Determining if a Product Has the 'links_exist' Attribute in Magento 2
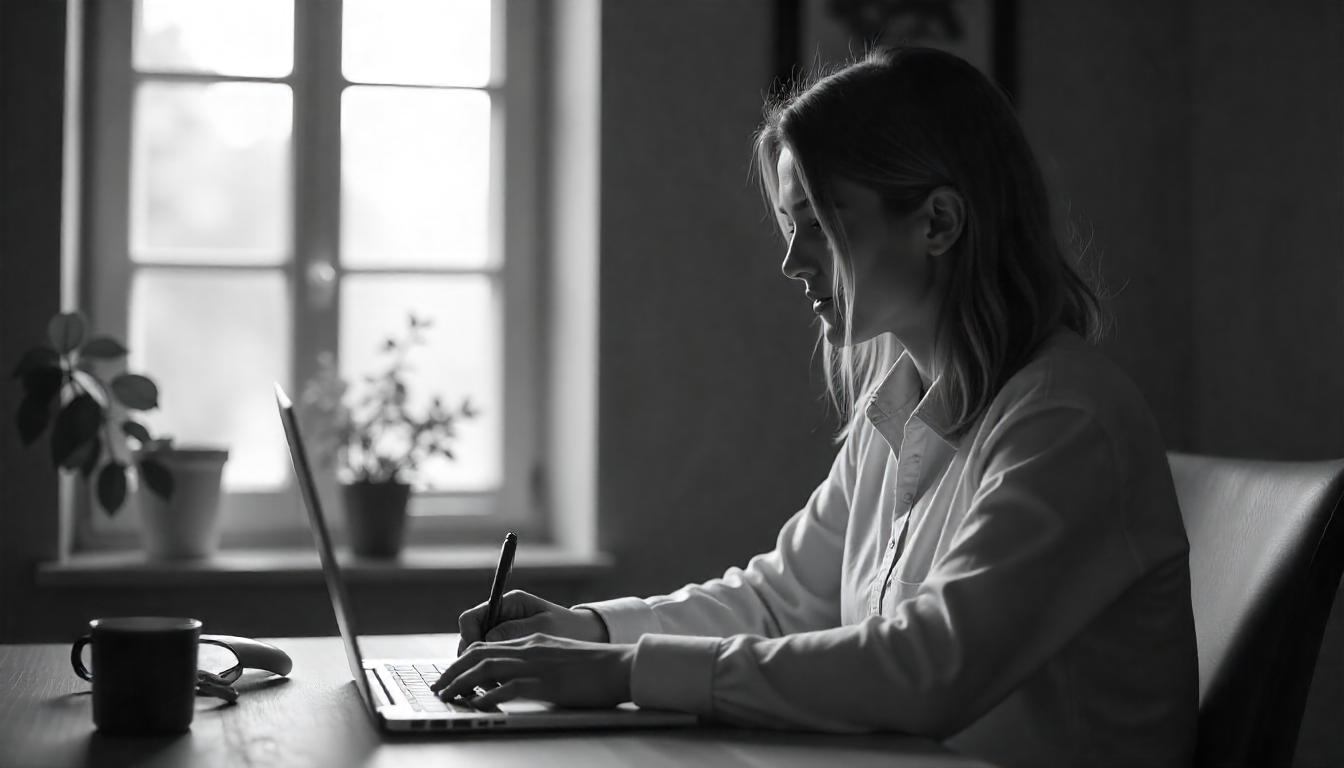
Determining if a Product Has the 'links_exist' Attribute in Magento 2
When working with Magento 2, it's essential to retrieve data from the database securely. Instead of using raw SQL queries, which can pose security risks, Magento 2 offers a standard method to perform select operations safely.
Determining if a Product Has the 'links_exist' Attribute in Magento 2
In Magento 2, the 'links_exist' attribute is specific to downloadable products. To programmatically check if a product possesses this attribute, you can utilize the Magento\Downloadable\Model\Product\Type
class. This approach ensures that your code accurately identifies the presence of downloadable links associated with a product.
Implementing the Check
Here's a straightforward method to determine if a product has associated downloadable links:
- Create a Custom Model Class: Develop a class that leverages dependency injection to access the necessary Magento components.
<?php
namespace YourNamespace\YourModule\Model;
use Magento\Catalog\Api\ProductRepositoryInterface;
use Magento\Downloadable\Model\Product\Type;
use Magento\Framework\Exception\NoSuchEntityException;
use Psr\Log\LoggerInterface;
class ProductLinksChecker
{
private $productRepository;
private $downloadableType;
private $logger;
public function __construct(
ProductRepositoryInterface $productRepository,
Type $downloadableType,
LoggerInterface $logger
) {
$this->productRepository = $productRepository;
$this->downloadableType = $downloadableType;
$this->logger = $logger;
}
public function hasDownloadableLinks(string $sku): bool
{
try {
$product = $this->productRepository->get($sku);
return $this->downloadableType->hasLinks($product);
} catch (NoSuchEntityException $e) {
$this->logger->error("Product with SKU {$sku} not found: " . $e->getMessage());
}
return $product;
}
}
Usage
: Invoke the hasDownloadableLinks method with the product's SKU to check for the presence of downloadable links.
$sku = 'your-product-sku';
$productLinksChecker = new \YourNamespace\YourModule\Model\ProductLinksChecker(
$productRepository,
$downloadableType,
$logger
);
$hasLinks = $productLinksChecker->hasDownloadableLinks($sku);
if ($hasLinks) {
// The product has downloadable links
} else {
// The product does not have downloadable links
}
Key Points
- Attribute Specificity: The 'links_exist' attribute is exclusive to downloadable products. Attempting to access this attribute on other product types will not yield meaningful results.
- Error Handling: The method includes a try-catch block to handle scenarios where a product with the specified SKU does not exist, ensuring that your application can gracefully handle such cases.
By following this approach, you can efficiently determine the presence of downloadable links for products in Magento 2, enhancing your store's functionality and user experience.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
What Is the 'links_exist' Attribute in Magento 2?
The 'links_exist' attribute in Magento 2 is specific to downloadable products. It indicates whether a product has associated downloadable links.
How Can I Check if a Product Has Downloadable Links?
You can check for downloadable links by using the Magento\Downloadable\Model\Product\Type
class and calling the hasLinks()
method on a product object.
What Code Can I Use to Verify Downloadable Links?
You can create a custom model class and use dependency injection to check for downloadable links. Here’s a basic example:
$product = $this->productRepository->get($sku);
$hasLinks = $this->downloadableType->hasLinks($product);
What Happens If the Product Does Not Exist?
If the product SKU is incorrect or does not exist, Magento will throw a NoSuchEntityException
. It's best to handle this error using a try-catch block.
Can the 'links_exist' Attribute Be Used for Non-Downloadable Products?
No, the 'links_exist' attribute is exclusive to downloadable products. Attempting to use it on other product types will not return meaningful results.
How Can I Log Errors When Checking Downloadable Links?
You can use the Psr\Log\LoggerInterface
to log errors when checking for downloadable links. This ensures you capture issues like missing SKUs or API failures.