How to Check If Cookie Restriction Mode Is Enabled Programmatically in Magento 2
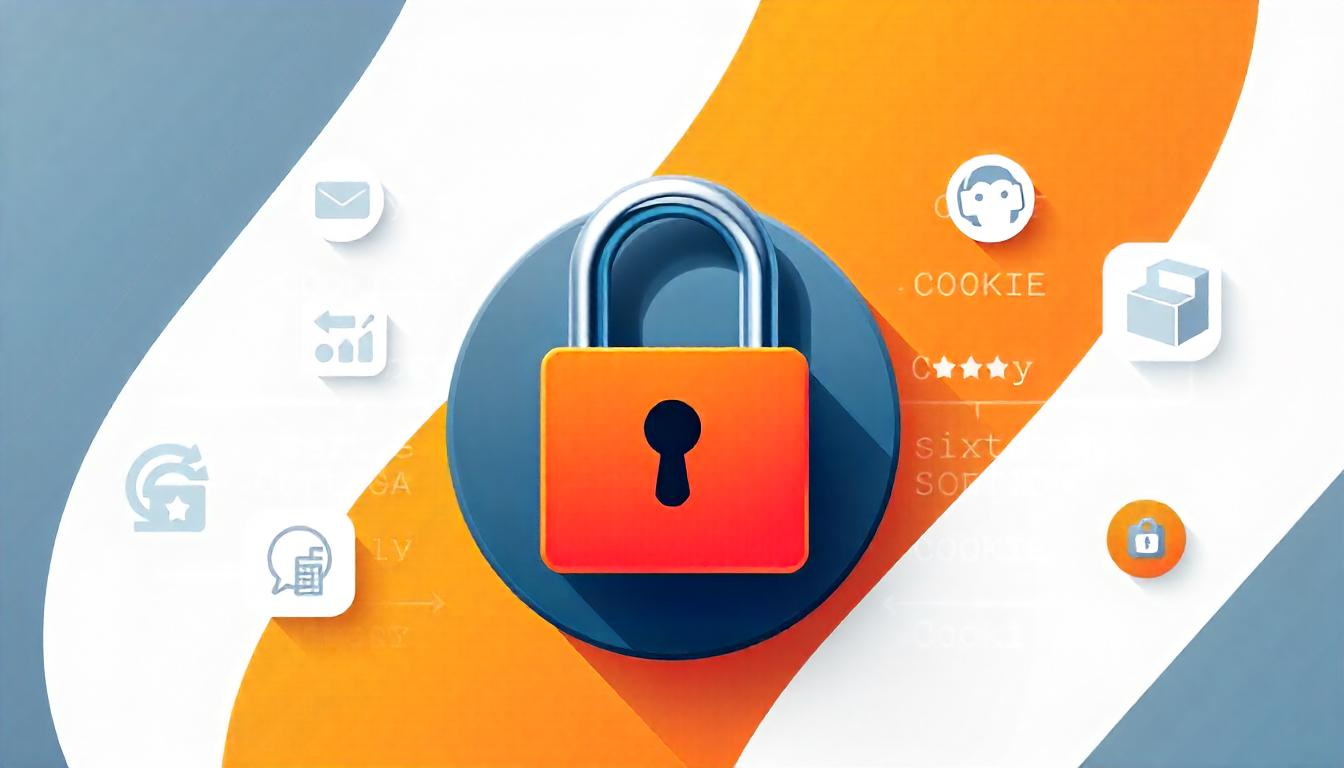
How to Check If Cookie Restriction Mode Is Enabled Programmatically in Magento 2
Cookie restriction mode in Magento 2 is a critical feature for ensuring compliance with data privacy laws such as GDPR, CCPA, and other global regulations. When enabled, it prevents Magento from setting cookies until the user explicitly consents.
This guide will cover:
- How cookie restriction mode works in Magento 2
- Programmatic methods to check if it is enabled
- Retrieving the setting via the admin panel
- Troubleshooting and best practices
Table Of Content
- What Is Cookie Restriction Mode in Magento 2?
- Checking Cookie Restriction Mode Programmatically
- Comparison of Methods for Checking Cookie Restriction Mode
- Common Issues and Troubleshooting in Magento 2 Cookie Restriction Mode
- Best Practices for Handling Cookie Restriction Mode in Magento 2
- Conclusion
- FAQs
What Is Cookie Restriction Mode in Magento 2?
