\ResourceConnection;
class CustomerDataProvider
{
private CollectionFactory $customerCollectionFactory;
private ResourceConnection $resource;
public function __construct(
CollectionFactory $customerCollectionFactory,
ResourceConnection $resource
) {
$this->customerCollectionFactory = $customerCollectionFactory;
$this->resource = $resource;
}
public function getCustomerData(string $email): array
{
$customerCollection = $this->customerCollectionFactory->create();
$customerCollection->addAttributeToFilter('email', $email);
$customerCollection->getSelect()->joinLeft(
['ca' => $this->getTableName('customer_address_entity')],
'e.entity_id = ca.parent_id',
['city', 'postcode']
);
return $customerCollection->getData();
}
private function getTableName(string $name): string
{
return $this->resource->getTableName($name);
}
}
Explanation
- The primary table is customer_entity, represented by $customerCollection.
- The joinLeft() method joins the customer_address_entity table (ca) on the condition that e.entity_id equals ca.parent_id.
- The columns city and postcode are selected from the joined table.
Extending to Multiple Joins:
You can perform multiple joins in a single query by chaining additional joinLeft() (or other join methods like joinInner()) calls. Ensure that each join has a clear condition and specifies the necessary columns.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What Is the Purpose of Left Join in Magento 2?
The LEFT JOIN
is used to fetch all records from the primary table and matching records from the second table. It helps in retrieving related data from multiple tables while ensuring no data from the left table is omitted.
When Should I Use LEFT JOIN in Magento 2 Resource Models?
Use LEFT JOIN
when you need to include all rows from the primary table, even if there is no match in the second table. It is useful for combining data from related tables, like retrieving customer data with address details.
How Can I Use LEFT JOIN in Magento 2 Code?
Here’s an example of using LEFT JOIN
in a Magento 2 resource model:
$customerCollection = $this->customerCollectionFactory->create();
$customerCollection->addAttributeToFilter('email', '[email protected]');
$customerCollection->getSelect()->joinLeft(
['ca' => $this->getTable('customer_address_entity')],
'e.entity_id = ca.parent_id',
['city', 'postcode']
);
This example fetches customer data along with city and postcode details from the customer_address_entity
table.
What Are the Benefits of Using LEFT JOIN in Magento 2?
Using LEFT JOIN
allows you to:
- Retrieve all records from the primary table, even when there are no matching rows in the secondary table.
- Combine data from multiple related tables efficiently.
- Ensure no data from the main table is left out, even if some joined tables do not have corresponding records.
What Are Common Mistakes When Using LEFT JOIN in Magento 2?
Common mistakes include:
- Not specifying the join condition properly, leading to incorrect results.
- Joining tables that don't have a clear relationship, which may cause data inconsistencies.
- Using
LEFT JOIN
when anINNER JOIN
is more appropriate for the data structure.
How Can I Perform Multiple Joins in a Single Query in Magento 2?
To perform multiple joins, chain additional joinLeft()
calls. Here’s an example:
$customerCollection->getSelect()->joinLeft(
['ca' => $this->getTable('customer_address_entity')],
'e.entity_id = ca.parent_id',
['city', 'postcode']
)->joinLeft(
['o' => $this->getTable('sales_order')],
'e.entity_id = o.customer_id',
['order_count' => 'COUNT(o.entity_id)']
);
This example retrieves customer data along with their address details and order count in a single query.