Retrieving Super Attribute Values for Child Products in Magento 2
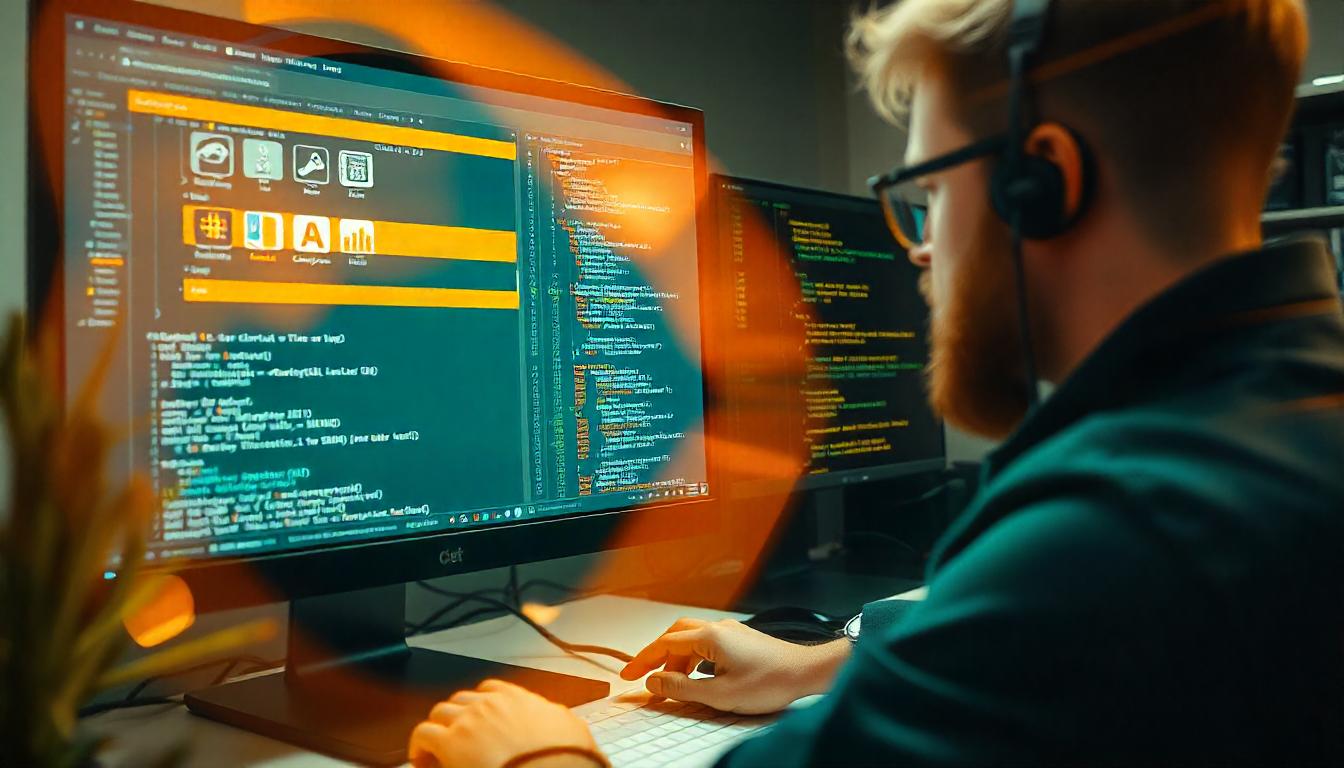
Retrieving Super Attribute Values for Child Products in Magento 2
When working with Magento 2, it's essential to retrieve data from the database securely. Instead of using raw SQL queries, which can pose security risks, Magento 2 offers a standard method to perform select operations safely.
Table Of Content
Retrieving Super Attribute Values for Child Products in Magento 2
When working with configurable products in Magento 2, it's often necessary to programmatically access the super attribute values of associated child products. This is particularly useful when adding configurable products to the cart dynamically.
Understanding Super Attributes
Super attributes are the options (like size or color) that define the variations of a configurable product. Each child product corresponds to a specific combination of these attributes.
Example Scenario
Consider a configurable product with the SKU 'MT12' and a child product with the SKU 'MT12-XL-Blue'. The child product has the following super attribute values:
- Size: XL
- Color: Blue
Retrieving Super Attribute Values Programmatically
To fetch the super attribute values of a child product, you can use the following PHP code:
namespace YourNamespace\SuperAttribute\Model;
use Magento\Catalog\Api\Data\ProductInterface;
use Magento\Catalog\Api\ProductAttributeRepositoryInterface;
use Magento\Catalog\Api\ProductRepositoryInterface;
use Magento\Framework\Exception\NoSuchEntityException;
use Psr\Log\LoggerInterface;
class Data
{
private $productRepository;
private $attributeRepository;
private $logger;
public function __construct(
ProductRepositoryInterface $productRepository,
ProductAttributeRepositoryInterface $attributeRepository,
LoggerInterface $logger
) {
$this->productRepository = $productRepository;
$this->attributeRepository = $attributeRepository;
$this->logger = $logger;
}
public function getChildSuperAttribute(string $parentSku, string $childSku)
{
$parentProduct = $this->getProduct($parentSku);
$childProduct = $this->getProduct($childSku);
$_attributes = $parentProduct->getTypeInstance(true)->getConfigurableAttributes($parentProduct);
$attributesPair = [];
foreach ($_attributes as $_attribute) {
$attributeId = (int)$_attribute->getAttributeId();
$attributeCode = $this->getAttributeCode($attributeId);
$attributesPair[$attributeId] = (int)$childProduct->getData($attributeCode);
}
return $attributesPair;
}
public function getAttributeCode(int $id)
{
return $this->attributeRepository->get($id)->getAttributeCode();
}
public function getProduct(string $sku)
{
$product = null;
try {
$product = $this->productRepository->get($sku);
} catch (NoSuchEntityException $exception) {
$this->logger->error(__($exception->getMessage()));
}
return $product;
}
}
Usage
To use this function, instantiate the Data class and call the getChildSuperAttribute
method with the parent and child SKUs:
<?php
$parentSku = 'MT12';
$childSku = 'MT12-XL-Blue';
$superAttributeByChild = $this->getChildSuperAttribute($parentSku, $childSku);
<?php echo $superAttributeByChild; ?>
Expected Output
The function will return an array with the attribute IDs as keys and the corresponding option IDs as values:
[194] => 5606
[93] => 5486
In this example:
- 194: Size attribute ID
- 5606: XL option ID
- 93: Color attribute ID
- 5486: Blue option ID
Ensure that the attribute IDs and option IDs correspond to your specific product attributes and options. You can retrieve these IDs from the Magento admin panel or by querying the database.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
How Can I Retrieve Super Attribute Values for Child Products in Magento 2?
Magento 2 allows you to retrieve super attribute values for child products by using the parent and child product SKUs. This is helpful when adding configurable products to the cart dynamically.
What Are Super Attributes in Magento 2?
Super attributes are the different variations (e.g., size, color) of a configurable product. Each child product corresponds to a unique combination of these attributes.
What Is the Expected Output When Fetching Super Attribute Values?
The expected output will be an array with attribute IDs as keys and option IDs as values. For example:
Array
(
[194] => 5606
[93] => 5486
)
What Are the Benefits of Using the Parent and Child SKUs?
Using the parent and child SKUs ensures that you fetch the correct super attribute values for each child product, especially when dealing with dynamic product additions to the cart.
Can I Use Super Attribute Values for Other Operations Besides Cart Addition?
Yes, you can use super attribute values for other operations such as customizing product displays or generating reports based on attribute variations.