Retrieving Parent Product ID from Bundle Option ID in Magento 2
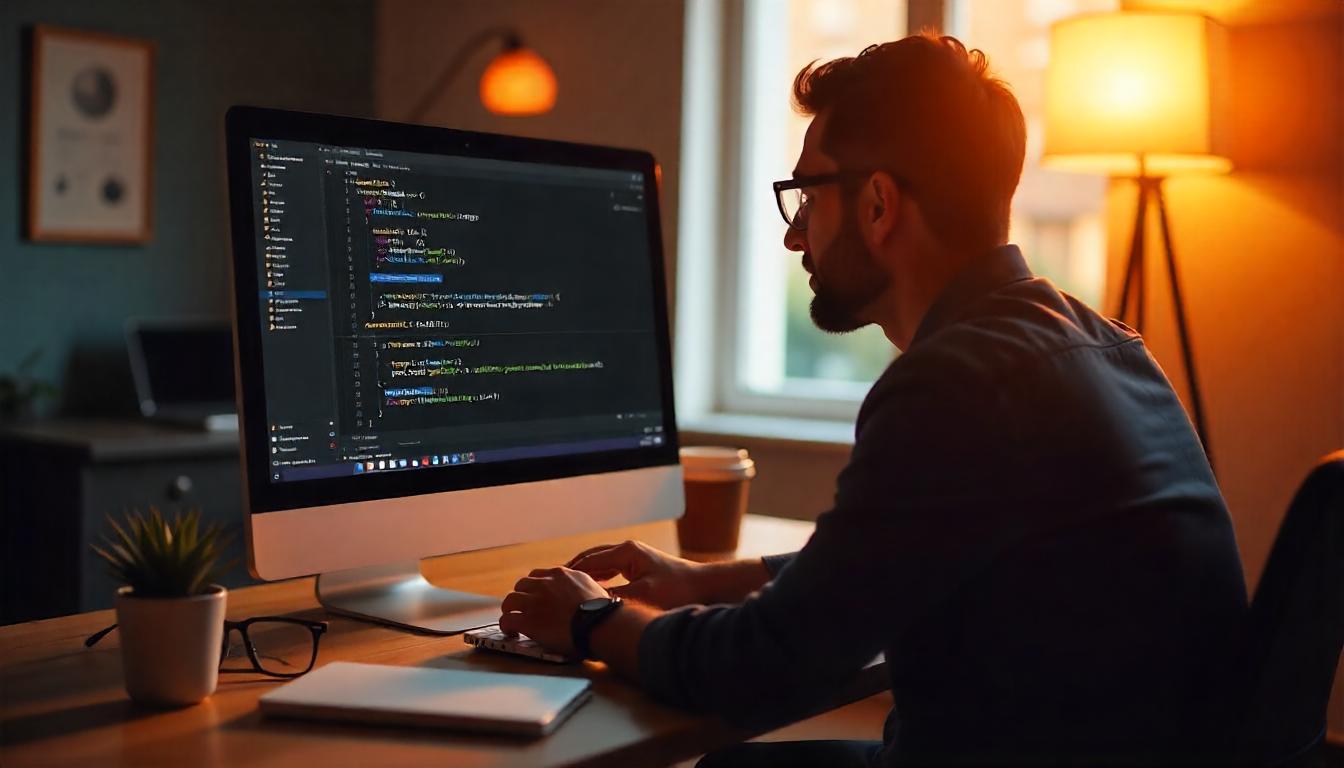
Retrieving Parent Product ID from Bundle Option ID in Magento 2
When working with bundle products in Magento 2, you might need to retrieve the parent product ID using a specific option ID. This process is often required for customizations, such as managing bundle product options or creating dynamic catalog updates.
Table Of Content
Retrieving Parent Product ID from Bundle Option ID in Magento 2
In Magento 2, when working with bundle products, you might need to find the parent product ID using a specific option ID. This is useful for various customizations and integrations.
Understanding Bundle Products
A bundle product in Magento 2 consists of multiple options, each containing a list of associated products, such as simple or virtual products. Each option has a unique option ID.