Cookie Restriction Mode is a built-in feature in Magento 2 that helps businesses comply with privacy regulations such as the GDPR (General Data Protection Regulation) by ensuring that cookies are only set after the user’s explicit consent. This is crucial for maintaining transparency and trust with customers, while also preventing unnecessary tracking or data collection before user consent.
Key Features of Cookie Restriction Mode:
Feature | Description |
---|---|
Purpose | Restricts cookies from being stored until the user gives consent. |
Default Behavior | Disabled by default in Magento 2; can be activated via configuration settings. |
Configuration Path | web/cookie/cookie_restriction (XML path). |
Magento Helper Class | Magento\Cookie\Helper\Cookie |
Admin Panel Setting | Stores > Configuration > General > Web > Default Cookie Settings |
Affects | Session cookies, tracking cookies, and custom cookies. |
User Experience | A banner or popup is typically displayed to inform users about cookie usage and request consent. |
Compliance | Ensures compliance with regulations like GDPR, CCPA, etc., by controlling the behavior of cookies. |
Granularity | Administrators can specify which cookies are considered essential and which require consent. |
Customization | Customize the appearance and message of the cookie consent banner in the admin settings. |
How Cookie Restriction Mode Works:
When enabled, Magento 2 will prevent non-essential cookies from being set in the user’s browser until they have given consent. Essential cookies that are necessary for the basic functioning of the site, such as session cookies, will still be placed. The system provides an option for users to accept or reject cookies through a visible notification (usually in the form of a banner).
- When a user visits a site: They are shown a notification asking for consent to use cookies.
- If consent is given: Cookies are stored in the browser as per the defined policies (e.g., session cookies, tracking cookies, etc.).
- If consent is denied: Only essential cookies, which are needed for basic functionality, are allowed. Non-essential cookies, such as tracking or analytics cookies, are not set.
Benefits of Cookie Restriction Mode in Magento 2:
Compliance with Privacy Laws:
- Ensures businesses comply with global data privacy regulations, such as GDPR and CCPA, by restricting the use of cookies without user consent.
Improved User Trust:
- Transparent cookie usage helps build trust with customers who are concerned about their privacy and data security.
Customizable User Consent Mechanism:
- Magento 2 allows customization of the cookie consent message and banner, providing flexibility in how consent is requested and displayed to users.
Control Over Cookie Usage:
- Magento 2’s configuration allows store owners to control which cookies are essential and which ones require user consent, reducing unnecessary data collection.
SEO Benefits:
- By controlling cookies and user tracking, the website remains compliant with privacy regulations, avoiding potential penalties or loss of rankings due to non-compliance.
How to Enable Cookie Restriction Mode in Magento 2:
- Step 1: Go to the Magento Admin Panel.
- Step 2: Navigate to Stores > Configuration.
- Step 3: Under General, select Web.
- Step 4: Scroll down to Default Cookie Settings.
- Step 5: Set the Cookie Restriction Mode option to Yes.
- Step 6: Customize the message shown to users about cookies (optional).
- Step 7: Save the configuration.
Once enabled, Magento will take care of displaying the cookie consent prompt on the frontend of the site, ensuring you are compliant with cookie usage regulations.
Considerations:
- SEO Impact: While cookie consent banners can impact user experience, they generally do not have a significant negative impact on SEO as long as the banner is implemented without affecting the site’s loading time or functionality.
- Cache and Session Management: Be mindful of how cookies are handled during sessions when cookie restriction mode is enabled, as this could affect functionality like user logins, cart data, and personalized content until consent is given.
Tip
To ensure a smooth user experience, use an async or deferred script to load the cookie consent banner. This prevents delays in page rendering and maintains SEO performance while complying with privacy regulations.
Checking Cookie Restriction Mode Programmatically
Magento offers multiple ways to check whether Cookie Restriction Mode is enabled. This is particularly useful for developers who need to control cookie behavior dynamically in custom modules, extensions, or frontend implementations. By programmatically verifying this setting, you can ensure compliance with data protection laws like GDPR and CCPA, optimize user experience, and manage session handling effectively.
Below are the different approaches to check the Cookie Restriction Mode in Magento 2:
Method 1: Using Magento Cookie Helper Class
Magento provides a built-in helper class to check whether Cookie Restriction Mode is enabled. This approach is efficient and ensures compliance with cookie consent policies without requiring direct database queries.
