Retrieving Selection IDs for Bundle Products in Magento 2
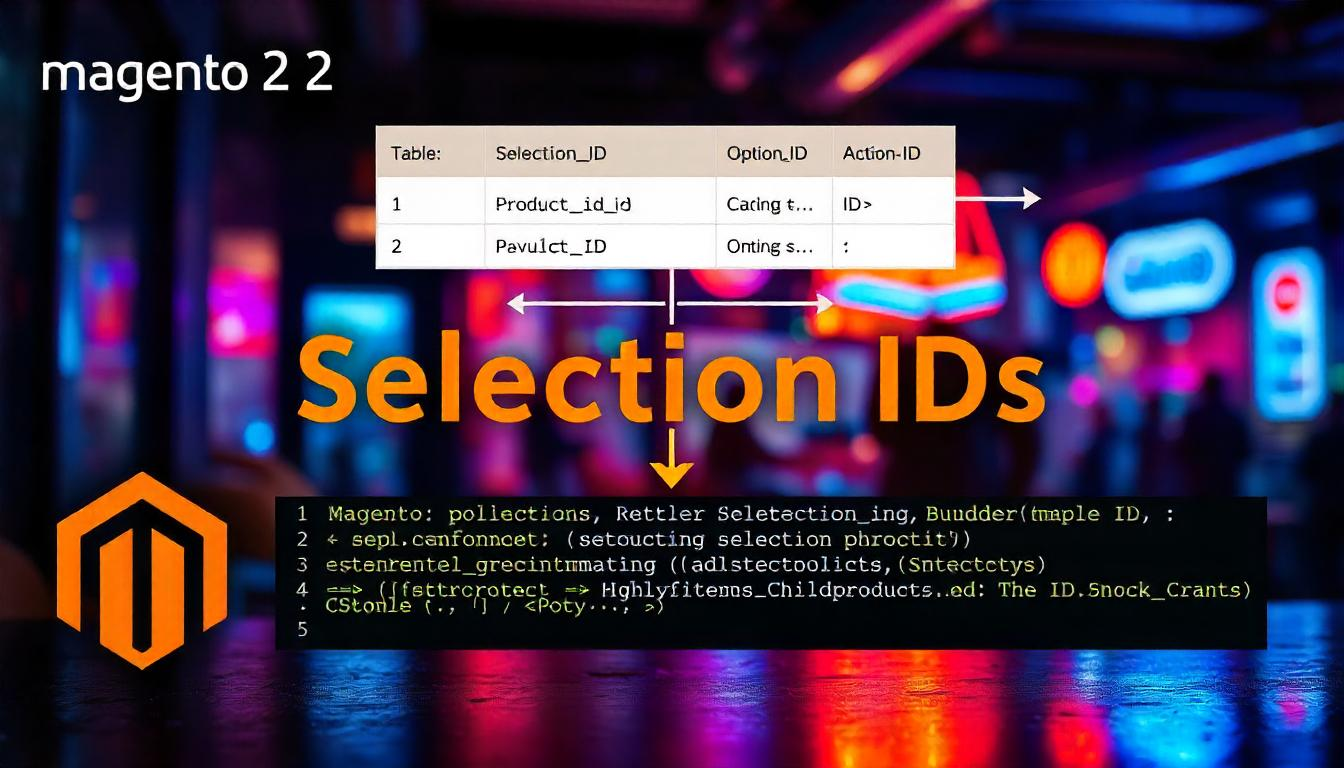
Retrieving Selection IDs for Bundle Products in Magento 2
In Magento 2, bundle products allow customers to customize their purchases by selecting options like size, color, and quantity, all within a single product. To manage this functionality programmatically, you may need to retrieve the selection ID associated with a bundle item and its child product.
Table Of Content
Retrieving Selection IDs for Bundle Products in Magento 2
Magento 2's bundle products allow customers to customize their purchases by selecting options and adding items to the cart with a single click. The catalog_product_bundle_selection
table stores the relationship between bundle items and their associated child products, including details like option ID, quantity, position, and default item selection.
Understanding the catalog_product_bundle_selection
Table
This table links bundle items to their child products. It contains columns such as selection_id
, option_id
, product_id
, and parent_product_id
, which are essential for identifying the selection ID based on the bundle item ID and child product ID.
Retrieving the Selection ID
To obtain the selection ID for a specific bundle item and its child product, execute a database query using the following function:
namespace YourNamespace\YourModule\Model;
use Magento\Framework\App\ResourceConnection;
class BundleSelectionId
{
private const BUNDLE_SELECTION_TABLE = "catalog_product_bundle_selection";
private $resourceConnection;
public function __construct(ResourceConnection $resourceConnection)
{
$this->resourceConnection = $resourceConnection;
}
public function getSelectionId(int $bundleItemId, int $childItemId)
{
$tableName = $this->resourceConnection->getTableName(self::BUNDLE_SELECTION_TABLE);
$connection = $this->resourceConnection->getConnection();
$select = $connection->select()
->from(['e' => $tableName], ['selection_id', 'option_id', 'product_id'])
->where("e.product_id = :product_id")
->where("e.parent_product_id = :parent_product_id");
$bind = ['product_id' => $childItemId, 'parent_product_id' => $bundleItemId];
return $connection->fetchRow($select, $bind);
}
}
Usage Example
To retrieve the selection ID for a bundle item with ID 52 and a child product with ID 29, use the following code:
$bundleItemId = 52;
$childItemId = 29;
$selectionId = $this->getSelectionId($bundleItemId, $childItemId);
echo $selectionId;
Additional Considerations
- Error Handling: Ensure to handle cases where no matching records are found to prevent errors in your application.
- Performance: For large datasets, consider optimizing your queries or using Magento's built-in models and collections to improve performance.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
How Can I Retrieve Selection IDs for Bundle Products in Magento 2?
To retrieve selection IDs for bundle products, use the catalog_product_bundle_selection
table, which stores the relationship between bundle items and child products. You can fetch the selection ID by querying the table with the bundle item ID and child product ID.
What Is the Purpose of the catalog_product_bundle_selection
Table?
The catalog_product_bundle_selection
table links bundle items to child products. It includes columns like selection_id
, option_id
, product_id
, and parent_product_id
, which are essential for identifying and retrieving selection IDs for bundle products.
How Do I Retrieve the Selection ID Using the Bundle Item ID and Child Product ID?
You can retrieve the selection ID by executing a database query with the following code:
$bundleItemId = 52;
$childItemId = 29;
$selectionId = $this->getSelectionId($bundleItemId, $childItemId);
echo $selectionId;
What Happens If No Selection ID Is Found?
If no selection ID is found for the given bundle item and child product IDs, an empty result will be returned. It's important to handle this case to avoid errors in your application.
Can I Optimize the Query for Retrieving Selection IDs?
Yes, for larger datasets, you can optimize the query by using Magento's built-in models or collections, or by adding appropriate indexes to the database table to improve performance.
What Is the Role of ResourceConnection
in the Code?
The ResourceConnection
class is used to manage database connections and execute queries. It provides access to the Magento database connection, which is necessary for executing the query to retrieve selection IDs.