Retrieving the Parent Product ID
To obtain the parent product ID from a given option ID, you can use the following approach:
Create a Helper Class
Define a helper class to encapsulate the logic for retrieving the parent product ID.
collectionFactory = $collectionFactory;'; ?>
collectionFactory->create();'; ?>
addFieldToFilter(\'option_id\', [\'eq\' => $optionId]);'; ?>
getParentId();'; ?>
Usage Example
To use this helper method and retrieve the parent product ID:
$optionId = 1; // Replace with your actual option ID
$parentId = $this->helper('YourNamespace\YourModule\Helper\Data')->getParentProductIdByOptionId($optionId);
echo $parentId; // Outputs the parent product ID
Alternative Method Using Product Types
If you need to retrieve the parent product ID from a child product ID, especially for configurable or grouped products, you can use the following approach:
Create a Helper Class
<?php
namespace YourNamespace\YourModule\Helper;
use Magento\ConfigurableProduct\Model\Product\Type\Configurable;
use Magento\GroupedProduct\Model\Product\Type\Grouped;
use Magento\Catalog\Model\ProductFactory;
class Data extends \Magento\Framework\App\Helper\AbstractHelper
{
protected $configurable;
protected $grouped;
protected $productFactory;
public function __construct(
\Magento\Framework\App\Helper\Context $context,
Configurable $configurable,
Grouped $grouped,
ProductFactory $productFactory
) {
$this->configurable = $configurable;
$this->grouped = $grouped;
$this->productFactory = $productFactory;
parent::__construct($context);
}
public function getParentProductId($childId)
{
// For simple product of configurable product
$parentIds = $this->configurable->getParentIdsByChild($childId);
if (isset($parentIds[0])) {
return $parentIds[0];
}
// For simple product of grouped product
$parentIds = $this->grouped->getParentIdsByChild($childId);
if (isset($parentIds[0])) {
return $parentIds[0];
}
// For bundle product
$parentIds = $this->productFactory->create()->load($childId)->getTypeInstance()->getParentIdsByChild($childId);
if (isset($parentIds[0])) {
return $parentIds[0];
}
return null;
}
}
Usage Example
$childProductId = 33; // Replace with your actual child product ID
$parentId = $this->helper('YourNamespace\YourModule\Helper\Data')->getParentProductId($childProductId);
echo $parentId; // Outputs the parent product ID
Conclusion
By implementing these methods, you can effectively retrieve the parent product ID from a given option ID or child product ID in Magento 2. This approach is essential for various customizations and integrations within your Magento store.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
How Can I Retrieve Parent Product ID from Bundle Option ID in Magento 2?
To retrieve the parent product ID from a bundle option ID in Magento 2, you can use a custom helper class and Magento's collection factory. By filtering the catalog_product_bundle_option
table with the option ID, you can get the corresponding parent product ID.
What Is the Purpose of the catalog_product_bundle_option
Table?
The catalog_product_bundle_option
table stores information about bundle options and their associated parent products. It is used to map the options selected in bundle products to their parent product IDs. Key columns include option_id
and parent_id
.
How Do I Retrieve the Parent Product ID Using the Bundle Option ID?
You can retrieve the parent product ID by executing a query using the following PHP code:
$optionId = 1;
$parentId = $this->getParentItemIdByOptionId($optionId);
echo $parentId;
What Happens If No Parent Product ID Is Found?
If no parent product ID is found for the given option ID, the result will be null. Ensure to handle this case to avoid potential errors when using the ID in your application.
Can I Optimize the Code for Retrieving Parent Product IDs?
Yes, you can optimize the code by using Magento's models or collections for better performance. Additionally, ensure your database is indexed correctly to speed up the retrieval process, especially when dealing with large datasets.
What Is the Role of CollectionFactory
in the Code?
The CollectionFactory
class is used to create and manage collections for retrieving records from the database. It simplifies querying the catalog_product_bundle_option
table to fetch the parent product ID based on the option ID.