How It Works
The Magento\Cookie\Helper\Cookie
class includes the function isCookieRestrictionModeEnabled()
, which returns a boolean value (true or false) depending on the configuration setting.
Feature | Description |
---|---|
Function Used | isCookieRestrictionModeEnabled() |
Return Type | Boolean (true or false ) |
Configuration Path | web/cookie/cookie_restriction |
Dependency | Magento\Cookie\Helper\Cookie |
Use Case | Verify if cookies should be restricted before setting them |
Step 1: Create a Custom Model Class
The following PHP class demonstrates how to inject the Cookie Helper and check if Cookie Restriction Mode is enabled.
PHP Code
<?php
namespace Vendor\Module\Model;
use Magento\Cookie\Helper\Cookie;
class CookieRestriction
{
/* @var Cookie */
private $cookieHelper;
/* CookieRestriction constructor./*
/* @param Cookie $cookieHelper /*
public function __construct(Cookie $cookieHelper)
{
$this->cookieHelper = $cookieHelper;
}
/* @return bool /*
public function isCookieRestrictionModeEnabled(): bool
{
return $this->cookieHelper->isCookieRestrictionModeEnabled();
}
}
>
Step 2: Use the Function in a Block or Controller
Now, you can use this function in a block, helper, or controller to check the cookie restriction mode before executing any cookie-dependent logic.
Example Code in Controller or Block
$cookieRestriction = $this->cookieRestriction->isCookieRestrictionModeEnabled();
if ($cookieRestriction) {
echo "Cookie Restriction Mode is ENABLED";
} else {
echo "Cookie Restriction Mode is DISABLED";
}
Tip
To prevent potential issues with session management, ensure that essential cookies (like authentication, cart, and checkout session cookies) are not blocked when Cookie Restriction Mode is enabled. You can configure this in Magento Admin Panel under: Stores > Configuration > General > Web > Default Cookie Settings.
Method 2: Fetch Value from Magento’s Configuration
In addition to using the Cookie Helper class, you can also programmatically fetch the cookie restriction mode value directly from Magento’s configuration using ScopeConfigInterface
. This approach is useful when you want to retrieve configuration settings dynamically from the store’s configuration.
Step 1: Create a Model Class Using ScopeConfigInterface
To implement this method, you'll need to inject the ScopeConfigInterface
into your custom model. Then, you can use it to fetch the value of the cookie_restriction
setting from the configuration.
Here’s the updated code for 2025, with a more detailed explanation:
<?php
namespace Vendor\Module\Model;
use Magento\Framework\App\Config\ScopeConfigInterface;
use Magento\Store\Model\ScopeInterface;
class CookieRestrictionChecker
{
/**
* @var ScopeConfigInterface
*/
private $scopeConfig;
/**
* CookieRestrictionChecker constructor.
*
* @param ScopeConfigInterface $scopeConfig
*/
public function __construct(ScopeConfigInterface $scopeConfig)
{
$this->scopeConfig = $scopeConfig;
}
/**
* Get cookie restriction mode value from configuration.
*
* Fetches the setting for cookie restriction mode, indicating whether cookies should be restricted until consent.
*
* @return bool
*/
public function isCookieRestrictionEnabled(): bool
{
return (bool) $this->scopeConfig->getValue(
'web/cookie/cookie_restriction', // Path to the cookie restriction setting
ScopeInterface::SCOPE_STORE // Scope of the configuration (store-level)
);
}
}
<?php
Explanation:
- ScopeConfigInterface: This interface provides a way to retrieve configuration values from Magento’s database. It's often used to fetch system configuration settings.
- ScopeInterface::SCOPE_STORE: The second parameter specifies the scope of the configuration value. In this case,
SCOPE_STORE
ensures that the setting is retrieved at the store level, which allows you to manage different configurations per store view if necessary. - Return Type: The function returns a
bool
value, wheretrue
means the cookie restriction mode is enabled, andfalse
means it is not.
Step 2: Use the Function in a Controller or Helper
Now that you have created the CookieRestrictionChecker
model, you can use the function isCookieRestrictionEnabled()
in your controllers, helpers, or anywhere you need to check if cookie restriction mode is enabled.
