How to Get Subcategory Details by Parent ID in Magento 2
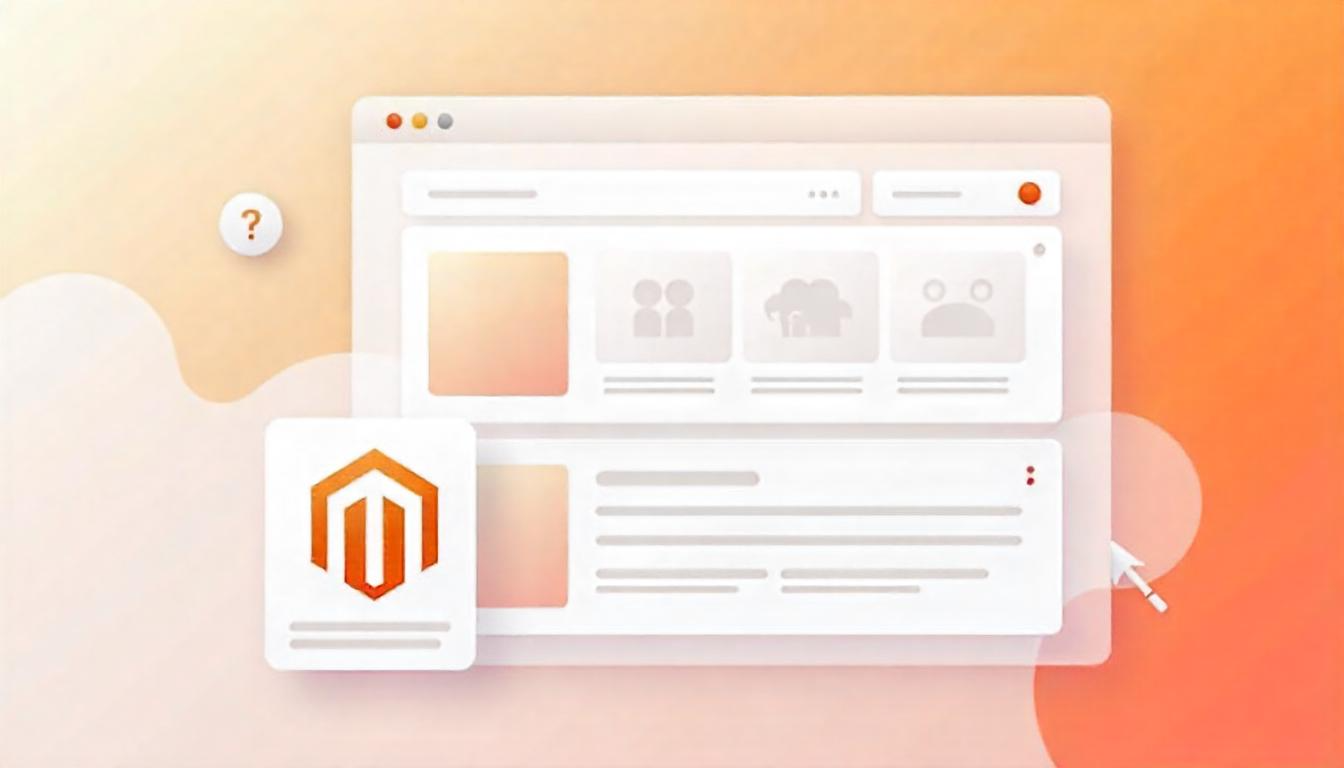
How to Get Subcategory Details by Parent ID in Magento 2
Retrieving subcategory details programmatically in Magento 2 is crucial for dynamic category navigation, SEO optimization, and structured product displays. By leveraging a parent category ID, you can efficiently fetch all child categories, including their names, URLs, images, descriptions, and metadata.
This method is especially useful when building custom mega menus, filtering products dynamically, or enhancing category-based landing pages. Below, we explore the best approaches to retrieve subcategories efficiently while following Magento’s best practices and performance optimizations.
