Managing Downloadable Product Links in Magento 2
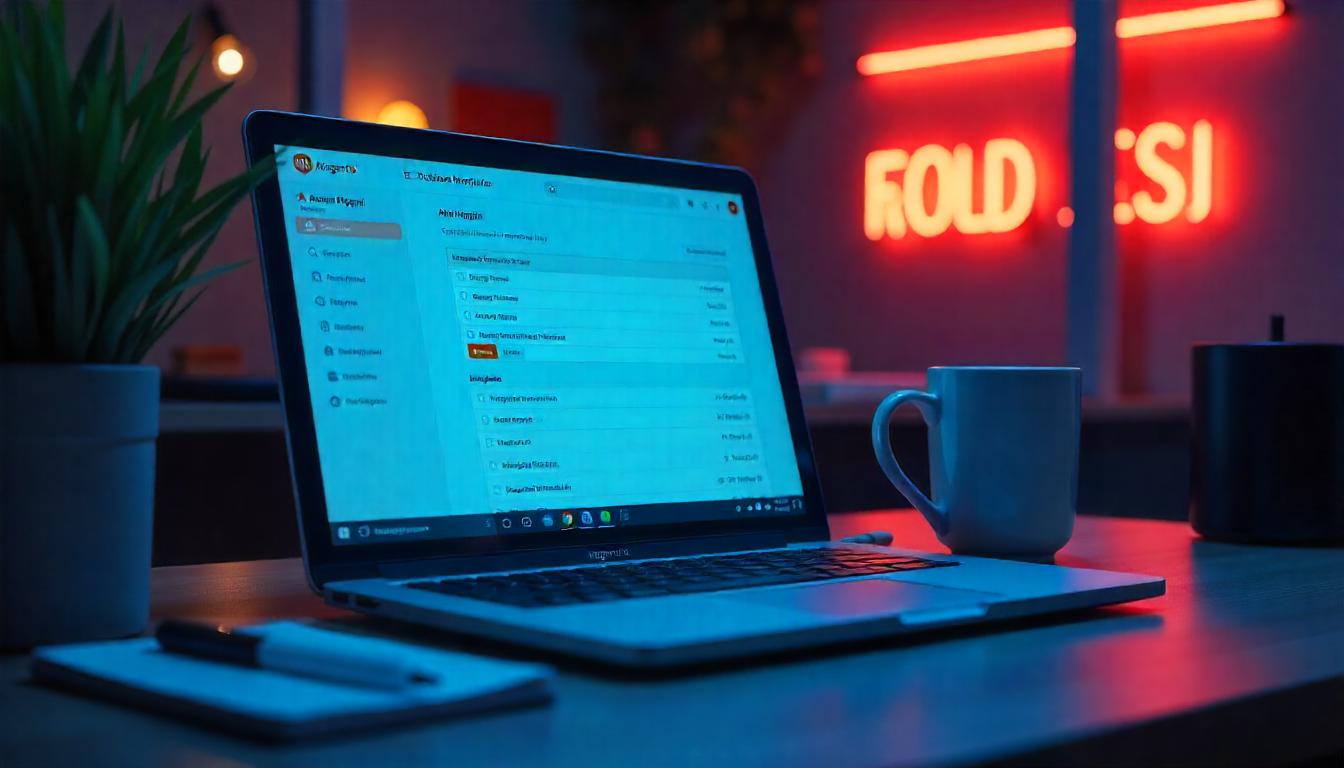
Managing Downloadable Product Links in Magento 2
In Magento 2, managing downloadable product links is an essential task for store owners offering digital products. These links allow customers to download files such as software, eBooks, music, or images after a successful purchase. Efficiently handling these links ensures a smooth customer experience and helps maintain accurate product data.
Table Of Content
Managing Downloadable Product Links in Magento 2
In Magento 2, you can programmatically delete downloadable product links using their link IDs. This is useful when you need to remove specific download links without affecting the entire product.
Understanding Downloadable Product Links
Downloadable products in Magento 2 allow customers to purchase and download items like e-books, music, or software. Each downloadable product can have multiple links associated with it, such as the main product file and sample files.
Deleting Downloadable Links by ID
To delete a downloadable link by its ID, follow these steps:
- Create a Model to Handle Deletion
Define a model that will manage the deletion process.
<?php
namespace YourNamespace\DeleteDownloadableLinks\Model;
use Magento\Downloadable\Api\LinkRepositoryInterface;
use Psr\Log\LoggerInterface;
use Magento\Framework\Exception\NoSuchEntityException;
class DeleteLinksByLinkId
{
private $linkRepository;
private $logger;
public function __construct(
LinkRepositoryInterface $linkRepository,
LoggerInterface $logger
) {
$this->linkRepository = $linkRepository;
$this->logger = $logger;
}
public function deleteLinks(int $linkId): bool
{
try {
$this->linkRepository->deleteById($linkId);
return true;
} catch (NoSuchEntityException $exception) {
$this->logger->error($exception->getMessage());
return false;
}
}
}
- Use the Model to Delete a Link
Invoke the deleteLinks method with the specific link ID you wish to remove.
$linkId = 1; // Replace with the actual link ID
$deleteLinksModel = $this->objectManager->create('YourNamespace\DeleteDownloadableLinks\Model\DeleteLinksByLinkId');
$isDeleted = $deleteLinksModel->deleteLinks($linkId);
Handling Errors
If the link ID does not exist, a NoSuchEntityException
will be thrown. Ensure you handle this exception to maintain smooth operation.
Additional Considerations
- Sample Files: If you're also managing sample files for downloadable products, you can delete them using a similar approach by interacting with the
SampleRepositoryInterface
. - Product Updates: When updating downloadable product links, ensure that the new links are correctly associated with the product to prevent any issues.
By following these steps, you can effectively manage and delete downloadable product links in Magento 2 programmatically.
Tip
To enhance your eCommerce store’s performance with Magento, focus on optimizing site speed by utilizing Emmo themes and extensions. These tools are designed for efficiency, ensuring your website loads quickly and provides a smooth user experience. Start leveraging Emmo's powerful solutions today to boost customer satisfaction and drive sales!
FAQs
How Do I Delete Downloadable Product Links in Magento 2?
You can delete downloadable product links programmatically using the LinkRepositoryInterface
and calling the deleteById()
method with the specific link ID.
What Is the Process to Delete a Downloadable Link by ID?
To delete a downloadable link by ID, create a model that utilizes the LinkRepositoryInterface
to delete the link using the deleteById()
method.
Can I Delete a Link by SKU Instead of Link ID?
While deleting by link ID is the most direct method, you can first retrieve the product by SKU and then obtain the associated link IDs before performing the deletion.
What Is the Code to Delete a Downloadable Link Programmatically?
You can use the following PHP code to delete a downloadable link by ID:
$linkId = 1; // Replace with the actual link ID
$deleteLinksModel = $this->objectManager->create('YourNamespace\DeleteDownloadableLinks\Model\DeleteLinksByLinkId');
$isDeleted = $deleteLinksModel->deleteLinks($linkId);
What If the Link ID Does Not Exist?
If the provided link ID does not exist, a NoSuchEntityException
will be thrown. It's important to handle this exception properly in your code.
How Can I Log Errors When Deleting Downloadable Links?
You can use Psr\Log\LoggerInterface
to log any errors that occur during the deletion process, such as invalid link IDs or other issues.