Here’s an example of using it in a controller:
<?php
$cookieRestriction = $this->cookieRestrictionChecker->isCookieRestrictionEnabled();
echo $cookieRestriction ? "Enabled" : "Disabled";
<?php
Table: Overview of the Method
Feature | Description |
---|---|
Function Used | isCookieRestrictionEnabled() |
Return Type | Boolean (true or false) |
Configuration Path | web/cookie/cookie_restriction |
Scope | ScopeInterface::SCOPE_STORE (store-level configuration) |
Dependency | Magento\Framework\App\Config\ScopeConfigInterface |
Use Case | Verify if the cookie restriction mode is enabled based on the store’s config |
Tip
Always ensure that the configuration value is fetched from the correct store scope to avoid conflicts across different views or websites. If you're working with multi-store setups, remember that each store view can have different settings, so ensure you're retrieving the correct setting at the right level (store, website, or global).
Additionally, be cautious about how caching behaves when fetching configuration values programmatically. Magento may cache these values for performance, so consider clearing the cache if you're testing changes.
Expected Output
When checking the cookie restriction mode programmatically, you can expect the following output depending on whether the mode is enabled or disabled:
Output Examples:
Cookie Restriction Mode is ENABLED
or
Cookie Restriction Mode is DISABLED
Tip
Always check the configuration setting before interacting with cookies, especially when Cookie Restriction Mode is enabled, to ensure that cookies are not set prematurely. This can prevent potential issues with user privacy and compliance with regulations like GDPR.
Comparison of Methods for Checking Cookie Restriction Mode
There are different ways to check the cookie restriction mode in Magento 2, each with its strengths and trade-offs. Below is a comparison of the three most common methods:
Method | Performance | Best Practice | Ease of Use |
---|---|---|---|
Using Cookie Helper Class | Fast | Recommended for direct programmatic access. | Easy – Simple function call to check mode. |
Using ScopeConfigInterface | Fast | Ideal for retrieving configuration values. | Requires more setup, involves additional classes. |
Checking Admin Panel | Manual | Good for quick checks and UI-based validation. | Simple to use but not automated. |
Using Dependency Injection (DI) | Fast | Best for complex implementations and flexibility. | Moderate, needs DI setup. |
Using Custom Observer | Moderate | Useful for event-based scenarios. | More complex – needs observer configuration. |
Method Breakdown
1. Using Cookie Helper Class
- Performance: Fast, as it directly uses Magento's helper class to check cookie restriction.
- Best Practice: Ideal for most use cases, especially when checking the cookie restriction mode programmatically in custom code. It abstracts away complexity and provides a straightforward solution.
- Ease of Use: Extremely easy, with minimal code required. The helper class provides a simple method to call that returns the required status.
2. Using ScopeConfigInterface
- Performance: Fast, as it retrieves the configuration value directly from Magento's configuration system.
- Best Practice: This method is excellent when you need to fetch configuration settings dynamically, especially in scenarios where the cookie restriction mode might differ across different stores.
- Ease of Use: Slightly more complex, as it requires the setup of ScopeConfigInterface and some configuration handling.
3. Checking Admin Panel
- Performance: Manual check, so it doesn’t apply to programmatic implementations but is useful for one-time checks or troubleshooting.
- Best Practice: Useful for quick verification of cookie restriction mode without needing to dig into the code. It provides a straightforward user interface to confirm the status.
- Ease of Use: Extremely easy for admin users but not automated, which makes it unsuitable for programmatic checks in production environments.
4. Using Dependency Injection (DI)
- Performance: Fast, as DI allows Magento to inject the necessary dependencies at runtime, minimizing overhead.
- Best Practice: Ideal for complex projects or when managing large codebases where dependencies need to be handled flexibly. DI ensures that the right class or helper is available where needed.
- Ease of Use: Moderate, as it involves setting up DI configuration and handling Magento's dependency injection framework.
5. Using Custom Observer
- Performance: Moderate, as it requires registering an observer and subscribing to the specific event to check the cookie restriction mode.
- Best Practice: Suitable for scenarios where you need to react to events and check the cookie restriction mode as part of the event-driven workflow in Magento.
- Ease of Use: Complex, as it requires setting up observers, events, and subscribing to them appropriately. This method is best used in more complex implementations.
Additional Considerations:
- Performance: All methods, except manual checks in the Admin Panel, offer fast performance, so performance concerns should not be the main deciding factor in your choice of method.
- Best Practice: The Cookie Helper Class is the recommended approach for most use cases because it's fast, easy, and fits well into Magento's ecosystem for checking cookie restriction mode.