By implementing these methods, you ensure a faster, scalable, and structured category retrieval process in Magento 2.
Table Of Content
Why Fetch Subcategories Programmatically in Magento 2?
Fetching subcategories programmatically in Magento 2 is a crucial practice for enhancing navigation, SEO, and store performance. By dynamically retrieving child categories, you can create custom mega menus, category-based landing pages, and structured product displays while ensuring speed, scalability, and multi-store compatibility.
Key Benefits of Fetching Subcategories Dynamically
Benefit | Description |
---|---|
Dynamic Navigation | Build interactive category menus that adapt to changes in the catalog. |
SEO Optimization | Generate SEO-friendly URLs, metadata, and breadcrumbs to boost search rankings. |
Custom Filtering | Display subcategories based on rules, such as product availability, promotions, or customer segments. |
Performance Boost | Load only necessary category data, reducing load times and optimizing queries. |
Multi-Store Support | Fetch subcategories dynamically for different store views and languages, ensuring localized navigation. |
Pro Tips for Efficient Subcategory Retrieval
Use Indexing: Instead of fetching categories on every page load, cache results for faster performance.
Avoid Direct Database Queries: Always use Magento’s CategoryRepositoryInterface or CategoryFactory for structured data retrieval.
Leverage Flat Catalog Categories: If performance is a concern, enable Flat Catalog Category for better query optimization.
implement Lazy Loading: For large category trees, use AJAX-based lazy loading to improve UX and reduce initial page load time.
Ensure Multi-Store Compatibility: When fetching subcategories, always consider store scope (ScopeInterface::SCOPE_STORE
) for accurate results.
Understanding Magento 2 Category Hierarchy
Magento 2 organizes products using a structured category hierarchy to ensure efficient navigation, product filtering, and SEO-friendly URLs. This hierarchical structure enables merchants to manage large catalogs while maintaining a seamless shopping experience.
Magento 2 Category Structure Overview
1. Root Category (Level 1)
- The highest level in the hierarchy.
- Acts as a container for all other categories.
- Each store in Magento 2 must have a single root category.
- It is not directly visible in the frontend.
2. Main Categories (Level 2)
- Direct child categories of the root category.
- Typically represent broad product categories like Men, Women, Electronics, Home & Living.
- Visible in the main navigation menu.
3. Subcategories (Level 3 & Beyond)
- Nested categories under main categories.
- Used to further classify products into more specific sections.
- Example:
- Electronics → Laptops → Gaming Laptops
- Men → Clothing → Jackets & Coats
- Subcategories can have multiple levels, allowing deep categorization.
Magento 2 Category Hierarchy Example
Category Level | Example | Visibility |
---|---|---|
Root Category (Level 1) | "Default Category" (Container for all) | Not visible in frontend |
Main Category (Level 2) | "Electronics", "Fashion", "Home & Kitchen" | Visible in navigation |
Subcategory (Level 3) | "Laptops", "Men’s Clothing", "Kitchen Appliances" | Visible in navigation & filters |
Sub-Subcategory (Level 4+) | "Gaming Laptops", "Jackets & Coats", "Coffee Makers" | Visible in filters & layered navigation |
Key Features of Magento 2 Category Structure
- SEO Benefits: Allows better URL structuring and breadcrumb navigation.
- User Experience: Helps customers find products quickly with a well-organized menu.
- Custom Sorting & Display: Categories can be manually sorted or set to auto-arrange.
- Multi-Store Support: Each store view can have its own category structure.
- Product Assignments: Products can belong to multiple categories for better visibility.
Tips for Optimizing Magento 2 Category Hierarchy
Keep It Simple
Avoid excessive category depth to maintain a user-friendly and fast-loading navigation experience. Stick to a logical structure with minimal sub-levels.
Use SEO-Optimized Category Names
Incorporate relevant keywords naturally in category names to enhance search engine rankings and improve discoverability.
