Determine if a Product is Downloadable in Magento 2
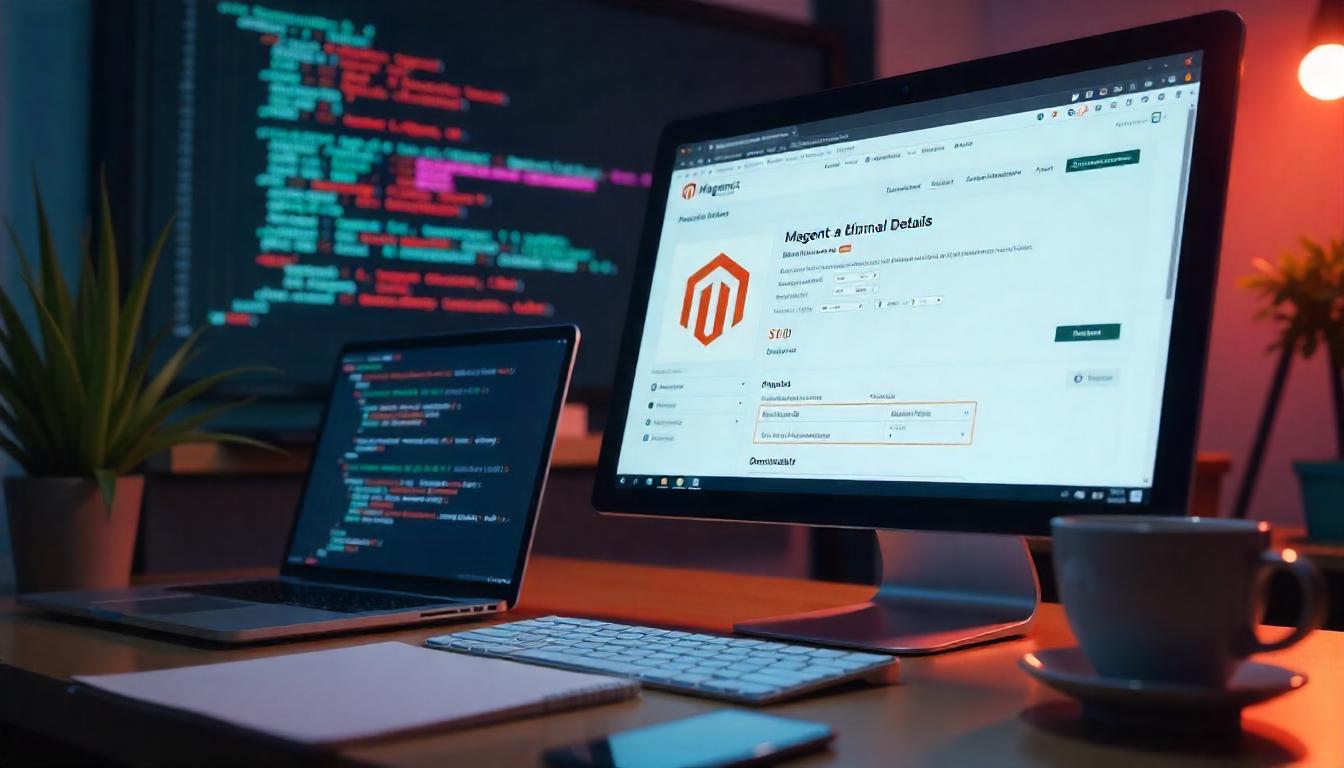
Determine if a Product is Downloadable in Magento 2
Learn how to check if a product in Magento 2 is downloadable using PHP. This guide explains how to retrieve a product by SKU or ID, use the getTypeId() method, and compare it with Type::TYPE_DOWNLOADABLE. Includes a code example and best practices for accurate product type detection.
Table Of Content
Determine if a Product is Downloadable in Magento 2
To check if a product is downloadable in Magento 2, use the ProductRepositoryInterface to fetch the product by its SKU or ID. Then, call the getTypeId()
method to identify the product type. Compare the result with the constant Type::TYPE_DOWNLOADABLE
from Magento\Downloadable\Model\Product\Type.
Here's a concise example:
<?php
namespace Vendor\Module\Model;
use Magento\Catalog\Api\ProductRepositoryInterface;
use Magento\Downloadable\Model\Product\Type as DownloadableType;
use Magento\Framework\Exception\NoSuchEntityException;
use Psr\Log\LoggerInterface;
class DownloadableTypeChecker
{
private LoggerInterface $logger;
private ProductRepositoryInterface $productRepository;
public function __construct(
LoggerInterface $logger,
ProductRepositoryInterface $productRepository
) {
$this->logger = $logger;
$this->productRepository = $productRepository;
}
public function isDownloadableProduct(string $sku): bool
{
try {
$product = $this->productRepository->get($sku);
return $product->getTypeId() === DownloadableType::TYPE_DOWNLOADABLE;
} catch (NoSuchEntityException $exception) {
$this->logger->error("Product with SKU '{$sku}' not found: " . $exception->getMessage());
return false;
}
}
}
Usage Example:
$sku = 'your-product-sku';
$isDownloadable = $this->isDownloadableProduct($sku);
If you have the product ID instead of the SKU, replace $this->productRepository->get($sku)
with $this->productRepository->getById($productId).
Key Points:
- Fetch the product using
ProductRepositoryInterface
. - Use
getTypeId()
to determine the product type. - Compare the type ID with
Type::TYPE_DOWNLOADABLE
to check if it's a downloadable product.
This method ensures a straightforward way to programmatically verify if a product in Magento 2 is downloadable.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
How Do I Check If a Product Is Downloadable in Magento 2?
You can check if a product is downloadable by retrieving the product using the ProductRepositoryInterface
and calling the getTypeId()
method. If the type ID matches Type::TYPE_DOWNLOADABLE
, the product is downloadable.
What Is the Best Way to Verify a Downloadable Product by SKU?
The best approach is to use the get()
method of ProductRepositoryInterface
with the SKU, then compare the product type ID with Type::TYPE_DOWNLOADABLE
.
Can I Check Downloadable Product Type Using Product ID?
Yes, instead of fetching the product by SKU, you can use the getById()
method of ProductRepositoryInterface
to check the product type.
What Code Can I Use to Check If a Product Is Downloadable?
You can use the following PHP code to determine if a product is downloadable:
$product = $this->productRepository->get($sku);
$isDownloadable = ($product->getTypeId() === Type::TYPE_DOWNLOADABLE);
What Happens If the Product Does Not Exist?
If the product does not exist, Magento will throw a NoSuchEntityException
. It's recommended to use a try-catch block to handle this error and log it properly.
How Can I Log Errors When Checking Product Type?
You can log errors using Psr\Log\LoggerInterface
. This helps track issues like missing SKUs or retrieval failures.