- Ease of Use: Checking the Admin Panel is the simplest way for non-developers to verify the mode, but for automated or code-based solutions, using Cookie Helper Class or ScopeConfigInterface is the easiest and most efficient.
- Advanced Configurations: For more complex needs, such as handling different cookie settings for different stores or events, you may need to rely on ScopeConfigInterface or Custom Observers.
Tip
While the Cookie Helper Class is the most efficient and simplest for developers, the Admin Panel is useful for those who want a quick visual confirmation of the setting. For configuration-driven checks across multiple stores, ScopeConfigInterface will provide more flexibility.
Common Issues and Troubleshooting in Magento 2 Cookie Restriction Mode
Even though checking the cookie restriction mode in Magento 2 is straightforward, developers often encounter issues due to misconfigurations, caching, or dependency injection problems. Below is a detailed troubleshooting guide to help resolve common problems efficiently.
Troubleshooting:
Issue | Cause | Solution |
---|---|---|
Cookie restriction mode is not changing | Cache is enabled and old settings are being used. | Run bin/magento cache:flush and bin/magento cache:clean . Clear browser cache. |
Returns incorrect value | Store view settings override global settings. | Check the configuration at different levels: Store, Website, and Global in Stores > Configuration. |
Error: Undefined class CookieHelper |
Dependency injection (DI) issue or missing class in di.xml . |
Ensure that Magento\Cookie\Helper\Cookie is properly injected in your constructor. |
Error: Class ScopeConfigInterface not found |
Missing use statement or incorrect namespace. | Add use Magento\Framework\App\Config\ScopeConfigInterface; at the top of your file. |
Returns false even when enabled in the admin |
Incorrect retrieval method used (wrong scope). | Ensure ScopeInterface::SCOPE_STORE is used while retrieving values using ScopeConfigInterface . |
Cookie restriction banner not showing | Theme override or JavaScript issue. | Check if the theme overrides Magento’s default cookie consent banner. Inspect browser console for JS errors. |
Cookie banner appears even when restriction mode is disabled | JavaScript cache or third-party module conflict. | Disable third-party extensions related to cookies. Clear pub/static and var/cache . Run bin/magento setup:upgrade && bin/magento deploy:mode:set production . |
Debugging Tips :
Check Config via CLI:
Run this command to directly check the value from Magento’s configuration:
bin/magento config:show web/cookie/cookie_restriction
If the output is 1
, it means enabled; if 0
, it’s disabled.
Manually Verify in Database:
Run the following SQL query to check the configuration directly in the database:
SELECT * FROM core_config_data WHERE path = 'web/cookie/cookie_restriction';
If the value is 1
, the cookie restriction mode is enabled.
Best Practices for Handling Cookie Restriction Mode in Magento 2
1. Use Dependency Injection for Better Code Maintainability
- Always use dependency injection (DI) instead of direct
ObjectManager
calls. - Define dependencies in the constructor and let Magento’s DI container handle them.
- Avoid calling
ObjectManager
directly, as it violates Magento’s coding standards.
2. Ensure GDPR and Privacy Compliance
- Enable cookie consent in Magento 2 to comply with GDPR, CCPA, and other privacy regulations.
- Display clear and transparent cookie policies to users before setting non-essential cookies.
- Regularly review legal compliance requirements based on the target audience’s region.
3. Verify Cookie Settings Across Store Views
- If you run a multi-store Magento setup, verify that cookie restriction mode is enabled across all store views.
- Configuration path:
Stores > Configuration > General > Web > Default< Cookie Settings
. - Use ScopeConfigInterface to retrieve store-specific configurations.
4. Use Magento’s Built-in Cookie Consent Banner
- Magento provides a default cookie
consent banner
to manage user consent properly. - Customize the banner’s appearance and behavior to match your branding.
- Ensure JavaScript files related to cookie banners are not blocked or overridden by third-party themes.
5. Regularly Clear Cache After Updating Settings
- If cookie settings do not reflect immediately, run:
- Also, clear
browser cache
and test in an incognito window.
bin/magento cache:flush && bin/magento cache:clean
6. Use Logging for Debugging Cookie Issues
- Enable Magento logging to track potential cookie-related issues:
- This helps in debugging cases where cookie settings are inconsistent.
<?php
$writer = new \Zend\Log\Writer\Stream(BP . '/var/log/cookie_restriction.log');
$logger = new \Zend\Log\Logger();
$logger->addWriter($writer);
$logger->info('Cookie restriction mode status: ' . $status);
<?php
7. Test in Different Browsers and Devices
- Some browsers handle third-party cookies differently (e.g., Safari blocks third-party cookies by default).