Enable Layered Navigation
Activate filters based on product attributes like brand, price, and features to enhance user experience and make product discovery seamless.
Optimize Category Pages
Enrich category pages with compelling descriptions, high-quality images, and well-structured metadata to boost engagement and conversions.
Ensure Clean URL Structure
Use short, meaningful, and SEO-friendly URLs like /electronics/laptops/gaming-laptops instead of long, confusing ones. Avoid special characters and redundant words.
Implement Breadcrumbs
Breadcrumb navigation helps users understand their location within the store and improves internal linking for SEO.
Regularly Audit & Clean Up Categories
Periodically review and remove outdated or redundant categories to keep the structure organized and efficient.
Pro Tip:
Keep your category hierarchy shallow and intuitive—limit depth to 3-4 levels for better UX, faster navigation, and improved SEO rankings.
How to Get Subcategory Details Programmatically in Magento 2
Fetching subcategory details dynamically in Magento 2 can be useful when creating custom navigation menus, filtering products, or enhancing user experience. Below is a step-by-step guide to retrieving subcategories programmatically.
Step 1: Create a Custom Module (If Needed)
If you don't already have a module, create one by following these steps:
1.1 Create module.xml
File: app/code/Vendor/Module/etc/module.xml
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:noNamespaceSchemaLocation="urn:magento:framework:Module/etc/module.xsd">
<module name="Vendor_Module" setup_version="1.0.0"/>
</config>
1.2 Register the Module
File: app/code/Vendor/Module/registration.php
<?php
\Magento\Framework\Component\ComponentRegistrar::register(
\Magento\Framework\Component\ComponentRegistrar::MODULE,
'Vendor_Module',
__DIR__
);
1.3 Enable and Install the Module
Run the following commands:
bin/magento module:enable Vendor_Module
bin/magento setup:upgrade
bin/magento cache:flush
Step 2: Implement the Block Class to Fetch Subcategories
Now, create a block class to fetch subcategory details using CategoryManagementInterface
.
2.1 Create the Block File
File: app/code/Vendor/Module/Block/SubCategory.php
<?php
namespace Vendor\Module\Block;
use Magento\Catalog\Api\CategoryManagementInterface;
use Magento\Framework\Exception\NoSuchEntityException;
use Magento\Framework\View\Element\Template;
use Magento\Framework\View\Element\Template\Context;
use Psr\Log\LoggerInterface;
class SubCategory extends Template
{
private $logger;
private $categoryManagement;
public function __construct(
Context $context,
CategoryManagementInterface $categoryManagement,
LoggerInterface $logger,
array $data = []
) {
$this->categoryManagement = $categoryManagement;
$this->logger = $logger;
parent::__construct($context, $data);
}
public function getSubCategoryByParentID(int $categoryId): array
{
$categoryData = [];
try {
$getSubCategory = $this->categoryManagement->getTree($categoryId);
foreach ($getSubCategory->getChildrenData() as $category) {
$categoryData[$category->getId()] = [
'name' => $category->getName(),
'url' => $category->getUrl()
];
// Fetch deeper subcategories
if (!empty($category->getChildrenData())) {
foreach ($category->getChildrenData() as $subcategory) {
$categoryData[$subcategory->getId()] = [
'name' => $subcategory->getName(),
'url' => $subcategory->getUrl()
];
}
}
}
} catch (NoSuchEntityException $e) {
$this->logger->error("Category not found", [$e]);
}
return $categoryData;
}
}
Step 3: Call the Block Method in Your Template File
Now, create a .phtml
file to display subcategories dynamically.
3.1 Create the Template File
File: app/code/Vendor/Module/view/frontend/templates/subcategories.phtml
<?php
$parentID = 11; // Example: Parent Category ID
$getCategoryList = $this->getSubCategoryByParentID($parentID);
?>
<?php if (!empty($getCategoryList)) { ?>
<ul class="subcategory-list">
<?php foreach ($getCategoryList as $id => $category) : ?>
<li>
<a href="<?= $category['url'] ?>" title="<?= $category['name']; ?>">
<?= $category['name']; ?>
</a>
</li>
<?php endforeach; ?>
</ul>
<?php } else { ?>
<p>No subcategories found.</p>
<?php } ?>
Step 4: Add the Block to a Layout File
Define the block in the catalog_category_view.xml
layout file.