- Test in multiple browsers and devices to ensure smooth operation.
8. Monitor Third-Party Modules That Affect Cookies
- Some third-party extensions might override or interfere with Magento’s default cookie handling.
- Disable extensions one by one and check if they impact cookie restriction behavior.
9. Keep Magento and Extensions Updated
- Outdated versions might introduce security risks and compliance issues.
- Always update Magento and third-party modules to ensure compatibility with the latest cookie policies.
10. Implement Server-Side Cookie Checks for Enhanced Security
- Validate cookies on the server-side for better security against cross-site scripting (XSS) attacks.
- Ensure sensitive user data is not stored in client-side cookies.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Conclusion
Managing cookie restriction mode in Magento 2 is essential for GDPR, CCPA, and other privacy law compliance. Whether you're checking the status programmatically, configuring it via the admin panel, or troubleshooting common issues, following best practices ensures a seamless and legally compliant user experience
By leveraging Magento’s built-in cookie helper, ScopeConfigInterface, and admin settings, you can efficiently control cookie behavior while maintaining site performance. Regularly clearing cache, testing across multiple store views, and monitoring third-party extensions can help prevent unexpected issues.
Security and compliance go hand in hand, so always ensure proper server-side validation, dependency injection, and user consent mechanisms. Keeping Magento updated and following a structured approach will help you maintain a secure, fast, and legally compliant online store.
With the right strategies in place, your Magento 2 store will be fully optimized to handle cookie restrictions efficiently, offering both performance and user trust.
FAQs
What is Cookie Restriction Mode in Magento 2?
Cookie Restriction Mode in Magento 2 ensures that cookies are only set once users consent to it, in compliance with privacy laws like GDPR.
How can I check if Cookie Restriction Mode is enabled in Magento 2?
You can check the status of Cookie Restriction Mode by checking the configuration setting `web/cookie/cookie_restriction` in the system configuration programmatically.
What is the default value of Cookie Restriction Mode?
By default, Cookie Restriction Mode is disabled in Magento 2. You need to enable it through the admin panel or programmatically.
How do I enable Cookie Restriction Mode in Magento 2?
You can enable Cookie Restriction Mode in Magento 2 by navigating to Stores > Configuration > Web > Default Cookie Settings, and setting "Cookie Restriction Mode" to Yes.
Can I check if Cookie Restriction Mode is enabled programmatically in Magento 2?
Yes, you can check it by using the `\Magento\Framework\App\Config\ScopeConfigInterface` to fetch the value of the `web/cookie/cookie_restriction` setting.
What is the role of the Cookie Restriction Mode?
The Cookie Restriction Mode restricts Magento from setting cookies before the user has consented to them, ensuring compliance with privacy regulations like GDPR.
Where can I find the Cookie Restriction Mode setting in the admin panel?
You can find it in the admin panel under Stores > Configuration > Web > Default Cookie Settings, where you can enable or disable it.
How can I disable Cookie Restriction Mode programmatically?
You can disable it by updating the `web/cookie/cookie_restriction` configuration setting to 'No' programmatically using the `ScopeConfigInterface`.
Can Cookie Restriction Mode affect the performance of my website?
Cookie Restriction Mode does not significantly impact website performance. It only limits the setting of non-essential cookies until consent is given.
Is Cookie Restriction Mode necessary for GDPR compliance in Magento 2?
Yes, enabling Cookie Restriction Mode is a crucial step for ensuring GDPR compliance in Magento 2, as it helps to manage user consent for cookies.
How do I check Cookie Restriction Mode status using code?
You can check the status programmatically using the following code: $cookieRestriction = $this->_scopeConfig->getValue('web/cookie/cookie_restriction');
.
Can I apply custom cookie consent logic along with Cookie Restriction Mode?
Yes, you can customize the logic for cookie consent by extending the default behavior of Cookie Restriction Mode through custom modules or plugins.
What happens if Cookie Restriction Mode is disabled in Magento 2?
If Cookie Restriction Mode is disabled, Magento 2 will set cookies as needed without waiting for user consent, which may not comply with GDPR or similar laws.
How do I check if a user has consented to cookies in Magento 2?
You can check if the user has consented to cookies using the `cookie_consent` cookie that Magento sets once the user provides consent.