4.1 Create the Layout File
File: app/code/Vendor/Module/view/frontend/layout/catalog_category_view.xml
<?xml version="1.0"?>
<page xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:noNamespaceSchemaLocation="urn:magento:framework:View/Layout/etc/page_configuration.xsd">
<body>
<referenceContainer name="content">
<block class="Vendor\Module\Block\SubCategory"
name="subcategory.list"
template="Vendor_Module::subcategories.phtml"/>
</referenceContainer>
</body>
</page>
Step 5: Flush Cache and Test
Run the following commands to clear cache and check the results on the frontend:
bin/magento cache:flush
bin/magento cache:clean
Now, navigate to any category page (e.g., yourstore.com/category-name.html
), and you should see the list of subcategories displayed dynamically.
Subcategory Data Structure (Example Output)
Attribute | Description |
---|---|
ID | Unique identifier of the category |
Name | Category name |
URL | SEO-friendly category URL |
Is Active | Indicates if the category is enabled/visible |
Image | URL of the category image |
Position | Sorting order in the navigation |
Pro Tip:
To improve performance, cache subcategory data using Magento’s built-in caching mechanism and avoid fetching data on every page load.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
Conclusion
Fetching subcategory details by parent ID in Magento 2 is an essential skill for developers looking to enhance category-based navigation, optimize store organization, and improve user experience. Whether you're customizing menus, creating dynamic category pages, or filtering products, leveraging Magento’s built-in repository and factories allows for efficient data retrieval.
By following best practices like using dependency injection instead of the object manager, caching results for better performance, and ensuring proper indexing, you can make your Magento store more scalable and SEO-friendly. Implementing these techniques not only enhances functionality but also contributes to a seamless shopping experience.
Stay ahead by continuously refining your category structure and optimizing your code for speed and efficiency. A well-structured navigation system not only boosts user engagement but also strengthens your store’s search engine visibility.
FAQs
How do I get subcategories by parent ID in Magento 2?
You can retrieve subcategories using Magento’s Category Repository and filtering by the parent category ID.
Which method is best for fetching subcategories in Magento 2?
Using dependency injection with the Category Repository is the best approach for performance and maintainability.
Can I get subcategory details without using Object Manager?
Yes, it’s recommended to use dependency injection instead of Object Manager for better code structure and performance.
How can I display subcategories in a custom menu?
You can loop through the subcategories retrieved via the Category Repository and render them in your custom navigation template.
How do I get subcategory URLs in Magento 2?
You can use the getUrl() method on the category model to fetch the subcategory URLs.
Can I get subcategories in GraphQL?
Yes, Magento 2 provides GraphQL queries to fetch category details, including subcategories.
Is it possible to filter subcategories by attributes?
Yes, you can filter subcategories by attributes such as visibility, status, and custom attributes using Magento’s repository and filters.
Why are my subcategories not appearing?
Ensure the subcategories are set to 'Enabled,' visible in the store view, and properly assigned to a parent category.
How can I improve the performance of subcategory retrieval?
Caching the results, limiting attribute selection, and using indexed queries help improve performance.
Can I retrieve subcategories in a custom Magento 2 module?
Yes, you can use the Category Repository in your custom module to fetch and display subcategory details.
How do I sort subcategories by position?
You can sort subcategories using the ‘position’ attribute while retrieving them through the Category Repository.
How do I get subcategories programmatically in a phtml file?
You can use the Category Repository in your block class and pass the data to the phtml file for rendering.
How do I exclude empty categories from the subcategory list?
Filter out subcategories that have zero products by checking the category’s product count before displaying them.